Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / SMSvcHost / System / ServiceModel / Activation / NetTcpSectionData.cs / 1 / NetTcpSectionData.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Activation { using System.Collections.Generic; using System.Security.Principal; using System.Configuration; using System.Diagnostics; using System.ServiceModel.Diagnostics; using System.ServiceModel.Activation.Configuration; class NetTcpSectionData { int listenBacklog; int maxPendingConnections; int maxPendingAccepts; TimeSpan receiveTimeout; bool teredoEnabled; ListallowAccounts; public NetTcpSectionData() { NetTcpSection section = (NetTcpSection)ConfigurationManager.GetSection(ConfigurationStrings.NetTcpSectionPath); if (section == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException()); } this.listenBacklog = section.ListenBacklog; this.maxPendingConnections = section.MaxPendingConnections; this.maxPendingAccepts = section.MaxPendingAccepts; this.receiveTimeout = section.ReceiveTimeout; this.teredoEnabled = section.TeredoEnabled; this.allowAccounts = new List (); foreach (SecurityIdentifierElement element in section.AllowAccounts) { this.allowAccounts.Add(element.SecurityIdentifier); } } public int ListenBacklog { get { return this.listenBacklog; } } public int MaxPendingConnections { get { return this.maxPendingConnections; } } public int MaxPendingAccepts { get { return this.maxPendingAccepts; } } public TimeSpan ReceiveTimeout { get { return this.receiveTimeout; } } public bool TeredoEnabled { get { return this.teredoEnabled; } } public List AllowAccounts { get { return this.allowAccounts; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
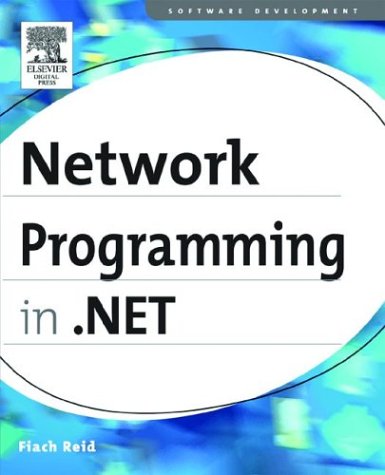
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CoTaskMemSafeHandle.cs
- Win32MouseDevice.cs
- DbMetaDataCollectionNames.cs
- XmlCountingReader.cs
- EnvelopedSignatureTransform.cs
- Quaternion.cs
- DisplayMemberTemplateSelector.cs
- XamlTypeMapperSchemaContext.cs
- CodeTypeReference.cs
- GridItemCollection.cs
- TableCellCollection.cs
- XmlAttributeProperties.cs
- SQLString.cs
- WhileDesigner.xaml.cs
- SignatureDescription.cs
- ObjectListField.cs
- StylusPointPropertyId.cs
- MatrixTransform.cs
- DeflateEmulationStream.cs
- CodeAccessSecurityEngine.cs
- UnmanagedBitmapWrapper.cs
- UnSafeCharBuffer.cs
- Transform3DCollection.cs
- ProjectionCamera.cs
- QueryServiceConfigHandle.cs
- SendMailErrorEventArgs.cs
- TrackingStringDictionary.cs
- SqlTriggerContext.cs
- SafeRegistryHandle.cs
- Canvas.cs
- WebServiceClientProxyGenerator.cs
- KeyValueSerializer.cs
- ExtractorMetadata.cs
- PropertyValueChangedEvent.cs
- KnownTypes.cs
- SingleAnimation.cs
- XmlILStorageConverter.cs
- DefaultBindingPropertyAttribute.cs
- NavigationPropertyAccessor.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- Int64.cs
- DbProviderManifest.cs
- CmsInterop.cs
- TextDecorationCollectionConverter.cs
- ToolStripOverflow.cs
- MarkedHighlightComponent.cs
- SessionStateContainer.cs
- AnimationClockResource.cs
- ConfigXmlComment.cs
- ObjectIDGenerator.cs
- XsdBuilder.cs
- ListViewSelectEventArgs.cs
- HttpListenerTimeoutManager.cs
- TraceHandler.cs
- AppDomainEvidenceFactory.cs
- EdmError.cs
- CqlBlock.cs
- FormattedText.cs
- CodeCommentStatement.cs
- PropertyPushdownHelper.cs
- JavaScriptSerializer.cs
- QilList.cs
- IconBitmapDecoder.cs
- ClientScriptItemCollection.cs
- IQueryable.cs
- JoinQueryOperator.cs
- VariantWrapper.cs
- tibetanshape.cs
- TextHidden.cs
- FilterableData.cs
- LoadGrammarCompletedEventArgs.cs
- TimeSpanValidatorAttribute.cs
- OnOperation.cs
- VirtualizedCellInfoCollection.cs
- MetadataArtifactLoaderResource.cs
- WebPart.cs
- ToolStripSeparatorRenderEventArgs.cs
- ResourcePool.cs
- TaskForm.cs
- HashMembershipCondition.cs
- cookiecontainer.cs
- Enum.cs
- HyperLinkColumn.cs
- OrderPreservingMergeHelper.cs
- XmlSchemaInfo.cs
- WebPartDeleteVerb.cs
- GridViewAutoFormat.cs
- ConcurrencyMode.cs
- RoleManagerSection.cs
- RawMouseInputReport.cs
- controlskin.cs
- Padding.cs
- DotExpr.cs
- ACE.cs
- DependentList.cs
- SQLMoneyStorage.cs
- ResXResourceSet.cs
- Win32.cs
- ConstructorNeedsTagAttribute.cs
- SimpleHandlerFactory.cs