Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Imaging / UnmanagedBitmapWrapper.cs / 1 / UnmanagedBitmapWrapper.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation. All Rights Reserved. // // File: UnmanagedBitmapWrapper.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { internal sealed class UnmanagedBitmapWrapper : System.Windows.Media.Imaging.BitmapSource { ////// Critical - calls critical code method BitmapSource.UpdateCachedSettings /// TreatAsSafe - all inputs are checked /// [SecurityCritical, SecurityTreatAsSafe] public UnmanagedBitmapWrapper(BitmapSourceSafeMILHandle bitmapSource) : base(true) { _bitmapInit.BeginInit(); bitmapSource.CalculateSize(); WicSourceHandle = bitmapSource; _bitmapInit.EndInit(); UpdateCachedSettings(); } #region Protected Methods ////// Critical - eventually access'es critical resources (_wicSource) /// TreatAsSafe - all inputs are checked /// [SecurityCritical, SecurityTreatAsSafe] internal UnmanagedBitmapWrapper(bool initialize) : base(true) { // Call BeginInit and EndInit if initialize is true. if (initialize) { _bitmapInit.BeginInit(); _bitmapInit.EndInit(); } } ////// Critical - calls critical code method BitmapSource.UpdateCachedSettings /// TreatAsSafe - all inputs are checked /// [SecurityCritical, SecurityTreatAsSafe] internal void UpdateBitmapSource(BitmapSource bitmapSource, DUCE.Channel channel) { WicSourceHandle = bitmapSource.WicSourceHandle; UpdateCachedSettings(); _needsUpdate = true; UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } ////// Implementation of protected override Freezable CreateInstanceCore() { return new UnmanagedBitmapWrapper(false); } private void CopyCommon(UnmanagedBitmapWrapper sourceBitmap) { _bitmapInit.BeginInit(); _bitmapInit.EndInit(); } ///Freezable.CreateInstanceCore . ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { UnmanagedBitmapWrapper sourceBitmap = (UnmanagedBitmapWrapper)sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { UnmanagedBitmapWrapper sourceBitmap = (UnmanagedBitmapWrapper)sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { UnmanagedBitmapWrapper sourceBitmap = (UnmanagedBitmapWrapper)sourceFreezable; base.GetAsFrozenCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { UnmanagedBitmapWrapper sourceBitmap = (UnmanagedBitmapWrapper)sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); CopyCommon(sourceBitmap); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation. All Rights Reserved. // // File: UnmanagedBitmapWrapper.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { internal sealed class UnmanagedBitmapWrapper : System.Windows.Media.Imaging.BitmapSource { ///Freezable.GetCurrentValueAsFrozenCore . ////// Critical - calls critical code method BitmapSource.UpdateCachedSettings /// TreatAsSafe - all inputs are checked /// [SecurityCritical, SecurityTreatAsSafe] public UnmanagedBitmapWrapper(BitmapSourceSafeMILHandle bitmapSource) : base(true) { _bitmapInit.BeginInit(); bitmapSource.CalculateSize(); WicSourceHandle = bitmapSource; _bitmapInit.EndInit(); UpdateCachedSettings(); } #region Protected Methods ////// Critical - eventually access'es critical resources (_wicSource) /// TreatAsSafe - all inputs are checked /// [SecurityCritical, SecurityTreatAsSafe] internal UnmanagedBitmapWrapper(bool initialize) : base(true) { // Call BeginInit and EndInit if initialize is true. if (initialize) { _bitmapInit.BeginInit(); _bitmapInit.EndInit(); } } ////// Critical - calls critical code method BitmapSource.UpdateCachedSettings /// TreatAsSafe - all inputs are checked /// [SecurityCritical, SecurityTreatAsSafe] internal void UpdateBitmapSource(BitmapSource bitmapSource, DUCE.Channel channel) { WicSourceHandle = bitmapSource.WicSourceHandle; UpdateCachedSettings(); _needsUpdate = true; UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } ////// Implementation of protected override Freezable CreateInstanceCore() { return new UnmanagedBitmapWrapper(false); } private void CopyCommon(UnmanagedBitmapWrapper sourceBitmap) { _bitmapInit.BeginInit(); _bitmapInit.EndInit(); } ///Freezable.CreateInstanceCore . ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { UnmanagedBitmapWrapper sourceBitmap = (UnmanagedBitmapWrapper)sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { UnmanagedBitmapWrapper sourceBitmap = (UnmanagedBitmapWrapper)sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { UnmanagedBitmapWrapper sourceBitmap = (UnmanagedBitmapWrapper)sourceFreezable; base.GetAsFrozenCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { UnmanagedBitmapWrapper sourceBitmap = (UnmanagedBitmapWrapper)sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); CopyCommon(sourceBitmap); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Freezable.GetCurrentValueAsFrozenCore . ///
Link Menu
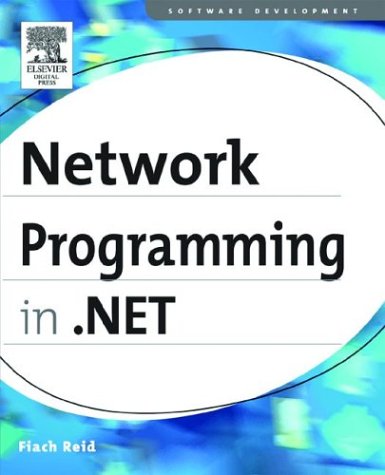
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReadWriteObjectLock.cs
- CodeStatementCollection.cs
- ParentControlDesigner.cs
- webproxy.cs
- ContentElementAutomationPeer.cs
- HttpPostedFileBase.cs
- ConstraintCollection.cs
- UpnEndpointIdentity.cs
- BindingElementCollection.cs
- SqlBuilder.cs
- TraversalRequest.cs
- QuinticEase.cs
- TdsValueSetter.cs
- _SafeNetHandles.cs
- PropVariant.cs
- AuthenticationSection.cs
- InternalControlCollection.cs
- ListViewDeletedEventArgs.cs
- ExpressionBindingCollection.cs
- CompletedAsyncResult.cs
- CorePropertiesFilter.cs
- WebServiceReceiveDesigner.cs
- SizeAnimation.cs
- ImageListImageEditor.cs
- GroupItem.cs
- DisplayInformation.cs
- PathGradientBrush.cs
- DataGridViewIntLinkedList.cs
- DataView.cs
- Publisher.cs
- NavigationPropertyEmitter.cs
- Profiler.cs
- DragStartedEventArgs.cs
- followingsibling.cs
- SafePointer.cs
- TextLineResult.cs
- SqlClientWrapperSmiStreamChars.cs
- DispatcherExceptionEventArgs.cs
- ArcSegment.cs
- StreamInfo.cs
- RequiredFieldValidator.cs
- ZipArchive.cs
- CodeIterationStatement.cs
- Common.cs
- WebBrowserDesigner.cs
- RawKeyboardInputReport.cs
- InkCanvasSelection.cs
- GeneralTransform3DGroup.cs
- HyperLink.cs
- AutoFocusStyle.xaml.cs
- ParameterToken.cs
- MenuItemStyleCollection.cs
- MouseButtonEventArgs.cs
- StoreAnnotationsMap.cs
- BindingFormattingDialog.cs
- InvalidPropValue.cs
- CollectionEditorDialog.cs
- ComponentResourceKeyConverter.cs
- SetState.cs
- ObjectHelper.cs
- TagPrefixCollection.cs
- XmlILAnnotation.cs
- Int16Converter.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- ContextMenuStripGroup.cs
- CompatibleComparer.cs
- GrammarBuilderBase.cs
- ObjectAssociationEndMapping.cs
- SolidColorBrush.cs
- HtmlInputSubmit.cs
- ThemeConfigurationDialog.cs
- ContainerParagraph.cs
- UnicodeEncoding.cs
- DynamicValueConverter.cs
- XamlTemplateSerializer.cs
- MessageDroppedTraceRecord.cs
- BinHexDecoder.cs
- BooleanConverter.cs
- WebPartDescriptionCollection.cs
- SQLDateTime.cs
- SudsCommon.cs
- HttpEncoder.cs
- CorrelationToken.cs
- ScrollChrome.cs
- BoolExpressionVisitors.cs
- SubqueryTrackingVisitor.cs
- XmlWrappingReader.cs
- Variant.cs
- RawStylusInput.cs
- TemplateControlBuildProvider.cs
- ArrayConverter.cs
- SimpleType.cs
- ImageListImageEditor.cs
- AstTree.cs
- CellCreator.cs
- XmlSchemaAnyAttribute.cs
- Equal.cs
- SrgsRuleRef.cs
- XPathNodeList.cs
- FixedPageAutomationPeer.cs