Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Base / System / Windows / Converters / Generated / Int32RectValueSerializer.cs / 2 / Int32RectValueSerializer.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.WindowsBase; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Windows.Markup; using System.Windows.Converters; using System.Windows; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Converters { ////// Int32RectValueSerializer - ValueSerializer class for converting instances of strings to and from Int32Rect instances /// This is used by the MarkupWriter class. /// public class Int32RectValueSerializer : ValueSerializer { ////// Returns true. /// public override bool CanConvertFromString(string value, IValueSerializerContext context) { return true; } ////// Returns true if the given value can be converted into a string /// public override bool CanConvertToString(object value, IValueSerializerContext context) { // Validate the input type if (!(value is Int32Rect)) { return false; } return true; } ////// Converts a string into a Int32Rect. /// public override object ConvertFromString(string value, IValueSerializerContext context) { if (value != null) { return Int32Rect.Parse(value ); } else { return base.ConvertFromString( value, context ); } } ////// Converts the value into a string. /// public override string ConvertToString(object value, IValueSerializerContext context) { if (value is Int32Rect) { Int32Rect instance = (Int32Rect) value; #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, System.Windows.Markup.TypeConverterHelper.EnglishUSCulture ); } return base.ConvertToString(value, context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.WindowsBase; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Windows.Markup; using System.Windows.Converters; using System.Windows; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Converters { ////// Int32RectValueSerializer - ValueSerializer class for converting instances of strings to and from Int32Rect instances /// This is used by the MarkupWriter class. /// public class Int32RectValueSerializer : ValueSerializer { ////// Returns true. /// public override bool CanConvertFromString(string value, IValueSerializerContext context) { return true; } ////// Returns true if the given value can be converted into a string /// public override bool CanConvertToString(object value, IValueSerializerContext context) { // Validate the input type if (!(value is Int32Rect)) { return false; } return true; } ////// Converts a string into a Int32Rect. /// public override object ConvertFromString(string value, IValueSerializerContext context) { if (value != null) { return Int32Rect.Parse(value ); } else { return base.ConvertFromString( value, context ); } } ////// Converts the value into a string. /// public override string ConvertToString(object value, IValueSerializerContext context) { if (value is Int32Rect) { Int32Rect instance = (Int32Rect) value; #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, System.Windows.Markup.TypeConverterHelper.EnglishUSCulture ); } return base.ConvertToString(value, context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
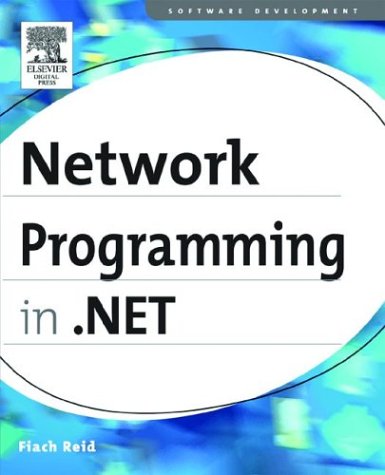
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FontCacheLogic.cs
- SchemaType.cs
- StreamingContext.cs
- SpotLight.cs
- PropertyEmitterBase.cs
- GridViewDeletedEventArgs.cs
- TypeDefinition.cs
- ErrorEventArgs.cs
- XPathNodePointer.cs
- ScriptControlManager.cs
- LinqDataView.cs
- DataGridViewCheckBoxCell.cs
- IisNotInstalledException.cs
- Matrix3DConverter.cs
- XMLUtil.cs
- SmiEventSink.cs
- WebPartChrome.cs
- Predicate.cs
- SystemThemeKey.cs
- DeferredBinaryDeserializerExtension.cs
- ComplexPropertyEntry.cs
- PolicyException.cs
- OutputCacheModule.cs
- SortableBindingList.cs
- Missing.cs
- WorkItem.cs
- WebBrowserUriTypeConverter.cs
- ClockController.cs
- InplaceBitmapMetadataWriter.cs
- NetPipeSectionData.cs
- HtmlFormParameterWriter.cs
- ArcSegment.cs
- BrowserDefinition.cs
- ColorConvertedBitmap.cs
- Comparer.cs
- Mapping.cs
- RtfToken.cs
- ExceptionUtil.cs
- PackageDigitalSignatureManager.cs
- MetabaseServerConfig.cs
- CodeDomSerializer.cs
- XmlSiteMapProvider.cs
- DataViewManager.cs
- CheckBox.cs
- DbMetaDataColumnNames.cs
- VerticalAlignConverter.cs
- XamlSerializerUtil.cs
- SoapFaultCodes.cs
- ParserContext.cs
- Pen.cs
- SqlDataReaderSmi.cs
- XomlCompilerError.cs
- MatcherBuilder.cs
- AsymmetricKeyExchangeFormatter.cs
- ApplicationInterop.cs
- DataTableTypeConverter.cs
- SqlAliasesReferenced.cs
- XamlInterfaces.cs
- ButtonStandardAdapter.cs
- LogStore.cs
- UrlUtility.cs
- ISAPIApplicationHost.cs
- RichTextBoxContextMenu.cs
- Window.cs
- CodeMemberEvent.cs
- FormViewDeletedEventArgs.cs
- Accessors.cs
- TokenizerHelper.cs
- XmlReflectionMember.cs
- ImageFormatConverter.cs
- WebServiceErrorEvent.cs
- VirtualPathProvider.cs
- BrushValueSerializer.cs
- AuthenticationServiceManager.cs
- RegexCaptureCollection.cs
- CompilerErrorCollection.cs
- BufferModeSettings.cs
- CodeDirectiveCollection.cs
- ResXDataNode.cs
- ISAPIRuntime.cs
- GridViewCellAutomationPeer.cs
- WhitespaceRuleLookup.cs
- AccessDataSourceView.cs
- SqlDependencyListener.cs
- AsyncOperation.cs
- InheritanceRules.cs
- Parser.cs
- DbTransaction.cs
- Internal.cs
- MatrixValueSerializer.cs
- ConvertTextFrag.cs
- RegisteredArrayDeclaration.cs
- WindowsStatusBar.cs
- SRDisplayNameAttribute.cs
- AccessibleObject.cs
- FlowDocumentPageViewerAutomationPeer.cs
- TemplateBamlTreeBuilder.cs
- ResolveNameEventArgs.cs
- CompositeScriptReference.cs
- TitleStyle.cs