Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / UI / WebControls / Unit.cs / 1 / Unit.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Globalization; using System.ComponentModel; using System.ComponentModel.Design; using System.Security.Permissions; using System.Web.Util; [ TypeConverterAttribute(typeof(UnitConverter)) ] [Serializable] public struct Unit { ////// Specifies an empty unit. /// public static readonly Unit Empty = new Unit(); internal const int MaxValue = 32767; internal const int MinValue = -32768; private readonly UnitType type; private readonly double value; ////// public Unit(int value) { if ((value < MinValue) || (value > MaxValue)) { throw new ArgumentOutOfRangeException("value"); } this.value = value; this.type = UnitType.Pixel; } ///Initializes a new instance of the ///structure with the specified 32-bit signed integer as /// the unit value and as the (default) unit type. /// public Unit(double value) { if ((value < MinValue) || (value > MaxValue)) { throw new ArgumentOutOfRangeException("value"); } this.value = (int)value; this.type = UnitType.Pixel; } ///Initializes a new instance of the ///structure with the /// specified double-precision /// floating point number as the unit value and /// as the (default) unit type. /// public Unit(double value, UnitType type) { if ((value < MinValue) || (value > MaxValue)) { throw new ArgumentOutOfRangeException("value"); } if (type == UnitType.Pixel) { this.value = (int)value; } else { this.value = value; } this.type = type; } ///Initializes a new instance of the ///structure with the specified /// double-precision floating point number as the unit value and the specified /// as the unit type. /// public Unit(string value) : this(value, CultureInfo.CurrentCulture, UnitType.Pixel) { } ///Initializes a new instance of the ///structure with the specified text /// string that contains the unit value and unit type. If the unit type is not /// specified, the default is /// . /// public Unit(string value, CultureInfo culture) : this(value, culture, UnitType.Pixel) { } internal Unit(string value, CultureInfo culture, UnitType defaultType) { if (String.IsNullOrEmpty(value)) { this.value = 0; this.type = (UnitType)0; } else { if (culture == null) { culture = CultureInfo.CurrentCulture; } // This is invariant because it acts like an enum with a number together. // The enum part is invariant, but the number uses current culture. string trimLcase = value.Trim().ToLower(CultureInfo.InvariantCulture); int len = trimLcase.Length; int lastDigit = -1; for (int i = 0; i < len; i++) { char ch = trimLcase[i]; if (((ch < '0') || (ch > '9')) && (ch != '-') && (ch != '.') && (ch != ',')) break; lastDigit = i; } if (lastDigit == -1) { throw new FormatException(SR.GetString(SR.UnitParseNoDigits, value)); } if (lastDigit < len - 1) { type = (UnitType)GetTypeFromString(trimLcase.Substring(lastDigit+1).Trim()); } else { type = defaultType; } string numericPart = trimLcase.Substring(0, lastDigit+1); // Cannot use Double.FromString, because we don't use it in the ToString implementation try { TypeConverter converter = new SingleConverter(); this.value = (Single)converter.ConvertFromString(null, culture, numericPart); if (type == UnitType.Pixel) { this.value = (int)this.value; } } catch { throw new FormatException(SR.GetString(SR.UnitParseNumericPart, value, numericPart, type.ToString("G"))); } if ((this.value < MinValue) || (this.value > MaxValue)) { throw new ArgumentOutOfRangeException("value"); } } } ///[To be supplied.] ////// public bool IsEmpty { get { return type == (UnitType)0; } } ///Gets a value indicating whether the ///is empty. /// public UnitType Type { get { if (!IsEmpty) { return this.type; } else { return UnitType.Pixel; } } } ///Gets or sets the type of the ///. /// public double Value { get { return this.value; } } ///Gets the value of the ///. /// public override int GetHashCode() { return HashCodeCombiner.CombineHashCodes(type.GetHashCode(), value.GetHashCode()); } ///[To be supplied.] ////// public override bool Equals(object obj) { if (obj == null || !(obj is Unit)) { return false; } Unit u = (Unit)obj; // compare internal values to avoid "defaulting" in the case of "Empty" // if (u.type == type && u.value == value) { return true; } return false; } ///Compares this ///with the specified object. /// public static bool operator ==(Unit left, Unit right) { // compare internal values to avoid "defaulting" in the case of "Empty" // return (left.type == right.type && left.value == right.value); } ///Compares two units to find out if they have the same value and type. ////// public static bool operator !=(Unit left, Unit right) { // compare internal values to avoid "defaulting" in the case of "Empty" // return (left.type != right.type || left.value != right.value); } ///Compares two units to find out if they have different /// values and/or types. ////// Converts UnitType to persistence string. /// private static string GetStringFromType(UnitType type) { switch (type) { case UnitType.Pixel: return "px"; case UnitType.Point: return "pt"; case UnitType.Pica: return "pc"; case UnitType.Inch: return "in"; case UnitType.Mm: return "mm"; case UnitType.Cm: return "cm"; case UnitType.Percentage: return "%"; case UnitType.Em: return "em"; case UnitType.Ex: return "ex"; } return String.Empty; } ////// Converts persistence string to UnitType. /// private static UnitType GetTypeFromString(string value) { if (!String.IsNullOrEmpty(value)) { if (value.Equals("px")) { return UnitType.Pixel; } else if (value.Equals("pt")) { return UnitType.Point; } else if (value.Equals("%")) { return UnitType.Percentage; } else if (value.Equals("pc")) { return UnitType.Pica; } else if (value.Equals("in")) { return UnitType.Inch; } else if (value.Equals("mm")) { return UnitType.Mm; } else if (value.Equals("cm")) { return UnitType.Cm; } else if (value.Equals("em")) { return UnitType.Em; } else if (value.Equals("ex")) { return UnitType.Ex; } else { throw new ArgumentOutOfRangeException("value"); } } return UnitType.Pixel; } ////// public static Unit Parse(string s) { return new Unit(s, CultureInfo.CurrentCulture); } ///[To be supplied.] ////// public static Unit Parse(string s, CultureInfo culture) { return new Unit(s, culture); } ///[To be supplied.] ////// public static Unit Percentage(double n) { return new Unit(n,UnitType.Percentage); } ///Creates a ///of type from the specified 32-bit signed integer. /// public static Unit Pixel(int n) { return new Unit(n); } ///Creates a ///of type from the specified 32-bit signed integer. /// public static Unit Point(int n) { return new Unit(n,UnitType.Point); } ///Creates a ///of type from the /// specified 32-bit signed integer. /// /// public override string ToString() { return ToString((IFormatProvider)CultureInfo.CurrentCulture); } public string ToString(CultureInfo culture) { return ToString((IFormatProvider)culture); } public string ToString(IFormatProvider formatProvider) { if (IsEmpty) return String.Empty; // Double.ToString does not do the right thing, we get extra bits at the end string valuePart; if (type == UnitType.Pixel) { valuePart = ((int)value).ToString(formatProvider); } else { valuePart = ((float)value).ToString(formatProvider); } return valuePart + Unit.GetStringFromType(type); } ///Converts a ///to a . /// public static implicit operator Unit(int n) { return Unit.Pixel(n); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Implicitly creates a ///of type from the specified 32-bit unsigned integer. // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Globalization; using System.ComponentModel; using System.ComponentModel.Design; using System.Security.Permissions; using System.Web.Util; [ TypeConverterAttribute(typeof(UnitConverter)) ] [Serializable] public struct Unit { ////// Specifies an empty unit. /// public static readonly Unit Empty = new Unit(); internal const int MaxValue = 32767; internal const int MinValue = -32768; private readonly UnitType type; private readonly double value; ////// public Unit(int value) { if ((value < MinValue) || (value > MaxValue)) { throw new ArgumentOutOfRangeException("value"); } this.value = value; this.type = UnitType.Pixel; } ///Initializes a new instance of the ///structure with the specified 32-bit signed integer as /// the unit value and as the (default) unit type. /// public Unit(double value) { if ((value < MinValue) || (value > MaxValue)) { throw new ArgumentOutOfRangeException("value"); } this.value = (int)value; this.type = UnitType.Pixel; } ///Initializes a new instance of the ///structure with the /// specified double-precision /// floating point number as the unit value and /// as the (default) unit type. /// public Unit(double value, UnitType type) { if ((value < MinValue) || (value > MaxValue)) { throw new ArgumentOutOfRangeException("value"); } if (type == UnitType.Pixel) { this.value = (int)value; } else { this.value = value; } this.type = type; } ///Initializes a new instance of the ///structure with the specified /// double-precision floating point number as the unit value and the specified /// as the unit type. /// public Unit(string value) : this(value, CultureInfo.CurrentCulture, UnitType.Pixel) { } ///Initializes a new instance of the ///structure with the specified text /// string that contains the unit value and unit type. If the unit type is not /// specified, the default is /// . /// public Unit(string value, CultureInfo culture) : this(value, culture, UnitType.Pixel) { } internal Unit(string value, CultureInfo culture, UnitType defaultType) { if (String.IsNullOrEmpty(value)) { this.value = 0; this.type = (UnitType)0; } else { if (culture == null) { culture = CultureInfo.CurrentCulture; } // This is invariant because it acts like an enum with a number together. // The enum part is invariant, but the number uses current culture. string trimLcase = value.Trim().ToLower(CultureInfo.InvariantCulture); int len = trimLcase.Length; int lastDigit = -1; for (int i = 0; i < len; i++) { char ch = trimLcase[i]; if (((ch < '0') || (ch > '9')) && (ch != '-') && (ch != '.') && (ch != ',')) break; lastDigit = i; } if (lastDigit == -1) { throw new FormatException(SR.GetString(SR.UnitParseNoDigits, value)); } if (lastDigit < len - 1) { type = (UnitType)GetTypeFromString(trimLcase.Substring(lastDigit+1).Trim()); } else { type = defaultType; } string numericPart = trimLcase.Substring(0, lastDigit+1); // Cannot use Double.FromString, because we don't use it in the ToString implementation try { TypeConverter converter = new SingleConverter(); this.value = (Single)converter.ConvertFromString(null, culture, numericPart); if (type == UnitType.Pixel) { this.value = (int)this.value; } } catch { throw new FormatException(SR.GetString(SR.UnitParseNumericPart, value, numericPart, type.ToString("G"))); } if ((this.value < MinValue) || (this.value > MaxValue)) { throw new ArgumentOutOfRangeException("value"); } } } ///[To be supplied.] ////// public bool IsEmpty { get { return type == (UnitType)0; } } ///Gets a value indicating whether the ///is empty. /// public UnitType Type { get { if (!IsEmpty) { return this.type; } else { return UnitType.Pixel; } } } ///Gets or sets the type of the ///. /// public double Value { get { return this.value; } } ///Gets the value of the ///. /// public override int GetHashCode() { return HashCodeCombiner.CombineHashCodes(type.GetHashCode(), value.GetHashCode()); } ///[To be supplied.] ////// public override bool Equals(object obj) { if (obj == null || !(obj is Unit)) { return false; } Unit u = (Unit)obj; // compare internal values to avoid "defaulting" in the case of "Empty" // if (u.type == type && u.value == value) { return true; } return false; } ///Compares this ///with the specified object. /// public static bool operator ==(Unit left, Unit right) { // compare internal values to avoid "defaulting" in the case of "Empty" // return (left.type == right.type && left.value == right.value); } ///Compares two units to find out if they have the same value and type. ////// public static bool operator !=(Unit left, Unit right) { // compare internal values to avoid "defaulting" in the case of "Empty" // return (left.type != right.type || left.value != right.value); } ///Compares two units to find out if they have different /// values and/or types. ////// Converts UnitType to persistence string. /// private static string GetStringFromType(UnitType type) { switch (type) { case UnitType.Pixel: return "px"; case UnitType.Point: return "pt"; case UnitType.Pica: return "pc"; case UnitType.Inch: return "in"; case UnitType.Mm: return "mm"; case UnitType.Cm: return "cm"; case UnitType.Percentage: return "%"; case UnitType.Em: return "em"; case UnitType.Ex: return "ex"; } return String.Empty; } ////// Converts persistence string to UnitType. /// private static UnitType GetTypeFromString(string value) { if (!String.IsNullOrEmpty(value)) { if (value.Equals("px")) { return UnitType.Pixel; } else if (value.Equals("pt")) { return UnitType.Point; } else if (value.Equals("%")) { return UnitType.Percentage; } else if (value.Equals("pc")) { return UnitType.Pica; } else if (value.Equals("in")) { return UnitType.Inch; } else if (value.Equals("mm")) { return UnitType.Mm; } else if (value.Equals("cm")) { return UnitType.Cm; } else if (value.Equals("em")) { return UnitType.Em; } else if (value.Equals("ex")) { return UnitType.Ex; } else { throw new ArgumentOutOfRangeException("value"); } } return UnitType.Pixel; } ////// public static Unit Parse(string s) { return new Unit(s, CultureInfo.CurrentCulture); } ///[To be supplied.] ////// public static Unit Parse(string s, CultureInfo culture) { return new Unit(s, culture); } ///[To be supplied.] ////// public static Unit Percentage(double n) { return new Unit(n,UnitType.Percentage); } ///Creates a ///of type from the specified 32-bit signed integer. /// public static Unit Pixel(int n) { return new Unit(n); } ///Creates a ///of type from the specified 32-bit signed integer. /// public static Unit Point(int n) { return new Unit(n,UnitType.Point); } ///Creates a ///of type from the /// specified 32-bit signed integer. /// /// public override string ToString() { return ToString((IFormatProvider)CultureInfo.CurrentCulture); } public string ToString(CultureInfo culture) { return ToString((IFormatProvider)culture); } public string ToString(IFormatProvider formatProvider) { if (IsEmpty) return String.Empty; // Double.ToString does not do the right thing, we get extra bits at the end string valuePart; if (type == UnitType.Pixel) { valuePart = ((int)value).ToString(formatProvider); } else { valuePart = ((float)value).ToString(formatProvider); } return valuePart + Unit.GetStringFromType(type); } ///Converts a ///to a . /// public static implicit operator Unit(int n) { return Unit.Pixel(n); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Implicitly creates a ///of type from the specified 32-bit unsigned integer.
Link Menu
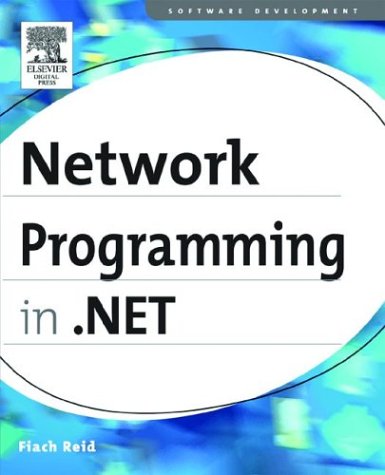
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlDataSourceStatusEventArgs.cs
- ColumnProvider.cs
- LinqDataSourceView.cs
- MexTcpBindingElement.cs
- DataBindingList.cs
- OdbcEnvironmentHandle.cs
- IPAddressCollection.cs
- LockedActivityGlyph.cs
- WorkflowLayouts.cs
- GridViewColumnHeaderAutomationPeer.cs
- Registry.cs
- ListViewDataItem.cs
- DecoderReplacementFallback.cs
- SmtpClient.cs
- DoubleLinkList.cs
- FrameDimension.cs
- LayoutManager.cs
- BindingSource.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- returneventsaver.cs
- HtmlContainerControl.cs
- SqlBulkCopyColumnMapping.cs
- EDesignUtil.cs
- Vector3DCollectionValueSerializer.cs
- SqlCacheDependencySection.cs
- DomainUpDown.cs
- FormatConvertedBitmap.cs
- XmlElementList.cs
- LoadWorkflowCommand.cs
- ListControl.cs
- DynamicValidatorEventArgs.cs
- DataRelationCollection.cs
- FrameworkElementFactoryMarkupObject.cs
- HtmlTableCell.cs
- Size3DValueSerializer.cs
- Span.cs
- FontNamesConverter.cs
- ProgressBar.cs
- Point3DAnimationUsingKeyFrames.cs
- XmlReaderSettings.cs
- UnrecognizedAssertionsBindingElement.cs
- WindowsScrollBarBits.cs
- ExpressionNormalizer.cs
- MenuItem.cs
- DataTrigger.cs
- CultureInfoConverter.cs
- CanExecuteRoutedEventArgs.cs
- ConditionBrowserDialog.cs
- ReadWriteObjectLock.cs
- EtwTrackingParticipant.cs
- ReflectionServiceProvider.cs
- VisualStyleInformation.cs
- DynamicArgumentDesigner.xaml.cs
- SecurityManager.cs
- DesignerTransactionCloseEvent.cs
- WorkflowServiceHostFactory.cs
- SwitchElementsCollection.cs
- Multiply.cs
- TemplatedWizardStep.cs
- AlternateViewCollection.cs
- SchemaImporterExtension.cs
- TemporaryBitmapFile.cs
- ExecutionEngineException.cs
- KeyConstraint.cs
- BaseParagraph.cs
- EntityWrapperFactory.cs
- NativeMethods.cs
- lengthconverter.cs
- Activator.cs
- ProvideValueServiceProvider.cs
- LicenseProviderAttribute.cs
- ScrollPattern.cs
- OleDbCommand.cs
- ModelUIElement3D.cs
- CanonicalFontFamilyReference.cs
- Hex.cs
- DataControlExtensions.cs
- Crypto.cs
- TagPrefixInfo.cs
- AlignmentXValidation.cs
- PostBackOptions.cs
- CommonProperties.cs
- FixUpCollection.cs
- TreeNodeStyleCollection.cs
- FilterException.cs
- ColumnPropertiesGroup.cs
- HostUtils.cs
- CompressEmulationStream.cs
- WpfXamlMember.cs
- XmlChoiceIdentifierAttribute.cs
- RemoteDebugger.cs
- DesignBindingPropertyDescriptor.cs
- XmlDocumentType.cs
- EncodingTable.cs
- RegexStringValidatorAttribute.cs
- VectorCollectionConverter.cs
- DoubleLink.cs
- HtmlTernaryTree.cs
- SqlDataSourceCache.cs
- ResourceContainer.cs