Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Description / ServiceCredentials.cs / 1 / ServiceCredentials.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Description { using System.Collections.Generic; using System.Collections.ObjectModel; using System.ServiceModel.Channels; using System.ServiceModel.Dispatcher; using System.IdentityModel.Selectors; using System.Runtime.Serialization; using System.ServiceModel.Security; using System.Net; using System.Security.Principal; using System.ServiceModel.Security.Tokens; using System.Security.Cryptography.X509Certificates; using System.Web.Security; public class ServiceCredentials : SecurityCredentialsManager, IServiceBehavior { UserNamePasswordServiceCredential userName; X509CertificateInitiatorServiceCredential clientCertificate; X509CertificateRecipientServiceCredential serviceCertificate; WindowsServiceCredential windows; IssuedTokenServiceCredential issuedToken; PeerCredential peer; SecureConversationServiceCredential secureConversation; public ServiceCredentials() { this.userName = new UserNamePasswordServiceCredential(); this.clientCertificate = new X509CertificateInitiatorServiceCredential(); this.serviceCertificate = new X509CertificateRecipientServiceCredential(); this.windows = new WindowsServiceCredential(); this.issuedToken = new IssuedTokenServiceCredential(); this.peer = new PeerCredential(); this.secureConversation = new SecureConversationServiceCredential(); } protected ServiceCredentials(ServiceCredentials other) { if (other == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("other"); } this.userName = new UserNamePasswordServiceCredential(other.userName); this.clientCertificate = new X509CertificateInitiatorServiceCredential(other.clientCertificate); this.serviceCertificate = new X509CertificateRecipientServiceCredential(other.serviceCertificate); this.windows = new WindowsServiceCredential(other.windows); this.issuedToken = new IssuedTokenServiceCredential(other.issuedToken); this.peer = new PeerCredential(other.peer); this.secureConversation = new SecureConversationServiceCredential(other.secureConversation); } public UserNamePasswordServiceCredential UserNameAuthentication { get { return this.userName; } } public X509CertificateInitiatorServiceCredential ClientCertificate { get { return this.clientCertificate; } } public X509CertificateRecipientServiceCredential ServiceCertificate { get { return this.serviceCertificate; } } public WindowsServiceCredential WindowsAuthentication { get { return this.windows; } } public IssuedTokenServiceCredential IssuedTokenAuthentication { get { return this.issuedToken; } } public PeerCredential Peer { get { return this.peer; } } public SecureConversationServiceCredential SecureConversationAuthentication { get { return this.secureConversation; } } internal static ServiceCredentials CreateDefaultCredentials() { return new ServiceCredentials(); } public override SecurityTokenManager CreateSecurityTokenManager() { return new ServiceCredentialsSecurityTokenManager(this.Clone()); } protected virtual ServiceCredentials CloneCore() { return new ServiceCredentials(this); } public ServiceCredentials Clone() { ServiceCredentials result = CloneCore(); if (result == null || result.GetType() != this.GetType()) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException(SR.GetString(SR.CloneNotImplementedCorrectly, this.GetType(), (result != null)? result.ToString() : "null"))); } return result; } void IServiceBehavior.Validate(ServiceDescription description, ServiceHostBase serviceHostBase) { } void IServiceBehavior.AddBindingParameters(ServiceDescription description, ServiceHostBase serviceHostBase, Collectionendpoints, BindingParameterCollection parameters) { if (parameters == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("parameters"); } // throw if bindingParameters already has a SecurityCredentialsManager SecurityCredentialsManager otherCredentialsManager = parameters.Find (); if (otherCredentialsManager != null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.MultipleSecurityCredentialsManagersInServiceBindingParameters, otherCredentialsManager))); } parameters.Add(this); } void IServiceBehavior.ApplyDispatchBehavior(ServiceDescription description, ServiceHostBase serviceHostBase) { } internal void MakeReadOnly() { this.ClientCertificate.MakeReadOnly(); this.IssuedTokenAuthentication.MakeReadOnly(); this.Peer.MakeReadOnly(); this.SecureConversationAuthentication.MakeReadOnly(); this.ServiceCertificate.MakeReadOnly(); this.UserNameAuthentication.MakeReadOnly(); this.WindowsAuthentication.MakeReadOnly(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
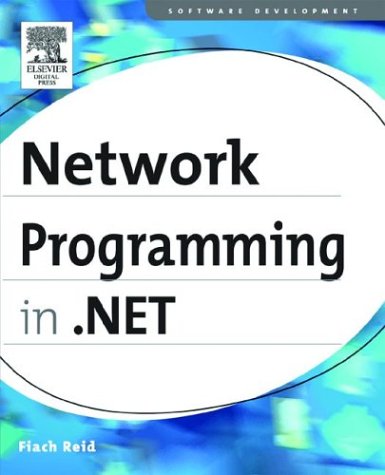
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompositeKey.cs
- XmlSchemaSubstitutionGroup.cs
- EventBuilder.cs
- Ops.cs
- EntitySqlQueryBuilder.cs
- DataGridViewToolTip.cs
- PersianCalendar.cs
- XsdDuration.cs
- CodeCatchClauseCollection.cs
- BuilderInfo.cs
- MachineKeyConverter.cs
- TranslateTransform.cs
- TextEditorCopyPaste.cs
- ParameterBuilder.cs
- HttpListenerContext.cs
- RPIdentityRequirement.cs
- AxisAngleRotation3D.cs
- RequestNavigateEventArgs.cs
- ViewGenResults.cs
- HttpRawResponse.cs
- DataError.cs
- ProtectedConfiguration.cs
- DataGridItemCollection.cs
- TaiwanLunisolarCalendar.cs
- CmsInterop.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- TileBrush.cs
- ColorAnimationBase.cs
- FontFamily.cs
- RootNamespaceAttribute.cs
- HandlerBase.cs
- AssociationTypeEmitter.cs
- SizeValueSerializer.cs
- arc.cs
- ArraySortHelper.cs
- SiteMapHierarchicalDataSourceView.cs
- StyleCollection.cs
- PlainXmlSerializer.cs
- FixedTextPointer.cs
- GeometryValueSerializer.cs
- WorkflowApplicationTerminatedException.cs
- TemplateBindingExtension.cs
- HttpListenerContext.cs
- AccessDataSource.cs
- Header.cs
- XsltArgumentList.cs
- AppDomainGrammarProxy.cs
- _SslState.cs
- CssStyleCollection.cs
- sqlpipe.cs
- ADRoleFactory.cs
- SourceLineInfo.cs
- WebPartHeaderCloseVerb.cs
- PersistenceProviderDirectory.cs
- SetterBase.cs
- BamlRecords.cs
- NestPullup.cs
- DocumentPageTextView.cs
- ResourcePermissionBaseEntry.cs
- DescriptionAttribute.cs
- ReflectionHelper.cs
- WriteLine.cs
- Track.cs
- DoubleCollection.cs
- ObjectSecurity.cs
- DropShadowBitmapEffect.cs
- Stroke.cs
- TextTreeObjectNode.cs
- ListParagraph.cs
- ValuePatternIdentifiers.cs
- MouseBinding.cs
- log.cs
- XmlStreamStore.cs
- XPathNodeInfoAtom.cs
- GridViewRow.cs
- FunctionImportMapping.cs
- ArrayTypeMismatchException.cs
- DesignTimeTemplateParser.cs
- TextSimpleMarkerProperties.cs
- InfiniteTimeSpanConverter.cs
- SqlRetyper.cs
- MarkupExtensionSerializer.cs
- WSHttpBindingElement.cs
- Expander.cs
- AsyncDataRequest.cs
- DropShadowBitmapEffect.cs
- HttpPostProtocolReflector.cs
- EventSource.cs
- ColumnHeaderConverter.cs
- LiteralLink.cs
- PropagatorResult.cs
- StylusSystemGestureEventArgs.cs
- SecurityStandardsManager.cs
- FixedSOMLineRanges.cs
- TraceListener.cs
- DataContractJsonSerializerOperationBehavior.cs
- TableLayoutColumnStyleCollection.cs
- SAPIEngineTypes.cs
- XamlReader.cs
- VariableDesigner.xaml.cs