Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / UIAutomation / UIAutomationClient / System / Windows / Automation / GridItemPattern.cs / 1 / GridItemPattern.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for GridItem Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Allows clients to quickly determine if an item they discover is part /// of a grid and, if so, where the item is in the grid in terms of row/column coordinates /// and spans. /// #if (INTERNAL_COMPILE) internal class GridItemPattern: BasePattern #else public class GridItemPattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal GridItemPattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern) { _hPattern = hPattern; _cached = cached; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///GridItem pattern public static readonly AutomationPattern Pattern = GridItemPatternIdentifiers.Pattern; ///RowCount public static readonly AutomationProperty RowProperty = GridItemPatternIdentifiers.RowProperty; ///ColumnCount public static readonly AutomationProperty ColumnProperty = GridItemPatternIdentifiers.ColumnProperty; ///RowSpan public static readonly AutomationProperty RowSpanProperty = GridItemPatternIdentifiers.RowSpanProperty; ///ColumnSpan public static readonly AutomationProperty ColumnSpanProperty = GridItemPatternIdentifiers.ColumnSpanProperty; ///The logical element that supports the GripPattern for this Item public static readonly AutomationProperty ContainingGridProperty = GridItemPatternIdentifiers.ContainingGridProperty; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// public GridItemPatternInformation Cached { get { Misc.ValidateCached(_cached); return new GridItemPatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// public GridItemPatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new GridItemPatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal static object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new GridItemPattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; internal bool _cached; // internal, since protected makes this publically visible #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct GridItemPatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal GridItemPatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// the row number of the element. This is zero based. /// /// ////// This API does not work inside the secure execution environment. /// public int Row { get { return (int)_el.GetPatternPropertyValue(RowProperty, _useCache); } } ////// /// the column number of the element. This is zero based. /// /// ////// This API does not work inside the secure execution environment. /// public int Column { get { return (int)_el.GetPatternPropertyValue(ColumnProperty, _useCache); } } ////// /// count of how many rows the element spans /// -- non merged cells should always return 1 /// /// ////// This API does not work inside the secure execution environment. /// public int RowSpan { get { return (int)_el.GetPatternPropertyValue(RowSpanProperty, _useCache); } } ////// /// count of how many columns the element spans /// -- non merged cells should always return 1 /// /// ////// This API does not work inside the secure execution environment. /// public int ColumnSpan { get { return (int)_el.GetPatternPropertyValue(ColumnSpanProperty, _useCache); } } ////// /// The logical element that supports the GripPattern for this Item /// /// ////// This API does not work inside the secure execution environment. /// public AutomationElement ContainingGrid { get { return (AutomationElement)_el.GetPatternPropertyValue(ContainingGridProperty, _useCache); } } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // ///// // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for GridItem Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Allows clients to quickly determine if an item they discover is part /// of a grid and, if so, where the item is in the grid in terms of row/column coordinates /// and spans. /// #if (INTERNAL_COMPILE) internal class GridItemPattern: BasePattern #else public class GridItemPattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal GridItemPattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern) { _hPattern = hPattern; _cached = cached; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///GridItem pattern public static readonly AutomationPattern Pattern = GridItemPatternIdentifiers.Pattern; ///RowCount public static readonly AutomationProperty RowProperty = GridItemPatternIdentifiers.RowProperty; ///ColumnCount public static readonly AutomationProperty ColumnProperty = GridItemPatternIdentifiers.ColumnProperty; ///RowSpan public static readonly AutomationProperty RowSpanProperty = GridItemPatternIdentifiers.RowSpanProperty; ///ColumnSpan public static readonly AutomationProperty ColumnSpanProperty = GridItemPatternIdentifiers.ColumnSpanProperty; ///The logical element that supports the GripPattern for this Item public static readonly AutomationProperty ContainingGridProperty = GridItemPatternIdentifiers.ContainingGridProperty; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// public GridItemPatternInformation Cached { get { Misc.ValidateCached(_cached); return new GridItemPatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// public GridItemPatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new GridItemPatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal static object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new GridItemPattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; internal bool _cached; // internal, since protected makes this publically visible #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct GridItemPatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal GridItemPatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// the row number of the element. This is zero based. /// /// ////// This API does not work inside the secure execution environment. /// public int Row { get { return (int)_el.GetPatternPropertyValue(RowProperty, _useCache); } } ////// /// the column number of the element. This is zero based. /// /// ////// This API does not work inside the secure execution environment. /// public int Column { get { return (int)_el.GetPatternPropertyValue(ColumnProperty, _useCache); } } ////// /// count of how many rows the element spans /// -- non merged cells should always return 1 /// /// ////// This API does not work inside the secure execution environment. /// public int RowSpan { get { return (int)_el.GetPatternPropertyValue(RowSpanProperty, _useCache); } } ////// /// count of how many columns the element spans /// -- non merged cells should always return 1 /// /// ////// This API does not work inside the secure execution environment. /// public int ColumnSpan { get { return (int)_el.GetPatternPropertyValue(ColumnSpanProperty, _useCache); } } ////// /// The logical element that supports the GripPattern for this Item /// /// ////// This API does not work inside the secure execution environment. /// public AutomationElement ContainingGrid { get { return (AutomationElement)_el.GetPatternPropertyValue(ContainingGridProperty, _useCache); } } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
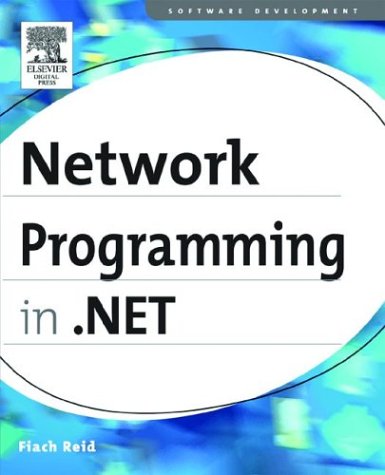
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CollectionChangedEventManager.cs
- HwndSubclass.cs
- InfiniteTimeSpanConverter.cs
- ReferentialConstraint.cs
- LocatorPartList.cs
- HttpListener.cs
- LoadedOrUnloadedOperation.cs
- Filter.cs
- MemberHolder.cs
- SingleObjectCollection.cs
- DataGridViewTextBoxEditingControl.cs
- XmlSchemaComplexType.cs
- XPathArrayIterator.cs
- PkcsMisc.cs
- SystemInformation.cs
- FontCacheUtil.cs
- ViewPort3D.cs
- DataSourceViewSchemaConverter.cs
- Repeater.cs
- DelayDesigner.cs
- CodeEntryPointMethod.cs
- DbParameterCollection.cs
- ArrangedElementCollection.cs
- TcpWorkerProcess.cs
- CompatibleIComparer.cs
- _FixedSizeReader.cs
- RegistrySecurity.cs
- TextAdaptor.cs
- DomainConstraint.cs
- HostingEnvironmentSection.cs
- Help.cs
- FullTrustAssembly.cs
- SiteMapPath.cs
- TileBrush.cs
- PseudoWebRequest.cs
- ComponentEditorPage.cs
- WindowsRichEditRange.cs
- Addressing.cs
- ResourceKey.cs
- GridView.cs
- SweepDirectionValidation.cs
- ShutDownListener.cs
- DragEventArgs.cs
- XsdCachingReader.cs
- GZipObjectSerializer.cs
- RuntimeConfigLKG.cs
- MultitargetUtil.cs
- Typeface.cs
- dsa.cs
- SrgsGrammarCompiler.cs
- DesignerExtenders.cs
- Typeface.cs
- followingquery.cs
- FormsAuthenticationCredentials.cs
- SystemException.cs
- ItemsChangedEventArgs.cs
- SafeNativeMethods.cs
- FilterEventArgs.cs
- altserialization.cs
- AdornerHitTestResult.cs
- HitTestDrawingContextWalker.cs
- WinFormsSecurity.cs
- XmlMapping.cs
- DocumentApplication.cs
- FillBehavior.cs
- XmlSchemaGroup.cs
- PtsContext.cs
- ColorAnimationUsingKeyFrames.cs
- ProcessModuleCollection.cs
- DynamicActivityTypeDescriptor.cs
- RegexParser.cs
- RenderOptions.cs
- XmlSchemaAny.cs
- WindowsTooltip.cs
- AppModelKnownContentFactory.cs
- ValueCollectionParameterReader.cs
- __Error.cs
- SqlServer2KCompatibilityAnnotation.cs
- GridViewDeleteEventArgs.cs
- DragDropManager.cs
- SafeJobHandle.cs
- ReflectionServiceProvider.cs
- ToolStripProgressBar.cs
- CheckBoxPopupAdapter.cs
- TextBoxAutoCompleteSourceConverter.cs
- HierarchicalDataTemplate.cs
- FontDriver.cs
- StructuredTypeEmitter.cs
- WizardPanelChangingEventArgs.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- QueryAsyncResult.cs
- HostedNamedPipeTransportManager.cs
- TextServicesCompartmentContext.cs
- ResourceDictionaryCollection.cs
- KeyInstance.cs
- TypeDescriptionProviderAttribute.cs
- WeakHashtable.cs
- ScrollProperties.cs
- SqlException.cs
- ActivityCodeDomSerializer.cs