Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / SystemInformation.cs / 1 / SystemInformation.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Text; using System.Configuration.Assemblies; using System.Threading; using System.Runtime.Remoting; using System.Runtime.InteropServices; using System.Diagnostics; using System; using Microsoft.Win32; using System.IO; using System.Security; using System.Security.Permissions; using System.Drawing; using System.ComponentModel; ////// /// public class SystemInformation { // private constructor to prevent creation // private SystemInformation() { } // Figure out if all the multimon stuff is supported on the OS // private static bool checkMultiMonitorSupport = false; private static bool multiMonitorSupport = false; private static bool checkNativeMouseWheelSupport = false; private static bool nativeMouseWheelSupport = true; private static bool highContrast = false; private static bool systemEventsAttached = false; private static bool systemEventsDirty = true; private static IntPtr processWinStation = IntPtr.Zero; private static bool isUserInteractive = false; private static PowerStatus powerStatus = null; private const int DefaultMouseWheelScrollLines = 3; //////////////////////////////////////////////////////////////////////////// // SystemParametersInfo // ///Provides information about the operating system. ////// /// public static bool DragFullWindows { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETDRAGFULLWINDOWS, 0, ref data, 0); return data != 0; } } ////// Gets a value indicating whether the user has enabled full window drag. /// ////// /// public static bool HighContrast { get { EnsureSystemEvents(); if (systemEventsDirty) { NativeMethods.HIGHCONTRAST_I data = new NativeMethods.HIGHCONTRAST_I(); data.cbSize = Marshal.SizeOf(data); data.dwFlags = 0; data.lpszDefaultScheme = IntPtr.Zero; bool b = UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETHIGHCONTRAST, data.cbSize, ref data, 0); // NT4 does not support this parameter, so we always force // it to false if we fail to get the parameter. // if (b) { highContrast = (data.dwFlags & NativeMethods.HCF_HIGHCONTRASTON) != 0; } else { highContrast = false; } systemEventsDirty = false; } return highContrast; } } ////// Gets a value indicating whether the user has selected to run in high contrast /// mode. /// ////// /// public static int MouseWheelScrollLines { get { if (NativeMouseWheelSupport) { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETWHEELSCROLLLINES, 0, ref data, 0); return data; } else { IntPtr hWndMouseWheel = IntPtr.Zero; // Check for the MouseZ "service". This is a little app that generated the // MSH_MOUSEWHEEL messages by monitoring the hardware. If this app isn't // found, then there is no support for MouseWheels on the system. // hWndMouseWheel = UnsafeNativeMethods.FindWindow(NativeMethods.MOUSEZ_CLASSNAME, NativeMethods.MOUSEZ_TITLE); if (hWndMouseWheel != IntPtr.Zero) { // Register the MSH_SCROLL_LINES message... // int message = SafeNativeMethods.RegisterWindowMessage(NativeMethods.MSH_SCROLL_LINES); int lines = (int)UnsafeNativeMethods.SendMessage(new HandleRef(null, hWndMouseWheel), message, 0, 0); // this fails under terminal server, so we default to 3, which is the windows // default. Nobody seems to pay attention to this value anyways... if (lines != 0) { return lines; } } } return DefaultMouseWheelScrollLines; } } //////////////////////////////////////////////////////////////////////////// // SystemMetrics // ////// Gets the number of lines to scroll when the mouse wheel is rotated. /// ////// /// public static Size PrimaryMonitorSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXSCREEN), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYSCREEN)); } } ////// Gets the dimensions of the primary display monitor in pixels. /// ////// /// public static int VerticalScrollBarWidth { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXVSCROLL); } } ////// Gets the width of the vertical scroll bar in pixels. /// ////// /// public static int HorizontalScrollBarHeight { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYHSCROLL); } } ////// Gets the height of the horizontal scroll bar in pixels. /// ////// /// public static int CaptionHeight { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYCAPTION); } } ////// Gets the height of the normal caption area of a window in pixels. /// ////// /// public static Size BorderSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXBORDER), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYBORDER)); } } ////// Gets the width and /// height of a window border in pixels. /// ////// /// public static Size FixedFrameBorderSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXFIXEDFRAME), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYFIXEDFRAME)); } } ////// Gets the thickness in pixels, of the border for a window that has a caption /// and is not resizable. /// ////// /// public static int VerticalScrollBarThumbHeight { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYVTHUMB); } } ///Gets the height of the scroll box in a vertical scroll bar in pixels. ////// /// public static int HorizontalScrollBarThumbWidth { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXHTHUMB); } } /* ///Gets the width of the scroll box in a horizontal scroll bar in pixels. ///public static Font IconFont { get { Font iconFont = IconFontInternal; return iconFont != null ? iconFont : Control.DefaultFont; } } // IconFontInternal is the same as IconFont, only it does not fall back to Control.DefaultFont // if the icon font can not be retrieved. It returns null instead. internal static Font IconFontInternal { get { Font iconFont = null; NativeMethods.ICONMETRICS data = new NativeMethods.ICONMETRICS(); bool result = UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETICONMETRICS, data.cbSize, data, 0); Debug.Assert(!result || data.iHorzSpacing == IconHorizontalSpacing, "Spacing in ICONMETRICS does not match IconHorizontalSpacing."); Debug.Assert(!result || data.iVertSpacing == IconVerticalSpacing, "Spacing in ICONMETRICS does not match IconVerticalSpacing."); if (result && data.lfFont != null) { try { iconFont = Font.FromLogFont(data.lfFont); } catch {} } return iconFont; } } */ /// /// /// public static Size IconSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXICON), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYICON)); } } ////// Gets the default dimensions of an icon in pixels. /// ////// /// public static Size CursorSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXCURSOR), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYCURSOR)); } } ////// Gets the dimensions of a cursor in pixels. /// ////// /// public static Font MenuFont { get { Font menuFont = null; //we can get the system's menu font through the NONCLIENTMETRICS structure via SystemParametersInfo // NativeMethods.NONCLIENTMETRICS data = new NativeMethods.NONCLIENTMETRICS(); bool result = UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETNONCLIENTMETRICS, data.cbSize, data, 0); if (result && data.lfMenuFont != null) { // SECREVIEW : This assert is safe since we created the NONCLIENTMETRICS struct. // IntSecurity.ObjectFromWin32Handle.Assert(); try { menuFont = Font.FromLogFont(data.lfMenuFont); } catch { // menu font is not true type. Default to standard control font. // menuFont = Control.DefaultFont; } finally { CodeAccessPermission.RevertAssert(); } } return menuFont; } } ////// Gets the system's font for menus. /// ////// /// public static int MenuHeight { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYMENU); } } ////// Gets the height of a one line of a menu in pixels. /// ////// /// public static PowerStatus PowerStatus { get { if (powerStatus == null) { powerStatus = new PowerStatus(); } return powerStatus; } } ////// Returns the current system power status. /// ////// /// public static Rectangle WorkingArea { get { NativeMethods.RECT rc = new NativeMethods.RECT(); UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETWORKAREA, 0, ref rc, 0); return Rectangle.FromLTRB(rc.left, rc.top, rc.right, rc.bottom); } } ////// Gets the size of the working area in pixels. /// ////// /// public static int KanjiWindowHeight { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYKANJIWINDOW); } } ////// Gets /// the height, in pixels, of the Kanji window at the bottom /// of the screen for double-byte (DBCS) character set versions of Windows. /// ////// /// [EditorBrowsable(EditorBrowsableState.Never)] public static bool MousePresent { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_MOUSEPRESENT) != 0; } } ////// Gets a value indicating whether the system has a mouse installed. /// ////// /// public static int VerticalScrollBarArrowHeight { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYVSCROLL); } } ////// Gets the height in pixels, of the arrow bitmap on the vertical scroll bar. /// ////// /// public static int HorizontalScrollBarArrowWidth { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXHSCROLL); } } ////// Gets the width, in pixels, of the arrow bitmap on the horizontal scrollbar. /// ////// /// public static bool DebugOS { get { Debug.WriteLineIf(IntSecurity.SecurityDemand.TraceVerbose, "SensitiveSystemInformation Demanded"); IntSecurity.SensitiveSystemInformation.Demand(); return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_DEBUG) != 0; } } ////// Gets a value indicating whether this is a debug version of the operating /// system. /// ////// /// public static bool MouseButtonsSwapped { get { return(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_SWAPBUTTON) != 0); } } ////// Gets a value indicating whether the functions of the left and right mouse /// buttons have been swapped. /// ////// /// public static Size MinimumWindowSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXMIN), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYMIN)); } } ////// Gets the minimum allowable dimensions of a window in pixels. /// ////// /// public static Size CaptionButtonSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXSIZE), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYSIZE)); } } ////// Gets the dimensions in pixels, of a caption bar or title bar /// button. /// ////// /// public static Size FrameBorderSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXFRAME), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYFRAME)); } } ////// Gets the thickness in pixels, of the border for a window that can be resized. /// ////// /// public static Size MinWindowTrackSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXMINTRACK), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYMINTRACK)); } } ////// Gets the system's default /// minimum tracking dimensions of a window in pixels. /// ////// /// public static Size DoubleClickSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXDOUBLECLK), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYDOUBLECLK)); } } ////// Gets the dimensions in pixels, of the area that the user must click within /// for the system to consider the two clicks a double-click. The rectangle is /// centered around the first click. /// ////// /// public static int DoubleClickTime { get { return SafeNativeMethods.GetDoubleClickTime(); } } ////// Gets the maximum number of milliseconds allowed between mouse clicks for a /// double-click. /// ////// /// public static Size IconSpacingSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXICONSPACING), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYICONSPACING)); } } ////// Gets /// the dimensions in pixels, of the grid used /// to arrange icons in a large icon view. /// ////// /// public static bool RightAlignedMenus { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_MENUDROPALIGNMENT) != 0; } } ////// Gets a value indicating whether drop down menus should be right-aligned with /// the corresponding menu bar item. /// ////// /// public static bool PenWindows { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_PENWINDOWS) != 0; } } ////// Gets a value indicating whether the Microsoft Windows for Pen computing /// extensions are installed. /// ////// /// public static bool DbcsEnabled { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_DBCSENABLED) != 0; } } ////// Gets a value indicating whether the operating system is capable of handling /// double-byte (DBCS) characters. /// ////// /// public static int MouseButtons { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CMOUSEBUTTONS); } } ////// Gets the number of buttons on mouse. /// ////// /// public static bool Secure { get { Debug.WriteLineIf(IntSecurity.SecurityDemand.TraceVerbose, "SensitiveSystemInformation Demanded"); IntSecurity.SensitiveSystemInformation.Demand(); return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_SECURE) != 0; } } ////// Gets a value indicating whether security is present on this operating system. /// ////// /// public static Size Border3DSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXEDGE), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYEDGE)); } } ////// Gets the dimensions in pixels, of a 3-D /// border. /// ////// /// public static Size MinimizedWindowSpacingSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXMINSPACING), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYMINSPACING)); } } ///Gets the dimensions /// in pixels, of /// the grid into which minimized windows will be placed. ////// /// public static Size SmallIconSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXSMICON), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYSMICON)); } } ////// Gets /// the recommended dimensions of a small icon in pixels. /// ////// /// public static int ToolWindowCaptionHeight { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYSMCAPTION); } } ////// Gets the height of /// a small caption in pixels. /// ////// /// public static Size ToolWindowCaptionButtonSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXSMSIZE), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYSMSIZE)); } } ////// Gets the /// dimensions of small caption buttons in pixels. /// ////// /// public static Size MenuButtonSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXMENUSIZE), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYMENUSIZE)); } } ////// Gets /// the dimensions in pixels, of menu bar buttons. /// ////// /// public static ArrangeStartingPosition ArrangeStartingPosition { get { ArrangeStartingPosition mask = ArrangeStartingPosition.BottomLeft | ArrangeStartingPosition.BottomRight | ArrangeStartingPosition.Hide | ArrangeStartingPosition.TopLeft | ArrangeStartingPosition.TopRight; int compoundValue = UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_ARRANGE); return mask & (ArrangeStartingPosition) compoundValue; } } ///Gets flags specifying how the system arranges minimized windows. ////// /// public static ArrangeDirection ArrangeDirection { get { ArrangeDirection mask = ArrangeDirection.Down | ArrangeDirection.Left | ArrangeDirection.Right | ArrangeDirection.Up; int compoundValue = UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_ARRANGE); return mask & (ArrangeDirection) compoundValue; } } ////// Gets flags specifying how the system arranges minimized windows. /// ////// /// public static Size MinimizedWindowSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXMINIMIZED), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYMINIMIZED)); } } ////// Gets the dimensions in pixels, of a normal minimized window. /// ////// /// public static Size MaxWindowTrackSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXMAXTRACK), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYMAXTRACK)); } } ////// Gets the default maximum dimensions in pixels, of a /// window that has a caption and sizing borders. /// ////// /// public static Size PrimaryMonitorMaximizedWindowSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXMAXIMIZED), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYMAXIMIZED)); } } ////// Gets the default dimensions, in pixels, of a maximized top-left window on the /// primary monitor. /// ////// /// public static bool Network { get { return(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_NETWORK) & 0x00000001) != 0; } } ////// Gets a value indicating whether this computer is connected to a network. /// ////// /// public static bool TerminalServerSession { get { return(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_REMOTESESSION) & 0x00000001) != 0; } } ////// To be supplied. /// ////// /// public static BootMode BootMode { get { Debug.WriteLineIf(IntSecurity.SecurityDemand.TraceVerbose, "SensitiveSystemInformation Demanded"); IntSecurity.SensitiveSystemInformation.Demand(); return(BootMode) UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CLEANBOOT); } } ////// Gets a value that specifies how the system was started. /// ////// /// public static Size DragSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXDRAG), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYDRAG)); } } ////// Gets the dimensions in pixels, of the rectangle that a drag operation /// must extend to be considered a drag. The rectangle is centered on a drag /// point. /// ////// /// public static bool ShowSounds { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_SHOWSOUNDS) != 0; } } ////// Gets a value indicating whether the user requires an application to present /// information visually in situations where it would otherwise present the /// information in audible form. /// ////// /// public static Size MenuCheckSize { get { return new Size(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXMENUCHECK), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYMENUCHECK)); } } ////// Gets the /// dimensions of the default size of a menu checkmark in pixels. /// ////// /// public static bool MidEastEnabled { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_MIDEASTENABLED) != 0; } } private static bool MultiMonitorSupport { get { if (!checkMultiMonitorSupport) { multiMonitorSupport = (UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CMONITORS) != 0); checkMultiMonitorSupport = true; } return multiMonitorSupport; } } ////// Gets a value /// indicating whether the system is enabled for Hebrew and Arabic languages. /// ////// /// public static bool NativeMouseWheelSupport { get { if (!checkNativeMouseWheelSupport) { nativeMouseWheelSupport = (UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_MOUSEWHEELPRESENT) != 0); checkNativeMouseWheelSupport = true; } return nativeMouseWheelSupport; } } ////// Gets a value indicating whether the system natively supports the mouse wheel /// in newer mice. /// ////// /// public static bool MouseWheelPresent { get { bool mouseWheelPresent = false; if (!NativeMouseWheelSupport) { IntPtr hWndMouseWheel = IntPtr.Zero; // Check for the MouseZ "service". This is a little app that generated the // MSH_MOUSEWHEEL messages by monitoring the hardware. If this app isn't // found, then there is no support for MouseWheels on the system. // hWndMouseWheel = UnsafeNativeMethods.FindWindow(NativeMethods.MOUSEZ_CLASSNAME, NativeMethods.MOUSEZ_TITLE); if (hWndMouseWheel != IntPtr.Zero) { mouseWheelPresent = true; } } else { mouseWheelPresent = (UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_MOUSEWHEELPRESENT) != 0); } return mouseWheelPresent; } } ////// Gets a value indicating whether there is a mouse with a mouse wheel /// installed on this machine. /// ////// /// public static Rectangle VirtualScreen { get { if (MultiMonitorSupport) { return new Rectangle(UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_XVIRTUALSCREEN), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_YVIRTUALSCREEN), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXVIRTUALSCREEN), UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYVIRTUALSCREEN)); } else { Size size = PrimaryMonitorSize; return new Rectangle(0, 0, size.Width, size.Height); } } } ////// Gets the /// bounds of the virtual screen. /// ////// /// public static int MonitorCount { get { if (MultiMonitorSupport) { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CMONITORS); } else { return 1; } } } ////// Gets the number of display monitors on the desktop. /// ////// /// public static bool MonitorsSameDisplayFormat { get { if (MultiMonitorSupport) { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_SAMEDISPLAYFORMAT) != 0; } else { return true; } } } //////////////////////////////////////////////////////////////////////////// // Misc // ////// Gets a value indicating whether all the display monitors have the /// same color format. /// ////// /// public static string ComputerName { get { Debug.WriteLineIf(IntSecurity.SecurityDemand.TraceVerbose, "SensitiveSystemInformation Demanded"); IntSecurity.SensitiveSystemInformation.Demand(); StringBuilder sb = new StringBuilder(256); UnsafeNativeMethods.GetComputerName(sb, new int[] {sb.Capacity}); return sb.ToString(); } } ////// Gets the computer name of the current system. /// ////// /// Gets the user's domain name. /// public static string UserDomainName { get { return Environment.UserDomainName; } } ////// /// public static bool UserInteractive { get { if (Environment.OSVersion.Platform == System.PlatformID.Win32NT) { IntPtr hwinsta = IntPtr.Zero; hwinsta = UnsafeNativeMethods.GetProcessWindowStation(); if (hwinsta != IntPtr.Zero && processWinStation != hwinsta) { isUserInteractive = true; int lengthNeeded = 0; NativeMethods.USEROBJECTFLAGS flags = new NativeMethods.USEROBJECTFLAGS(); if (UnsafeNativeMethods.GetUserObjectInformation(new HandleRef(null, hwinsta), NativeMethods.UOI_FLAGS, flags, Marshal.SizeOf(flags), ref lengthNeeded)) { if ((flags.dwFlags & NativeMethods.WSF_VISIBLE) == 0) { isUserInteractive = false; } } processWinStation = hwinsta; } } else { isUserInteractive = true; } return isUserInteractive; } } ////// Gets a value indicating whether the current process is running in user /// interactive mode. /// ////// /// public static string UserName { get { Debug.WriteLineIf(IntSecurity.SecurityDemand.TraceVerbose, "SensitiveSystemInformation Demanded"); IntSecurity.SensitiveSystemInformation.Demand(); StringBuilder sb = new StringBuilder(256); UnsafeNativeMethods.GetUserName(sb, new int[] {sb.Capacity}); return sb.ToString(); } } private static void EnsureSystemEvents() { if (!systemEventsAttached) { SystemEvents.UserPreferenceChanged += new UserPreferenceChangedEventHandler(SystemInformation.OnUserPreferenceChanged); systemEventsAttached = true; } } private static void OnUserPreferenceChanged(object sender, UserPreferenceChangedEventArgs pref) { systemEventsDirty = true; } ////////////////////////////////////////////////////////////////////////////////////////////////////////// // NEW ADDITIONS FOR WHIDBEY // ////////////////////////////////////////////////////////////////////////////////////////////////////////// ////// Gets the user name for the current thread, that is, the name of the /// user currently logged onto the system. /// ////// /// public static bool IsDropShadowEnabled { get { if (OSFeature.Feature.OnXp) { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETDROPSHADOW, 0, ref data, 0); return data != 0; } return false; } } ////// Gets a value indicating whether the drop shadow effect in enabled. Defaults to false /// downlevel. /// ////// /// public static bool IsFlatMenuEnabled { get { if (OSFeature.Feature.OnXp) { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETFLATMENU, 0, ref data, 0); return data != 0; } return false; } } ////// Gets a value indicating whether the native user menus have a flat menu appearance. Defaults to false /// downlevel. /// ////// /// public static bool IsFontSmoothingEnabled { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETFONTSMOOTHING, 0, ref data, 0); return data != 0; } } ////// Gets a value indicating whether the Font Smoothing OSFeature.Feature is enabled. /// ////// /// public static int FontSmoothingContrast { get { if (OSFeature.Feature.OnXp) { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETFONTSMOOTHINGCONTRAST, 0, ref data, 0); return data; } else { throw new NotSupportedException(SR.GetString(SR.SystemInformationFeatureNotSupported)); } } } ////// Returns a contrast value that is ClearType smoothing. /// ////// /// public static int FontSmoothingType { get { if (OSFeature.Feature.OnXp) { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETFONTSMOOTHINGTYPE, 0, ref data, 0); return data; } else { throw new NotSupportedException(SR.GetString(SR.SystemInformationFeatureNotSupported)); } } } ////// Returns a type of Font smoothing. /// ////// /// public static int IconHorizontalSpacing { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_ICONHORIZONTALSPACING, 0, ref data, 0); return data; } } ////// Retrieves the width in pixels of an icon cell. /// ////// /// public static int IconVerticalSpacing { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_ICONVERTICALSPACING, 0, ref data, 0); return data; } } ////// Retrieves the height in pixels of an icon cell. /// ////// /// public static bool IsIconTitleWrappingEnabled { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETICONTITLEWRAP, 0, ref data, 0); return data != 0; } } ////// Gets a value indicating whether the Icon title wrapping is enabled. /// ////// /// public static bool MenuAccessKeysUnderlined { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETKEYBOARDCUES, 0, ref data, 0); return data != 0; } } ////// Gets a value indicating whether the menu access keys are always underlined. /// ////// /// public static int KeyboardDelay { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETKEYBOARDDELAY, 0, ref data, 0); return data; } } ////// Retrieves the Keyboard repeat delay setting, which is a value in the /// range from 0 through 3. The Actual Delay Associated with each value may vary depending on the /// hardware. /// ////// /// public static bool IsKeyboardPreferred { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETKEYBOARDPREF, 0, ref data, 0); return data != 0; } } ////// Gets a value indicating whether the user relies on Keyboard instead of mouse and wants /// applications to display keyboard interfaces that would be otherwise hidden. /// ////// /// public static int KeyboardSpeed { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETKEYBOARDSPEED, 0, ref data, 0); return data; } } ////// Retrieves the Keyboard repeat speed setting, which is a value in the /// range from 0 through 31. The actual rate may vary depending on the /// hardware. /// ////// /// public static Size MouseHoverSize { get { int height = 0; int width = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMOUSEHOVERHEIGHT, 0, ref height, 0); UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMOUSEHOVERWIDTH, 0, ref width, 0); return new Size(width, height); } } ////// Gets the Size in pixels of the rectangle within which the mouse pointer has to stay. /// ////// /// public static int MouseHoverTime { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMOUSEHOVERTIME, 0, ref data, 0); return data; } } ////// Gets the time, in milliseconds, that the mouse pointer has to stay in the hover rectangle. /// ////// /// public static int MouseSpeed { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMOUSESPEED, 0, ref data, 0); return data; } } ////// Gets the current mouse speed. /// ////// /// public static bool IsSnapToDefaultEnabled { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETSNAPTODEFBUTTON, 0, ref data, 0); return data != 0; } } ////// Determines whether the snap-to-default-button feature is enabled. /// ////// /// public static LeftRightAlignment PopupMenuAlignment { get { bool data = false; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMENUDROPALIGNMENT, 0, ref data, 0); if (data) { return LeftRightAlignment.Left; } else { return LeftRightAlignment.Right; } } } ////// Determines whether the Popup Menus are left Aligned or Right Aligned. /// ////// /// public static bool IsMenuFadeEnabled { get { if (OSFeature.Feature.OnXp || OSFeature.Feature.OnWin2k) { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMENUFADE, 0, ref data, 0); return data != 0; } return false; } } ////// Determines whether the maenu fade animation feature is enabled. Defaults to false /// downlevel. /// ////// /// public static int MenuShowDelay { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMENUSHOWDELAY, 0, ref data, 0); return data; } } ////// Indicates the time, in milliseconds, that the system waits before displaying a shortcut menu. /// ////// /// public static bool IsComboBoxAnimationEnabled { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETCOMBOBOXANIMATION, 0, ref data, 0); return data != 0; } } ////// Indicates whether the slide open effect for combo boxes is enabled. /// ////// /// public static bool IsTitleBarGradientEnabled { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETGRADIENTCAPTIONS, 0, ref data, 0); return data != 0; } } ////// Indicates whether the gradient effect for windows title bars is enabled. /// ////// /// public static bool IsHotTrackingEnabled { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETHOTTRACKING, 0, ref data, 0); return data != 0; } } ////// Indicates whether the hot tracking of user interface elements is enabled. /// ////// /// public static bool IsListBoxSmoothScrollingEnabled { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETLISTBOXSMOOTHSCROLLING, 0, ref data, 0); return data != 0; } } ////// Indicates whether the smooth scrolling effect for listbox is enabled. /// ////// /// public static bool IsMenuAnimationEnabled { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMENUANIMATION, 0, ref data, 0); return data != 0; } } ////// Indicates whether the menu animation feature is enabled. /// ////// /// public static bool IsSelectionFadeEnabled { get { if (OSFeature.Feature.OnXp || OSFeature.Feature.OnWin2k) { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETSELECTIONFADE, 0, ref data, 0); return data != 0; } return false; } } ////// Indicates whether the selection fade effect is enabled. Defaults to false /// downlevel. /// ////// /// public static bool IsToolTipAnimationEnabled { get { if (OSFeature.Feature.OnXp || OSFeature.Feature.OnWin2k) { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETTOOLTIPANIMATION, 0, ref data, 0); return data != 0; } return false; } } ////// Indicates whether the tool tip animation is enabled. Defaults to false /// downlevel. /// ////// /// public static bool UIEffectsEnabled { get { if (OSFeature.Feature.OnXp || OSFeature.Feature.OnWin2k) { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETUIEFFECTS, 0, ref data, 0); return data != 0; } return false; } } ////// Indicates whether all the UI Effects are enabled. Defaults to false /// downlevel. /// ////// /// public static bool IsActiveWindowTrackingEnabled { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETACTIVEWINDOWTRACKING, 0, ref data, 0); return data != 0; } } ////// Indicates whether the active windows tracking (activating the window the mouse in on) is ON or OFF. /// ////// /// public static int ActiveWindowTrackingDelay { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETACTIVEWNDTRKTIMEOUT, 0, ref data, 0); return data; } } ////// Retrieves the active window tracking delay, in milliseconds. /// ////// /// public static bool IsMinimizeRestoreAnimationEnabled { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETANIMATION, 0, ref data, 0); return data != 0; } } ////// Indicates whether the active windows tracking (activating the window the mouse in on) is ON or OFF. /// ////// /// public static int BorderMultiplierFactor { get { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETBORDER, 0, ref data, 0); return data; } } ////// Retrieves the border multiplier factor that determines the width of a windo's sizing border. /// ////// /// public static int CaretBlinkTime { get { return unchecked((int)SafeNativeMethods.GetCaretBlinkTime()); } } ////// Indicates the caret blink time. /// ////// /// public static int CaretWidth { get { if (OSFeature.Feature.OnXp || OSFeature.Feature.OnWin2k) { int data = 0; UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETCARETWIDTH, 0, ref data, 0); return data; } else { throw new NotSupportedException(SR.GetString(SR.SystemInformationFeatureNotSupported)); } } } ////// Indicates the caret width in edit controls. /// ////// /// public static int MouseWheelScrollDelta { get { return NativeMethods.WHEEL_DELTA; } } ////// None. /// ////// /// public static int VerticalFocusThickness { get { if (OSFeature.Feature.OnXp) { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYFOCUSBORDER); } else { throw new NotSupportedException(SR.GetString(SR.SystemInformationFeatureNotSupported)); } } } ////// The width of the left and right edges of the focus rectangle. /// ////// /// public static int HorizontalFocusThickness { get { if (OSFeature.Feature.OnXp) { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXFOCUSBORDER); } else { throw new NotSupportedException(SR.GetString(SR.SystemInformationFeatureNotSupported)); } } } ////// The width of the top and bottom edges of the focus rectangle. /// ////// /// public static int VerticalResizeBorderThickness { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYSIZEFRAME); } } ////// The height of the vertical sizing border around the perimeter of the window that can be resized. /// ////// /// public static int HorizontalResizeBorderThickness { get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXSIZEFRAME); } } ////// The width of the horizontal sizing border around the perimeter of the window that can be resized. /// ////// /// public static ScreenOrientation ScreenOrientation { get { ScreenOrientation so = ScreenOrientation.Angle0; NativeMethods.DEVMODE dm = new NativeMethods.DEVMODE(); dm.dmSize = (short) Marshal.SizeOf(typeof(NativeMethods.DEVMODE)); dm.dmDriverExtra = 0; try { SafeNativeMethods.EnumDisplaySettings(null, -1 /*ENUM_CURRENT_SETTINGS*/, ref dm); if ( (dm.dmFields & NativeMethods.DM_DISPLAYORIENTATION) > 0) { so = dm.dmDisplayOrientation; } } catch { // empty catch, we'll just return the default if the call to EnumDisplaySettings fails. } return so; } } ////// The orientation of the screen in degrees. /// ////// /// public static int SizingBorderWidth { get { //we can get the system's menu font through the NONCLIENTMETRICS structure via SystemParametersInfo // NativeMethods.NONCLIENTMETRICS data = new NativeMethods.NONCLIENTMETRICS(); bool result = UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETNONCLIENTMETRICS, data.cbSize, data, 0); if (result && data.iBorderWidth > 0) { return data.iBorderWidth; } else { return 0; } } } /* ////// Specifies the thikness, in pixels, of the Sizing Border. /// ////// /// public static int VerticalScrollBarWidth { get { //we can get the system's menu font through the NONCLIENTMETRICS structure via SystemParametersInfo // NativeMethods.NONCLIENTMETRICS data = new NativeMethods.NONCLIENTMETRICS(); UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETNONCLIENTMETRICS, data.cbSize, data, 0); if (result && data.iScrollWidth > 0) { return data.iScrollWidth; } else { return 0; } } } ////// Specified the width, in pixels, of a standard vertical scrollbar. /// ////// /// public static int HorizontalScrollBarWidth { get { //we can get the system's menu font through the NONCLIENTMETRICS structure via SystemParametersInfo // NativeMethods.NONCLIENTMETRICS data = new NativeMethods.NONCLIENTMETRICS(); UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETNONCLIENTMETRICS, data.cbSize, data, 0); if (result && data.iScrollHeight > 0) { return data.iScrollHeight; } else { return 0; } } } ////// Specified the height, in pixels, of a standard horizontal scrollbar. /// ////// /// public static Size CaptionButtonSize { get { //we can get the system's menu font through the NONCLIENTMETRICS structure via SystemParametersInfo // NativeMethods.NONCLIENTMETRICS data = new NativeMethods.NONCLIENTMETRICS(); UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETNONCLIENTMETRICS, data.cbSize, data, 0); if (result && data.iCaptionHeight > 0 && data.iCaptionWidth > 0) { return new Size(data.iCaptionWidth, data.iCaptionHeight); } else { return return new Size(0, 0); } } } */ ////// Specified the Size, in pixels, of the caption buttons. /// ////// /// public static Size SmallCaptionButtonSize { get { //we can get the system's menu font through the NONCLIENTMETRICS structure via SystemParametersInfo // NativeMethods.NONCLIENTMETRICS data = new NativeMethods.NONCLIENTMETRICS(); bool result = UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETNONCLIENTMETRICS, data.cbSize, data, 0); if (result && data.iSmCaptionHeight > 0 && data.iSmCaptionWidth > 0) { return new Size(data.iSmCaptionWidth, data.iSmCaptionHeight); } else { return Size.Empty; } } } ////// Specified the Size, in pixels, of the small caption buttons. /// ////// /// public static Size MenuBarButtonSize { get { //we can get the system's menu font through the NONCLIENTMETRICS structure via SystemParametersInfo // NativeMethods.NONCLIENTMETRICS data = new NativeMethods.NONCLIENTMETRICS(); bool result = UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETNONCLIENTMETRICS, data.cbSize, data, 0); if (result && data.iMenuHeight > 0 && data.iMenuWidth > 0) { return new Size(data.iMenuWidth, data.iMenuHeight); } else { return Size.Empty; } } } ////////////////////////////////////////////////////////////////////////////////////////////////////////// // End ADDITIONS FOR WHIDBEY // ////////////////////////////////////////////////////////////////////////////////////////////////////////// } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Specified the Size, in pixels, of the menu bar buttons. /// ///
Link Menu
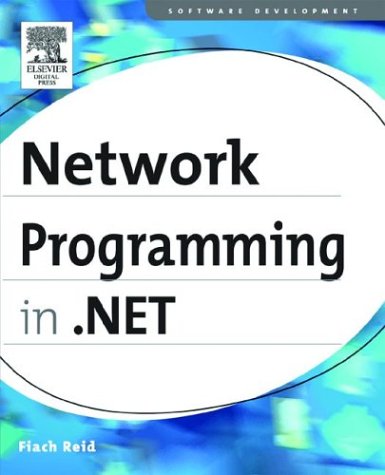
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- contentDescriptor.cs
- ProcessModelSection.cs
- SqlInternalConnection.cs
- ToolStripAdornerWindowService.cs
- PropertyValueUIItem.cs
- ServerType.cs
- StrokeCollectionConverter.cs
- ReceiveActivityDesignerTheme.cs
- EntityDataSourceContainerNameConverter.cs
- TransactionFlowAttribute.cs
- HttpProfileGroupBase.cs
- SqlPersonalizationProvider.cs
- StyleSelector.cs
- PropertyValueChangedEvent.cs
- SiteMapSection.cs
- TargetConverter.cs
- ListMarkerLine.cs
- SecurityKeyIdentifier.cs
- ChildrenQuery.cs
- InstanceContextMode.cs
- ClusterSafeNativeMethods.cs
- FrameworkPropertyMetadata.cs
- CodeDefaultValueExpression.cs
- XmlSchemaObjectTable.cs
- CommentEmitter.cs
- GridViewRowPresenterBase.cs
- FormatPage.cs
- BlockCollection.cs
- MetadataHelper.cs
- PathFigure.cs
- SinglePageViewer.cs
- SliderAutomationPeer.cs
- WindowsEditBoxRange.cs
- XmlTextWriter.cs
- KeyConverter.cs
- WhereaboutsReader.cs
- Literal.cs
- cookiecontainer.cs
- XmlExceptionHelper.cs
- RuntimeIdentifierPropertyAttribute.cs
- AlphabeticalEnumConverter.cs
- FillErrorEventArgs.cs
- ValidationEventArgs.cs
- DataGridToolTip.cs
- InputLanguageManager.cs
- DataRecordInternal.cs
- BindingEntityInfo.cs
- ProxyManager.cs
- SoapCodeExporter.cs
- Operators.cs
- PageContent.cs
- CodeRemoveEventStatement.cs
- TripleDES.cs
- CharUnicodeInfo.cs
- PageCatalogPart.cs
- WindowsScrollBarBits.cs
- HtmlProps.cs
- TargetControlTypeCache.cs
- DropShadowBitmapEffect.cs
- SoapFault.cs
- PropertyConverter.cs
- XmlWriter.cs
- CodeObject.cs
- TreeBuilderBamlTranslator.cs
- CollectionViewGroupInternal.cs
- StrongBox.cs
- HostSecurityManager.cs
- CookieProtection.cs
- ExpressionLink.cs
- BaseValidatorDesigner.cs
- StorageConditionPropertyMapping.cs
- ThreadAbortException.cs
- xmlsaver.cs
- LabelAutomationPeer.cs
- CompensatableTransactionScopeActivityDesigner.cs
- RegisteredArrayDeclaration.cs
- DynamicDiscoveryDocument.cs
- TextServicesManager.cs
- BrowserCapabilitiesCodeGenerator.cs
- TextFormatterContext.cs
- Literal.cs
- ResolveNameEventArgs.cs
- AttributeUsageAttribute.cs
- DataMemberFieldEditor.cs
- Choices.cs
- InnerItemCollectionView.cs
- BulletedListEventArgs.cs
- SqlInternalConnection.cs
- QilNode.cs
- PartDesigner.cs
- RTLAwareMessageBox.cs
- RenderTargetBitmap.cs
- UserValidatedEventArgs.cs
- DbgCompiler.cs
- MenuScrollingVisibilityConverter.cs
- PageAsyncTask.cs
- AuthenticationService.cs
- EdmRelationshipRoleAttribute.cs
- SpotLight.cs
- TransformedBitmap.cs