Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / SplitterPanelDesigner.cs / 1 / SplitterPanelDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Design.SplitterPanelDesigner..ctor()")] namespace System.Windows.Forms.Design { using System.Windows.Forms; using System.Design; using System.Runtime.InteropServices; using System.ComponentModel; using System.Collections; using System.Diagnostics; using System; using System.ComponentModel.Design; using System.Drawing; using System.Drawing.Design; using System.Drawing.Drawing2D; using Microsoft.Win32; ////// /// This class handles all design time behavior for the panel class. This /// draws a visible border on the panel if it doesn't have a border so the /// user knows where the boundaries of the panel lie. /// internal class SplitterPanelDesigner : PanelDesigner { private IDesignerHost designerHost; private SplitContainerDesigner splitContainerDesigner; SplitterPanel splitterPanel; private bool selected; public override bool CanBeParentedTo(IDesigner parentDesigner) { return (parentDesigner is SplitContainerDesigner); } protected override InheritanceAttribute InheritanceAttribute { get { if (splitterPanel != null && splitterPanel.Parent != null) { return (InheritanceAttribute)TypeDescriptor.GetAttributes(splitterPanel.Parent)[typeof(InheritanceAttribute)]; } return base.InheritanceAttribute; } } internal bool Selected { get { return selected; } set { selected = value; if (selected) { DrawSelectedBorder(); } else { EraseBorder(); } } } ////// /// Called in response to a drag enter for OLE drag and drop. /// protected override void OnDragEnter(DragEventArgs de) { if (InheritanceAttribute == InheritanceAttribute.InheritedReadOnly) { de.Effect = DragDropEffects.None; return; } base.OnDragEnter(de); } ////// /// Called when a drag drop object is dragged over the control designer view /// protected override void OnDragOver(DragEventArgs de) { if (InheritanceAttribute == InheritanceAttribute.InheritedReadOnly) { de.Effect = DragDropEffects.None; return; } base.OnDragOver(de); } ////// /// Called when a drag-drop operation leaves the control designer view /// /// protected override void OnDragLeave(EventArgs e) { if (InheritanceAttribute == InheritanceAttribute.InheritedReadOnly) { return; } base.OnDragLeave(e); } protected override void OnDragDrop(DragEventArgs de) { if (InheritanceAttribute == InheritanceAttribute.InheritedReadOnly) { de.Effect = DragDropEffects.None; return; } base.OnDragDrop(de); } ////// /// Called when the user hovers over the splitterpanel. Here, we'll internally forward this message /// to the SplitContainerDesigner so we have ContainerGrabHandle functionality for the entire component. /// protected override void OnMouseHover() { if (splitContainerDesigner != null) { splitContainerDesigner.SplitterPanelHover(); } } protected override void Dispose(bool disposing) { IComponentChangeService cs = (IComponentChangeService)GetService(typeof(IComponentChangeService)); if (cs != null) { cs.ComponentChanged -= new ComponentChangedEventHandler(this.OnComponentChanged); } base.Dispose(disposing); } public override void Initialize(IComponent component) { base.Initialize(component); splitterPanel = (SplitterPanel)component; designerHost = (IDesignerHost)component.Site.GetService(typeof(IDesignerHost)); splitContainerDesigner = (SplitContainerDesigner)designerHost.GetDesigner(splitterPanel.Parent); IComponentChangeService cs = (IComponentChangeService)GetService(typeof(IComponentChangeService)); if (cs != null) { cs.ComponentChanged += new ComponentChangedEventHandler(this.OnComponentChanged); } PropertyDescriptor lockedProp = TypeDescriptor.GetProperties(component)["Locked"]; if (lockedProp != null && splitterPanel.Parent is SplitContainer) { lockedProp.SetValue(component, true); } } private void OnComponentChanged(object sender, ComponentChangedEventArgs e) { if (splitterPanel.Parent == null) { return; } if (splitterPanel.Controls.Count == 0) { Graphics g = splitterPanel.CreateGraphics(); DrawWaterMark(g); g.Dispose(); } else { // Erase WaterMark ... splitterPanel.Invalidate(); } } internal void DrawSelectedBorder() { Control ctl = Control; Rectangle rc = ctl.ClientRectangle; using (Graphics g = ctl.CreateGraphics()) { Color penColor; // Black or white pen? Depends on the color of the control. // if (ctl.BackColor.GetBrightness() < .5) { penColor = ControlPaint.Light(ctl.BackColor); } else { penColor = ControlPaint.Dark(ctl.BackColor); } using (Pen pen = new Pen(penColor)) { pen.DashStyle = DashStyle.Dash; rc.Inflate(-4,-4); g.DrawRectangle(pen, rc); } } } internal void EraseBorder() { Control ctl = Control; Rectangle rc = ctl.ClientRectangle; Graphics g = ctl.CreateGraphics(); Color penColor = ctl.BackColor; Pen pen = new Pen(penColor); pen.DashStyle = DashStyle.Dash; rc.Inflate(-4,-4); g.DrawRectangle(pen, rc); pen.Dispose(); g.Dispose(); ctl.Invalidate(); } internal void DrawWaterMark(Graphics g) { Control ctl = Control; Rectangle rc = ctl.ClientRectangle; String name = ctl.Name; using (Font drawFont = new Font("Arial", 8)) { int watermarkX = rc.Width / 2 - (int)g.MeasureString(name,drawFont).Width / 2; int watermarkY = rc.Height / 2 ; TextRenderer.DrawText(g, name, drawFont, new Point(watermarkX, watermarkY), Color.Black, TextFormatFlags.Default); } } protected override void OnPaintAdornments(PaintEventArgs pe) { base.OnPaintAdornments(pe); if (splitterPanel.BorderStyle == BorderStyle.None) { DrawBorder(pe.Graphics); } if (Selected) { DrawSelectedBorder(); } if (splitterPanel.Controls.Count == 0) { DrawWaterMark(pe.Graphics); } } ////// Remove some basic properties that are not supported by the /// SplitterPanel /// /// protected override void PreFilterProperties(IDictionary properties) { base.PreFilterProperties(properties); properties.Remove("Modifiers"); properties.Remove("Locked"); properties.Remove("GenerateMember"); //remove the "(Name)" property from the property grid. foreach(DictionaryEntry de in properties) { PropertyDescriptor p = (PropertyDescriptor)de.Value; if (p.Name.Equals("Name") && p.DesignTimeOnly) { properties[de.Key] =TypeDescriptor.CreateProperty(p.ComponentType, p, BrowsableAttribute.No, DesignerSerializationVisibilityAttribute.Hidden); break; } } } ////// /// Returns a list of SnapLine objects representing interesting /// alignment points for this control. These SnapLines are used /// to assist in the positioning of the control on a parent's /// surface. /// public override IList SnapLines { get { ArrayList snapLines = null; // We only want PaddingSnaplines for splitterpanels. AddPaddingSnapLines(ref snapLines); return snapLines; } } ////// /// Retrieves a set of rules concerning the movement capabilities of a component. /// This should be one or more flags from the SelectionRules class. If no designer /// provides rules for a component, the component will not get any UI services. /// public override SelectionRules SelectionRules { get { SelectionRules rules = SelectionRules.None; Control ctl = Control; if (ctl.Parent is SplitContainer) { rules = SelectionRules.Locked; } return rules; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
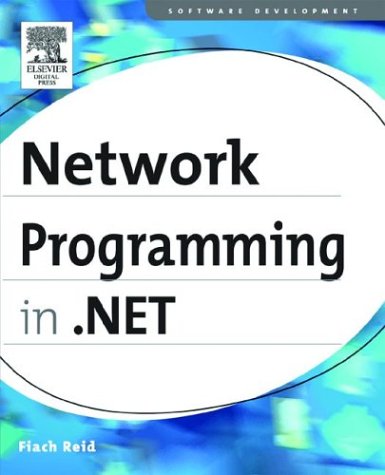
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Binding.cs
- SystemResources.cs
- ToolStripScrollButton.cs
- TextMetrics.cs
- DataAccessor.cs
- XNameTypeConverter.cs
- OrderedDictionary.cs
- TemplateColumn.cs
- ClassImporter.cs
- ServiceSecurityAuditElement.cs
- InputLanguageManager.cs
- NetCodeGroup.cs
- UnsafeNativeMethods.cs
- RelationshipEndCollection.cs
- ModifierKeysValueSerializer.cs
- HttpResponse.cs
- DockingAttribute.cs
- XmlElementAttributes.cs
- basevalidator.cs
- ReachUIElementCollectionSerializer.cs
- MessageHeaderDescription.cs
- XXXOnTypeBuilderInstantiation.cs
- MultipleViewPattern.cs
- GifBitmapDecoder.cs
- SafeEventLogWriteHandle.cs
- Pair.cs
- TemplateBindingExpression.cs
- BoundField.cs
- UInt64Storage.cs
- InputLangChangeRequestEvent.cs
- ZipFileInfo.cs
- ToolStripPanelRow.cs
- ControlPropertyNameConverter.cs
- documentation.cs
- Zone.cs
- SqlMultiplexer.cs
- WebBrowserUriTypeConverter.cs
- X509CertificateValidator.cs
- ComplexTypeEmitter.cs
- Logging.cs
- SponsorHelper.cs
- ApplicationInfo.cs
- TraceXPathNavigator.cs
- SignedXmlDebugLog.cs
- QueueSurrogate.cs
- GroupItem.cs
- SmtpFailedRecipientsException.cs
- ListView.cs
- XmlSiteMapProvider.cs
- SHA256.cs
- MbpInfo.cs
- ImageSource.cs
- KnownTypeAttribute.cs
- XmlParserContext.cs
- XmlNamedNodeMap.cs
- IdentityReference.cs
- CatalogPartCollection.cs
- DateTimeValueSerializer.cs
- WebConfigurationHostFileChange.cs
- MaskedTextBoxTextEditor.cs
- FlowDocumentPage.cs
- GridViewPageEventArgs.cs
- XmlDataCollection.cs
- AutoSizeToolBoxItem.cs
- Figure.cs
- SafeThreadHandle.cs
- ImageDesigner.cs
- AudioFileOut.cs
- SchemaManager.cs
- WebPartZone.cs
- Cloud.cs
- xml.cs
- ModelToObjectValueConverter.cs
- ChannelFactoryBase.cs
- StringAttributeCollection.cs
- RedirectionProxy.cs
- PeerCredential.cs
- SelectionRangeConverter.cs
- CheckBox.cs
- PKCS1MaskGenerationMethod.cs
- DocumentCollection.cs
- TraceHandlerErrorFormatter.cs
- Panel.cs
- SchemaSetCompiler.cs
- SplitContainer.cs
- HostingEnvironmentSection.cs
- SettingsProviderCollection.cs
- RuntimeHelpers.cs
- AssemblyCollection.cs
- controlskin.cs
- RegisteredExpandoAttribute.cs
- PlaceHolder.cs
- XmlILAnnotation.cs
- TemplateBamlRecordReader.cs
- ColumnMapCopier.cs
- TextEditorCopyPaste.cs
- UrlPath.cs
- PassportAuthentication.cs
- Identifier.cs
- ControlAdapter.cs