Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / UIAutomation / UIAutomationClient / System / Windows / Automation / SelectionPattern.cs / 1 / SelectionPattern.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for Selection Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Windows.Automation.Provider; using System.ComponentModel; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Class representing containers that manage selection. /// #if (INTERNAL_COMPILE) internal class SelectionPattern: BasePattern #else public class SelectionPattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private SelectionPattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern) { _hPattern = hPattern; _cached = cached; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///Selection pattern public static readonly AutomationPattern Pattern = SelectionPatternIdentifiers.Pattern; ///Get the currently selected elements public static readonly AutomationProperty SelectionProperty = SelectionPatternIdentifiers.SelectionProperty; ///Indicates whether the control allows more than one element to be selected public static readonly AutomationProperty CanSelectMultipleProperty = SelectionPatternIdentifiers.CanSelectMultipleProperty; ///Indicates whether the control requires at least one element to be selected public static readonly AutomationProperty IsSelectionRequiredProperty = SelectionPatternIdentifiers.IsSelectionRequiredProperty; ////// Event ID: SelectionInvalidated - indicates that selection changed in a selection conainer. /// sourceElement refers to the selection container /// public static readonly AutomationEvent InvalidatedEvent = SelectionPatternIdentifiers.InvalidatedEvent; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods // No public methods #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// public SelectionPatternInformation Cached { get { Misc.ValidateCached(_cached); return new SelectionPatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// public SelectionPatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new SelectionPatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal static object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new SelectionPattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; private bool _cached; #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct SelectionPatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal SelectionPatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Get the currently selected elements /// ///An AutomationElement array containing the currently selected elements /// ////// This API does not work inside the secure execution environment. /// public AutomationElement[] GetSelection() { return (AutomationElement[])_el.GetPatternPropertyValue(SelectionProperty, _useCache); } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- #region Public Properties ////// /// Indicates whether the control allows more than one element to be selected /// ///Boolean indicating whether the control allows more than one element to be selected ///If this is false, then the control is a single-select ccntrol /// ////// This API does not work inside the secure execution environment. /// public bool CanSelectMultiple { get { return (bool)_el.GetPatternPropertyValue(CanSelectMultipleProperty, _useCache); } } ////// /// Indicates whether the control requires at least one element to be selected /// ///Boolean indicating whether the control requires at least one element to be selected ///If this is false, then the control allows all elements to be unselected /// ////// This API does not work inside the secure execution environment. /// public bool IsSelectionRequired { get { return (bool)_el.GetPatternPropertyValue(IsSelectionRequiredProperty, _useCache); } } #endregion Public Properties //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // ///// // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for Selection Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Windows.Automation.Provider; using System.ComponentModel; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Class representing containers that manage selection. /// #if (INTERNAL_COMPILE) internal class SelectionPattern: BasePattern #else public class SelectionPattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private SelectionPattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern) { _hPattern = hPattern; _cached = cached; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///Selection pattern public static readonly AutomationPattern Pattern = SelectionPatternIdentifiers.Pattern; ///Get the currently selected elements public static readonly AutomationProperty SelectionProperty = SelectionPatternIdentifiers.SelectionProperty; ///Indicates whether the control allows more than one element to be selected public static readonly AutomationProperty CanSelectMultipleProperty = SelectionPatternIdentifiers.CanSelectMultipleProperty; ///Indicates whether the control requires at least one element to be selected public static readonly AutomationProperty IsSelectionRequiredProperty = SelectionPatternIdentifiers.IsSelectionRequiredProperty; ////// Event ID: SelectionInvalidated - indicates that selection changed in a selection conainer. /// sourceElement refers to the selection container /// public static readonly AutomationEvent InvalidatedEvent = SelectionPatternIdentifiers.InvalidatedEvent; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods // No public methods #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// public SelectionPatternInformation Cached { get { Misc.ValidateCached(_cached); return new SelectionPatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// public SelectionPatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new SelectionPatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal static object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new SelectionPattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; private bool _cached; #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct SelectionPatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal SelectionPatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Get the currently selected elements /// ///An AutomationElement array containing the currently selected elements /// ////// This API does not work inside the secure execution environment. /// public AutomationElement[] GetSelection() { return (AutomationElement[])_el.GetPatternPropertyValue(SelectionProperty, _useCache); } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- #region Public Properties ////// /// Indicates whether the control allows more than one element to be selected /// ///Boolean indicating whether the control allows more than one element to be selected ///If this is false, then the control is a single-select ccntrol /// ////// This API does not work inside the secure execution environment. /// public bool CanSelectMultiple { get { return (bool)_el.GetPatternPropertyValue(CanSelectMultipleProperty, _useCache); } } ////// /// Indicates whether the control requires at least one element to be selected /// ///Boolean indicating whether the control requires at least one element to be selected ///If this is false, then the control allows all elements to be unselected /// ////// This API does not work inside the secure execution environment. /// public bool IsSelectionRequired { get { return (bool)_el.GetPatternPropertyValue(IsSelectionRequiredProperty, _useCache); } } #endregion Public Properties //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
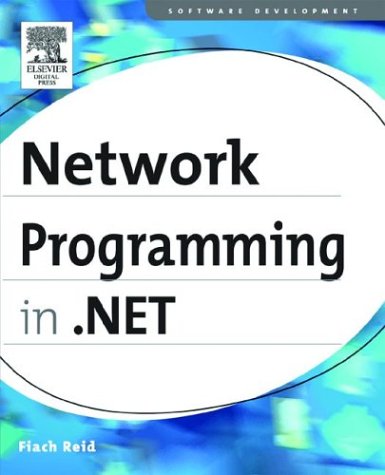
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebSysDefaultValueAttribute.cs
- InteropBitmapSource.cs
- Win32KeyboardDevice.cs
- ResourceAssociationTypeEnd.cs
- SiteMapNodeItemEventArgs.cs
- WebEventTraceProvider.cs
- RestHandlerFactory.cs
- ActivityValidationServices.cs
- ClientRuntimeConfig.cs
- SoapServerProtocol.cs
- PropertyItemInternal.cs
- EmptyReadOnlyDictionaryInternal.cs
- VisualTreeHelper.cs
- Empty.cs
- NetTcpSectionData.cs
- TextParagraphCache.cs
- RegularExpressionValidator.cs
- HandleExceptionArgs.cs
- Slider.cs
- Certificate.cs
- StateMachineSubscription.cs
- compensatingcollection.cs
- PropertySourceInfo.cs
- SizeChangedInfo.cs
- EmptyControlCollection.cs
- SecurityRuntime.cs
- IPAddressCollection.cs
- WsiProfilesElement.cs
- SqlClientWrapperSmiStreamChars.cs
- ServerValidateEventArgs.cs
- MeasureData.cs
- XamlSerializerUtil.cs
- ToolBarButtonClickEvent.cs
- UTF32Encoding.cs
- SuppressMessageAttribute.cs
- ConfigurationStrings.cs
- SafeViewOfFileHandle.cs
- Transform.cs
- SHA256Managed.cs
- WebColorConverter.cs
- ComAdminWrapper.cs
- FacetChecker.cs
- OracleCommandSet.cs
- WebBrowserBase.cs
- TextBoxAutoCompleteSourceConverter.cs
- QilGenerator.cs
- BrowserCapabilitiesCodeGenerator.cs
- AlphabeticalEnumConverter.cs
- TextEditorCopyPaste.cs
- WebPartMinimizeVerb.cs
- XmlSchemaSet.cs
- IdentityElement.cs
- Select.cs
- OleServicesContext.cs
- HandleExceptionArgs.cs
- KeyFrames.cs
- MarshalByValueComponent.cs
- XmlSchemaSimpleType.cs
- LinkedList.cs
- QueryableDataSourceEditData.cs
- HttpPostedFile.cs
- CodeTypeMemberCollection.cs
- FlatButtonAppearance.cs
- PopupRoot.cs
- RegisteredExpandoAttribute.cs
- JsonXmlDataContract.cs
- WaveHeader.cs
- HGlobalSafeHandle.cs
- BoolExpressionVisitors.cs
- MessageBodyDescription.cs
- SchemaInfo.cs
- IIS7UserPrincipal.cs
- DeflateEmulationStream.cs
- DiagnosticTraceRecords.cs
- ImageDrawing.cs
- SpellerInterop.cs
- ParameterElement.cs
- CodeGotoStatement.cs
- BordersPage.cs
- ApplicationCommands.cs
- MetadataItemCollectionFactory.cs
- WebColorConverter.cs
- RequestQueue.cs
- TemplatePropertyEntry.cs
- DataStreams.cs
- DataServiceQueryProvider.cs
- Type.cs
- ActivityDesigner.cs
- UserPersonalizationStateInfo.cs
- PriorityRange.cs
- Operator.cs
- PeerApplication.cs
- Errors.cs
- ColumnWidthChangedEvent.cs
- WebPartConnection.cs
- RegisteredExpandoAttribute.cs
- IPAddress.cs
- EventWaitHandle.cs
- ConnectionPointCookie.cs
- DataTablePropertyDescriptor.cs