Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Administration / EndpointInfo.cs / 1 / EndpointInfo.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Administration { using System; using System.Collections.Generic; using System.ServiceModel.Channels; using System.ServiceModel; using System.ServiceModel.Description; using System.Diagnostics; using System.Runtime.Serialization; using System.ServiceModel.Diagnostics; internal sealed class EndpointInfo { Uri address; KeyedByTypeCollectionbehaviors; EndpointIdentity identity; AddressHeaderCollection headers; CustomBinding binding; ContractDescription contract; ServiceEndpoint endpoint; string serviceName; internal EndpointInfo(ServiceEndpoint endpoint, string serviceName) { DiagnosticUtility.DebugAssert(null != endpoint, "endpoint cannot be null"); this.endpoint = endpoint; this.address = endpoint.Address.Uri; this.headers = endpoint.Address.Headers; this.identity = endpoint.Address.Identity; this.behaviors = endpoint.Behaviors; this.serviceName = serviceName; this.binding = null == endpoint.Binding ? new CustomBinding() : new CustomBinding(endpoint.Binding); this.contract = endpoint.Contract; } public Uri Address { get { return this.address; } } public Uri ListenUri { get { return null != this.Endpoint.ListenUri ? this.Endpoint.ListenUri : this.Address; } } public KeyedByTypeCollection Behaviors { get { return this.behaviors; } } public ContractDescription Contract { get { return this.contract; } } public CustomBinding Binding { get { return this.binding; } } public ServiceEndpoint Endpoint { get { return this.endpoint; } } public AddressHeaderCollection Headers { get { return this.headers; } } public EndpointIdentity Identity { get { return this.identity; } } public string Name { get { return this.ServiceName + "." + this.Contract.Name + "@" + this.Address.AbsoluteUri; } } public string ServiceName { get { return this.serviceName; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
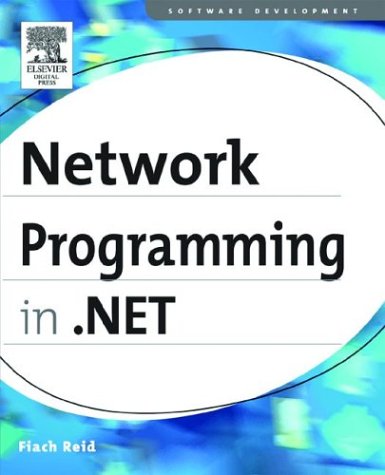
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SplitterEvent.cs
- XmlStreamStore.cs
- MediaElement.cs
- ClientOptions.cs
- Empty.cs
- TreeNodeBindingCollection.cs
- RightsManagementEncryptedStream.cs
- HttpCookieCollection.cs
- InternalException.cs
- WebSysDefaultValueAttribute.cs
- ExtentCqlBlock.cs
- BufferedReadStream.cs
- IteratorFilter.cs
- Repeater.cs
- CommandBinding.cs
- TrackBarRenderer.cs
- PerfProviderCollection.cs
- ContextMenu.cs
- AssemblyBuilder.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- Logging.cs
- Typography.cs
- RenderDataDrawingContext.cs
- TextTreeUndo.cs
- AlgoModule.cs
- ColorConvertedBitmapExtension.cs
- ToolboxDataAttribute.cs
- EdmType.cs
- VectorCollectionConverter.cs
- SplitterEvent.cs
- XmlNodeChangedEventArgs.cs
- Material.cs
- PnrpPermission.cs
- TextStore.cs
- RequestCacheManager.cs
- AppDomain.cs
- DataPagerFieldItem.cs
- WebConfigurationFileMap.cs
- KnownBoxes.cs
- ProcessManager.cs
- XamlSerializer.cs
- _NegoStream.cs
- MessageSecurityVersionConverter.cs
- DynamicValidator.cs
- BamlResourceDeserializer.cs
- ItemContainerGenerator.cs
- BaseResourcesBuildProvider.cs
- Int32RectValueSerializer.cs
- MergeLocalizationDirectives.cs
- OletxVolatileEnlistment.cs
- LocatorPartList.cs
- ZipIOExtraFieldPaddingElement.cs
- DataErrorValidationRule.cs
- GridPattern.cs
- PropertyRecord.cs
- Attributes.cs
- CalendarDateRange.cs
- FragmentQueryProcessor.cs
- Deflater.cs
- CommandValueSerializer.cs
- DeferredSelectedIndexReference.cs
- SudsCommon.cs
- RtfFormatStack.cs
- DrawingAttributes.cs
- OleDbRowUpdatedEvent.cs
- GraphicsPathIterator.cs
- AdornerLayer.cs
- BulletChrome.cs
- UniqueIdentifierService.cs
- ipaddressinformationcollection.cs
- smtpconnection.cs
- RefType.cs
- SimpleModelProvider.cs
- SiteMembershipCondition.cs
- OdbcError.cs
- RawMouseInputReport.cs
- VideoDrawing.cs
- ImportedNamespaceContextItem.cs
- SelectionRange.cs
- XmlSerializationReader.cs
- AppSecurityManager.cs
- RichTextBox.cs
- SqlDataSourceFilteringEventArgs.cs
- XmlArrayItemAttribute.cs
- UnsafeMethods.cs
- Line.cs
- MetadataPropertyAttribute.cs
- ComplexTypeEmitter.cs
- Hyperlink.cs
- RawTextInputReport.cs
- ComUdtElementCollection.cs
- StorageSetMapping.cs
- DispatcherProcessingDisabled.cs
- connectionpool.cs
- ColorMap.cs
- ConditionCollection.cs
- DragCompletedEventArgs.cs
- BamlTreeNode.cs
- RadioButtonBaseAdapter.cs
- AccessorTable.cs