Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / GrammarBuilding / GrammarBuilderBase.cs / 1 / GrammarBuilderBase.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System.Speech.Recognition; using System.Speech.Internal.SrgsParser; namespace System.Speech.Internal.GrammarBuilding { ////// /// internal abstract class GrammarBuilderBase { //******************************************************************* // // Internal Methods // //******************************************************************* #region Internal Methods ////// /// ///internal abstract GrammarBuilderBase Clone (); /// /// /// /// /// /// /// ///internal abstract IElement CreateElement(IElementFactory elementFactory, IElement parent, IRule rule, IdentifierCollection ruleIds); /// /// /// ///internal virtual int CalcCount (BuilderElements parent) { Marked = false; Parent = parent; return Count; } #endregion //******************************************************************** // // Internal Properties // //******************************************************************* #region Internal Properties /// /// Used by the GrammarBuilder optimizer to count the number of children and decendant for /// an element /// internal virtual int Count { get { return _count; } set { _count = value; } } ////// Marker to know if an element has already been visited. /// internal virtual bool Marked { get { return _marker; } set { _marker = value; } } ////// Marker to know if an element has already been visited. /// internal virtual BuilderElements Parent { get { return _parent; } set { _parent = value; } } ////// /// internal abstract string DebugSummary { get; } #endregion //******************************************************************** // // Private Fields // //******************************************************************** #region Private Fields private int _count = 1; private bool _marker; private BuilderElements _parent; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System.Speech.Recognition; using System.Speech.Internal.SrgsParser; namespace System.Speech.Internal.GrammarBuilding { ////// /// internal abstract class GrammarBuilderBase { //******************************************************************* // // Internal Methods // //******************************************************************* #region Internal Methods ////// /// ///internal abstract GrammarBuilderBase Clone (); /// /// /// /// /// /// /// ///internal abstract IElement CreateElement(IElementFactory elementFactory, IElement parent, IRule rule, IdentifierCollection ruleIds); /// /// /// ///internal virtual int CalcCount (BuilderElements parent) { Marked = false; Parent = parent; return Count; } #endregion //******************************************************************** // // Internal Properties // //******************************************************************* #region Internal Properties /// /// Used by the GrammarBuilder optimizer to count the number of children and decendant for /// an element /// internal virtual int Count { get { return _count; } set { _count = value; } } ////// Marker to know if an element has already been visited. /// internal virtual bool Marked { get { return _marker; } set { _marker = value; } } ////// Marker to know if an element has already been visited. /// internal virtual BuilderElements Parent { get { return _parent; } set { _parent = value; } } ////// /// internal abstract string DebugSummary { get; } #endregion //******************************************************************** // // Private Fields // //******************************************************************** #region Private Fields private int _count = 1; private bool _marker; private BuilderElements _parent; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
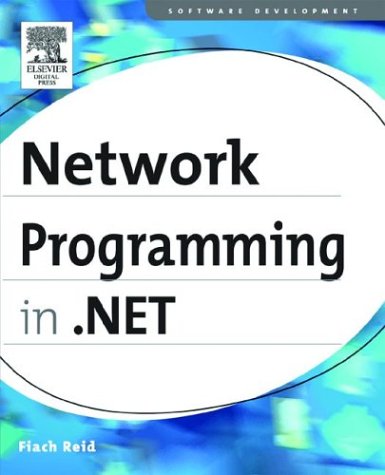
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeComment.cs
- InputLangChangeEvent.cs
- WebResourceAttribute.cs
- ArgIterator.cs
- SqlBulkCopy.cs
- GridViewPageEventArgs.cs
- AsyncStreamReader.cs
- RecordManager.cs
- HostExecutionContextManager.cs
- HScrollBar.cs
- InputScope.cs
- EntityProxyFactory.cs
- SemanticTag.cs
- AndMessageFilterTable.cs
- ValueType.cs
- CLSCompliantAttribute.cs
- Parser.cs
- AutoGeneratedField.cs
- Literal.cs
- CatalogPart.cs
- ObjectQueryExecutionPlan.cs
- DropTarget.cs
- DocumentScope.cs
- OuterGlowBitmapEffect.cs
- RightsManagementPermission.cs
- UpdatableWrapper.cs
- PolyBezierSegment.cs
- XmlCharType.cs
- CompositeTypefaceMetrics.cs
- CryptoProvider.cs
- OleDbWrapper.cs
- TableLayoutRowStyleCollection.cs
- CredentialSelector.cs
- ServiceModelTimeSpanValidator.cs
- HorizontalAlignConverter.cs
- RoleGroupCollectionEditor.cs
- ContainerAction.cs
- ModelFunctionTypeElement.cs
- DataTableClearEvent.cs
- TextWriterTraceListener.cs
- ValidatorUtils.cs
- ServiceNameCollection.cs
- SortExpressionBuilder.cs
- QueryConverter.cs
- URI.cs
- InvokePatternIdentifiers.cs
- Floater.cs
- AlternateViewCollection.cs
- PackageController.cs
- BuildProvidersCompiler.cs
- ToolStripPanelRow.cs
- ScriptResourceHandler.cs
- TraceProvider.cs
- TakeOrSkipQueryOperator.cs
- FunctionImportElement.cs
- MappingException.cs
- ContextBase.cs
- CellTreeNodeVisitors.cs
- SmtpNegotiateAuthenticationModule.cs
- Splitter.cs
- SystemUnicastIPAddressInformation.cs
- CompilationSection.cs
- IdentitySection.cs
- TaskFormBase.cs
- LogStream.cs
- KeyedCollection.cs
- ModelFunctionTypeElement.cs
- XsltArgumentList.cs
- BaseCodePageEncoding.cs
- IssuanceLicense.cs
- FullTrustAssembly.cs
- MailAddress.cs
- Zone.cs
- CqlErrorHelper.cs
- BaseValidator.cs
- FrameSecurityDescriptor.cs
- _CacheStreams.cs
- Parameter.cs
- Rect3D.cs
- Fonts.cs
- SchemaElement.cs
- MetafileHeaderWmf.cs
- ObjRef.cs
- ProgressPage.cs
- SqlDataSourceCache.cs
- EncryptedData.cs
- CodeParameterDeclarationExpression.cs
- FormsAuthentication.cs
- ListViewCommandEventArgs.cs
- MediaPlayer.cs
- SemaphoreFullException.cs
- Peer.cs
- DocumentApplicationJournalEntryEventArgs.cs
- DependencyPropertyChangedEventArgs.cs
- WizardDesigner.cs
- BindingMemberInfo.cs
- DynamicResourceExtensionConverter.cs
- ProjectionPlanCompiler.cs
- CmsUtils.cs
- SelectedCellsCollection.cs