Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Data / System / Data / Common / SafeNativeMethods.cs / 1305376 / SafeNativeMethods.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Text; using System.Threading; using System.Runtime.ConstrainedExecution; using System.Runtime.Versioning; namespace System.Data.Common { [SuppressUnmanagedCodeSecurityAttribute()] internal static class SafeNativeMethods { [DllImport(ExternDll.Ole32, SetLastError=false)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [ResourceExposure(ResourceScope.None)] static internal extern IntPtr CoTaskMemAlloc(IntPtr cb); [DllImport(ExternDll.Ole32, SetLastError=false)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [ResourceExposure(ResourceScope.None)] static internal extern void CoTaskMemFree(IntPtr handle); [DllImport(ExternDll.Kernel32, CharSet=CharSet.Unicode, PreserveSig=true)] [ResourceExposure(ResourceScope.None)] static internal extern int GetUserDefaultLCID(); #if !ORACLE [DllImport(ExternDll.Kernel32, PreserveSig=true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [ResourceExposure(ResourceScope.None)] static internal extern void ZeroMemory(IntPtr dest, IntPtr length); #endif /*[DllImport(ExternDll.Kernel32)] static internal extern IntPtr InterlockedCompareExchange( IntPtr Destination, // destination address IntPtr Exchange, // exchange value IntPtr Comperand // value to compare );*/ //// Using the int versions of the Increment() and Decrement() methods is correct. // Please check \fx\src\Data\System\Data\Odbc\OdbcHandle.cs for the memory layout. // //// The following casting operations require these three methods to be unsafe. This is // a workaround for this issue to meet the M1 exit criteria. We need to revisit this in M2. [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static internal unsafe IntPtr InterlockedExchangePointer( IntPtr lpAddress, IntPtr lpValue) { IntPtr previousPtr; IntPtr actualPtr = *(IntPtr *)lpAddress.ToPointer(); do { previousPtr = actualPtr; actualPtr = Interlocked.CompareExchange(ref *(IntPtr *)lpAddress.ToPointer(), lpValue, previousPtr); } while (actualPtr != previousPtr); return actualPtr; } // http://msdn.microsoft.com/library/default.asp?url=/library/en-us/sysinfo/base/getcomputernameex.asp [DllImport(ExternDll.Kernel32, CharSet = CharSet.Unicode, EntryPoint="GetComputerNameExW", SetLastError=true)] [ResourceExposure(ResourceScope.None)] static internal extern int GetComputerNameEx(int nameType, StringBuilder nameBuffer, ref int bufferSize); [DllImport(ExternDll.Kernel32, CharSet=System.Runtime.InteropServices.CharSet.Auto)] [ResourceExposure(ResourceScope.Process)] static internal extern int GetCurrentProcessId(); [DllImport(ExternDll.Kernel32, CharSet=CharSet.Auto, BestFitMapping=false, ThrowOnUnmappableChar=true)] // [DllImport(ExternDll.Kernel32, CharSet=CharSet.Auto)] [ResourceExposure(ResourceScope.Process)] static internal extern IntPtr GetModuleHandle([MarshalAs(UnmanagedType.LPTStr), In] string moduleName/*lpctstr*/); [DllImport(ExternDll.Kernel32, CharSet=CharSet.Ansi, BestFitMapping=false, ThrowOnUnmappableChar=true)] // [DllImport(ExternDll.Kernel32, CharSet=CharSet.Ansi)] [ResourceExposure(ResourceScope.None)] static internal extern IntPtr GetProcAddress(IntPtr HModule, [MarshalAs(UnmanagedType.LPStr), In] string funcName/*lpcstr*/); [DllImport(ExternDll.Kernel32)] [ResourceExposure(ResourceScope.None)] internal static extern void GetSystemTimeAsFileTime(out long lpSystemTimeAsFileTime); [DllImport(ExternDll.Kernel32, SetLastError=true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [ResourceExposure(ResourceScope.None)] static internal extern IntPtr LocalAlloc(int flags, IntPtr countOfBytes); [DllImport(ExternDll.Kernel32, SetLastError=true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [ResourceExposure(ResourceScope.None)] static internal extern IntPtr LocalFree(IntPtr handle); [DllImport(ExternDll.Oleaut32, CharSet=CharSet.Unicode)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [ResourceExposure(ResourceScope.None)] internal static extern IntPtr SysAllocStringLen(String src, int len); // BSTR [DllImport(ExternDll.Oleaut32)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [ResourceExposure(ResourceScope.None)] internal static extern void SysFreeString(IntPtr bstr); // only using this to clear existing error info with null [DllImport(ExternDll.Oleaut32, CharSet=CharSet.Unicode, PreserveSig=false)] // TLS values are preserved between threads, need to check that we use this API to clear the error state only. [ResourceExposure(ResourceScope.Process)] static private extern void SetErrorInfo(Int32 dwReserved, IntPtr pIErrorInfo); [DllImport(ExternDll.Kernel32, SetLastError=true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [ResourceExposure(ResourceScope.Machine)] static internal extern int ReleaseSemaphore(IntPtr handle, int releaseCount, IntPtr previousCount); [DllImport(ExternDll.Kernel32, SetLastError=true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [ResourceExposure(ResourceScope.None)] static internal extern int WaitForMultipleObjectsEx(uint nCount, IntPtr lpHandles, bool bWaitAll, uint dwMilliseconds, bool bAlertable); [DllImport(ExternDll.Kernel32/*, SetLastError=true*/)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [ResourceExposure(ResourceScope.None)] static internal extern int WaitForSingleObjectEx(IntPtr lpHandles, uint dwMilliseconds, bool bAlertable); [DllImport(ExternDll.Ole32, PreserveSig=false)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [ResourceExposure(ResourceScope.None)] static internal extern void PropVariantClear(IntPtr pObject); [DllImport(ExternDll.Oleaut32, PreserveSig=false)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [ResourceExposure(ResourceScope.None)] static internal extern void VariantClear(IntPtr pObject); sealed internal class Wrapper { private Wrapper() { } // SxS: clearing error information is considered safe [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Process, ResourceScope.Process)] static internal void ClearErrorInfo() { // MDAC 68199 SafeNativeMethods.SetErrorInfo(0, ADP.PtrZero); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Text; using System.Threading; using System.Runtime.ConstrainedExecution; using System.Runtime.Versioning; namespace System.Data.Common { [SuppressUnmanagedCodeSecurityAttribute()] internal static class SafeNativeMethods { [DllImport(ExternDll.Ole32, SetLastError=false)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [ResourceExposure(ResourceScope.None)] static internal extern IntPtr CoTaskMemAlloc(IntPtr cb); [DllImport(ExternDll.Ole32, SetLastError=false)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [ResourceExposure(ResourceScope.None)] static internal extern void CoTaskMemFree(IntPtr handle); [DllImport(ExternDll.Kernel32, CharSet=CharSet.Unicode, PreserveSig=true)] [ResourceExposure(ResourceScope.None)] static internal extern int GetUserDefaultLCID(); #if !ORACLE [DllImport(ExternDll.Kernel32, PreserveSig=true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [ResourceExposure(ResourceScope.None)] static internal extern void ZeroMemory(IntPtr dest, IntPtr length); #endif /*[DllImport(ExternDll.Kernel32)] static internal extern IntPtr InterlockedCompareExchange( IntPtr Destination, // destination address IntPtr Exchange, // exchange value IntPtr Comperand // value to compare );*/ //// Using the int versions of the Increment() and Decrement() methods is correct. // Please check \fx\src\Data\System\Data\Odbc\OdbcHandle.cs for the memory layout. // //// The following casting operations require these three methods to be unsafe. This is // a workaround for this issue to meet the M1 exit criteria. We need to revisit this in M2. [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static internal unsafe IntPtr InterlockedExchangePointer( IntPtr lpAddress, IntPtr lpValue) { IntPtr previousPtr; IntPtr actualPtr = *(IntPtr *)lpAddress.ToPointer(); do { previousPtr = actualPtr; actualPtr = Interlocked.CompareExchange(ref *(IntPtr *)lpAddress.ToPointer(), lpValue, previousPtr); } while (actualPtr != previousPtr); return actualPtr; } // http://msdn.microsoft.com/library/default.asp?url=/library/en-us/sysinfo/base/getcomputernameex.asp [DllImport(ExternDll.Kernel32, CharSet = CharSet.Unicode, EntryPoint="GetComputerNameExW", SetLastError=true)] [ResourceExposure(ResourceScope.None)] static internal extern int GetComputerNameEx(int nameType, StringBuilder nameBuffer, ref int bufferSize); [DllImport(ExternDll.Kernel32, CharSet=System.Runtime.InteropServices.CharSet.Auto)] [ResourceExposure(ResourceScope.Process)] static internal extern int GetCurrentProcessId(); [DllImport(ExternDll.Kernel32, CharSet=CharSet.Auto, BestFitMapping=false, ThrowOnUnmappableChar=true)] // [DllImport(ExternDll.Kernel32, CharSet=CharSet.Auto)] [ResourceExposure(ResourceScope.Process)] static internal extern IntPtr GetModuleHandle([MarshalAs(UnmanagedType.LPTStr), In] string moduleName/*lpctstr*/); [DllImport(ExternDll.Kernel32, CharSet=CharSet.Ansi, BestFitMapping=false, ThrowOnUnmappableChar=true)] // [DllImport(ExternDll.Kernel32, CharSet=CharSet.Ansi)] [ResourceExposure(ResourceScope.None)] static internal extern IntPtr GetProcAddress(IntPtr HModule, [MarshalAs(UnmanagedType.LPStr), In] string funcName/*lpcstr*/); [DllImport(ExternDll.Kernel32)] [ResourceExposure(ResourceScope.None)] internal static extern void GetSystemTimeAsFileTime(out long lpSystemTimeAsFileTime); [DllImport(ExternDll.Kernel32, SetLastError=true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [ResourceExposure(ResourceScope.None)] static internal extern IntPtr LocalAlloc(int flags, IntPtr countOfBytes); [DllImport(ExternDll.Kernel32, SetLastError=true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [ResourceExposure(ResourceScope.None)] static internal extern IntPtr LocalFree(IntPtr handle); [DllImport(ExternDll.Oleaut32, CharSet=CharSet.Unicode)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [ResourceExposure(ResourceScope.None)] internal static extern IntPtr SysAllocStringLen(String src, int len); // BSTR [DllImport(ExternDll.Oleaut32)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [ResourceExposure(ResourceScope.None)] internal static extern void SysFreeString(IntPtr bstr); // only using this to clear existing error info with null [DllImport(ExternDll.Oleaut32, CharSet=CharSet.Unicode, PreserveSig=false)] // TLS values are preserved between threads, need to check that we use this API to clear the error state only. [ResourceExposure(ResourceScope.Process)] static private extern void SetErrorInfo(Int32 dwReserved, IntPtr pIErrorInfo); [DllImport(ExternDll.Kernel32, SetLastError=true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [ResourceExposure(ResourceScope.Machine)] static internal extern int ReleaseSemaphore(IntPtr handle, int releaseCount, IntPtr previousCount); [DllImport(ExternDll.Kernel32, SetLastError=true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [ResourceExposure(ResourceScope.None)] static internal extern int WaitForMultipleObjectsEx(uint nCount, IntPtr lpHandles, bool bWaitAll, uint dwMilliseconds, bool bAlertable); [DllImport(ExternDll.Kernel32/*, SetLastError=true*/)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] [ResourceExposure(ResourceScope.None)] static internal extern int WaitForSingleObjectEx(IntPtr lpHandles, uint dwMilliseconds, bool bAlertable); [DllImport(ExternDll.Ole32, PreserveSig=false)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [ResourceExposure(ResourceScope.None)] static internal extern void PropVariantClear(IntPtr pObject); [DllImport(ExternDll.Oleaut32, PreserveSig=false)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [ResourceExposure(ResourceScope.None)] static internal extern void VariantClear(IntPtr pObject); sealed internal class Wrapper { private Wrapper() { } // SxS: clearing error information is considered safe [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Process, ResourceScope.Process)] static internal void ClearErrorInfo() { // MDAC 68199 SafeNativeMethods.SetErrorInfo(0, ADP.PtrZero); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
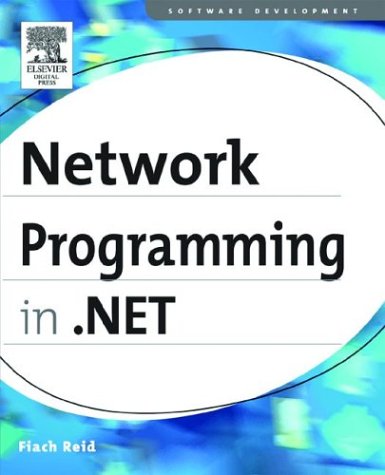
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XsltOutput.cs
- XmlTextEncoder.cs
- Quad.cs
- XmlSchemaAny.cs
- ReflectionUtil.cs
- SafeCryptContextHandle.cs
- DetailsViewDeletedEventArgs.cs
- PackageRelationshipSelector.cs
- Int32Animation.cs
- TextDecoration.cs
- TreeNodeConverter.cs
- ConstructorExpr.cs
- UInt16Converter.cs
- invalidudtexception.cs
- FtpWebResponse.cs
- LicenseManager.cs
- CompilerResults.cs
- FormCollection.cs
- ProcessModuleCollection.cs
- ClientTarget.cs
- HttpsTransportBindingElement.cs
- CreateUserWizardDesigner.cs
- Line.cs
- XmlImplementation.cs
- ExpressionVisitorHelpers.cs
- TemplateBindingExpressionConverter.cs
- ByteFacetDescriptionElement.cs
- ExpressionBuilder.cs
- StandardToolWindows.cs
- ObservableCollection.cs
- WaitingCursor.cs
- ProxyHelper.cs
- Publisher.cs
- IntSecurity.cs
- LifetimeServices.cs
- TimeSpanSecondsConverter.cs
- BulletedListEventArgs.cs
- WindowInteropHelper.cs
- AddIn.cs
- ConfigXmlReader.cs
- UnaryExpression.cs
- Utility.cs
- GraphicsPath.cs
- MethodCallTranslator.cs
- WebBrowserPermission.cs
- Header.cs
- RtfNavigator.cs
- DictionaryItemsCollection.cs
- EncoderFallback.cs
- InstanceNotReadyException.cs
- PassportAuthenticationModule.cs
- XPathDescendantIterator.cs
- ComplexTypeEmitter.cs
- TracingConnectionInitiator.cs
- Freezable.cs
- QuaternionAnimation.cs
- HttpResponseWrapper.cs
- CornerRadius.cs
- ObjectAnimationUsingKeyFrames.cs
- DbTransaction.cs
- SortAction.cs
- RequestCachePolicy.cs
- PinnedBufferMemoryStream.cs
- WebPartChrome.cs
- AssemblyResolver.cs
- Point3D.cs
- SeekableMessageNavigator.cs
- CodeObjectCreateExpression.cs
- UnknownWrapper.cs
- FileClassifier.cs
- ConnectionStringsSection.cs
- XMLUtil.cs
- TextTreeObjectNode.cs
- ScriptIgnoreAttribute.cs
- SignedXmlDebugLog.cs
- BitmapEditor.cs
- BindingCollectionElement.cs
- httpserverutility.cs
- WebPartsSection.cs
- UInt64.cs
- SocketElement.cs
- InsufficientExecutionStackException.cs
- Converter.cs
- ReadContentAsBinaryHelper.cs
- SingleKeyFrameCollection.cs
- UmAlQuraCalendar.cs
- AnonymousIdentificationSection.cs
- Column.cs
- TextEncodedRawTextWriter.cs
- PopupRoot.cs
- WebZone.cs
- HttpListenerException.cs
- ChannelToken.cs
- AsyncDataRequest.cs
- SchemaElement.cs
- Soap12ProtocolImporter.cs
- isolationinterop.cs
- CodeArgumentReferenceExpression.cs
- ContextStack.cs
- PartialList.cs