Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / Configuration / CompilationSection.cs / 5 / CompilationSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Web.Compilation; using System.Reflection; using System.Web.Hosting; using System.Web.UI; using System.CodeDom.Compiler; using System.Web.Util; using System.ComponentModel; using System.Security.Permissions; /**/ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class CompilationSection : ConfigurationSection { private const string tempDirectoryAttributeName = "tempDirectory"; private const string assemblyPostProcessorTypeAttributeName = "assemblyPostProcessorType"; private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propTempDirectory = new ConfigurationProperty(tempDirectoryAttributeName, typeof(string), String.Empty, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDebug = new ConfigurationProperty("debug", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propStrict = new ConfigurationProperty("strict", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propExplicit = new ConfigurationProperty("explicit", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propBatch = new ConfigurationProperty("batch", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propBatchTimeout = new ConfigurationProperty("batchTimeout", typeof(TimeSpan), TimeSpan.FromMinutes(15.0), StdValidatorsAndConverters.TimeSpanSecondsOrInfiniteConverter, StdValidatorsAndConverters.PositiveTimeSpanValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMaxBatchSize = new ConfigurationProperty("maxBatchSize", typeof(int), 1000, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMaxBatchGeneratedFileSize = new ConfigurationProperty("maxBatchGeneratedFileSize", typeof(int), 1000, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propNumRecompilesBeforeAppRestart = new ConfigurationProperty("numRecompilesBeforeAppRestart", typeof(int), 15, ConfigurationPropertyOptions.None); #if !FEATURE_PAL // FEATURE_PAL does not support VisualBasic private static readonly ConfigurationProperty _propDefaultLanguage = new ConfigurationProperty("defaultLanguage", typeof(string), "vb", ConfigurationPropertyOptions.None); #else // !FEATURE_PAL private static readonly ConfigurationProperty _propDefaultLanguage = new ConfigurationProperty("defaultLanguage", typeof(string),"c#",ConfigurationPropertyFlags.None); #endif // !FEATURE_PAL private static readonly ConfigurationProperty _propCompilers = new ConfigurationProperty("compilers", typeof(CompilerCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private static readonly ConfigurationProperty _propAssemblies = new ConfigurationProperty("assemblies", typeof(AssemblyCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private static readonly ConfigurationProperty _propBuildProviders = new ConfigurationProperty("buildProviders", typeof(BuildProviderCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private static readonly ConfigurationProperty _propExpressionBuilders = new ConfigurationProperty("expressionBuilders", typeof(ExpressionBuilderCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private static readonly ConfigurationProperty _propUrlLinePragmas = new ConfigurationProperty("urlLinePragmas", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propCodeSubDirs = new ConfigurationProperty("codeSubDirectories", typeof(CodeSubDirectoriesCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private static readonly ConfigurationProperty _propAssemblyPreprocessorType = new ConfigurationProperty(assemblyPostProcessorTypeAttributeName, typeof(string), String.Empty, ConfigurationPropertyOptions.None); const char fieldSeparator = ';'; private bool _referenceSet; // _compilerLanguages : Hashtable // NOTE: This hashtable may contain either Compiler objects or CompilerType objects. // It'll contain the later if the data has been read from config, but the particular // language compiler type hasn't been resolved yet. Otherwise, it'll contain CompilerType objects. private Hashtable _compilerLanguages; // _compilerExtensions : Hashtable // NOTE: This hashtable may contain either Compiler objects or CompilerType objects. // It'll contain the later if the data has been read from config, but the particular // language compiler type hasn't been resolved yet. Otherwise, it'll contain CompilerType objects. private Hashtable _compilerExtensions; private long _recompilationHash = -1; private bool _isRuntimeObject = false; private Type _assemblyPostProcessorType; static CompilationSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propTempDirectory); _properties.Add(_propDebug); _properties.Add(_propStrict); _properties.Add(_propExplicit); _properties.Add(_propBatch); _properties.Add(_propBatchTimeout); _properties.Add(_propMaxBatchSize); _properties.Add(_propMaxBatchGeneratedFileSize); _properties.Add(_propNumRecompilesBeforeAppRestart); _properties.Add(_propDefaultLanguage); _properties.Add(_propCompilers); _properties.Add(_propAssemblies); _properties.Add(_propBuildProviders); _properties.Add(_propExpressionBuilders); _properties.Add(_propUrlLinePragmas); _properties.Add(_propCodeSubDirs); _properties.Add(_propAssemblyPreprocessorType); } public CompilationSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } protected override object GetRuntimeObject() { _isRuntimeObject = true; return base.GetRuntimeObject(); } [ConfigurationProperty(tempDirectoryAttributeName, DefaultValue = "")] public string TempDirectory { get { return (string)base[_propTempDirectory]; } set { base[_propTempDirectory] = value; } } // Used for error handling when there is a problem withe the temp dir internal void GetTempDirectoryErrorInfo(out string tempDirAttribName, out string configFileName, out int configLineNumber) { tempDirAttribName = tempDirectoryAttributeName; configFileName = ElementInformation.Properties[tempDirectoryAttributeName].Source; configLineNumber = ElementInformation.Properties[tempDirectoryAttributeName].LineNumber; } [ConfigurationProperty("debug", DefaultValue = false)] public bool Debug { get { return (bool)base[_propDebug]; } set { base[_propDebug] = value; } } [ConfigurationProperty("strict", DefaultValue = false)] public bool Strict { get { return (bool)base[_propStrict]; } set { base[_propStrict] = value; } } [ConfigurationProperty("explicit", DefaultValue = true)] public bool Explicit { get { return (bool)base[_propExplicit]; } set { base[_propExplicit] = value; } } [ConfigurationProperty("batch", DefaultValue = true)] public bool Batch { get { return (bool)base[_propBatch]; } set { base[_propBatch] = value; } } [ConfigurationProperty("urlLinePragmas", DefaultValue = false)] public bool UrlLinePragmas { get { return (bool)base[_propUrlLinePragmas]; } set { base[_propUrlLinePragmas] = value; } } [ConfigurationProperty("batchTimeout", DefaultValue = "00:15:00")] [TimeSpanValidator(MinValueString="00:00:00", MaxValueString=TimeSpanValidatorAttribute.TimeSpanMaxValue)] [TypeConverter(typeof(TimeSpanSecondsOrInfiniteConverter))] public TimeSpan BatchTimeout { get { return (TimeSpan)base[_propBatchTimeout]; } set { base[_propBatchTimeout] = value; } } [ConfigurationProperty("maxBatchSize", DefaultValue = 1000)] public int MaxBatchSize { get { return (int)base[_propMaxBatchSize]; } set { base[_propMaxBatchSize] = value; } } [ConfigurationProperty("maxBatchGeneratedFileSize", DefaultValue = 1000)] public int MaxBatchGeneratedFileSize { get { return (int)base[_propMaxBatchGeneratedFileSize]; } set { base[_propMaxBatchGeneratedFileSize] = value; } } [ConfigurationProperty("numRecompilesBeforeAppRestart", DefaultValue = 15)] public int NumRecompilesBeforeAppRestart { get { return (int)base[_propNumRecompilesBeforeAppRestart]; } set { base[_propNumRecompilesBeforeAppRestart] = value; } } [ConfigurationProperty("defaultLanguage", DefaultValue = "vb")] public string DefaultLanguage { get { return (string)base[_propDefaultLanguage]; } set { base[_propDefaultLanguage] = value; } } [ConfigurationProperty("compilers")] public CompilerCollection Compilers { get { return (CompilerCollection)base[_propCompilers]; } } [ConfigurationProperty("assemblies")] public AssemblyCollection Assemblies { get { if (_isRuntimeObject) { EnsureReferenceSet(); } return GetAssembliesCollection(); } } private AssemblyCollection GetAssembliesCollection() { return (AssemblyCollection)base[_propAssemblies]; } [ConfigurationProperty("buildProviders")] public BuildProviderCollection BuildProviders { get { return (BuildProviderCollection)base[_propBuildProviders]; } } [ConfigurationProperty("expressionBuilders")] public ExpressionBuilderCollection ExpressionBuilders { get { return (ExpressionBuilderCollection)base[_propExpressionBuilders]; } } [ConfigurationProperty(assemblyPostProcessorTypeAttributeName, DefaultValue = "")] public string AssemblyPostProcessorType { get { return (string)base[_propAssemblyPreprocessorType]; } set { base[_propAssemblyPreprocessorType] = value; } } internal Type AssemblyPostProcessorTypeInternal { get { if (_assemblyPostProcessorType == null && !String.IsNullOrEmpty(AssemblyPostProcessorType)) { lock (this) { if (_assemblyPostProcessorType == null) { // Only allow this in full trust if (!HttpRuntime.HasUnmanagedPermission()) { throw new ConfigurationErrorsException(SR.GetString( SR.Insufficient_trust_for_attribute, assemblyPostProcessorTypeAttributeName), ElementInformation.Properties[assemblyPostProcessorTypeAttributeName].Source, ElementInformation.Properties[assemblyPostProcessorTypeAttributeName].LineNumber); } Type assemblyPostProcessorType = ConfigUtil.GetType(AssemblyPostProcessorType, assemblyPostProcessorTypeAttributeName, this); ConfigUtil.CheckBaseType(typeof(System.Web.Compilation.IAssemblyPostProcessor), assemblyPostProcessorType, "assemblyPostProcessorType", this); _assemblyPostProcessorType = assemblyPostProcessorType; } } } return _assemblyPostProcessorType; } } [ConfigurationProperty("codeSubDirectories")] public CodeSubDirectoriesCollection CodeSubDirectories { get { return (CodeSubDirectoriesCollection)base[_propCodeSubDirs]; } } private void EnsureCompilerCacheInit() { if (_compilerLanguages == null) { lock (this) { if (_compilerLanguages == null) { Hashtable compilerLanguages = new Hashtable(StringComparer.OrdinalIgnoreCase); _compilerExtensions = new Hashtable(StringComparer.OrdinalIgnoreCase); foreach (Compiler compiler in Compilers) { // Parse the semicolon separated lists string[] languageList = compiler.Language.Split(fieldSeparator); string[] extensionList = compiler.Extension.Split(fieldSeparator); foreach (string language in languageList) { compilerLanguages[language] = compiler; } foreach (string extension in extensionList) { _compilerExtensions[extension] = compiler; } } // Only assign it at the end to make sure everything was successful _compilerLanguages = compilerLanguages; } } } } /* * Return a CompilerType that a extension maps to. */ internal CompilerType GetCompilerInfoFromExtension(string extension, bool throwOnFail) { EnsureCompilerCacheInit(); // First, try the cache (i.e. old section) CompilerType compilerType; object obj = _compilerExtensions[extension]; Compiler compiler = obj as Compiler; if (compiler != null) { compilerType = compiler.CompilerTypeInternal; _compilerExtensions[extension] = compilerType; } else { compilerType = obj as CompilerType; } if (compilerType == null) { // If not, try the section if (CodeDomProvider.IsDefinedExtension(extension)) { string language = CodeDomProvider.GetLanguageFromExtension(extension); CompilerInfo ci = CodeDomProvider.GetCompilerInfo(language); compilerType = new CompilerType( ci.CodeDomProviderType, ci.CreateDefaultCompilerParameters()); // Cache it _compilerExtensions[extension] = compilerType; } } if (compilerType == null) { if (!throwOnFail) return null; // Unsupported extension: throw an exception throw new HttpException(SR.GetString(SR.Invalid_lang_extension, extension)); } // Clone it so the original is not modified compilerType = compilerType.Clone(); // Set the value of the debug flag in the copy compilerType.CompilerParameters.IncludeDebugInformation = Debug; return compilerType; } /* * Return a CompilerType that a language maps to. */ internal CompilerType GetCompilerInfoFromLanguage(string language) { EnsureCompilerCacheInit(); // First, try the cache (i.e. old section) CompilerType compilerType; object obj = _compilerLanguages[language]; Compiler compiler = obj as Compiler; if (compiler != null) { compilerType = compiler.CompilerTypeInternal; _compilerLanguages[language] = compilerType; } else { compilerType = obj as CompilerType; } if (compilerType == null) { // Try the section if (CodeDomProvider.IsDefinedLanguage(language)) { CompilerInfo ci = CodeDomProvider.GetCompilerInfo(language); compilerType = new CompilerType(ci.CodeDomProviderType, ci.CreateDefaultCompilerParameters()); // Cache it _compilerLanguages[language] = compilerType; } } if (compilerType == null) { // Unsupported language: throw an exception throw new HttpException(SR.GetString(SR.Invalid_lang, language)); } // Only allow the use of compilerOptions when we have UnmanagedCode access (ASURT 73678) CompilationUtil.CheckCompilerOptionsAllowed(compilerType.CompilerParameters.CompilerOptions, true /*config*/, null, 0); // Clone it so the original is not modified compilerType = compilerType.Clone(); // Set the value of the debug flag in the copy compilerType.CompilerParameters.IncludeDebugInformation = Debug; return compilerType; } // This will only set the section pointer private void EnsureReferenceSet() { if (!_referenceSet) { System.Web.Util.Debug.Assert(HttpRuntime.CodegenDirInternal != null); foreach (AssemblyInfo ai in GetAssembliesCollection()) { ai.SetCompilationReference(this); } _referenceSet = true; } } internal Assembly[] LoadAssembly(AssemblyInfo ai) { Assembly[] assemblies = null; if (ai.Assembly == "*") { assemblies = LoadAllAssembliesFromAppDomainBinDirectory(); } else { Assembly a; a = LoadAssemblyHelper(ai.Assembly, false); if (a != null) { assemblies = new Assembly[1]; assemblies[0] = a; } } return assemblies; } internal Assembly LoadAssembly(string assemblyName, bool throwOnFail) { // The trust should always be set before we load any assembly (VSWhidbey 317295) System.Web.Util.Debug.Assert(HttpRuntime.TrustLevel != null); try { // First, try to just load the assembly return Assembly.Load(assemblyName); } catch { AssemblyName asmName = new AssemblyName(assemblyName); // Check if it's simply named Byte[] publicKeyToken = asmName.GetPublicKeyToken(); if ((publicKeyToken == null || publicKeyToken.Length == 0) && asmName.Version == null) { EnsureReferenceSet(); // It is simply named. Go through all the assemblies from // the section, and if we find one that matches // the simple name, return it (ASURT 100546) foreach (AssemblyInfo ai in GetAssembliesCollection()) { Assembly[] a = ai.AssemblyInternal; if (a != null) { for (int i = 0; i < a.Length; i++) { if (StringUtil.EqualsIgnoreCase(asmName.Name, a[i].GetName().Name)) { return a[i]; } } } } } if (throwOnFail) { throw; } } return null; } private Assembly LoadAssemblyHelper(string assemblyName, bool starDirective) { // The trust should always be set before we load any assembly (VSWhidbey 317295) System.Web.Util.Debug.Assert(HttpRuntime.TrustLevel != null); Assembly retAssembly = null; // Load the assembly and add it to the dictionary. try { retAssembly = System.Reflection.Assembly.Load(assemblyName); } catch (Exception e) { // Check if this assembly came from the '*' directive bool ignoreException = false; if (starDirective) { int hresult = System.Runtime.InteropServices.Marshal.GetHRForException(e); // This is expected to fail for unmanaged DLLs that happen // to be in the bin dir. Ignore them. // Also, if the DLL is not an assembly, ignore the exception (ASURT 93073, VSWhidbey 319486) // Test for COR_E_ASSEMBLYEXPECTED=0x80131018=-2146234344 if (hresult == -2146234344) { ignoreException = true; } } if (!ignoreException) { string Message = e.Message; if (String.IsNullOrEmpty(Message)) { // try and make a better message than empty string if (e is FileLoadException) { Message = SR.GetString(SR.Config_base_file_load_exception_no_message, "assembly"); } else if (e is BadImageFormatException) { Message = SR.GetString(SR.Config_base_bad_image_exception_no_message, assemblyName); } else { Message = SR.GetString(SR.Config_base_report_exception_type, e.GetType().ToString()); // at least this is better than no message } } // default to section if the assembly is not in the collection // which may happen it the assembly is being loaded from the bindir // and not named in configuration. String source = ElementInformation.Properties["assemblies"].Source; int lineNumber = ElementInformation.Properties["assemblies"].LineNumber; // If processing the * directive, look up the line information for it if (starDirective) assemblyName = "*"; if (Assemblies[assemblyName] != null) { source = Assemblies[assemblyName].ElementInformation.Source; lineNumber = Assemblies[assemblyName].ElementInformation.LineNumber; } throw new ConfigurationErrorsException(Message, e, source, lineNumber); } } System.Web.Util.Debug.Trace("LoadAssembly", "Successfully loaded assembly '" + assemblyName + "'"); return retAssembly; } internal Assembly[] LoadAllAssembliesFromAppDomainBinDirectory() { // Get the path to the bin directory string binPath = HttpRuntime.BinDirectoryInternal; FileInfo[] binDlls; Assembly assembly = null; Assembly[] assemblies = null; ArrayList list; if (!FileUtil.DirectoryExists(binPath)) { // This is expected to fail if there is no 'bin' dir System.Web.Util.Debug.Trace("Template", "Failed to access bin dir \"" + binPath + "\""); } else { DirectoryInfo binPathDirectory = new DirectoryInfo(binPath); // Get a list of all the DLL's in the bin directory binDlls = binPathDirectory.GetFiles("*.dll"); if (binDlls.Length > 0) { list = new ArrayList(binDlls.Length); for (int i = 0; i < binDlls.Length; i++) { string assemblyName = Util.GetAssemblyNameFromFileName(binDlls[i].Name); // Don't autoload generated assemblies in bin (VSWhidbey 467936) if (assemblyName.StartsWith(BuildManager.WebAssemblyNamePrefix, StringComparison.Ordinal)) continue; if (!GetAssembliesCollection().IsRemoved(assemblyName)) { assembly = LoadAssemblyHelper(assemblyName, true); } if (assembly != null) { list.Add(assembly); } } assemblies = (System.Reflection.Assembly[])list.ToArray(typeof(System.Reflection.Assembly)); } } if (assemblies == null) { // If there were no assemblies loaded, return a zero-length array assemblies = new Assembly[0]; } return assemblies; } internal long RecompilationHash { get { if (_recompilationHash == -1) { lock (this) { if (_recompilationHash == -1) { _recompilationHash = CompilationUtil.GetRecompilationHash(this); } } } return _recompilationHash; } } protected override void PostDeserialize() { // check to see if the _propCodeSubDirs was defined below the app level WebContext context = EvaluationContext.HostingContext as WebContext; if (context != null) { if (context.ApplicationLevel == WebApplicationLevel.BelowApplication) { if (CodeSubDirectories.ElementInformation.IsPresent) { throw new ConfigurationErrorsException( SR.GetString(SR.Config_element_below_app_illegal, _propCodeSubDirs.Name), CodeSubDirectories.ElementInformation.Source, CodeSubDirectories.ElementInformation.LineNumber); } if (BuildProviders.ElementInformation.IsPresent) { throw new ConfigurationErrorsException( SR.GetString(SR.Config_element_below_app_illegal, _propBuildProviders.Name), BuildProviders.ElementInformation.Source, BuildProviders.ElementInformation.LineNumber); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Web.Compilation; using System.Reflection; using System.Web.Hosting; using System.Web.UI; using System.CodeDom.Compiler; using System.Web.Util; using System.ComponentModel; using System.Security.Permissions; /**/ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class CompilationSection : ConfigurationSection { private const string tempDirectoryAttributeName = "tempDirectory"; private const string assemblyPostProcessorTypeAttributeName = "assemblyPostProcessorType"; private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propTempDirectory = new ConfigurationProperty(tempDirectoryAttributeName, typeof(string), String.Empty, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDebug = new ConfigurationProperty("debug", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propStrict = new ConfigurationProperty("strict", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propExplicit = new ConfigurationProperty("explicit", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propBatch = new ConfigurationProperty("batch", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propBatchTimeout = new ConfigurationProperty("batchTimeout", typeof(TimeSpan), TimeSpan.FromMinutes(15.0), StdValidatorsAndConverters.TimeSpanSecondsOrInfiniteConverter, StdValidatorsAndConverters.PositiveTimeSpanValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMaxBatchSize = new ConfigurationProperty("maxBatchSize", typeof(int), 1000, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMaxBatchGeneratedFileSize = new ConfigurationProperty("maxBatchGeneratedFileSize", typeof(int), 1000, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propNumRecompilesBeforeAppRestart = new ConfigurationProperty("numRecompilesBeforeAppRestart", typeof(int), 15, ConfigurationPropertyOptions.None); #if !FEATURE_PAL // FEATURE_PAL does not support VisualBasic private static readonly ConfigurationProperty _propDefaultLanguage = new ConfigurationProperty("defaultLanguage", typeof(string), "vb", ConfigurationPropertyOptions.None); #else // !FEATURE_PAL private static readonly ConfigurationProperty _propDefaultLanguage = new ConfigurationProperty("defaultLanguage", typeof(string),"c#",ConfigurationPropertyFlags.None); #endif // !FEATURE_PAL private static readonly ConfigurationProperty _propCompilers = new ConfigurationProperty("compilers", typeof(CompilerCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private static readonly ConfigurationProperty _propAssemblies = new ConfigurationProperty("assemblies", typeof(AssemblyCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private static readonly ConfigurationProperty _propBuildProviders = new ConfigurationProperty("buildProviders", typeof(BuildProviderCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private static readonly ConfigurationProperty _propExpressionBuilders = new ConfigurationProperty("expressionBuilders", typeof(ExpressionBuilderCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private static readonly ConfigurationProperty _propUrlLinePragmas = new ConfigurationProperty("urlLinePragmas", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propCodeSubDirs = new ConfigurationProperty("codeSubDirectories", typeof(CodeSubDirectoriesCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private static readonly ConfigurationProperty _propAssemblyPreprocessorType = new ConfigurationProperty(assemblyPostProcessorTypeAttributeName, typeof(string), String.Empty, ConfigurationPropertyOptions.None); const char fieldSeparator = ';'; private bool _referenceSet; // _compilerLanguages : Hashtable // NOTE: This hashtable may contain either Compiler objects or CompilerType objects. // It'll contain the later if the data has been read from config, but the particular // language compiler type hasn't been resolved yet. Otherwise, it'll contain CompilerType objects. private Hashtable _compilerLanguages; // _compilerExtensions : Hashtable // NOTE: This hashtable may contain either Compiler objects or CompilerType objects. // It'll contain the later if the data has been read from config, but the particular // language compiler type hasn't been resolved yet. Otherwise, it'll contain CompilerType objects. private Hashtable _compilerExtensions; private long _recompilationHash = -1; private bool _isRuntimeObject = false; private Type _assemblyPostProcessorType; static CompilationSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propTempDirectory); _properties.Add(_propDebug); _properties.Add(_propStrict); _properties.Add(_propExplicit); _properties.Add(_propBatch); _properties.Add(_propBatchTimeout); _properties.Add(_propMaxBatchSize); _properties.Add(_propMaxBatchGeneratedFileSize); _properties.Add(_propNumRecompilesBeforeAppRestart); _properties.Add(_propDefaultLanguage); _properties.Add(_propCompilers); _properties.Add(_propAssemblies); _properties.Add(_propBuildProviders); _properties.Add(_propExpressionBuilders); _properties.Add(_propUrlLinePragmas); _properties.Add(_propCodeSubDirs); _properties.Add(_propAssemblyPreprocessorType); } public CompilationSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } protected override object GetRuntimeObject() { _isRuntimeObject = true; return base.GetRuntimeObject(); } [ConfigurationProperty(tempDirectoryAttributeName, DefaultValue = "")] public string TempDirectory { get { return (string)base[_propTempDirectory]; } set { base[_propTempDirectory] = value; } } // Used for error handling when there is a problem withe the temp dir internal void GetTempDirectoryErrorInfo(out string tempDirAttribName, out string configFileName, out int configLineNumber) { tempDirAttribName = tempDirectoryAttributeName; configFileName = ElementInformation.Properties[tempDirectoryAttributeName].Source; configLineNumber = ElementInformation.Properties[tempDirectoryAttributeName].LineNumber; } [ConfigurationProperty("debug", DefaultValue = false)] public bool Debug { get { return (bool)base[_propDebug]; } set { base[_propDebug] = value; } } [ConfigurationProperty("strict", DefaultValue = false)] public bool Strict { get { return (bool)base[_propStrict]; } set { base[_propStrict] = value; } } [ConfigurationProperty("explicit", DefaultValue = true)] public bool Explicit { get { return (bool)base[_propExplicit]; } set { base[_propExplicit] = value; } } [ConfigurationProperty("batch", DefaultValue = true)] public bool Batch { get { return (bool)base[_propBatch]; } set { base[_propBatch] = value; } } [ConfigurationProperty("urlLinePragmas", DefaultValue = false)] public bool UrlLinePragmas { get { return (bool)base[_propUrlLinePragmas]; } set { base[_propUrlLinePragmas] = value; } } [ConfigurationProperty("batchTimeout", DefaultValue = "00:15:00")] [TimeSpanValidator(MinValueString="00:00:00", MaxValueString=TimeSpanValidatorAttribute.TimeSpanMaxValue)] [TypeConverter(typeof(TimeSpanSecondsOrInfiniteConverter))] public TimeSpan BatchTimeout { get { return (TimeSpan)base[_propBatchTimeout]; } set { base[_propBatchTimeout] = value; } } [ConfigurationProperty("maxBatchSize", DefaultValue = 1000)] public int MaxBatchSize { get { return (int)base[_propMaxBatchSize]; } set { base[_propMaxBatchSize] = value; } } [ConfigurationProperty("maxBatchGeneratedFileSize", DefaultValue = 1000)] public int MaxBatchGeneratedFileSize { get { return (int)base[_propMaxBatchGeneratedFileSize]; } set { base[_propMaxBatchGeneratedFileSize] = value; } } [ConfigurationProperty("numRecompilesBeforeAppRestart", DefaultValue = 15)] public int NumRecompilesBeforeAppRestart { get { return (int)base[_propNumRecompilesBeforeAppRestart]; } set { base[_propNumRecompilesBeforeAppRestart] = value; } } [ConfigurationProperty("defaultLanguage", DefaultValue = "vb")] public string DefaultLanguage { get { return (string)base[_propDefaultLanguage]; } set { base[_propDefaultLanguage] = value; } } [ConfigurationProperty("compilers")] public CompilerCollection Compilers { get { return (CompilerCollection)base[_propCompilers]; } } [ConfigurationProperty("assemblies")] public AssemblyCollection Assemblies { get { if (_isRuntimeObject) { EnsureReferenceSet(); } return GetAssembliesCollection(); } } private AssemblyCollection GetAssembliesCollection() { return (AssemblyCollection)base[_propAssemblies]; } [ConfigurationProperty("buildProviders")] public BuildProviderCollection BuildProviders { get { return (BuildProviderCollection)base[_propBuildProviders]; } } [ConfigurationProperty("expressionBuilders")] public ExpressionBuilderCollection ExpressionBuilders { get { return (ExpressionBuilderCollection)base[_propExpressionBuilders]; } } [ConfigurationProperty(assemblyPostProcessorTypeAttributeName, DefaultValue = "")] public string AssemblyPostProcessorType { get { return (string)base[_propAssemblyPreprocessorType]; } set { base[_propAssemblyPreprocessorType] = value; } } internal Type AssemblyPostProcessorTypeInternal { get { if (_assemblyPostProcessorType == null && !String.IsNullOrEmpty(AssemblyPostProcessorType)) { lock (this) { if (_assemblyPostProcessorType == null) { // Only allow this in full trust if (!HttpRuntime.HasUnmanagedPermission()) { throw new ConfigurationErrorsException(SR.GetString( SR.Insufficient_trust_for_attribute, assemblyPostProcessorTypeAttributeName), ElementInformation.Properties[assemblyPostProcessorTypeAttributeName].Source, ElementInformation.Properties[assemblyPostProcessorTypeAttributeName].LineNumber); } Type assemblyPostProcessorType = ConfigUtil.GetType(AssemblyPostProcessorType, assemblyPostProcessorTypeAttributeName, this); ConfigUtil.CheckBaseType(typeof(System.Web.Compilation.IAssemblyPostProcessor), assemblyPostProcessorType, "assemblyPostProcessorType", this); _assemblyPostProcessorType = assemblyPostProcessorType; } } } return _assemblyPostProcessorType; } } [ConfigurationProperty("codeSubDirectories")] public CodeSubDirectoriesCollection CodeSubDirectories { get { return (CodeSubDirectoriesCollection)base[_propCodeSubDirs]; } } private void EnsureCompilerCacheInit() { if (_compilerLanguages == null) { lock (this) { if (_compilerLanguages == null) { Hashtable compilerLanguages = new Hashtable(StringComparer.OrdinalIgnoreCase); _compilerExtensions = new Hashtable(StringComparer.OrdinalIgnoreCase); foreach (Compiler compiler in Compilers) { // Parse the semicolon separated lists string[] languageList = compiler.Language.Split(fieldSeparator); string[] extensionList = compiler.Extension.Split(fieldSeparator); foreach (string language in languageList) { compilerLanguages[language] = compiler; } foreach (string extension in extensionList) { _compilerExtensions[extension] = compiler; } } // Only assign it at the end to make sure everything was successful _compilerLanguages = compilerLanguages; } } } } /* * Return a CompilerType that a extension maps to. */ internal CompilerType GetCompilerInfoFromExtension(string extension, bool throwOnFail) { EnsureCompilerCacheInit(); // First, try the cache (i.e. old section) CompilerType compilerType; object obj = _compilerExtensions[extension]; Compiler compiler = obj as Compiler; if (compiler != null) { compilerType = compiler.CompilerTypeInternal; _compilerExtensions[extension] = compilerType; } else { compilerType = obj as CompilerType; } if (compilerType == null) { // If not, try the section if (CodeDomProvider.IsDefinedExtension(extension)) { string language = CodeDomProvider.GetLanguageFromExtension(extension); CompilerInfo ci = CodeDomProvider.GetCompilerInfo(language); compilerType = new CompilerType( ci.CodeDomProviderType, ci.CreateDefaultCompilerParameters()); // Cache it _compilerExtensions[extension] = compilerType; } } if (compilerType == null) { if (!throwOnFail) return null; // Unsupported extension: throw an exception throw new HttpException(SR.GetString(SR.Invalid_lang_extension, extension)); } // Clone it so the original is not modified compilerType = compilerType.Clone(); // Set the value of the debug flag in the copy compilerType.CompilerParameters.IncludeDebugInformation = Debug; return compilerType; } /* * Return a CompilerType that a language maps to. */ internal CompilerType GetCompilerInfoFromLanguage(string language) { EnsureCompilerCacheInit(); // First, try the cache (i.e. old section) CompilerType compilerType; object obj = _compilerLanguages[language]; Compiler compiler = obj as Compiler; if (compiler != null) { compilerType = compiler.CompilerTypeInternal; _compilerLanguages[language] = compilerType; } else { compilerType = obj as CompilerType; } if (compilerType == null) { // Try the section if (CodeDomProvider.IsDefinedLanguage(language)) { CompilerInfo ci = CodeDomProvider.GetCompilerInfo(language); compilerType = new CompilerType(ci.CodeDomProviderType, ci.CreateDefaultCompilerParameters()); // Cache it _compilerLanguages[language] = compilerType; } } if (compilerType == null) { // Unsupported language: throw an exception throw new HttpException(SR.GetString(SR.Invalid_lang, language)); } // Only allow the use of compilerOptions when we have UnmanagedCode access (ASURT 73678) CompilationUtil.CheckCompilerOptionsAllowed(compilerType.CompilerParameters.CompilerOptions, true /*config*/, null, 0); // Clone it so the original is not modified compilerType = compilerType.Clone(); // Set the value of the debug flag in the copy compilerType.CompilerParameters.IncludeDebugInformation = Debug; return compilerType; } // This will only set the section pointer private void EnsureReferenceSet() { if (!_referenceSet) { System.Web.Util.Debug.Assert(HttpRuntime.CodegenDirInternal != null); foreach (AssemblyInfo ai in GetAssembliesCollection()) { ai.SetCompilationReference(this); } _referenceSet = true; } } internal Assembly[] LoadAssembly(AssemblyInfo ai) { Assembly[] assemblies = null; if (ai.Assembly == "*") { assemblies = LoadAllAssembliesFromAppDomainBinDirectory(); } else { Assembly a; a = LoadAssemblyHelper(ai.Assembly, false); if (a != null) { assemblies = new Assembly[1]; assemblies[0] = a; } } return assemblies; } internal Assembly LoadAssembly(string assemblyName, bool throwOnFail) { // The trust should always be set before we load any assembly (VSWhidbey 317295) System.Web.Util.Debug.Assert(HttpRuntime.TrustLevel != null); try { // First, try to just load the assembly return Assembly.Load(assemblyName); } catch { AssemblyName asmName = new AssemblyName(assemblyName); // Check if it's simply named Byte[] publicKeyToken = asmName.GetPublicKeyToken(); if ((publicKeyToken == null || publicKeyToken.Length == 0) && asmName.Version == null) { EnsureReferenceSet(); // It is simply named. Go through all the assemblies from // the section, and if we find one that matches // the simple name, return it (ASURT 100546) foreach (AssemblyInfo ai in GetAssembliesCollection()) { Assembly[] a = ai.AssemblyInternal; if (a != null) { for (int i = 0; i < a.Length; i++) { if (StringUtil.EqualsIgnoreCase(asmName.Name, a[i].GetName().Name)) { return a[i]; } } } } } if (throwOnFail) { throw; } } return null; } private Assembly LoadAssemblyHelper(string assemblyName, bool starDirective) { // The trust should always be set before we load any assembly (VSWhidbey 317295) System.Web.Util.Debug.Assert(HttpRuntime.TrustLevel != null); Assembly retAssembly = null; // Load the assembly and add it to the dictionary. try { retAssembly = System.Reflection.Assembly.Load(assemblyName); } catch (Exception e) { // Check if this assembly came from the '*' directive bool ignoreException = false; if (starDirective) { int hresult = System.Runtime.InteropServices.Marshal.GetHRForException(e); // This is expected to fail for unmanaged DLLs that happen // to be in the bin dir. Ignore them. // Also, if the DLL is not an assembly, ignore the exception (ASURT 93073, VSWhidbey 319486) // Test for COR_E_ASSEMBLYEXPECTED=0x80131018=-2146234344 if (hresult == -2146234344) { ignoreException = true; } } if (!ignoreException) { string Message = e.Message; if (String.IsNullOrEmpty(Message)) { // try and make a better message than empty string if (e is FileLoadException) { Message = SR.GetString(SR.Config_base_file_load_exception_no_message, "assembly"); } else if (e is BadImageFormatException) { Message = SR.GetString(SR.Config_base_bad_image_exception_no_message, assemblyName); } else { Message = SR.GetString(SR.Config_base_report_exception_type, e.GetType().ToString()); // at least this is better than no message } } // default to section if the assembly is not in the collection // which may happen it the assembly is being loaded from the bindir // and not named in configuration. String source = ElementInformation.Properties["assemblies"].Source; int lineNumber = ElementInformation.Properties["assemblies"].LineNumber; // If processing the * directive, look up the line information for it if (starDirective) assemblyName = "*"; if (Assemblies[assemblyName] != null) { source = Assemblies[assemblyName].ElementInformation.Source; lineNumber = Assemblies[assemblyName].ElementInformation.LineNumber; } throw new ConfigurationErrorsException(Message, e, source, lineNumber); } } System.Web.Util.Debug.Trace("LoadAssembly", "Successfully loaded assembly '" + assemblyName + "'"); return retAssembly; } internal Assembly[] LoadAllAssembliesFromAppDomainBinDirectory() { // Get the path to the bin directory string binPath = HttpRuntime.BinDirectoryInternal; FileInfo[] binDlls; Assembly assembly = null; Assembly[] assemblies = null; ArrayList list; if (!FileUtil.DirectoryExists(binPath)) { // This is expected to fail if there is no 'bin' dir System.Web.Util.Debug.Trace("Template", "Failed to access bin dir \"" + binPath + "\""); } else { DirectoryInfo binPathDirectory = new DirectoryInfo(binPath); // Get a list of all the DLL's in the bin directory binDlls = binPathDirectory.GetFiles("*.dll"); if (binDlls.Length > 0) { list = new ArrayList(binDlls.Length); for (int i = 0; i < binDlls.Length; i++) { string assemblyName = Util.GetAssemblyNameFromFileName(binDlls[i].Name); // Don't autoload generated assemblies in bin (VSWhidbey 467936) if (assemblyName.StartsWith(BuildManager.WebAssemblyNamePrefix, StringComparison.Ordinal)) continue; if (!GetAssembliesCollection().IsRemoved(assemblyName)) { assembly = LoadAssemblyHelper(assemblyName, true); } if (assembly != null) { list.Add(assembly); } } assemblies = (System.Reflection.Assembly[])list.ToArray(typeof(System.Reflection.Assembly)); } } if (assemblies == null) { // If there were no assemblies loaded, return a zero-length array assemblies = new Assembly[0]; } return assemblies; } internal long RecompilationHash { get { if (_recompilationHash == -1) { lock (this) { if (_recompilationHash == -1) { _recompilationHash = CompilationUtil.GetRecompilationHash(this); } } } return _recompilationHash; } } protected override void PostDeserialize() { // check to see if the _propCodeSubDirs was defined below the app level WebContext context = EvaluationContext.HostingContext as WebContext; if (context != null) { if (context.ApplicationLevel == WebApplicationLevel.BelowApplication) { if (CodeSubDirectories.ElementInformation.IsPresent) { throw new ConfigurationErrorsException( SR.GetString(SR.Config_element_below_app_illegal, _propCodeSubDirs.Name), CodeSubDirectories.ElementInformation.Source, CodeSubDirectories.ElementInformation.LineNumber); } if (BuildProviders.ElementInformation.IsPresent) { throw new ConfigurationErrorsException( SR.GetString(SR.Config_element_below_app_illegal, _propBuildProviders.Name), BuildProviders.ElementInformation.Source, BuildProviders.ElementInformation.LineNumber); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
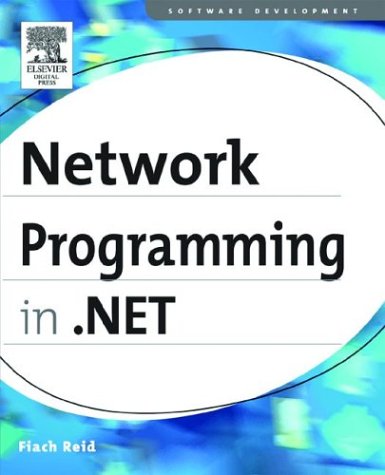
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CaretElement.cs
- NavigationHelper.cs
- ColumnTypeConverter.cs
- HTTPNotFoundHandler.cs
- GeneralTransformCollection.cs
- SmtpAuthenticationManager.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- DLinqDataModelProvider.cs
- PartialCachingAttribute.cs
- DataBinding.cs
- BasicBrowserDialog.designer.cs
- DataRowView.cs
- SecurityVersion.cs
- SizeIndependentAnimationStorage.cs
- BinHexDecoder.cs
- ProtocolsConfiguration.cs
- FrameworkReadOnlyPropertyMetadata.cs
- ContextBase.cs
- ClientConfigPaths.cs
- RootAction.cs
- sortedlist.cs
- HostedHttpRequestAsyncResult.cs
- RelatedCurrencyManager.cs
- DivideByZeroException.cs
- _ChunkParse.cs
- MdiWindowListItemConverter.cs
- XXXOnTypeBuilderInstantiation.cs
- SchemaTableOptionalColumn.cs
- PrePrepareMethodAttribute.cs
- DuplicateWaitObjectException.cs
- ContentPropertyAttribute.cs
- WaitForChangedResult.cs
- securitycriticaldataClass.cs
- DataGridViewSortCompareEventArgs.cs
- XamlClipboardData.cs
- DateTimeParse.cs
- ParsedAttributeCollection.cs
- ContainerParaClient.cs
- GeneralTransformGroup.cs
- ResourceContainer.cs
- TouchPoint.cs
- AxisAngleRotation3D.cs
- InputScopeConverter.cs
- ReservationNotFoundException.cs
- RIPEMD160.cs
- XmlSchemaAnnotated.cs
- FlowNode.cs
- Pen.cs
- Int64AnimationBase.cs
- MulticastNotSupportedException.cs
- CqlErrorHelper.cs
- validation.cs
- UpdateRecord.cs
- FixedNode.cs
- SamlAuthorityBinding.cs
- ProvidersHelper.cs
- ContextStaticAttribute.cs
- DbConnectionFactory.cs
- GroupBox.cs
- PinnedBufferMemoryStream.cs
- BitmapFrame.cs
- ResourceAssociationSetEnd.cs
- Byte.cs
- TripleDES.cs
- DataFormat.cs
- ObjectViewQueryResultData.cs
- DbConnectionFactory.cs
- DataGridViewTextBoxCell.cs
- HttpProfileBase.cs
- DocumentViewerBase.cs
- GridViewDeleteEventArgs.cs
- WebContext.cs
- ProgressBar.cs
- DictionaryTraceRecord.cs
- ButtonPopupAdapter.cs
- SchemaTableOptionalColumn.cs
- SqlIdentifier.cs
- XmlReturnWriter.cs
- LayoutTableCell.cs
- GatewayDefinition.cs
- Configuration.cs
- WebPartConnectionsCancelVerb.cs
- BaseUriHelper.cs
- TypeDescriptionProvider.cs
- XappLauncher.cs
- initElementDictionary.cs
- OpenFileDialog.cs
- PasswordValidationException.cs
- HandlerBase.cs
- CodeAssignStatement.cs
- DemultiplexingDispatchMessageFormatter.cs
- XmlElementAttributes.cs
- PropertyChangeTracker.cs
- _CommandStream.cs
- Stack.cs
- EntityDataSourceWizardForm.cs
- UserControlAutomationPeer.cs
- BoundField.cs
- Serializer.cs
- WebBrowsableAttribute.cs