Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Map / Update / Internal / ChangeNode.cs / 1 / ChangeNode.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Collections.Generic; using System.Text; using System.Globalization; namespace System.Data.Mapping.Update.Internal { ////// This class encapsulates changes propagated to a node in an update mapping view. /// It contains lists of deleted and inserted rows. Key intersections betweens rows /// in the two sets are treated as updates in the store. /// ////// internal class ChangeNode { #region Constructors ////// Additional tags indicating the roles of particular values (e.g., concurrency, undefined, /// etc.) are stored within each row: where appropriate, constants appearing /// within a row are associated with a ///through the . /// /// The 'leaves' of an update mapping view (UMV) are extent expressions. A change node /// associated with an extent expression is simply the list of changes to the C-Space /// requested by a caller. As changes propagate 'up' the UMV expression tree, we recursively /// apply transformations such that the change node associated with the root of the UMV /// represents changes to apply in the S-Space. /// ////// Constructs a change node containing changes belonging to the specified collection /// schema definition. /// /// Setsproperty. internal ChangeNode(TypeUsage elementType) { m_elementType = elementType; } #endregion #region Fields private TypeUsage m_elementType; private List m_inserted = new List (); private List m_deleted = new List (); private PropagatorResult m_placeholder; #endregion #region Properties /// /// Gets the type of the rows contained in this node. This type corresponds (not coincidentally) /// to the type of an expression in an update mapping view. /// internal TypeUsage ElementType { get { return m_elementType; } } ////// Gets a list of rows to be inserted. /// internal ListInserted { get { return m_inserted; } } /// /// Gets a list of rows to be deleted. /// internal ListDeleted { get { return m_deleted; } } /// /// Gets or sets a version of a record at this node with default record. The record has the type /// of the node we are visiting. /// internal PropagatorResult Placeholder { get { return m_placeholder; } set { m_placeholder = value; } } #endregion #if DEBUG public override string ToString() { StringBuilder builder = new StringBuilder(); builder.AppendLine("{"); builder.AppendFormat(CultureInfo.InvariantCulture, " ElementType = {0}", ElementType).AppendLine(); builder.AppendLine(" Inserted = {"); foreach (PropagatorResult insert in Inserted) { builder.Append(" ").AppendLine(insert.ToString()); } builder.AppendLine(" }"); builder.AppendLine(" Deleted = {"); foreach (PropagatorResult delete in Deleted) { builder.Append(" ").AppendLine(delete.ToString()); } builder.AppendLine(" }"); builder.AppendFormat(CultureInfo.InvariantCulture, " PlaceHolder = {0}", Placeholder).AppendLine(); builder.Append("}"); return builder.ToString(); } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Collections.Generic; using System.Text; using System.Globalization; namespace System.Data.Mapping.Update.Internal { ////// This class encapsulates changes propagated to a node in an update mapping view. /// It contains lists of deleted and inserted rows. Key intersections betweens rows /// in the two sets are treated as updates in the store. /// ////// internal class ChangeNode { #region Constructors ////// Additional tags indicating the roles of particular values (e.g., concurrency, undefined, /// etc.) are stored within each row: where appropriate, constants appearing /// within a row are associated with a ///through the . /// /// The 'leaves' of an update mapping view (UMV) are extent expressions. A change node /// associated with an extent expression is simply the list of changes to the C-Space /// requested by a caller. As changes propagate 'up' the UMV expression tree, we recursively /// apply transformations such that the change node associated with the root of the UMV /// represents changes to apply in the S-Space. /// ////// Constructs a change node containing changes belonging to the specified collection /// schema definition. /// /// Setsproperty. internal ChangeNode(TypeUsage elementType) { m_elementType = elementType; } #endregion #region Fields private TypeUsage m_elementType; private List m_inserted = new List (); private List m_deleted = new List (); private PropagatorResult m_placeholder; #endregion #region Properties /// /// Gets the type of the rows contained in this node. This type corresponds (not coincidentally) /// to the type of an expression in an update mapping view. /// internal TypeUsage ElementType { get { return m_elementType; } } ////// Gets a list of rows to be inserted. /// internal ListInserted { get { return m_inserted; } } /// /// Gets a list of rows to be deleted. /// internal ListDeleted { get { return m_deleted; } } /// /// Gets or sets a version of a record at this node with default record. The record has the type /// of the node we are visiting. /// internal PropagatorResult Placeholder { get { return m_placeholder; } set { m_placeholder = value; } } #endregion #if DEBUG public override string ToString() { StringBuilder builder = new StringBuilder(); builder.AppendLine("{"); builder.AppendFormat(CultureInfo.InvariantCulture, " ElementType = {0}", ElementType).AppendLine(); builder.AppendLine(" Inserted = {"); foreach (PropagatorResult insert in Inserted) { builder.Append(" ").AppendLine(insert.ToString()); } builder.AppendLine(" }"); builder.AppendLine(" Deleted = {"); foreach (PropagatorResult delete in Deleted) { builder.Append(" ").AppendLine(delete.ToString()); } builder.AppendLine(" }"); builder.AppendFormat(CultureInfo.InvariantCulture, " PlaceHolder = {0}", Placeholder).AppendLine(); builder.Append("}"); return builder.ToString(); } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
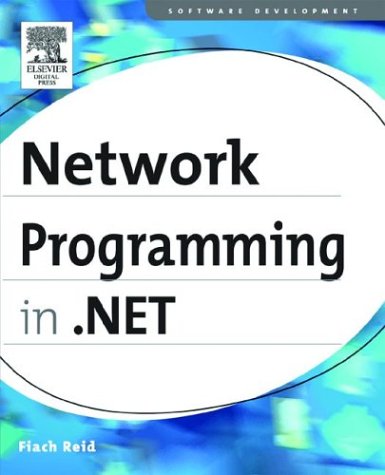
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigurationProperty.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- DetailsViewPagerRow.cs
- OracleConnectionString.cs
- DocumentApplication.cs
- XamlBrushSerializer.cs
- DataBindEngine.cs
- SecurityCriticalDataForSet.cs
- EventDescriptorCollection.cs
- SubMenuStyle.cs
- PropertyEntry.cs
- Rect.cs
- FirewallWrapper.cs
- BrowserCapabilitiesCompiler.cs
- SupportingTokenParameters.cs
- Brush.cs
- RunInstallerAttribute.cs
- ScriptResourceMapping.cs
- ImagingCache.cs
- ProtocolsConfigurationHandler.cs
- ToolStripDropTargetManager.cs
- MarshalByRefObject.cs
- TabControlCancelEvent.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- Polygon.cs
- XmlQueryContext.cs
- RemotingAttributes.cs
- DataServiceProviderMethods.cs
- oledbconnectionstring.cs
- ExceptionUtil.cs
- TypeHelper.cs
- EventLogException.cs
- HostSecurityManager.cs
- DebugView.cs
- DataGridViewComboBoxCell.cs
- UITypeEditor.cs
- DataTableCollection.cs
- IndividualDeviceConfig.cs
- X509RawDataKeyIdentifierClause.cs
- FilteredAttributeCollection.cs
- MaskInputRejectedEventArgs.cs
- SoapTransportImporter.cs
- SafeProcessHandle.cs
- SQLDateTime.cs
- TreeNodeBinding.cs
- FunctionCommandText.cs
- WsatStrings.cs
- ToolStripPanelRow.cs
- ColumnPropertiesGroup.cs
- Adorner.cs
- View.cs
- Operand.cs
- SmiTypedGetterSetter.cs
- EngineSite.cs
- ValidationPropertyAttribute.cs
- AuthenticationService.cs
- BindingOperations.cs
- PassportAuthenticationModule.cs
- UserPersonalizationStateInfo.cs
- GenerateTemporaryTargetAssembly.cs
- EntityCommandCompilationException.cs
- LongValidator.cs
- DataGridViewImageCell.cs
- FixedNode.cs
- SplitterPanelDesigner.cs
- XmlQualifiedName.cs
- ArrayConverter.cs
- ComplexType.cs
- FormClosedEvent.cs
- CdpEqualityComparer.cs
- CallbackValidatorAttribute.cs
- RadioButtonBaseAdapter.cs
- LazyTextWriterCreator.cs
- ExceptionUtility.cs
- VectorAnimationUsingKeyFrames.cs
- RtfNavigator.cs
- NavigationWindow.cs
- FullTextLine.cs
- AttachedAnnotation.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- Resources.Designer.cs
- HtmlUtf8RawTextWriter.cs
- GroupItemAutomationPeer.cs
- ListChangedEventArgs.cs
- DataView.cs
- ChangeInterceptorAttribute.cs
- ImageList.cs
- ToolStripDropDownButton.cs
- IndexOutOfRangeException.cs
- RegularExpressionValidator.cs
- SolidColorBrush.cs
- DescendantBaseQuery.cs
- MaskedTextProvider.cs
- EntityParameterCollection.cs
- NamespaceInfo.cs
- WebRequestModulesSection.cs
- CheckoutException.cs
- sqlstateclientmanager.cs
- LoginUtil.cs
- XmlNamespaceManager.cs