Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / OpenTypes / OpenTypeMethods.cs / 1305376 / OpenTypeMethods.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// OpenTypeMethods methods used for queries over open types. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { #region Namespaces using System; using System.Collections.Generic; using System.Data.Services.Providers; using System.Diagnostics; using System.Linq.Expressions; using System.Reflection; #endregion ///Use this class to perform late-bound operations on open properties. public static class OpenTypeMethods { #region Reflection OpenType MethodInfos ///MethodInfo for Add. internal static readonly MethodInfo AddMethodInfo = typeof(OpenTypeMethods).GetMethod("Add", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for AndAlso. internal static readonly MethodInfo AndAlsoMethodInfo = typeof(OpenTypeMethods).GetMethod("AndAlso", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Convert. internal static readonly MethodInfo ConvertMethodInfo = typeof(OpenTypeMethods).GetMethod("Convert", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Divide. internal static readonly MethodInfo DivideMethodInfo = typeof(OpenTypeMethods).GetMethod("Divide", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Equal. internal static readonly MethodInfo EqualMethodInfo = typeof(OpenTypeMethods).GetMethod("Equal", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for GreaterThan. internal static readonly MethodInfo GreaterThanMethodInfo = typeof(OpenTypeMethods).GetMethod("GreaterThan", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for GreaterThanOrEqual. internal static readonly MethodInfo GreaterThanOrEqualMethodInfo = typeof(OpenTypeMethods).GetMethod("GreaterThanOrEqual", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for LessThan. internal static readonly MethodInfo LessThanMethodInfo = typeof(OpenTypeMethods).GetMethod("LessThan", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for LessThanOrEqual. internal static readonly MethodInfo LessThanOrEqualMethodInfo = typeof(OpenTypeMethods).GetMethod("LessThanOrEqual", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Modulo. internal static readonly MethodInfo ModuloMethodInfo = typeof(OpenTypeMethods).GetMethod("Modulo", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Multiply. internal static readonly MethodInfo MultiplyMethodInfo = typeof(OpenTypeMethods).GetMethod("Multiply", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Negate. internal static readonly MethodInfo NegateMethodInfo = typeof(OpenTypeMethods).GetMethod("Negate", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Not. internal static readonly MethodInfo NotMethodInfo = typeof(OpenTypeMethods).GetMethod("Not", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for NotEqual. internal static readonly MethodInfo NotEqualMethodInfo = typeof(OpenTypeMethods).GetMethod("NotEqual", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for OrElse. internal static readonly MethodInfo OrElseMethodInfo = typeof(OpenTypeMethods).GetMethod("OrElse", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Subtract. internal static readonly MethodInfo SubtractMethodInfo = typeof(OpenTypeMethods).GetMethod("Subtract", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for TypeIs. internal static readonly MethodInfo TypeIsMethodInfo = typeof(OpenTypeMethods).GetMethod("TypeIs", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for object OpenTypeMethods.GetValue(this object value, string propertyName). internal static readonly MethodInfo GetValueOpenPropertyMethodInfo = typeof(OpenTypeMethods).GetMethod( "GetValue", BindingFlags.Static | BindingFlags.Public, null, new Type[] { typeof(object), typeof(string) }, null); #endregion Internal fields. #region Property Accessor ///Gets a named value from the specified object. /// Object to get value from. /// Name of property to get. ///The requested value; null if not found. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object GetValue(object value, string propertyName) { throw new NotImplementedException(); } #endregion #region binary operators ///Adds two values with no overflow checking. /// Left value.Right value. ///The added value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Add(object left, object right) { throw new NotImplementedException(); } ///Performs logical and of two expressions. /// Left value.Right value. ///The result of logical and. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object AndAlso(object left, object right) { throw new NotImplementedException(); } ///Divides two values. /// Left value.Right value. ///The divided value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Divide(object left, object right) { throw new NotImplementedException(); } ///Checks whether two values are equal. /// Left value.Right value. ///true if left equals right; false otherwise. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Equal(object left, object right) { throw new NotImplementedException(); } ///Checks whether the left value is greater than the right value. /// Left value.Right value. ///true if left is greater than right; false otherwise. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object GreaterThan(object left, object right) { throw new NotImplementedException(); } ///Checks whether the left value is greater than or equal to the right value. /// Left value.Right value. ///true if left is greater than or equal to right; false otherwise. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object GreaterThanOrEqual(object left, object right) { throw new NotImplementedException(); } ///Checks whether the left value is less than the right value. /// Left value.Right value. ///true if left is less than right; false otherwise. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object LessThan(object left, object right) { throw new NotImplementedException(); } ///Checks whether the left value is less than or equal to the right value. /// Left value.Right value. ///true if left is less than or equal to right; false otherwise. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object LessThanOrEqual(object left, object right) { throw new NotImplementedException(); } ///Calculates the remainder of dividing the left value by the right value. /// Left value.Right value. ///The remainder value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Modulo(object left, object right) { throw new NotImplementedException(); } ///Multiplies two values with no overflow checking. /// Left value.Right value. ///The multiplication value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Multiply(object left, object right) { throw new NotImplementedException(); } ///Checks whether two values are not equal. /// Left value.Right value. ///true if left is does not equal right; false otherwise. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object NotEqual(object left, object right) { throw new NotImplementedException(); } ///Performs logical or of two expressions. /// Left value.Right value. ///The result of logical or. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object OrElse(object left, object right) { throw new NotImplementedException(); } ///Subtracts the right value from the left value. /// Left value.Right value. ///The subtraction value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Subtract(object left, object right) { throw new NotImplementedException(); } #endregion #region unary operators ///Negates (arithmetically) the specified value. /// Value. ///The negated value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Negate(object value) { throw new NotImplementedException(); } ///Negates (logically) the specified value. /// Value. ///The negated value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Not(object value) { throw new NotImplementedException(); } #endregion #region Type Conversions ///Performs an type cast on the specified value. /// Value. /// Resource type to check for. ///Casted value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Convert(object value, ResourceType type) { throw new NotImplementedException(); } ///Performs an type check on the specified value. /// Value. /// Resource type to check for. ///True if value is-a type; false otherwise. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object TypeIs(object value, ResourceType type) { throw new NotImplementedException(); } #endregion #region Canonical functions #region String functions ////// Concats the given 2 string. /// /// first string. /// second string. ///returns a new instance of the concatenated string. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Concat(object first, object second) { throw new NotImplementedException(); } ////// Checks with the parameters are of string type, if no, then they throw. /// Otherwise returns true if the target string ends with the given sub string /// /// target string /// sub string ///Returns true if the target string ends with the given sub string, otherwise return false. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object EndsWith(object targetString, object substring) { throw new NotImplementedException(); } ////// Returns the index of the given substring in the target string. /// /// target string /// sub string to match ///returns the index of the given substring in the target string if present, otherwise returns null. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object IndexOf(object targetString, object substring) { throw new NotImplementedException(); } ////// Returns the length of the given string value. If the value is not of string type, then it throws. /// /// value whose length needs to be calculated. ///length of the string value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Length(object value) { throw new NotImplementedException(); } ////// Replaces the given substring with the new string in the target string. /// /// target string /// substring to be replaced. /// new string that replaces the sub string. ///returns a new string with the substring replaced with new string. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Replace(object targetString, object substring, object newString) { throw new NotImplementedException(); } ////// Checks whether the target string starts with the substring. /// /// target string. /// substring ///returns true if the target string starts with the given sub string, otherwise returns false. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object StartsWith(object targetString, object substring) { throw new NotImplementedException(); } ////// Returns the substring given the starting index /// /// target string /// starting index for the substring. ///the substring given the starting index. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Substring(object targetString, object startIndex) { throw new NotImplementedException(); } ////// Returns the substring from the target string. /// /// target string. /// starting index for the substring. /// length of the substring. ///Returns the substring given the starting index and length. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Substring(object targetString, object startIndex, object length) { throw new NotImplementedException(); } ////// Checks whether the given string is a substring of the target string. /// /// substring to check for. /// target string. ///returns true if the target string contains the substring, otherwise returns false. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object SubstringOf(object substring, object targetString) { throw new NotImplementedException(); } ////// Returns a copy of the target string converted to lowercase. /// /// target string ///a new string instance with everything in lowercase. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Globalization", "CA1308", Justification = "Need to support ToLower function")] public static object ToLower(object targetString) { throw new NotImplementedException(); } ////// Returns a copy of the target string converted to uppercase. /// /// target string ///a new string instance with everything in uppercase. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object ToUpper(object targetString) { throw new NotImplementedException(); } ////// Removes all leading and trailing white-space characters from the target string. /// /// target string. ///returns the trimed string. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Trim(object targetString) { throw new NotImplementedException(); } #endregion #region Datetime functions ////// Returns the year value of the given datetime. /// /// datetime object. ///returns the year value of the given datetime. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Year(object dateTime) { throw new NotImplementedException(); } ////// Returns the month value of the given datetime. /// /// datetime object. ///returns the month value of the given datetime. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Month(object dateTime) { throw new NotImplementedException(); } ////// Returns the day value of the given datetime. /// /// datetime object. ///returns the day value of the given datetime. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Day(object dateTime) { throw new NotImplementedException(); } ////// Returns the hour value of the given datetime. /// /// datetime object. ///returns the hour value of the given datetime. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Hour(object dateTime) { throw new NotImplementedException(); } ////// Returns the minute value of the given datetime. /// /// datetime object. ///returns the minute value of the given datetime. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Minute(object dateTime) { throw new NotImplementedException(); } ////// Returns the second value of the given datetime. /// /// datetime object. ///returns the second value of the given datetime. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Second(object dateTime) { throw new NotImplementedException(); } #endregion #region Numeric functions ////// Returns the ceiling of the given value /// /// decimal or double object. ///returns the ceiling value for the given double or decimal value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Ceiling(object value) { throw new NotImplementedException(); } ////// returns the floor of the given value. /// /// decimal or double object. ///returns the floor value for the given double or decimal value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Floor(object value) { throw new NotImplementedException(); } ////// Rounds the given value. /// /// decimal or double object. ///returns the round value for the given double or decimal value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Round(object value) { throw new NotImplementedException(); } #endregion #endregion #region Factory methods for expression tree nodes. ///Creates an expression that adds two values with no overflow checking. /// Left value.Right value. ///The added value. internal static Expression AddExpression(Expression left, Expression right) { return Expression.Add( ExpressionAsObject(left), ExpressionAsObject(right), AddMethodInfo); } ///Creates a call expression that represents a conditional AND operation that evaluates the second operand only if it has to. /// Left value.Right value. ///The conditional expression; null if the expressions aren't of the right type. internal static Expression AndAlsoExpression(Expression left, Expression right) { return Expression.Call( OpenTypeMethods.AndAlsoMethodInfo, ExpressionAsObject(left), ExpressionAsObject(right)); } ///Creates an expression that divides two values. /// Left value.Right value. ///The divided value. internal static Expression DivideExpression(Expression left, Expression right) { return Expression.Divide( ExpressionAsObject(left), ExpressionAsObject(right), DivideMethodInfo); } ///Creates an expression that checks whether two values are equal. /// Left value.Right value. ///true if left equals right; false otherwise. internal static Expression EqualExpression(Expression left, Expression right) { return Expression.Equal( ExpressionAsObject(left), ExpressionAsObject(right), false, EqualMethodInfo); } ///Creates an expression that checks whether the left value is greater than the right value. /// Left value.Right value. ///true if left is greater than right; false otherwise. internal static Expression GreaterThanExpression(Expression left, Expression right) { return Expression.GreaterThan( ExpressionAsObject(left), ExpressionAsObject(right), false, GreaterThanMethodInfo); } ///Creates an expression that checks whether the left value is greater than or equal to the right value. /// Left value.Right value. ///true if left is greater than or equal to right; false otherwise. internal static Expression GreaterThanOrEqualExpression(Expression left, Expression right) { return Expression.GreaterThanOrEqual( ExpressionAsObject(left), ExpressionAsObject(right), false, GreaterThanOrEqualMethodInfo); } ///Creates an expression that checks whether the left value is less than the right value. /// Left value.Right value. ///true if left is less than right; false otherwise. internal static Expression LessThanExpression(Expression left, Expression right) { return Expression.LessThan( ExpressionAsObject(left), ExpressionAsObject(right), false, LessThanMethodInfo); } ///Creates an expression that checks whether the left value is less than or equal to the right value. /// Left value.Right value. ///true if left is less than or equal to right; false otherwise. internal static Expression LessThanOrEqualExpression(Expression left, Expression right) { return Expression.LessThanOrEqual( ExpressionAsObject(left), ExpressionAsObject(right), false, LessThanOrEqualMethodInfo); } ///Creates an expression that calculates the remainder of dividing the left value by the right value. /// Left value.Right value. ///The remainder value. internal static Expression ModuloExpression(Expression left, Expression right) { return Expression.Modulo( ExpressionAsObject(left), ExpressionAsObject(right), ModuloMethodInfo); } ///Creates an expression that multiplies two values with no overflow checking. /// Left value.Right value. ///The multiplication value. internal static Expression MultiplyExpression(Expression left, Expression right) { return Expression.Multiply( ExpressionAsObject(left), ExpressionAsObject(right), MultiplyMethodInfo); } ///Creates a call expression that represents a conditional OR operation that evaluates the second operand only if it has to. /// Left value.Right value. ///The conditional expression; null if the expressions aren't of the right type. internal static Expression OrElseExpression(Expression left, Expression right) { return Expression.Call( OpenTypeMethods.OrElseMethodInfo, ExpressionAsObject(left), ExpressionAsObject(right)); } ///Creates an expression that checks whether two values are not equal. /// Left value.Right value. ///true if left is does not equal right; false otherwise. internal static Expression NotEqualExpression(Expression left, Expression right) { return Expression.NotEqual( ExpressionAsObject(left), ExpressionAsObject(right), false, NotEqualMethodInfo); } ///Creates an expression that subtracts the right value from the left value. /// Left value.Right value. ///The subtraction value. internal static Expression SubtractExpression(Expression left, Expression right) { return Expression.Subtract( ExpressionAsObject(left), ExpressionAsObject(right), SubtractMethodInfo); } ///Creates an expression that negates (arithmetically) the specified value. /// Value expression. ///The negated value. internal static Expression NegateExpression(Expression expression) { return Expression.Negate( ExpressionAsObject(expression), NegateMethodInfo); } ///Creates an expression that negates (logically) the specified value. /// Value expression. ///The negated value. internal static Expression NotExpression(Expression expression) { return Expression.Not( ExpressionAsObject(expression), NotMethodInfo); } #endregion Factory methods for expression tree nodes. #region Helper methods ////// Returns the specified /// Expression to convert. ///with a /// type assignable to System.Object. /// /// The specified private static Expression ExpressionAsObject(Expression expression) { Debug.Assert(expression != null, "expression != null"); return expression.Type.IsValueType ? Expression.Convert(expression, typeof(object)) : expression; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //with a type assignable /// to System.Object. /// // Copyright (c) Microsoft Corporation. All rights reserved. // //// OpenTypeMethods methods used for queries over open types. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { #region Namespaces using System; using System.Collections.Generic; using System.Data.Services.Providers; using System.Diagnostics; using System.Linq.Expressions; using System.Reflection; #endregion ///Use this class to perform late-bound operations on open properties. public static class OpenTypeMethods { #region Reflection OpenType MethodInfos ///MethodInfo for Add. internal static readonly MethodInfo AddMethodInfo = typeof(OpenTypeMethods).GetMethod("Add", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for AndAlso. internal static readonly MethodInfo AndAlsoMethodInfo = typeof(OpenTypeMethods).GetMethod("AndAlso", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Convert. internal static readonly MethodInfo ConvertMethodInfo = typeof(OpenTypeMethods).GetMethod("Convert", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Divide. internal static readonly MethodInfo DivideMethodInfo = typeof(OpenTypeMethods).GetMethod("Divide", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Equal. internal static readonly MethodInfo EqualMethodInfo = typeof(OpenTypeMethods).GetMethod("Equal", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for GreaterThan. internal static readonly MethodInfo GreaterThanMethodInfo = typeof(OpenTypeMethods).GetMethod("GreaterThan", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for GreaterThanOrEqual. internal static readonly MethodInfo GreaterThanOrEqualMethodInfo = typeof(OpenTypeMethods).GetMethod("GreaterThanOrEqual", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for LessThan. internal static readonly MethodInfo LessThanMethodInfo = typeof(OpenTypeMethods).GetMethod("LessThan", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for LessThanOrEqual. internal static readonly MethodInfo LessThanOrEqualMethodInfo = typeof(OpenTypeMethods).GetMethod("LessThanOrEqual", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Modulo. internal static readonly MethodInfo ModuloMethodInfo = typeof(OpenTypeMethods).GetMethod("Modulo", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Multiply. internal static readonly MethodInfo MultiplyMethodInfo = typeof(OpenTypeMethods).GetMethod("Multiply", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Negate. internal static readonly MethodInfo NegateMethodInfo = typeof(OpenTypeMethods).GetMethod("Negate", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Not. internal static readonly MethodInfo NotMethodInfo = typeof(OpenTypeMethods).GetMethod("Not", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for NotEqual. internal static readonly MethodInfo NotEqualMethodInfo = typeof(OpenTypeMethods).GetMethod("NotEqual", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for OrElse. internal static readonly MethodInfo OrElseMethodInfo = typeof(OpenTypeMethods).GetMethod("OrElse", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for Subtract. internal static readonly MethodInfo SubtractMethodInfo = typeof(OpenTypeMethods).GetMethod("Subtract", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for TypeIs. internal static readonly MethodInfo TypeIsMethodInfo = typeof(OpenTypeMethods).GetMethod("TypeIs", BindingFlags.Static | BindingFlags.Public); ///MethodInfo for object OpenTypeMethods.GetValue(this object value, string propertyName). internal static readonly MethodInfo GetValueOpenPropertyMethodInfo = typeof(OpenTypeMethods).GetMethod( "GetValue", BindingFlags.Static | BindingFlags.Public, null, new Type[] { typeof(object), typeof(string) }, null); #endregion Internal fields. #region Property Accessor ///Gets a named value from the specified object. /// Object to get value from. /// Name of property to get. ///The requested value; null if not found. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object GetValue(object value, string propertyName) { throw new NotImplementedException(); } #endregion #region binary operators ///Adds two values with no overflow checking. /// Left value.Right value. ///The added value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Add(object left, object right) { throw new NotImplementedException(); } ///Performs logical and of two expressions. /// Left value.Right value. ///The result of logical and. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object AndAlso(object left, object right) { throw new NotImplementedException(); } ///Divides two values. /// Left value.Right value. ///The divided value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Divide(object left, object right) { throw new NotImplementedException(); } ///Checks whether two values are equal. /// Left value.Right value. ///true if left equals right; false otherwise. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Equal(object left, object right) { throw new NotImplementedException(); } ///Checks whether the left value is greater than the right value. /// Left value.Right value. ///true if left is greater than right; false otherwise. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object GreaterThan(object left, object right) { throw new NotImplementedException(); } ///Checks whether the left value is greater than or equal to the right value. /// Left value.Right value. ///true if left is greater than or equal to right; false otherwise. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object GreaterThanOrEqual(object left, object right) { throw new NotImplementedException(); } ///Checks whether the left value is less than the right value. /// Left value.Right value. ///true if left is less than right; false otherwise. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object LessThan(object left, object right) { throw new NotImplementedException(); } ///Checks whether the left value is less than or equal to the right value. /// Left value.Right value. ///true if left is less than or equal to right; false otherwise. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object LessThanOrEqual(object left, object right) { throw new NotImplementedException(); } ///Calculates the remainder of dividing the left value by the right value. /// Left value.Right value. ///The remainder value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Modulo(object left, object right) { throw new NotImplementedException(); } ///Multiplies two values with no overflow checking. /// Left value.Right value. ///The multiplication value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Multiply(object left, object right) { throw new NotImplementedException(); } ///Checks whether two values are not equal. /// Left value.Right value. ///true if left is does not equal right; false otherwise. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object NotEqual(object left, object right) { throw new NotImplementedException(); } ///Performs logical or of two expressions. /// Left value.Right value. ///The result of logical or. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object OrElse(object left, object right) { throw new NotImplementedException(); } ///Subtracts the right value from the left value. /// Left value.Right value. ///The subtraction value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Subtract(object left, object right) { throw new NotImplementedException(); } #endregion #region unary operators ///Negates (arithmetically) the specified value. /// Value. ///The negated value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Negate(object value) { throw new NotImplementedException(); } ///Negates (logically) the specified value. /// Value. ///The negated value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Not(object value) { throw new NotImplementedException(); } #endregion #region Type Conversions ///Performs an type cast on the specified value. /// Value. /// Resource type to check for. ///Casted value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Convert(object value, ResourceType type) { throw new NotImplementedException(); } ///Performs an type check on the specified value. /// Value. /// Resource type to check for. ///True if value is-a type; false otherwise. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object TypeIs(object value, ResourceType type) { throw new NotImplementedException(); } #endregion #region Canonical functions #region String functions ////// Concats the given 2 string. /// /// first string. /// second string. ///returns a new instance of the concatenated string. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Concat(object first, object second) { throw new NotImplementedException(); } ////// Checks with the parameters are of string type, if no, then they throw. /// Otherwise returns true if the target string ends with the given sub string /// /// target string /// sub string ///Returns true if the target string ends with the given sub string, otherwise return false. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object EndsWith(object targetString, object substring) { throw new NotImplementedException(); } ////// Returns the index of the given substring in the target string. /// /// target string /// sub string to match ///returns the index of the given substring in the target string if present, otherwise returns null. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object IndexOf(object targetString, object substring) { throw new NotImplementedException(); } ////// Returns the length of the given string value. If the value is not of string type, then it throws. /// /// value whose length needs to be calculated. ///length of the string value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Length(object value) { throw new NotImplementedException(); } ////// Replaces the given substring with the new string in the target string. /// /// target string /// substring to be replaced. /// new string that replaces the sub string. ///returns a new string with the substring replaced with new string. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Replace(object targetString, object substring, object newString) { throw new NotImplementedException(); } ////// Checks whether the target string starts with the substring. /// /// target string. /// substring ///returns true if the target string starts with the given sub string, otherwise returns false. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object StartsWith(object targetString, object substring) { throw new NotImplementedException(); } ////// Returns the substring given the starting index /// /// target string /// starting index for the substring. ///the substring given the starting index. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Substring(object targetString, object startIndex) { throw new NotImplementedException(); } ////// Returns the substring from the target string. /// /// target string. /// starting index for the substring. /// length of the substring. ///Returns the substring given the starting index and length. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Substring(object targetString, object startIndex, object length) { throw new NotImplementedException(); } ////// Checks whether the given string is a substring of the target string. /// /// substring to check for. /// target string. ///returns true if the target string contains the substring, otherwise returns false. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object SubstringOf(object substring, object targetString) { throw new NotImplementedException(); } ////// Returns a copy of the target string converted to lowercase. /// /// target string ///a new string instance with everything in lowercase. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Globalization", "CA1308", Justification = "Need to support ToLower function")] public static object ToLower(object targetString) { throw new NotImplementedException(); } ////// Returns a copy of the target string converted to uppercase. /// /// target string ///a new string instance with everything in uppercase. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object ToUpper(object targetString) { throw new NotImplementedException(); } ////// Removes all leading and trailing white-space characters from the target string. /// /// target string. ///returns the trimed string. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Trim(object targetString) { throw new NotImplementedException(); } #endregion #region Datetime functions ////// Returns the year value of the given datetime. /// /// datetime object. ///returns the year value of the given datetime. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Year(object dateTime) { throw new NotImplementedException(); } ////// Returns the month value of the given datetime. /// /// datetime object. ///returns the month value of the given datetime. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Month(object dateTime) { throw new NotImplementedException(); } ////// Returns the day value of the given datetime. /// /// datetime object. ///returns the day value of the given datetime. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Day(object dateTime) { throw new NotImplementedException(); } ////// Returns the hour value of the given datetime. /// /// datetime object. ///returns the hour value of the given datetime. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Hour(object dateTime) { throw new NotImplementedException(); } ////// Returns the minute value of the given datetime. /// /// datetime object. ///returns the minute value of the given datetime. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Minute(object dateTime) { throw new NotImplementedException(); } ////// Returns the second value of the given datetime. /// /// datetime object. ///returns the second value of the given datetime. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Second(object dateTime) { throw new NotImplementedException(); } #endregion #region Numeric functions ////// Returns the ceiling of the given value /// /// decimal or double object. ///returns the ceiling value for the given double or decimal value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Ceiling(object value) { throw new NotImplementedException(); } ////// returns the floor of the given value. /// /// decimal or double object. ///returns the floor value for the given double or decimal value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Floor(object value) { throw new NotImplementedException(); } ////// Rounds the given value. /// /// decimal or double object. ///returns the round value for the given double or decimal value. [Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA1801:ReviewUnusedParameters", Justification = "Parameters will be used in the actual impl")] public static object Round(object value) { throw new NotImplementedException(); } #endregion #endregion #region Factory methods for expression tree nodes. ///Creates an expression that adds two values with no overflow checking. /// Left value.Right value. ///The added value. internal static Expression AddExpression(Expression left, Expression right) { return Expression.Add( ExpressionAsObject(left), ExpressionAsObject(right), AddMethodInfo); } ///Creates a call expression that represents a conditional AND operation that evaluates the second operand only if it has to. /// Left value.Right value. ///The conditional expression; null if the expressions aren't of the right type. internal static Expression AndAlsoExpression(Expression left, Expression right) { return Expression.Call( OpenTypeMethods.AndAlsoMethodInfo, ExpressionAsObject(left), ExpressionAsObject(right)); } ///Creates an expression that divides two values. /// Left value.Right value. ///The divided value. internal static Expression DivideExpression(Expression left, Expression right) { return Expression.Divide( ExpressionAsObject(left), ExpressionAsObject(right), DivideMethodInfo); } ///Creates an expression that checks whether two values are equal. /// Left value.Right value. ///true if left equals right; false otherwise. internal static Expression EqualExpression(Expression left, Expression right) { return Expression.Equal( ExpressionAsObject(left), ExpressionAsObject(right), false, EqualMethodInfo); } ///Creates an expression that checks whether the left value is greater than the right value. /// Left value.Right value. ///true if left is greater than right; false otherwise. internal static Expression GreaterThanExpression(Expression left, Expression right) { return Expression.GreaterThan( ExpressionAsObject(left), ExpressionAsObject(right), false, GreaterThanMethodInfo); } ///Creates an expression that checks whether the left value is greater than or equal to the right value. /// Left value.Right value. ///true if left is greater than or equal to right; false otherwise. internal static Expression GreaterThanOrEqualExpression(Expression left, Expression right) { return Expression.GreaterThanOrEqual( ExpressionAsObject(left), ExpressionAsObject(right), false, GreaterThanOrEqualMethodInfo); } ///Creates an expression that checks whether the left value is less than the right value. /// Left value.Right value. ///true if left is less than right; false otherwise. internal static Expression LessThanExpression(Expression left, Expression right) { return Expression.LessThan( ExpressionAsObject(left), ExpressionAsObject(right), false, LessThanMethodInfo); } ///Creates an expression that checks whether the left value is less than or equal to the right value. /// Left value.Right value. ///true if left is less than or equal to right; false otherwise. internal static Expression LessThanOrEqualExpression(Expression left, Expression right) { return Expression.LessThanOrEqual( ExpressionAsObject(left), ExpressionAsObject(right), false, LessThanOrEqualMethodInfo); } ///Creates an expression that calculates the remainder of dividing the left value by the right value. /// Left value.Right value. ///The remainder value. internal static Expression ModuloExpression(Expression left, Expression right) { return Expression.Modulo( ExpressionAsObject(left), ExpressionAsObject(right), ModuloMethodInfo); } ///Creates an expression that multiplies two values with no overflow checking. /// Left value.Right value. ///The multiplication value. internal static Expression MultiplyExpression(Expression left, Expression right) { return Expression.Multiply( ExpressionAsObject(left), ExpressionAsObject(right), MultiplyMethodInfo); } ///Creates a call expression that represents a conditional OR operation that evaluates the second operand only if it has to. /// Left value.Right value. ///The conditional expression; null if the expressions aren't of the right type. internal static Expression OrElseExpression(Expression left, Expression right) { return Expression.Call( OpenTypeMethods.OrElseMethodInfo, ExpressionAsObject(left), ExpressionAsObject(right)); } ///Creates an expression that checks whether two values are not equal. /// Left value.Right value. ///true if left is does not equal right; false otherwise. internal static Expression NotEqualExpression(Expression left, Expression right) { return Expression.NotEqual( ExpressionAsObject(left), ExpressionAsObject(right), false, NotEqualMethodInfo); } ///Creates an expression that subtracts the right value from the left value. /// Left value.Right value. ///The subtraction value. internal static Expression SubtractExpression(Expression left, Expression right) { return Expression.Subtract( ExpressionAsObject(left), ExpressionAsObject(right), SubtractMethodInfo); } ///Creates an expression that negates (arithmetically) the specified value. /// Value expression. ///The negated value. internal static Expression NegateExpression(Expression expression) { return Expression.Negate( ExpressionAsObject(expression), NegateMethodInfo); } ///Creates an expression that negates (logically) the specified value. /// Value expression. ///The negated value. internal static Expression NotExpression(Expression expression) { return Expression.Not( ExpressionAsObject(expression), NotMethodInfo); } #endregion Factory methods for expression tree nodes. #region Helper methods ////// Returns the specified /// Expression to convert. ///with a /// type assignable to System.Object. /// /// The specified private static Expression ExpressionAsObject(Expression expression) { Debug.Assert(expression != null, "expression != null"); return expression.Type.IsValueType ? Expression.Convert(expression, typeof(object)) : expression; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.with a type assignable /// to System.Object. ///
Link Menu
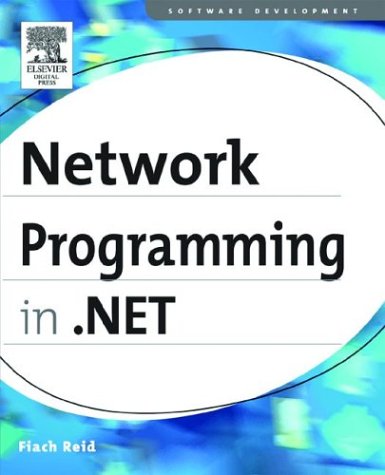
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OutOfProcStateClientManager.cs
- XPathExpr.cs
- ProfessionalColors.cs
- WindowsAuthenticationModule.cs
- QuotedPairReader.cs
- LoginName.cs
- Positioning.cs
- UnknownBitmapEncoder.cs
- BitmapCodecInfoInternal.cs
- DbConnectionPoolIdentity.cs
- IDictionary.cs
- LazyTextWriterCreator.cs
- XPathConvert.cs
- Label.cs
- NumericExpr.cs
- CodeSubDirectory.cs
- IteratorDescriptor.cs
- PolyLineSegment.cs
- XmlSchemaObjectCollection.cs
- InputLanguageProfileNotifySink.cs
- SourceSwitch.cs
- GridSplitterAutomationPeer.cs
- xmlfixedPageInfo.cs
- Codec.cs
- RowVisual.cs
- ApplicationDirectoryMembershipCondition.cs
- DocumentPage.cs
- TiffBitmapEncoder.cs
- X509WindowsSecurityToken.cs
- TypeSemantics.cs
- ACE.cs
- DetailsViewInsertEventArgs.cs
- CodeArrayCreateExpression.cs
- ProtocolViolationException.cs
- LogWriteRestartAreaAsyncResult.cs
- MobilePage.cs
- RadioButtonStandardAdapter.cs
- DataGridViewColumnStateChangedEventArgs.cs
- ALinqExpressionVisitor.cs
- _SpnDictionary.cs
- List.cs
- DiagnosticsConfigurationHandler.cs
- DataGridTableStyleMappingNameEditor.cs
- StandardOleMarshalObject.cs
- DecimalKeyFrameCollection.cs
- Pair.cs
- UndoManager.cs
- ValidationRule.cs
- EncodingTable.cs
- MappedMetaModel.cs
- CodeIdentifier.cs
- Menu.cs
- Html32TextWriter.cs
- Stylesheet.cs
- TraceListener.cs
- BooleanKeyFrameCollection.cs
- TreeView.cs
- ConfigurationLockCollection.cs
- MergeFilterQuery.cs
- TypeDescriptorContext.cs
- ToolStripLocationCancelEventArgs.cs
- Connector.cs
- XmlSchemaCollection.cs
- XmlSchemaValidationException.cs
- DataGridColumnStyleMappingNameEditor.cs
- MimeMapping.cs
- FormViewPageEventArgs.cs
- RoleManagerEventArgs.cs
- AutomationPropertyInfo.cs
- AssemblyAttributesGoHere.cs
- BindingCollectionElement.cs
- BindingElement.cs
- TextUtf8RawTextWriter.cs
- ToolStripItemClickedEventArgs.cs
- ControlBindingsCollection.cs
- BuildProvider.cs
- PackagingUtilities.cs
- HybridDictionary.cs
- TraceShell.cs
- ComAdminInterfaces.cs
- DesignerActionPanel.cs
- SoapReflectionImporter.cs
- ToolStripPanelDesigner.cs
- TypeBuilder.cs
- XhtmlBasicControlAdapter.cs
- Delay.cs
- BooleanFacetDescriptionElement.cs
- TransactionBridge.cs
- MILUtilities.cs
- CryptoConfig.cs
- ResourceExpression.cs
- AvTraceDetails.cs
- RootBrowserWindow.cs
- SafeSystemMetrics.cs
- UserControlCodeDomTreeGenerator.cs
- DataTableExtensions.cs
- NodeCounter.cs
- XmlMemberMapping.cs
- Vector3DIndependentAnimationStorage.cs
- CorePropertiesFilter.cs