Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / Internal / ExpressionList.cs / 2 / ExpressionList.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Data.Common; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; namespace System.Data.Common.CommandTrees.Internal { internal class ExpressionList : IList{ private string _name; private DbCommandTree _commandTree; private List _list; private ExpressionList(string name, DbCommandTree owner) { _name = name; _commandTree = owner; } internal List ExpressionLinks { get { return _list; } } internal ExpressionList(string name, DbCommandTree owner, int capacity) : this(name, owner) { _list = new List (capacity); for(int idx = 0; idx < capacity; idx++) { _list.Add(new ExpressionLink(CommandTreeUtils.FormatIndex(_name, idx), owner)); } } internal ExpressionList(string name, DbCommandTree owner, IList args) : this(name, owner) { Debug.Assert(args != null, "Argument list is null?"); int capacity = args.Count; List list = new List (capacity); for (int idx = 0; idx < capacity; idx++) { list.Add(new ExpressionLink(CommandTreeUtils.FormatIndex(_name, idx), _commandTree, args[idx])); } _list = list; } internal ExpressionList(string name, DbCommandTree owner, TypeUsage expectedElementType, IList args) : this(name, owner) { Debug.Assert(args != null, "Argument list is null?"); int capacity = args.Count; List list = new List (capacity); for (int idx = 0; idx < capacity; idx++) { list.Add(new ExpressionLink(CommandTreeUtils.FormatIndex(_name, idx), _commandTree, expectedElementType, args[idx])); } _list = list; } internal ExpressionList(string name, DbCommandTree owner, PrimitiveTypeKind expectedElementPrimitiveType, IList args) : this(name, owner) { Debug.Assert(args != null, "Argument list is null?"); int capacity = args.Count; List list = new List (capacity); for (int idx = 0; idx < capacity; idx++) { list.Add(new ExpressionLink(CommandTreeUtils.FormatIndex(_name, idx), _commandTree, expectedElementPrimitiveType, args[idx])); } _list = list; } // // Called by DbFunctionAggregate and DbFunctionExpression // internal ExpressionList(string name, DbCommandTree owner, ReadOnlyMetadataCollection paramInfo, IList args) : this(name, owner) { int capacity = paramInfo.Count; List list = new List (capacity); for (int idx = 0; idx < capacity; idx++) { string elemName = CommandTreeUtils.FormatIndex(_name, idx); FunctionParameter paramMeta = paramInfo[idx]; _commandTree.TypeHelper.CheckParameter(paramMeta, elemName); list.Add(new ExpressionLink(elemName, _commandTree, paramMeta.TypeUsage)); } _list = list; this.SetElements(args); } internal ExpressionList(string name, DbCommandTree owner, IBaseList members, IList args) : this(name, owner) { _list = new List (); int idx = 0; foreach (EdmMember memberMeta in members) { string elemName = CommandTreeUtils.FormatIndex(_name, idx++); _commandTree.TypeHelper.CheckMember(memberMeta, elemName); _list.Add(new ExpressionLink(elemName, _commandTree, Helper.GetModelTypeUsage(memberMeta))); } this.SetElements(args); } /// /// Sets the value of each element in the list without instructing /// the owning Command Tree to consider itself 'modified'. /// Should only be used to set initial values for the list elements, /// and not called again after that initialization has taken place /// (otherwise the Command Tree's VersionNumber property will not be /// updated even though changes have been made to the list). /// /// The DbExpression values to use as list elements. Must match the expected count of this list. internal void SetElements(IListargs) { int addedCount = 0; for(int idx = 0; idx < args.Count; idx++) { if (idx > _list.Count - 1) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_ExpressionList_IncorrectElementCount, _name); } _list[idx].InitializeValue(args[idx]); addedCount++; } if (addedCount != _list.Count) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_ExpressionList_IncorrectElementCount, _name); } } internal void SetExpectedElementType(TypeUsage type) { foreach (ExpressionLink link in _list) { link.SetExpectedType(type); } } internal TypeUsage GetCommonElementType() { TypeUsage commonType = null; foreach (ExpressionLink exprLink in _list) { if (null == commonType) { commonType = exprLink.Expression.ResultType; } else { commonType = TypeHelpers.GetCommonTypeUsage(commonType, exprLink.Expression.ResultType); } if (null == commonType) { break; } } return commonType; } internal void SetAt(int index, DbExpression value) { _list[index].Expression = value; } #region IList Members int IList .IndexOf(DbExpression item) { for (int idx = 0; idx < _list.Count; idx++) { if (_list[idx].Expression == item) { return idx; } } return -1; } void IList .Insert(int index, DbExpression item) { throw EntityUtil.NotSupported(); } void IList .RemoveAt(int index) { throw EntityUtil.NotSupported(); } DbExpression IList .this[int index] { get { return _list[index].Expression; } #if MUTABLE_CQT set { _list[index].Expression = value; } #else set { throw EntityUtil.NotSupported(); } #endif } #endregion #region ICollection Members void ICollection .Add(DbExpression item) { throw EntityUtil.NotSupported(); } void ICollection .Clear() { throw EntityUtil.NotSupported(); } bool ICollection .Contains(DbExpression item) { return (((IList )this).IndexOf(item) > -1); } void ICollection .CopyTo(DbExpression[] array, int arrayIndex) { List exprs = new List (); foreach (ExpressionLink exprLink in _list) { exprs.Add(exprLink.Expression); } exprs.CopyTo(array, arrayIndex); } int ICollection .Count { get { return _list.Count; } } bool ICollection .IsReadOnly { #if MUTABLE_CQT get { return false; } #else get { return true; } #endif } bool ICollection .Remove(DbExpression item) { throw EntityUtil.NotSupported(); } #endregion #region IEnumerable Members IEnumerator IEnumerable .GetEnumerator() { return new ExpressionListEnumerator(_list.GetEnumerator()); } #endregion #region IEnumerable Members System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion #region Nested Enumerator class private class ExpressionListEnumerator : IEnumerator { private IEnumerator m_Enumerator; internal ExpressionListEnumerator(IEnumerator innerEnum) { this.m_Enumerator = innerEnum; } #region IEnumerator Members DbExpression IEnumerator .Current { get { return this.m_Enumerator.Current.Expression; } } #endregion #region IDisposable Members void IDisposable.Dispose() { this.m_Enumerator.Dispose(); } #endregion #region IEnumerator Members object System.Collections.IEnumerator.Current { get { return this.m_Enumerator.Current.Expression; } } bool System.Collections.IEnumerator.MoveNext() { return this.m_Enumerator.MoveNext(); } void System.Collections.IEnumerator.Reset() { this.m_Enumerator.Reset(); } #endregion } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Data.Common; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; namespace System.Data.Common.CommandTrees.Internal { internal class ExpressionList : IList{ private string _name; private DbCommandTree _commandTree; private List _list; private ExpressionList(string name, DbCommandTree owner) { _name = name; _commandTree = owner; } internal List ExpressionLinks { get { return _list; } } internal ExpressionList(string name, DbCommandTree owner, int capacity) : this(name, owner) { _list = new List (capacity); for(int idx = 0; idx < capacity; idx++) { _list.Add(new ExpressionLink(CommandTreeUtils.FormatIndex(_name, idx), owner)); } } internal ExpressionList(string name, DbCommandTree owner, IList args) : this(name, owner) { Debug.Assert(args != null, "Argument list is null?"); int capacity = args.Count; List list = new List (capacity); for (int idx = 0; idx < capacity; idx++) { list.Add(new ExpressionLink(CommandTreeUtils.FormatIndex(_name, idx), _commandTree, args[idx])); } _list = list; } internal ExpressionList(string name, DbCommandTree owner, TypeUsage expectedElementType, IList args) : this(name, owner) { Debug.Assert(args != null, "Argument list is null?"); int capacity = args.Count; List list = new List (capacity); for (int idx = 0; idx < capacity; idx++) { list.Add(new ExpressionLink(CommandTreeUtils.FormatIndex(_name, idx), _commandTree, expectedElementType, args[idx])); } _list = list; } internal ExpressionList(string name, DbCommandTree owner, PrimitiveTypeKind expectedElementPrimitiveType, IList args) : this(name, owner) { Debug.Assert(args != null, "Argument list is null?"); int capacity = args.Count; List list = new List (capacity); for (int idx = 0; idx < capacity; idx++) { list.Add(new ExpressionLink(CommandTreeUtils.FormatIndex(_name, idx), _commandTree, expectedElementPrimitiveType, args[idx])); } _list = list; } // // Called by DbFunctionAggregate and DbFunctionExpression // internal ExpressionList(string name, DbCommandTree owner, ReadOnlyMetadataCollection paramInfo, IList args) : this(name, owner) { int capacity = paramInfo.Count; List list = new List (capacity); for (int idx = 0; idx < capacity; idx++) { string elemName = CommandTreeUtils.FormatIndex(_name, idx); FunctionParameter paramMeta = paramInfo[idx]; _commandTree.TypeHelper.CheckParameter(paramMeta, elemName); list.Add(new ExpressionLink(elemName, _commandTree, paramMeta.TypeUsage)); } _list = list; this.SetElements(args); } internal ExpressionList(string name, DbCommandTree owner, IBaseList members, IList args) : this(name, owner) { _list = new List (); int idx = 0; foreach (EdmMember memberMeta in members) { string elemName = CommandTreeUtils.FormatIndex(_name, idx++); _commandTree.TypeHelper.CheckMember(memberMeta, elemName); _list.Add(new ExpressionLink(elemName, _commandTree, Helper.GetModelTypeUsage(memberMeta))); } this.SetElements(args); } /// /// Sets the value of each element in the list without instructing /// the owning Command Tree to consider itself 'modified'. /// Should only be used to set initial values for the list elements, /// and not called again after that initialization has taken place /// (otherwise the Command Tree's VersionNumber property will not be /// updated even though changes have been made to the list). /// /// The DbExpression values to use as list elements. Must match the expected count of this list. internal void SetElements(IListargs) { int addedCount = 0; for(int idx = 0; idx < args.Count; idx++) { if (idx > _list.Count - 1) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_ExpressionList_IncorrectElementCount, _name); } _list[idx].InitializeValue(args[idx]); addedCount++; } if (addedCount != _list.Count) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_ExpressionList_IncorrectElementCount, _name); } } internal void SetExpectedElementType(TypeUsage type) { foreach (ExpressionLink link in _list) { link.SetExpectedType(type); } } internal TypeUsage GetCommonElementType() { TypeUsage commonType = null; foreach (ExpressionLink exprLink in _list) { if (null == commonType) { commonType = exprLink.Expression.ResultType; } else { commonType = TypeHelpers.GetCommonTypeUsage(commonType, exprLink.Expression.ResultType); } if (null == commonType) { break; } } return commonType; } internal void SetAt(int index, DbExpression value) { _list[index].Expression = value; } #region IList Members int IList .IndexOf(DbExpression item) { for (int idx = 0; idx < _list.Count; idx++) { if (_list[idx].Expression == item) { return idx; } } return -1; } void IList .Insert(int index, DbExpression item) { throw EntityUtil.NotSupported(); } void IList .RemoveAt(int index) { throw EntityUtil.NotSupported(); } DbExpression IList .this[int index] { get { return _list[index].Expression; } #if MUTABLE_CQT set { _list[index].Expression = value; } #else set { throw EntityUtil.NotSupported(); } #endif } #endregion #region ICollection Members void ICollection .Add(DbExpression item) { throw EntityUtil.NotSupported(); } void ICollection .Clear() { throw EntityUtil.NotSupported(); } bool ICollection .Contains(DbExpression item) { return (((IList )this).IndexOf(item) > -1); } void ICollection .CopyTo(DbExpression[] array, int arrayIndex) { List exprs = new List (); foreach (ExpressionLink exprLink in _list) { exprs.Add(exprLink.Expression); } exprs.CopyTo(array, arrayIndex); } int ICollection .Count { get { return _list.Count; } } bool ICollection .IsReadOnly { #if MUTABLE_CQT get { return false; } #else get { return true; } #endif } bool ICollection .Remove(DbExpression item) { throw EntityUtil.NotSupported(); } #endregion #region IEnumerable Members IEnumerator IEnumerable .GetEnumerator() { return new ExpressionListEnumerator(_list.GetEnumerator()); } #endregion #region IEnumerable Members System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion #region Nested Enumerator class private class ExpressionListEnumerator : IEnumerator { private IEnumerator m_Enumerator; internal ExpressionListEnumerator(IEnumerator innerEnum) { this.m_Enumerator = innerEnum; } #region IEnumerator Members DbExpression IEnumerator .Current { get { return this.m_Enumerator.Current.Expression; } } #endregion #region IDisposable Members void IDisposable.Dispose() { this.m_Enumerator.Dispose(); } #endregion #region IEnumerator Members object System.Collections.IEnumerator.Current { get { return this.m_Enumerator.Current.Expression; } } bool System.Collections.IEnumerator.MoveNext() { return this.m_Enumerator.MoveNext(); } void System.Collections.IEnumerator.Reset() { this.m_Enumerator.Reset(); } #endregion } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
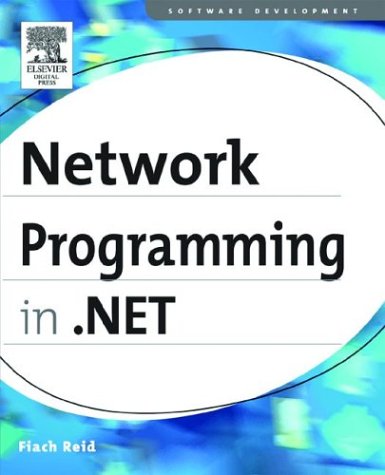
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TripleDES.cs
- ElementProxy.cs
- SortDescriptionCollection.cs
- EncodingTable.cs
- AddInAdapter.cs
- ToolStripStatusLabel.cs
- KeyConstraint.cs
- SrgsNameValueTag.cs
- WebHeaderCollection.cs
- TrustManager.cs
- XmlHierarchicalEnumerable.cs
- ObjectItemCollectionAssemblyCacheEntry.cs
- Filter.cs
- EntitySqlQueryBuilder.cs
- EntityTypeEmitter.cs
- StyleSelector.cs
- ReaderWriterLockWrapper.cs
- XmlAttributeCache.cs
- UrlAuthorizationModule.cs
- ConfigurationManagerHelper.cs
- URL.cs
- InternalEnumValidator.cs
- JournalEntryStack.cs
- BitmapDownload.cs
- TextRangeEditTables.cs
- PrintSchema.cs
- ObjectItemConventionAssemblyLoader.cs
- COM2IPerPropertyBrowsingHandler.cs
- SqlXmlStorage.cs
- GeneralTransform.cs
- EncoderBestFitFallback.cs
- CatalogPartChrome.cs
- SafeFindHandle.cs
- EdmEntityTypeAttribute.cs
- DataGridViewComboBoxCell.cs
- HostProtectionException.cs
- ValuePatternIdentifiers.cs
- TaskScheduler.cs
- BitmapScalingModeValidation.cs
- AmbientValueAttribute.cs
- Transactions.cs
- KnownBoxes.cs
- GridItemPattern.cs
- BitmapData.cs
- ViewBase.cs
- OutputWindow.cs
- SystemFonts.cs
- HttpCapabilitiesEvaluator.cs
- PageAction.cs
- StateManagedCollection.cs
- AnnotationResource.cs
- SqlUDTStorage.cs
- CollectionViewGroupInternal.cs
- IntranetCredentialPolicy.cs
- RegistryConfigurationProvider.cs
- FixedSOMTableRow.cs
- Convert.cs
- MediaSystem.cs
- AsyncInvokeContext.cs
- UInt16.cs
- WebPartDisplayModeCancelEventArgs.cs
- BitVector32.cs
- CodeExpressionRuleDeclaration.cs
- MenuItemCollection.cs
- BitmapEffectrendercontext.cs
- WebSysDisplayNameAttribute.cs
- BitVector32.cs
- MatrixConverter.cs
- BStrWrapper.cs
- RuntimeHandles.cs
- XmlValueConverter.cs
- TextBoxBase.cs
- InvokeMethod.cs
- EntityProviderFactory.cs
- DoubleMinMaxAggregationOperator.cs
- ReadOnlyTernaryTree.cs
- EntityParameter.cs
- DoubleAnimationUsingPath.cs
- LineServicesCallbacks.cs
- SizeF.cs
- ClientProtocol.cs
- DispatcherObject.cs
- XPathParser.cs
- RefExpr.cs
- PerfCounters.cs
- MediaContext.cs
- HelpFileFileNameEditor.cs
- ServicesUtilities.cs
- WorkflowView.cs
- NotSupportedException.cs
- FullTextLine.cs
- Paragraph.cs
- X509ThumbprintKeyIdentifierClause.cs
- HitTestDrawingContextWalker.cs
- ClipboardProcessor.cs
- RuleProcessor.cs
- XmlMessageFormatter.cs
- JpegBitmapDecoder.cs
- DesignerTextBoxAdapter.cs
- WebBrowser.cs