Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebControls / ControlParameter.cs / 1305376 / ControlParameter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.ComponentModel; using System.Data; using System.Web.UI.WebControls; ////// Represents a Parameter that gets its value from a control's property. /// [ DefaultProperty("ControlID"), ] public class ControlParameter : Parameter { ////// Creates an instance of the ControlParameter class. /// public ControlParameter() { } ////// Creates an instance of the ControlParameter class with the specified parameter name and control ID. /// public ControlParameter(string name, string controlID) : base(name) { ControlID = controlID; } ////// Creates an instance of the ControlParameter class with the specified parameter name, control ID, and property name. /// public ControlParameter(string name, string controlID, string propertyName) : base(name) { ControlID = controlID; PropertyName = propertyName; } ////// Creates an instance of the ControlParameter class with the specified parameter name, database type, /// control ID, and property name. /// public ControlParameter(string name, DbType dbType, string controlID, string propertyName) : base(name, dbType) { ControlID = controlID; PropertyName = propertyName; } ////// Creates an instance of the ControlParameter class with the specified parameter name, type, control ID, and property name. /// public ControlParameter(string name, TypeCode type, string controlID, string propertyName) : base(name, type) { ControlID = controlID; PropertyName = propertyName; } ////// Used to clone a parameter. /// protected ControlParameter(ControlParameter original) : base(original) { ControlID = original.ControlID; PropertyName = original.PropertyName; } ////// The ID of the control to get the value from. /// [ DefaultValue(""), IDReferenceProperty(), RefreshProperties(RefreshProperties.All), TypeConverter(typeof(ControlIDConverter)), WebCategory("Control"), WebSysDescription(SR.ControlParameter_ControlID), ] public string ControlID { get { object o = ViewState["ControlID"]; if (o == null) return String.Empty; return (string)o; } set { if (ControlID != value) { ViewState["ControlID"] = value; OnParameterChanged(); } } } ////// The name of the control's property to get the value from. /// If none is specified, the ControlValueProperty attribute of the control will be examined to determine the default property name. /// [ DefaultValue(""), TypeConverter(typeof(ControlPropertyNameConverter)), WebCategory("Control"), WebSysDescription(SR.ControlParameter_PropertyName), ] public string PropertyName { get { object o = ViewState["PropertyName"]; if (o == null) return String.Empty; return (string)o; } set { if (PropertyName != value) { ViewState["PropertyName"] = value; OnParameterChanged(); } } } ////// Creates a new ControlParameter that is a copy of this ControlParameter. /// protected override Parameter Clone() { return new ControlParameter(this); } ////// Returns the updated value of the parameter. /// protected internal override object Evaluate(HttpContext context, Control control) { if (control == null) { return null; } string controlID = ControlID; string propertyName = PropertyName; if (controlID.Length == 0) { throw new ArgumentException(SR.GetString(SR.ControlParameter_ControlIDNotSpecified, Name)); } Control foundControl = DataBoundControlHelper.FindControl(control, controlID); if (foundControl == null) { throw new InvalidOperationException(SR.GetString(SR.ControlParameter_CouldNotFindControl, controlID, Name)); } ControlValuePropertyAttribute controlValueProp = (ControlValuePropertyAttribute)TypeDescriptor.GetAttributes(foundControl)[typeof(ControlValuePropertyAttribute)]; // If no property name is specified, use the ControlValuePropertyAttribute to determine which property to use. if (propertyName.Length == 0) { if ((controlValueProp != null) && (!String.IsNullOrEmpty(controlValueProp.Name))) { propertyName = controlValueProp.Name; } else { throw new InvalidOperationException(SR.GetString(SR.ControlParameter_PropertyNameNotSpecified, controlID, Name)); } } // Get the value of the property object value = DataBinder.Eval(foundControl, propertyName); // Convert the value to null if this is the default property and the value is the property's default value if (controlValueProp != null && String.Equals(controlValueProp.Name, propertyName, StringComparison.OrdinalIgnoreCase) && controlValueProp.DefaultValue != null && controlValueProp.DefaultValue.Equals(value)) { return null; } return value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
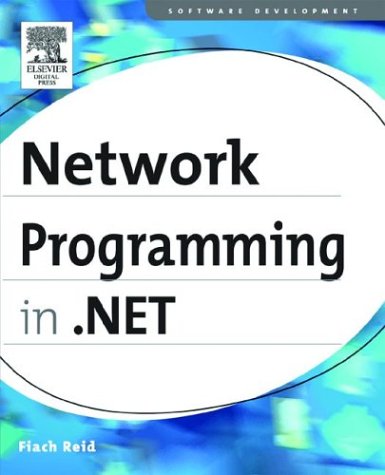
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LinkLabel.cs
- RootAction.cs
- Point3DCollectionValueSerializer.cs
- XPathQueryGenerator.cs
- BamlLocalizabilityResolver.cs
- ValueType.cs
- ParserStreamGeometryContext.cs
- SmtpDigestAuthenticationModule.cs
- BitmapEffectState.cs
- FrameDimension.cs
- EntityDataSourceMemberPath.cs
- SecurityTokenValidationException.cs
- TrustLevel.cs
- StorageConditionPropertyMapping.cs
- Image.cs
- ClientData.cs
- NativeMethods.cs
- Reference.cs
- DefinitionUpdate.cs
- DataGridViewCellValidatingEventArgs.cs
- SettingsAttributes.cs
- WindowsComboBox.cs
- KoreanLunisolarCalendar.cs
- ImportCatalogPart.cs
- PeerIPHelper.cs
- PlatformCulture.cs
- RuleInfoComparer.cs
- SymmetricKey.cs
- RefExpr.cs
- EventSinkHelperWriter.cs
- ConfigurationStrings.cs
- SHA512.cs
- ToggleButtonAutomationPeer.cs
- GlyphInfoList.cs
- DataControlPagerLinkButton.cs
- DesignBindingValueUIHandler.cs
- Brush.cs
- WebControlAdapter.cs
- NativeMethodsOther.cs
- ImageListUtils.cs
- DatatypeImplementation.cs
- loginstatus.cs
- AssociatedControlConverter.cs
- TypeSource.cs
- ServiceModelSecurityTokenRequirement.cs
- XmlToDatasetMap.cs
- FigureParaClient.cs
- _ListenerResponseStream.cs
- DesignerVerbCollection.cs
- ProtocolsConfiguration.cs
- ProfileBuildProvider.cs
- SqlUnionizer.cs
- FormatConvertedBitmap.cs
- Vector3DIndependentAnimationStorage.cs
- TrustManagerPromptUI.cs
- RecognizedWordUnit.cs
- FixedDSBuilder.cs
- CheckBoxStandardAdapter.cs
- ServiceAuthorizationManager.cs
- ToolConsole.cs
- UmAlQuraCalendar.cs
- SortDescription.cs
- OraclePermission.cs
- XmlUrlEditor.cs
- ManagedIStream.cs
- VariableExpressionConverter.cs
- StateRuntime.cs
- SyndicationSerializer.cs
- SimpleExpression.cs
- AdapterUtil.cs
- MachineKeySection.cs
- ProcessModuleCollection.cs
- TargetConverter.cs
- xml.cs
- XPathMultyIterator.cs
- Expression.cs
- Translator.cs
- StateDesigner.CommentLayoutGlyph.cs
- COM2Enum.cs
- RoutedEventValueSerializer.cs
- XmlSchemaInclude.cs
- StructuralObject.cs
- TablePatternIdentifiers.cs
- PrintPageEvent.cs
- SystemDropShadowChrome.cs
- DataBindingExpressionBuilder.cs
- FlowDocumentReader.cs
- HtmlContainerControl.cs
- DtrList.cs
- Scalars.cs
- UnicastIPAddressInformationCollection.cs
- CircleHotSpot.cs
- RangeValidator.cs
- UnsafeNativeMethodsPenimc.cs
- Transform3DCollection.cs
- CryptoProvider.cs
- RelationshipEndCollection.cs
- SqlDataSourceTableQuery.cs
- ConstructorArgumentAttribute.cs
- SessionPageStatePersister.cs