Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CompMod / System / CodeDOM / Compiler / TempFiles.cs / 1 / TempFiles.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom.Compiler { using System; using System.Collections; using System.Diagnostics; using System.IO; using System.Runtime.InteropServices; using System.Text; using Microsoft.Win32; using System.Security; using System.Security.Permissions; using System.Security.Principal; using System.ComponentModel; using System.Security.Cryptography; using System.Globalization; using System.Runtime.Versioning; ////// [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] [PermissionSet(SecurityAction.InheritanceDemand, Name="FullTrust")] [Serializable] public class TempFileCollection : ICollection, IDisposable { private static RNGCryptoServiceProvider rng; string basePath; string tempDir; bool keepFiles; Hashtable files; static TempFileCollection() { // Since creating the random generator can be expensive, only do it once rng = new RNGCryptoServiceProvider(); } ///Represents a collection of temporary file names that are all based on a /// single base filename located in a temporary directory. ////// public TempFileCollection() : this(null, false) { } ///[To be supplied.] ////// public TempFileCollection(string tempDir) : this(tempDir, false) { } ///[To be supplied.] ////// public TempFileCollection(string tempDir, bool keepFiles) { this.keepFiles = keepFiles; this.tempDir = tempDir; #if !FEATURE_CASE_SENSITIVE_FILESYSTEM files = new Hashtable(StringComparer.OrdinalIgnoreCase); #else files = new Hashtable(); #endif } ///[To be supplied.] ////// /// void IDisposable.Dispose() { Dispose(true); GC.SuppressFinalize(this); } protected virtual void Dispose(bool disposing) { // It is safe to call Delete from here even if Dispose is called from Finalizer // because the graph of objects is guaranteed to be there and // neither Hashtable nor String have a finalizer of their own that could // be called before TempFileCollection Finalizer Delete(); } ///To allow it's stuff to be cleaned up ////// ~TempFileCollection() { Dispose(false); } ///[To be supplied.] ////// public string AddExtension(string fileExtension) { return AddExtension(fileExtension, keepFiles); } ///[To be supplied.] ////// public string AddExtension(string fileExtension, bool keepFile) { if (fileExtension == null || fileExtension.Length == 0) throw new ArgumentException(SR.GetString(SR.InvalidNullEmptyArgument, "fileExtension"), "fileExtension"); // fileExtension not specified string fileName = BasePath + "." + fileExtension; AddFile(fileName, keepFile); return fileName; } ///[To be supplied.] ////// public void AddFile(string fileName, bool keepFile) { if (fileName == null || fileName.Length == 0) throw new ArgumentException(SR.GetString(SR.InvalidNullEmptyArgument, "fileName"), "fileName"); // fileName not specified if (files[fileName] != null) throw new ArgumentException(SR.GetString(SR.DuplicateFileName, fileName), "fileName"); // duplicate fileName files.Add(fileName, (object)keepFile); } ///[To be supplied.] ////// public IEnumerator GetEnumerator() { return files.Keys.GetEnumerator(); } ///[To be supplied.] ///IEnumerator IEnumerable.GetEnumerator() { return files.Keys.GetEnumerator(); } /// void ICollection.CopyTo(Array array, int start) { files.Keys.CopyTo(array, start); } /// /// public void CopyTo(string[] fileNames, int start) { files.Keys.CopyTo(fileNames, start); } ///[To be supplied.] ////// public int Count { get { return files.Count; } } ///[To be supplied.] ///int ICollection.Count { get { return files.Count; } } /// object ICollection.SyncRoot { get { return null; } } /// bool ICollection.IsSynchronized { get { return false; } } /// /// public string TempDir { get { return tempDir == null ? string.Empty : tempDir; } } ///[To be supplied.] ////// public string BasePath { get { EnsureTempNameCreated(); return basePath; } } void EnsureTempNameCreated() { if (basePath == null) { string tempFileName = null; FileStream tempFileStream; bool uniqueFile = false; int retryCount = 5000; do { try { basePath = GetTempFileName(TempDir); string full = basePath; new EnvironmentPermission(PermissionState.Unrestricted).Assert(); try { full = Path.GetFullPath(basePath); } finally { CodeAccessPermission.RevertAssert(); } new FileIOPermission(FileIOPermissionAccess.AllAccess, full).Demand(); // make sure the filename is unique. tempFileName = basePath + ".tmp"; using (tempFileStream = new FileStream(tempFileName, FileMode.CreateNew, FileAccess.Write)) { } uniqueFile = true; } catch (IOException e) { retryCount--; if (retryCount == 0 || Marshal.GetHRForException(e) != NativeMethods.ERROR_FILE_EXISTS) throw; uniqueFile = false; } }while (!uniqueFile); files.Add(tempFileName, keepFiles); } } ///[To be supplied.] ////// public bool KeepFiles { get { return keepFiles; } set { keepFiles = value; } } bool KeepFile(string fileName) { object keep = files[fileName]; if (keep == null) return false; return (bool)keep; } ///[To be supplied.] ////// [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] public void Delete() { if (files != null) { string[] fileNames = new string[files.Count]; files.Keys.CopyTo(fileNames, 0); foreach (string fileName in fileNames) { if (!KeepFile(fileName)) { Delete(fileName); files.Remove(fileName); } } } } // This function deletes files after reverting impersonation. internal void SafeDelete() { #if !FEATURE_PAL WindowsImpersonationContext impersonation = Executor.RevertImpersonation(); #endif try{ Delete(); } finally { #if !FEATURE_PAL Executor.ReImpersonate(impersonation); #endif } } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] void Delete(string fileName) { try { File.Delete(fileName); } catch { // Ignore all exceptions } } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] static string GetTempFileName(string tempDir) { if (tempDir == null || tempDir.Length == 0) { tempDir = Path.GetTempPath(); } string fileName = GenerateRandomFileName(); if (tempDir.EndsWith("\\", StringComparison.Ordinal)) return tempDir + fileName; return tempDir + "\\" + fileName; } // Generate a random file name with 8 characters static string GenerateRandomFileName() { // Generate random bytes byte[] data = new byte[6]; lock (rng) { rng.GetBytes(data); } // Turn them into a string containing only characters valid in file names/url string s = Convert.ToBase64String(data).ToLower(CultureInfo.InvariantCulture); s = s.Replace('/', '-'); s = s.Replace('+', '_'); return s; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom.Compiler { using System; using System.Collections; using System.Diagnostics; using System.IO; using System.Runtime.InteropServices; using System.Text; using Microsoft.Win32; using System.Security; using System.Security.Permissions; using System.Security.Principal; using System.ComponentModel; using System.Security.Cryptography; using System.Globalization; using System.Runtime.Versioning; ////// [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] [PermissionSet(SecurityAction.InheritanceDemand, Name="FullTrust")] [Serializable] public class TempFileCollection : ICollection, IDisposable { private static RNGCryptoServiceProvider rng; string basePath; string tempDir; bool keepFiles; Hashtable files; static TempFileCollection() { // Since creating the random generator can be expensive, only do it once rng = new RNGCryptoServiceProvider(); } ///Represents a collection of temporary file names that are all based on a /// single base filename located in a temporary directory. ////// public TempFileCollection() : this(null, false) { } ///[To be supplied.] ////// public TempFileCollection(string tempDir) : this(tempDir, false) { } ///[To be supplied.] ////// public TempFileCollection(string tempDir, bool keepFiles) { this.keepFiles = keepFiles; this.tempDir = tempDir; #if !FEATURE_CASE_SENSITIVE_FILESYSTEM files = new Hashtable(StringComparer.OrdinalIgnoreCase); #else files = new Hashtable(); #endif } ///[To be supplied.] ////// /// void IDisposable.Dispose() { Dispose(true); GC.SuppressFinalize(this); } protected virtual void Dispose(bool disposing) { // It is safe to call Delete from here even if Dispose is called from Finalizer // because the graph of objects is guaranteed to be there and // neither Hashtable nor String have a finalizer of their own that could // be called before TempFileCollection Finalizer Delete(); } ///To allow it's stuff to be cleaned up ////// ~TempFileCollection() { Dispose(false); } ///[To be supplied.] ////// public string AddExtension(string fileExtension) { return AddExtension(fileExtension, keepFiles); } ///[To be supplied.] ////// public string AddExtension(string fileExtension, bool keepFile) { if (fileExtension == null || fileExtension.Length == 0) throw new ArgumentException(SR.GetString(SR.InvalidNullEmptyArgument, "fileExtension"), "fileExtension"); // fileExtension not specified string fileName = BasePath + "." + fileExtension; AddFile(fileName, keepFile); return fileName; } ///[To be supplied.] ////// public void AddFile(string fileName, bool keepFile) { if (fileName == null || fileName.Length == 0) throw new ArgumentException(SR.GetString(SR.InvalidNullEmptyArgument, "fileName"), "fileName"); // fileName not specified if (files[fileName] != null) throw new ArgumentException(SR.GetString(SR.DuplicateFileName, fileName), "fileName"); // duplicate fileName files.Add(fileName, (object)keepFile); } ///[To be supplied.] ////// public IEnumerator GetEnumerator() { return files.Keys.GetEnumerator(); } ///[To be supplied.] ///IEnumerator IEnumerable.GetEnumerator() { return files.Keys.GetEnumerator(); } /// void ICollection.CopyTo(Array array, int start) { files.Keys.CopyTo(array, start); } /// /// public void CopyTo(string[] fileNames, int start) { files.Keys.CopyTo(fileNames, start); } ///[To be supplied.] ////// public int Count { get { return files.Count; } } ///[To be supplied.] ///int ICollection.Count { get { return files.Count; } } /// object ICollection.SyncRoot { get { return null; } } /// bool ICollection.IsSynchronized { get { return false; } } /// /// public string TempDir { get { return tempDir == null ? string.Empty : tempDir; } } ///[To be supplied.] ////// public string BasePath { get { EnsureTempNameCreated(); return basePath; } } void EnsureTempNameCreated() { if (basePath == null) { string tempFileName = null; FileStream tempFileStream; bool uniqueFile = false; int retryCount = 5000; do { try { basePath = GetTempFileName(TempDir); string full = basePath; new EnvironmentPermission(PermissionState.Unrestricted).Assert(); try { full = Path.GetFullPath(basePath); } finally { CodeAccessPermission.RevertAssert(); } new FileIOPermission(FileIOPermissionAccess.AllAccess, full).Demand(); // make sure the filename is unique. tempFileName = basePath + ".tmp"; using (tempFileStream = new FileStream(tempFileName, FileMode.CreateNew, FileAccess.Write)) { } uniqueFile = true; } catch (IOException e) { retryCount--; if (retryCount == 0 || Marshal.GetHRForException(e) != NativeMethods.ERROR_FILE_EXISTS) throw; uniqueFile = false; } }while (!uniqueFile); files.Add(tempFileName, keepFiles); } } ///[To be supplied.] ////// public bool KeepFiles { get { return keepFiles; } set { keepFiles = value; } } bool KeepFile(string fileName) { object keep = files[fileName]; if (keep == null) return false; return (bool)keep; } ///[To be supplied.] ////// [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] public void Delete() { if (files != null) { string[] fileNames = new string[files.Count]; files.Keys.CopyTo(fileNames, 0); foreach (string fileName in fileNames) { if (!KeepFile(fileName)) { Delete(fileName); files.Remove(fileName); } } } } // This function deletes files after reverting impersonation. internal void SafeDelete() { #if !FEATURE_PAL WindowsImpersonationContext impersonation = Executor.RevertImpersonation(); #endif try{ Delete(); } finally { #if !FEATURE_PAL Executor.ReImpersonate(impersonation); #endif } } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] void Delete(string fileName) { try { File.Delete(fileName); } catch { // Ignore all exceptions } } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] static string GetTempFileName(string tempDir) { if (tempDir == null || tempDir.Length == 0) { tempDir = Path.GetTempPath(); } string fileName = GenerateRandomFileName(); if (tempDir.EndsWith("\\", StringComparison.Ordinal)) return tempDir + fileName; return tempDir + "\\" + fileName; } // Generate a random file name with 8 characters static string GenerateRandomFileName() { // Generate random bytes byte[] data = new byte[6]; lock (rng) { rng.GetBytes(data); } // Turn them into a string containing only characters valid in file names/url string s = Convert.ToBase64String(data).ToLower(CultureInfo.InvariantCulture); s = s.Replace('/', '-'); s = s.Replace('+', '_'); return s; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
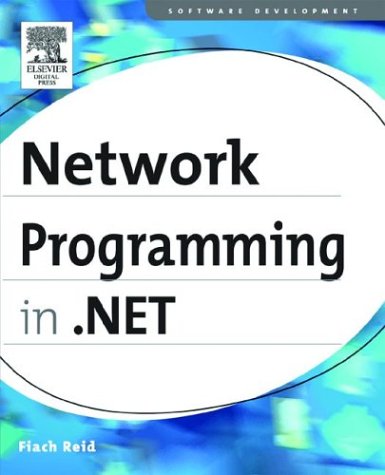
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FormsAuthenticationTicket.cs
- DisplayMemberTemplateSelector.cs
- MachineKeyValidationConverter.cs
- translator.cs
- QueryPageSettingsEventArgs.cs
- EntityDataSourceColumn.cs
- WindowsFormsHost.cs
- PropertyKey.cs
- ClassicBorderDecorator.cs
- AttributeQuery.cs
- HttpSocketManager.cs
- InvokePattern.cs
- PageParser.cs
- StorageBasedPackageProperties.cs
- UpdateManifestForBrowserApplication.cs
- BinaryMethodMessage.cs
- PersonalizationAdministration.cs
- SelectionUIService.cs
- SubMenuStyleCollectionEditor.cs
- Metafile.cs
- XmlCharCheckingWriter.cs
- HtmlEncodedRawTextWriter.cs
- PackWebRequestFactory.cs
- OverflowException.cs
- SerializableAttribute.cs
- InputBuffer.cs
- ByteViewer.cs
- CustomTypeDescriptor.cs
- MultiView.cs
- GroupBoxAutomationPeer.cs
- DbParameterCollectionHelper.cs
- DrawingDrawingContext.cs
- TypeValidationEventArgs.cs
- RelationshipConstraintValidator.cs
- xdrvalidator.cs
- WebPartEditorApplyVerb.cs
- UpDownBaseDesigner.cs
- XpsDigitalSignature.cs
- ExpressionParser.cs
- QuotedPrintableStream.cs
- CollectionViewGroup.cs
- MsmqTransportSecurity.cs
- StringTraceRecord.cs
- MatrixAnimationBase.cs
- XmlHierarchyData.cs
- ExtendedTransformFactory.cs
- TableItemPatternIdentifiers.cs
- UnmanagedMemoryStreamWrapper.cs
- AuthorizationRuleCollection.cs
- CompoundFileStreamReference.cs
- XmlTextReaderImpl.cs
- MSHTMLHost.cs
- X509ChainElement.cs
- XmlMemberMapping.cs
- HideDisabledControlAdapter.cs
- DrawingDrawingContext.cs
- CursorInteropHelper.cs
- DataRecordInfo.cs
- Enum.cs
- CodeComment.cs
- Transform.cs
- AuthorizationRule.cs
- ConnectionConsumerAttribute.cs
- RequestCache.cs
- DatagridviewDisplayedBandsData.cs
- HttpListener.cs
- ReferenceConverter.cs
- Model3DCollection.cs
- GridView.cs
- TransformerTypeCollection.cs
- ScriptIgnoreAttribute.cs
- PropertyManager.cs
- TypeConverter.cs
- ReaderContextStackData.cs
- QueryIntervalOp.cs
- AppSettingsReader.cs
- DateTimeFormatInfoScanner.cs
- TreeNodeCollection.cs
- IChannel.cs
- AsymmetricKeyExchangeFormatter.cs
- Control.cs
- DataGridViewCellStyleConverter.cs
- AnnouncementEventArgs.cs
- LinearGradientBrush.cs
- ClosureBinding.cs
- ApplicationContext.cs
- DefaultHttpHandler.cs
- DataStreams.cs
- ElasticEase.cs
- RenderingBiasValidation.cs
- XPathExpr.cs
- AttributeCollection.cs
- DirectoryInfo.cs
- StringFunctions.cs
- _RegBlobWebProxyDataBuilder.cs
- TextWriter.cs
- ComponentCollection.cs
- ChangeNode.cs
- RoutedEventArgs.cs
- MetadataItemEmitter.cs