Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / ButtonInternal / CheckBoxStandardAdapter.cs / 1 / CheckBoxStandardAdapter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ButtonInternal { using System; using System.Diagnostics; using System.Drawing; using System.Drawing.Drawing2D; using System.Drawing.Imaging; using System.Drawing.Text; using System.Windows.Forms; using System.Windows.Forms.Layout; internal sealed class CheckBoxStandardAdapter : CheckBoxBaseAdapter { internal CheckBoxStandardAdapter(ButtonBase control) : base(control) {} internal override void PaintUp(PaintEventArgs e, CheckState state) { if (Control.Appearance == Appearance.Button) { ButtonAdapter.PaintUp(e, Control.CheckState); } else { ColorData colors = PaintRender(e.Graphics).Calculate(); LayoutData layout = Layout(e).Layout(); PaintButtonBackground(e, Control.ClientRectangle, null); //minor adjustment to make sure the appearance is exactly the same as Win32 app. int focusRectFixup = layout.focus.X & 0x1; // if it's odd, subtract one pixel for fixup. if (!Application.RenderWithVisualStyles) { focusRectFixup = 1 - focusRectFixup; } if (!layout.options.everettButtonCompat) { layout.textBounds.Offset(-1, -1); } layout.imageBounds.Offset(-1, -1); layout.focus.Offset(-(focusRectFixup+1), -2); layout.focus.Width = layout.textBounds.Width + layout.imageBounds.Width - 1; layout.focus.Intersect(layout.textBounds); if( layout.options.textAlign != LayoutUtils.AnyLeft && layout.options.useCompatibleTextRendering && layout.options.font.Italic) { // fixup for GDI+ text rendering. VSW#515164 layout.focus.Width += 2; } PaintImage(e, layout); DrawCheckBox(e, layout); PaintField(e, layout, colors, colors.windowText, true); } } internal override void PaintDown(PaintEventArgs e, CheckState state) { if (Control.Appearance == Appearance.Button) { ButtonAdapter.PaintDown(e, Control.CheckState); } else { PaintUp(e, state); } } internal override void PaintOver(PaintEventArgs e, CheckState state) { if (Control.Appearance == Appearance.Button) { ButtonAdapter.PaintOver(e, Control.CheckState); } else { PaintUp(e, state); } } internal override Size GetPreferredSizeCore(Size proposedSize) { if (Control.Appearance == Appearance.Button) { ButtonStandardAdapter adapter = new ButtonStandardAdapter(Control); return adapter.GetPreferredSizeCore(proposedSize); } else { using (Graphics measurementGraphics = WindowsFormsUtils.CreateMeasurementGraphics()) { using (PaintEventArgs pe = new PaintEventArgs(measurementGraphics, new Rectangle())) { LayoutOptions options = Layout(pe); return options.GetPreferredSizeCore(proposedSize); } } } } #region Layout private new ButtonStandardAdapter ButtonAdapter { get { return ((ButtonStandardAdapter)base.ButtonAdapter); } } protected override ButtonBaseAdapter CreateButtonAdapter() { return new ButtonStandardAdapter(Control); } protected override LayoutOptions Layout(PaintEventArgs e) { LayoutOptions layout = CommonLayout(); layout.checkPaddingSize = 1; layout.everettButtonCompat = !Application.RenderWithVisualStyles; // VSWhidbey 420870 if (Application.RenderWithVisualStyles) { using (Graphics g = WindowsFormsUtils.CreateMeasurementGraphics()) { layout.checkSize = CheckBoxRenderer.GetGlyphSize(g, CheckBoxRenderer.ConvertFromButtonState(GetState(), true, Control.MouseIsOver)).Width; } } return layout; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
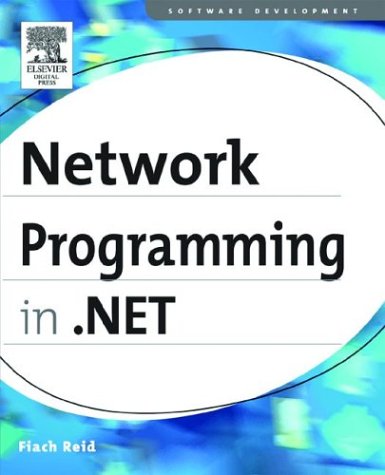
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DetailsViewPageEventArgs.cs
- ParseElementCollection.cs
- TargetInvocationException.cs
- GradientBrush.cs
- HtmlElementErrorEventArgs.cs
- WebServiceMethodData.cs
- DataRelation.cs
- HttpRuntimeSection.cs
- EventlogProvider.cs
- CornerRadiusConverter.cs
- DrawingState.cs
- GetImportedCardRequest.cs
- ButtonAutomationPeer.cs
- SectionRecord.cs
- RemoteWebConfigurationHost.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- ColorTranslator.cs
- LogSwitch.cs
- TrustManagerMoreInformation.cs
- OrderedEnumerableRowCollection.cs
- SqlDataRecord.cs
- PropertyDescriptor.cs
- BatchStream.cs
- SerializerWriterEventHandlers.cs
- ISessionStateStore.cs
- TrackBarRenderer.cs
- Message.cs
- BinaryHeap.cs
- XPathNavigatorReader.cs
- SQLInt16Storage.cs
- ListControl.cs
- StructuredTypeEmitter.cs
- MultipleViewProviderWrapper.cs
- NativeMethods.cs
- ValidatingPropertiesEventArgs.cs
- WindowsSpinner.cs
- CardSpaceSelector.cs
- DispatcherProcessingDisabled.cs
- DeploymentExceptionMapper.cs
- HandleCollector.cs
- WinFormsUtils.cs
- StatementContext.cs
- WindowsAuthenticationEventArgs.cs
- FontEditor.cs
- HttpEncoder.cs
- ColumnResizeUndoUnit.cs
- ChangeInterceptorAttribute.cs
- ProbeDuplex11AsyncResult.cs
- GlyphRunDrawing.cs
- WindowsToolbar.cs
- BrushConverter.cs
- WriteStateInfoBase.cs
- MailAddress.cs
- loginstatus.cs
- EventBuilder.cs
- TCPClient.cs
- HttpDebugHandler.cs
- WebPartMinimizeVerb.cs
- VisualStyleTypesAndProperties.cs
- _AutoWebProxyScriptEngine.cs
- EncodingTable.cs
- BrushMappingModeValidation.cs
- ErrorStyle.cs
- RoutingExtensionElement.cs
- ThreadExceptionDialog.cs
- StrokeCollection2.cs
- StoreAnnotationsMap.cs
- AssemblyCollection.cs
- XmlAttributeAttribute.cs
- GeneralTransformGroup.cs
- XmlTextAttribute.cs
- DesignerImageAdapter.cs
- RegisteredDisposeScript.cs
- SetUserLanguageRequest.cs
- WebHeaderCollection.cs
- ButtonRenderer.cs
- PointLight.cs
- MetabaseSettings.cs
- ModuleBuilder.cs
- SmiEventStream.cs
- DeferredSelectedIndexReference.cs
- CustomPopupPlacement.cs
- DebuggerService.cs
- DefaultTextStore.cs
- WebServiceHandlerFactory.cs
- odbcmetadatacollectionnames.cs
- TextEditorContextMenu.cs
- TabPage.cs
- TaskExtensions.cs
- FixedSOMContainer.cs
- DiscoveryProxy.cs
- QueueProcessor.cs
- StateMachine.cs
- DefaultParameterValueAttribute.cs
- InstallHelper.cs
- ImageIndexConverter.cs
- COSERVERINFO.cs
- ScrollItemProviderWrapper.cs
- WinFormsSpinner.cs
- QueryRewriter.cs