Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / DataSourceHelper.cs / 1305376 / DataSourceHelper.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; internal sealed class DataSourceHelper { private DataSourceHelper() { } internal static IEnumerable GetResolvedDataSource(object dataSource, string dataMember) { if (dataSource == null) return null; IListSource listSource = dataSource as IListSource; if (listSource != null) { IList memberList = listSource.GetList(); if (listSource.ContainsListCollection == false) { // the returned list is itself the list we need to bind to // NOTE: I am ignoring the DataMember parameter... ideally we might have // thrown an exception, but this would mess up design-time usage // where the user may change the data source from a DataSet to a // DataTable. return (IEnumerable)memberList; } if ((memberList != null) && (memberList is ITypedList)) { ITypedList typedMemberList = (ITypedList)memberList; PropertyDescriptorCollection propDescs = typedMemberList.GetItemProperties(new PropertyDescriptor[0]); if ((propDescs != null) && (propDescs.Count != 0)) { PropertyDescriptor listProperty = null; if (String.IsNullOrEmpty(dataMember)) { listProperty = propDescs[0]; } else { listProperty = propDescs.Find(dataMember, true); } if (listProperty != null) { object listRow = memberList[0]; object list = listProperty.GetValue(listRow); if ((list != null) && (list is IEnumerable)) { return (IEnumerable)list; } } throw new HttpException(SR.GetString(SR.ListSource_Missing_DataMember, dataMember)); } else { throw new HttpException(SR.GetString(SR.ListSource_Without_DataMembers)); } } } if (dataSource is IEnumerable) { return (IEnumerable)dataSource; } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
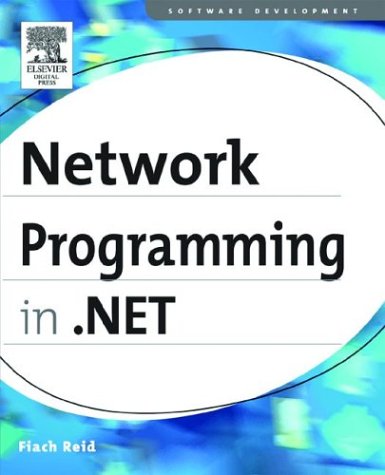
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IdentityManager.cs
- Processor.cs
- XmlNodeReader.cs
- ToolStripScrollButton.cs
- Stacktrace.cs
- ProcessStartInfo.cs
- PersonalizableAttribute.cs
- AuthorizationRuleCollection.cs
- JsonEnumDataContract.cs
- DbMetaDataColumnNames.cs
- EntityViewGenerationAttribute.cs
- altserialization.cs
- SmiMetaDataProperty.cs
- DataControlLinkButton.cs
- SafeFileHandle.cs
- ImageListStreamer.cs
- RangeBase.cs
- WorkflowElementDialog.cs
- TemplateManager.cs
- RuntimeHandles.cs
- MobileResource.cs
- RowType.cs
- TransformPattern.cs
- XPathAncestorIterator.cs
- CriticalHandle.cs
- ActivityTypeResolver.xaml.cs
- StandardOleMarshalObject.cs
- OdbcConnectionOpen.cs
- ParserContext.cs
- RegexInterpreter.cs
- CapiNative.cs
- XamlReader.cs
- DataGridCommandEventArgs.cs
- ObjectToken.cs
- DependencyPropertyHelper.cs
- InternalTypeHelper.cs
- WebAdminConfigurationHelper.cs
- XmlTextWriter.cs
- DecimalConverter.cs
- XmlKeywords.cs
- ThreadStartException.cs
- ConfigurationElement.cs
- AnnotationAuthorChangedEventArgs.cs
- TimeSpanStorage.cs
- GPPOINTF.cs
- AgileSafeNativeMemoryHandle.cs
- WebControlsSection.cs
- ParseHttpDate.cs
- StreamInfo.cs
- MasterPageParser.cs
- Baml2006ReaderSettings.cs
- BamlBinaryWriter.cs
- Highlights.cs
- BamlWriter.cs
- SetState.cs
- DocumentGridContextMenu.cs
- SmiRequestExecutor.cs
- ControlPaint.cs
- WebUtil.cs
- Wildcard.cs
- DaylightTime.cs
- WmiEventSink.cs
- MembershipUser.cs
- GridViewCommandEventArgs.cs
- ImpersonationContext.cs
- TemplateEditingFrame.cs
- FaultDescription.cs
- PropagatorResult.cs
- WorkflowMarkupSerializer.cs
- DiffuseMaterial.cs
- SoapSchemaExporter.cs
- Propagator.Evaluator.cs
- PassportIdentity.cs
- PageBuildProvider.cs
- RepeaterItemEventArgs.cs
- RbTree.cs
- XmlMapping.cs
- controlskin.cs
- WebPartHelpVerb.cs
- SamlAuthorizationDecisionClaimResource.cs
- CancellationHandler.cs
- Int32AnimationBase.cs
- AssemblyHash.cs
- XmlObjectSerializerContext.cs
- ProjectionPathSegment.cs
- EntityDataSourceEntityTypeFilterItem.cs
- SamlAttributeStatement.cs
- ClientRuntimeConfig.cs
- DataControlField.cs
- GridItemPattern.cs
- ContractListAdapter.cs
- ThaiBuddhistCalendar.cs
- SignatureConfirmations.cs
- OleDbStruct.cs
- CommonObjectSecurity.cs
- TrackingServices.cs
- SmtpNtlmAuthenticationModule.cs
- CheckBoxPopupAdapter.cs
- XmlToDatasetMap.cs
- TextServicesManager.cs