Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Recognition / SrgsGrammar / SrgsToken.cs / 1 / SrgsToken.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 5/1/2004 jeanfp Created from the Kurosawa Code //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Speech.Internal; using System.Speech.Internal.SrgsParser; using System.Text; using System.Xml; namespace System.Speech.Recognition.SrgsGrammar { /// TODOC <_include file='doc\Token.uex' path='docs/doc[@for="Token"]/*' /> // Note that currently if multiple words are stored in a Token they are treated internally // and in the result as multiple tokens. [Serializable] [DebuggerDisplay ("{DebuggerDisplayString ()}")] public class SrgsToken : SrgsElement, IToken { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors /// TODOC <_include file='doc\Token.uex' path='docs/doc[@for="Token.Token2"]/*' /> public SrgsToken (string text) { Helpers.ThrowIfEmptyOrNull (text, "text"); Text = text; } #endregion //******************************************************************** // // Public Properties // //******************************************************************* #region public Properties /// TODOC <_include file='doc\Token.uex' path='docs/doc[@for="Token.Text"]/*' /> public string Text { get { return _text; } set { Helpers.ThrowIfEmptyOrNull (value, "value"); // remove all spaces if any string text = value.Trim (Helpers._achTrimChars); if (string.IsNullOrEmpty (text) || text.IndexOf ('\"') >= 0) { throw new ArgumentException (SR.Get (SRID.InvalidTokenString), "value"); } _text = text; } } /// TODOC <_include file='doc\Token.uex' path='docs/doc[@for="Token.Pronunciation"]/*' /> public string Pronunciation { get { return _pronunciation; } set { Helpers.ThrowIfEmptyOrNull (value, "value"); _pronunciation = value; } } /// TODOC <_include file='doc\Token.uex' path='docs/doc[@for="Token.Display"]/*' /> public string Display { get { return _display; } set { Helpers.ThrowIfEmptyOrNull (value, "value"); _display = value; } } #endregion //******************************************************************** // // Internal methods // //******************************************************************** #region Internal methods internal override void WriteSrgs (XmlWriter writer) { // Write_text writer.WriteStartElement ("token"); if (_display != null) { writer.WriteAttributeString ("sapi", "display", XmlParser.sapiNamespace, _display); } if (_pronunciation != null) { writer.WriteAttributeString ("sapi", "pron", XmlParser.sapiNamespace, _pronunciation); } // If an empty string is provided, skip the WriteString // to have the XmlWrite to putrather than if (_text != null && _text.Length > 0) { writer.WriteString (_text); } writer.WriteEndElement (); } internal override void Validate (SrgsGrammar grammar) { if (_pronunciation != null || _display != null) { grammar.HasPronunciation = true; } // Validate the pronunciation if any if (_pronunciation != null) { for (int iCurPron = 0, iDeliminator = 0; iCurPron < _pronunciation.Length; iCurPron = iDeliminator + 1) { // Find semi-colon deliminator and replace with null iDeliminator = _pronunciation.IndexOf (';', iCurPron); if (iDeliminator == -1) { iDeliminator = _pronunciation.Length; } string subPronunciation = _pronunciation.Substring (iCurPron, iDeliminator - iCurPron); // Convert the pronunciation, will throw if error switch (grammar.PhoneticAlphabet) { case AlphabetType.Sapi: PhonemeConverter.ConvertPronToId (subPronunciation, grammar.Culture.LCID); break; case AlphabetType.Ups: PhonemeConverter.UpsConverter.ConvertPronToId (subPronunciation); break; case AlphabetType.Ipa: PhonemeConverter.ValidateUpsIds (subPronunciation.ToCharArray ()); break; } } } base.Validate (grammar); } internal override string DebuggerDisplayString () { StringBuilder sb = new StringBuilder ("Token '"); sb.Append (_text); sb.Append ("'"); if (_pronunciation != null) { sb.Append (" Pronunciation '"); sb.Append (_pronunciation); sb.Append ("'"); } return sb.ToString (); } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private string _text = string.Empty; private string _pronunciation; private string _display; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 5/1/2004 jeanfp Created from the Kurosawa Code //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Speech.Internal; using System.Speech.Internal.SrgsParser; using System.Text; using System.Xml; namespace System.Speech.Recognition.SrgsGrammar { /// TODOC <_include file='doc\Token.uex' path='docs/doc[@for="Token"]/*' /> // Note that currently if multiple words are stored in a Token they are treated internally // and in the result as multiple tokens. [Serializable] [DebuggerDisplay ("{DebuggerDisplayString ()}")] public class SrgsToken : SrgsElement, IToken { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors /// TODOC <_include file='doc\Token.uex' path='docs/doc[@for="Token.Token2"]/*' /> public SrgsToken (string text) { Helpers.ThrowIfEmptyOrNull (text, "text"); Text = text; } #endregion //******************************************************************** // // Public Properties // //******************************************************************* #region public Properties /// TODOC <_include file='doc\Token.uex' path='docs/doc[@for="Token.Text"]/*' /> public string Text { get { return _text; } set { Helpers.ThrowIfEmptyOrNull (value, "value"); // remove all spaces if any string text = value.Trim (Helpers._achTrimChars); if (string.IsNullOrEmpty (text) || text.IndexOf ('\"') >= 0) { throw new ArgumentException (SR.Get (SRID.InvalidTokenString), "value"); } _text = text; } } /// TODOC <_include file='doc\Token.uex' path='docs/doc[@for="Token.Pronunciation"]/*' /> public string Pronunciation { get { return _pronunciation; } set { Helpers.ThrowIfEmptyOrNull (value, "value"); _pronunciation = value; } } /// TODOC <_include file='doc\Token.uex' path='docs/doc[@for="Token.Display"]/*' /> public string Display { get { return _display; } set { Helpers.ThrowIfEmptyOrNull (value, "value"); _display = value; } } #endregion //******************************************************************** // // Internal methods // //******************************************************************** #region Internal methods internal override void WriteSrgs (XmlWriter writer) { // Write_text writer.WriteStartElement ("token"); if (_display != null) { writer.WriteAttributeString ("sapi", "display", XmlParser.sapiNamespace, _display); } if (_pronunciation != null) { writer.WriteAttributeString ("sapi", "pron", XmlParser.sapiNamespace, _pronunciation); } // If an empty string is provided, skip the WriteString // to have the XmlWrite to putrather than if (_text != null && _text.Length > 0) { writer.WriteString (_text); } writer.WriteEndElement (); } internal override void Validate (SrgsGrammar grammar) { if (_pronunciation != null || _display != null) { grammar.HasPronunciation = true; } // Validate the pronunciation if any if (_pronunciation != null) { for (int iCurPron = 0, iDeliminator = 0; iCurPron < _pronunciation.Length; iCurPron = iDeliminator + 1) { // Find semi-colon deliminator and replace with null iDeliminator = _pronunciation.IndexOf (';', iCurPron); if (iDeliminator == -1) { iDeliminator = _pronunciation.Length; } string subPronunciation = _pronunciation.Substring (iCurPron, iDeliminator - iCurPron); // Convert the pronunciation, will throw if error switch (grammar.PhoneticAlphabet) { case AlphabetType.Sapi: PhonemeConverter.ConvertPronToId (subPronunciation, grammar.Culture.LCID); break; case AlphabetType.Ups: PhonemeConverter.UpsConverter.ConvertPronToId (subPronunciation); break; case AlphabetType.Ipa: PhonemeConverter.ValidateUpsIds (subPronunciation.ToCharArray ()); break; } } } base.Validate (grammar); } internal override string DebuggerDisplayString () { StringBuilder sb = new StringBuilder ("Token '"); sb.Append (_text); sb.Append ("'"); if (_pronunciation != null) { sb.Append (" Pronunciation '"); sb.Append (_pronunciation); sb.Append ("'"); } return sb.ToString (); } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private string _text = string.Empty; private string _pronunciation; private string _display; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
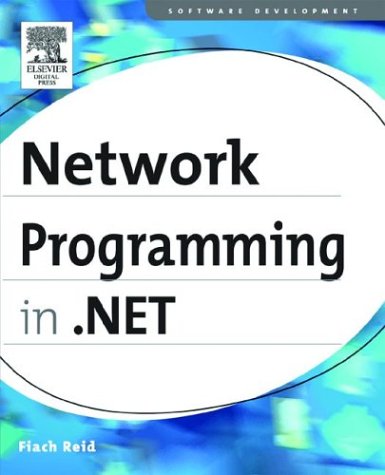
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExceptionHelpers.cs
- returneventsaver.cs
- GridViewRowEventArgs.cs
- HtmlPanelAdapter.cs
- WebRequest.cs
- HeaderUtility.cs
- PartialTrustHelpers.cs
- SaveWorkflowCommand.cs
- MappingException.cs
- FontStyle.cs
- SecurityContextCookieSerializer.cs
- QueryCacheManager.cs
- CodeAttributeDeclarationCollection.cs
- Metafile.cs
- MachineKeySection.cs
- OutputCacheSettingsSection.cs
- TypeKeyValue.cs
- PngBitmapDecoder.cs
- MarkupCompiler.cs
- Select.cs
- XmlWellformedWriterHelpers.cs
- KerberosRequestorSecurityToken.cs
- UriScheme.cs
- OracleInfoMessageEventArgs.cs
- RepeaterCommandEventArgs.cs
- SaveRecipientRequest.cs
- RuntimeConfig.cs
- Duration.cs
- AmbiguousMatchException.cs
- UnsafeNativeMethods.cs
- BuildProviderCollection.cs
- SmiEventSink.cs
- CodeBlockBuilder.cs
- EntityTypeEmitter.cs
- HtmlControl.cs
- ConvertEvent.cs
- TableRow.cs
- TextBreakpoint.cs
- EditingCoordinator.cs
- PiiTraceSource.cs
- RIPEMD160.cs
- WebControlsSection.cs
- JournalNavigationScope.cs
- EFColumnProvider.cs
- TemplateEditingFrame.cs
- InfiniteTimeSpanConverter.cs
- ControlCachePolicy.cs
- UIElementIsland.cs
- FactoryGenerator.cs
- StylusPointPropertyInfo.cs
- ModuleBuilderData.cs
- WriteTimeStream.cs
- MenuItem.cs
- ComboBox.cs
- IApplicationTrustManager.cs
- SqlNodeAnnotation.cs
- SwitchLevelAttribute.cs
- lengthconverter.cs
- ContainerParagraph.cs
- ToolZoneDesigner.cs
- TypedAsyncResult.cs
- InteropExecutor.cs
- BindingContext.cs
- TraceUtils.cs
- EdmProperty.cs
- MembershipUser.cs
- Propagator.ExtentPlaceholderCreator.cs
- FileSecurity.cs
- HostingEnvironmentSection.cs
- XsdBuildProvider.cs
- ILGenerator.cs
- XPathSingletonIterator.cs
- _emptywebproxy.cs
- IPEndPointCollection.cs
- PnrpPeerResolverBindingElement.cs
- SymbolType.cs
- TriggerCollection.cs
- QueryExpr.cs
- mediaclock.cs
- RunClient.cs
- SelectionChangedEventArgs.cs
- Polyline.cs
- HighContrastHelper.cs
- GridViewUpdatedEventArgs.cs
- EDesignUtil.cs
- WebPartManager.cs
- PropertyTabChangedEvent.cs
- URLMembershipCondition.cs
- DesignerActionItemCollection.cs
- Schema.cs
- IItemProperties.cs
- ItemsChangedEventArgs.cs
- StrongNameIdentityPermission.cs
- SoapFormatter.cs
- PeerNeighborManager.cs
- Certificate.cs
- CorrelationManager.cs
- TypefaceMetricsCache.cs
- HttpCookiesSection.cs
- Column.cs