Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / MS / Internal / Ink / Quad.cs / 1 / Quad.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using MS.Utility; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink { ////// A helper structure used in StrokeNode and StrokeNodeOperation implementations /// to store endpoints of the quad connecting two nodes of a stroke. /// The vertices of a quad are supposed to be clockwise with points A and D located /// on the begin node and B and C on the end one. /// internal struct Quad { #region Statics private static Quad s_empty = new Quad(new Point(0, 0), new Point(0, 0), new Point(0, 0), new Point(0, 0)); #endregion #region API ///Returns the static object representing an empty (unitialized) quad internal static Quad Empty { get { return s_empty; } } ///Constructor internal Quad(Point a, Point b, Point c, Point d) { _A = a; _B = b; _C = c; _D = d; } ///The A vertex of the quad internal Point A { get { return _A; } set { _A = value; } } ///The B vertex of the quad internal Point B { get { return _B; } set { _B = value; } } ///The C vertex of the quad internal Point C { get { return _C; } set { _C = value; } } ///The D vertex of the quad internal Point D { get { return _D; } set { _D = value; } } // Returns quad's vertex by index where A is of the index 0, B - is 1, etc internal Point this[int index] { get { switch (index) { case 0: return _A; case 1: return _B; case 2: return _C; case 3: return _D; default: throw new IndexOutOfRangeException("index"); } } } ///Tells whether the quad is invalid (empty) internal bool IsEmpty { get { return (_A == _B) && (_C == _D); } } internal void GetPoints(ListpointBuffer) { pointBuffer.Add(_A); pointBuffer.Add(_B); pointBuffer.Add(_C); pointBuffer.Add(_D); } /// Returns the bounds of the quad internal Rect Bounds { get { return IsEmpty ? Rect.Empty : Rect.Union(new Rect(_A, _B), new Rect(_C, _D)); } } #endregion #region Fields private Point _A; private Point _B; private Point _C; private Point _D; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
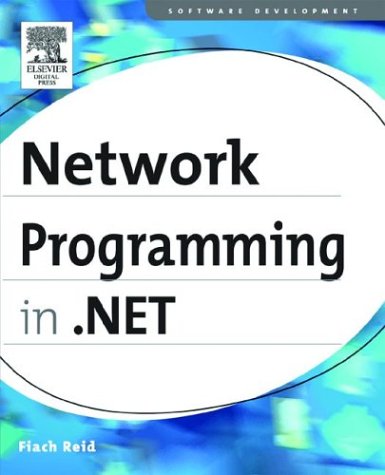
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _Win32.cs
- EntityContainer.cs
- CodeDefaultValueExpression.cs
- HitTestWithGeometryDrawingContextWalker.cs
- ListViewItem.cs
- DocumentGridContextMenu.cs
- RuntimeHelpers.cs
- DefaultPropertyAttribute.cs
- EventLogEntryCollection.cs
- RightsManagementResourceHelper.cs
- smtpconnection.cs
- PropertyIDSet.cs
- Stack.cs
- AnnouncementInnerClientCD1.cs
- Debug.cs
- RuleSetDialog.cs
- HtmlInputReset.cs
- PeerMessageDispatcher.cs
- MaskInputRejectedEventArgs.cs
- WindowShowOrOpenTracker.cs
- AnnotationHighlightLayer.cs
- EventWaitHandleSecurity.cs
- ObjectDataProvider.cs
- CanonicalizationDriver.cs
- Ray3DHitTestResult.cs
- HotSpotCollection.cs
- PromptEventArgs.cs
- HtmlControlDesigner.cs
- ListViewUpdateEventArgs.cs
- TcpTransportSecurityElement.cs
- State.cs
- ListViewGroup.cs
- TextTabProperties.cs
- DbDataSourceEnumerator.cs
- BatchServiceHost.cs
- TableParaClient.cs
- TextSpan.cs
- CellParagraph.cs
- DocumentViewerConstants.cs
- DataViewSetting.cs
- ItemCheckEvent.cs
- FormsAuthenticationUserCollection.cs
- DocumentSequence.cs
- IsolatedStorageException.cs
- TypeKeyValue.cs
- OdbcHandle.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- PtsPage.cs
- PageCatalogPart.cs
- BidOverLoads.cs
- Assembly.cs
- Line.cs
- CfgRule.cs
- BaseParagraph.cs
- AsyncCompletedEventArgs.cs
- FontStyleConverter.cs
- AppSettingsExpressionBuilder.cs
- GPRECTF.cs
- ToolStripPanelCell.cs
- ArraySortHelper.cs
- ResXDataNode.cs
- XmlUnspecifiedAttribute.cs
- ReadOnlyCollection.cs
- FillErrorEventArgs.cs
- TreeNode.cs
- BindableTemplateBuilder.cs
- PageParser.cs
- WebPartConnectionsConnectVerb.cs
- UrlMappingsSection.cs
- XmlSchemaType.cs
- KoreanLunisolarCalendar.cs
- SettingsBindableAttribute.cs
- ModuleConfigurationInfo.cs
- FloaterBaseParaClient.cs
- EventEntry.cs
- UpDownEvent.cs
- ScheduleChanges.cs
- Win32Exception.cs
- Oid.cs
- SourceFilter.cs
- DefaultTraceListener.cs
- RepeaterItemEventArgs.cs
- ResourcePermissionBase.cs
- EventLogTraceListener.cs
- MimeMultiPart.cs
- HostProtectionException.cs
- WebServiceFault.cs
- AnnotationAuthorChangedEventArgs.cs
- ObjectContext.cs
- UnmanagedBitmapWrapper.cs
- EllipseGeometry.cs
- UrlPath.cs
- AuthStoreRoleProvider.cs
- TreeNode.cs
- DataListCommandEventArgs.cs
- MapPathBasedVirtualPathProvider.cs
- ListManagerBindingsCollection.cs
- MenuBindingsEditorForm.cs
- DataGridRowAutomationPeer.cs
- ActivityDesignerResources.cs