Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripComboBox.cs / 1 / ToolStripComboBox.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Windows.Forms; using System.ComponentModel; using System.Diagnostics; using System.Drawing.Design; using System.Collections.Specialized; using System.Drawing.Drawing2D; using System.Windows.Forms.Design; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Windows.Forms.Internal; using System.Globalization; ////// [ToolStripItemDesignerAvailability(ToolStripItemDesignerAvailability.MenuStrip | ToolStripItemDesignerAvailability.ToolStrip | ToolStripItemDesignerAvailability.ContextMenuStrip)] [DefaultProperty("Items")] public class ToolStripComboBox : ToolStripControlHost { internal static readonly object EventDropDown = new object(); internal static readonly object EventDropDownClosed = new object(); internal static readonly object EventDropDownStyleChanged = new object(); internal static readonly object EventSelectedIndexChanged = new object(); internal static readonly object EventSelectionChangeCommitted = new object(); internal static readonly object EventTextUpdate = new object(); /// public ToolStripComboBox() : base(CreateControlInstance()) { ToolStripComboBoxControl combo = Control as ToolStripComboBoxControl; combo.Owner = this; } public ToolStripComboBox(string name) : this() { this.Name = name; } /// [EditorBrowsable(EditorBrowsableState.Never)] public ToolStripComboBox(Control c) : base(c) { throw new NotSupportedException(SR.GetString(SR.ToolStripMustSupplyItsOwnComboBox)); } private static Control CreateControlInstance() { ComboBox comboBox = new ToolStripComboBoxControl(); comboBox.FlatStyle = FlatStyle.Popup; comboBox.Font = ToolStripManager.DefaultFont; return comboBox; } /// [ DesignerSerializationVisibility(DesignerSerializationVisibility.Content), Localizable(true), SRDescription(SR.ComboBoxAutoCompleteCustomSourceDescr), Editor("System.Windows.Forms.Design.ListControlStringCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), Browsable(true), EditorBrowsable(EditorBrowsableState.Always) ] public System.Windows.Forms.AutoCompleteStringCollection AutoCompleteCustomSource { get { return ComboBox.AutoCompleteCustomSource; } set { ComboBox.AutoCompleteCustomSource = value;} } /// [ DefaultValue(AutoCompleteMode.None), SRDescription(SR.ComboBoxAutoCompleteModeDescr), Browsable(true), EditorBrowsable(EditorBrowsableState.Always) ] public AutoCompleteMode AutoCompleteMode { get { return ComboBox.AutoCompleteMode; } set { ComboBox.AutoCompleteMode = value;} } /// [ DefaultValue(AutoCompleteSource.None), SRDescription(SR.ComboBoxAutoCompleteSourceDescr), Browsable(true), EditorBrowsable(EditorBrowsableState.Always) ] public AutoCompleteSource AutoCompleteSource { get { return ComboBox.AutoCompleteSource; } set { ComboBox.AutoCompleteSource = value;} } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public override Image BackgroundImage { get { return base.BackgroundImage; } set { base.BackgroundImage = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override ImageLayout BackgroundImageLayout { get { return base.BackgroundImageLayout; } set { base.BackgroundImageLayout = value; } } /// [Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public ComboBox ComboBox { get{ return Control as ComboBox; } } /// protected override Size DefaultSize { get { return new Size(100,22); } } /// /// /// Deriving classes can override this to configure a default size for their control. /// This is more efficient than setting the size in the control's constructor. /// protected internal override Padding DefaultMargin { get { if (IsOnDropDown) { return new Padding(2); } else { return new Padding(1, 0, 1, 0); } } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never) ] public new event EventHandler DoubleClick { add { base.DoubleClick+=value; } remove { base.DoubleClick -= value; } } ///[SRCategory(SR.CatBehavior), SRDescription(SR.ComboBoxOnDropDownDescr)] public event EventHandler DropDown { add { Events.AddHandler(EventDropDown, value); } remove { Events.RemoveHandler(EventDropDown, value); } } /// [SRCategory(SR.CatBehavior), SRDescription(SR.ComboBoxOnDropDownClosedDescr)] public event EventHandler DropDownClosed { add { Events.AddHandler(EventDropDownClosed, value); } remove { Events.RemoveHandler(EventDropDownClosed, value); } } /// [SRCategory(SR.CatBehavior), SRDescription(SR.ComboBoxDropDownStyleChangedDescr)] public event EventHandler DropDownStyleChanged { add { Events.AddHandler(EventDropDownStyleChanged, value); } remove { Events.RemoveHandler(EventDropDownStyleChanged, value); } } /// [ SRCategory(SR.CatBehavior), SRDescription(SR.ComboBoxDropDownHeightDescr), Browsable(true), EditorBrowsable(EditorBrowsableState.Always), DefaultValue(106) ] public int DropDownHeight { get { return ComboBox.DropDownHeight; } set { ComboBox.DropDownHeight = value;} } /// [ SRCategory(SR.CatAppearance), DefaultValue(ComboBoxStyle.DropDown), SRDescription(SR.ComboBoxStyleDescr), RefreshPropertiesAttribute(RefreshProperties.Repaint) ] public ComboBoxStyle DropDownStyle { get { return ComboBox.DropDownStyle; } set { ComboBox.DropDownStyle = value;} } /// [ SRCategory(SR.CatBehavior), SRDescription(SR.ComboBoxDropDownWidthDescr) ] public int DropDownWidth { get { return ComboBox.DropDownWidth; } set { ComboBox.DropDownWidth = value;} } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ComboBoxDroppedDownDescr) ] public bool DroppedDown { get { return ComboBox.DroppedDown; } set { ComboBox.DroppedDown = value;} } /// [ SRCategory(SR.CatAppearance), DefaultValue(FlatStyle.Popup), Localizable(true), SRDescription(SR.ComboBoxFlatStyleDescr) ] public FlatStyle FlatStyle { get { return ComboBox.FlatStyle; } set { ComboBox.FlatStyle = value;} } /// [ SRCategory(SR.CatBehavior), DefaultValue(true), Localizable(true), SRDescription(SR.ComboBoxIntegralHeightDescr) ] public bool IntegralHeight { get { return ComboBox.IntegralHeight; } set { ComboBox.IntegralHeight = value;} } /// /// /// Collection of the items contained in this ComboBox. /// [ SRCategory(SR.CatData), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), Localizable(true), SRDescription(SR.ComboBoxItemsDescr), Editor("System.Windows.Forms.Design.ListControlStringCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] public ComboBox.ObjectCollection Items { get{ return ComboBox.Items; } } ///[ SRCategory(SR.CatBehavior), DefaultValue(8), Localizable(true), SRDescription(SR.ComboBoxMaxDropDownItemsDescr) ] public int MaxDropDownItems { get { return ComboBox.MaxDropDownItems; } set { ComboBox.MaxDropDownItems = value;} } /// [ SRCategory(SR.CatBehavior), DefaultValue(0), Localizable(true), SRDescription(SR.ComboBoxMaxLengthDescr) ] public int MaxLength { get { return ComboBox.MaxLength; } set { ComboBox.MaxLength = value; } } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ComboBoxSelectedIndexDescr) ] public int SelectedIndex { get { return ComboBox.SelectedIndex; } set { ComboBox.SelectedIndex = value;} } /// [SRCategory(SR.CatBehavior), SRDescription(SR.selectedIndexChangedEventDescr)] public event EventHandler SelectedIndexChanged { add { Events.AddHandler(EventSelectedIndexChanged, value); } remove { Events.RemoveHandler(EventSelectedIndexChanged, value); } } /// [ Browsable(false), Bindable(true), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ComboBoxSelectedItemDescr) ] public object SelectedItem { get { return ComboBox.SelectedItem; } set { ComboBox.SelectedItem = value;} } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ComboBoxSelectedTextDescr) ] public string SelectedText { get { return ComboBox.SelectedText; } set { ComboBox.SelectedText = value;} } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ComboBoxSelectionLengthDescr) ] public int SelectionLength { get { return ComboBox.SelectionLength; } set { ComboBox.SelectionLength = value;} } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ComboBoxSelectionStartDescr) ] public int SelectionStart { get { return ComboBox.SelectionStart; } set { ComboBox.SelectionStart = value;} } /// [ SRCategory(SR.CatBehavior), DefaultValue(false), SRDescription(SR.ComboBoxSortedDescr) ] public bool Sorted { get { return ComboBox.Sorted; } set {ComboBox.Sorted = value;} } /// [SRCategory(SR.CatBehavior), SRDescription(SR.ComboBoxOnTextUpdateDescr)] public event EventHandler TextUpdate { add { Events.AddHandler(EventTextUpdate, value); } remove { Events.RemoveHandler(EventTextUpdate, value); } } #region WrappedMethods /// public void BeginUpdate() { ComboBox.BeginUpdate(); } /// public void EndUpdate() { ComboBox.EndUpdate(); } /// public int FindString(string s) { return ComboBox.FindString(s); } /// public int FindString(string s, int startIndex) { return ComboBox.FindString(s, startIndex); } /// public int FindStringExact(string s) { return ComboBox.FindStringExact(s); } /// public int FindStringExact(string s, int startIndex) { return ComboBox.FindStringExact(s, startIndex); } /// public int GetItemHeight(int index) { return ComboBox.GetItemHeight(index); } /// public void Select(int start, int length) { ComboBox.Select(start, length); } /// public void SelectAll() { ComboBox.SelectAll(); } #endregion WrappedMethods /// public override Size GetPreferredSize(Size constrainingSize) { // Size preferredSize = base.GetPreferredSize(constrainingSize); preferredSize.Width = Math.Max(preferredSize.Width, 75); return preferredSize; } private void HandleDropDown(object sender, System.EventArgs e) { OnDropDown(e); } private void HandleDropDownClosed(object sender, System.EventArgs e) { OnDropDownClosed(e); } private void HandleDropDownStyleChanged(object sender, System.EventArgs e) { OnDropDownStyleChanged(e); } private void HandleSelectedIndexChanged(object sender, System.EventArgs e) { OnSelectedIndexChanged(e); } private void HandleSelectionChangeCommitted(object sender, System.EventArgs e) { OnSelectionChangeCommitted(e); } private void HandleTextUpdate(object sender, System.EventArgs e) { OnTextUpdate(e); } /// protected virtual void OnDropDown(EventArgs e) { if (ParentInternal != null) { // PERF, SECREVIEW: dont call Application.RemoveMessageFilter as this could // get called a lot and we want to have to assert AWP. Application.ThreadContext.FromCurrent().RemoveMessageFilter(ParentInternal.RestoreFocusFilter); ToolStripManager.ModalMenuFilter.SuspendMenuMode(); } RaiseEvent(EventDropDown, e); } /// protected virtual void OnDropDownClosed(EventArgs e) { if (ParentInternal != null) { // PERF, SECREVIEW: dont call Application.RemoveMessageFilter as this could // get called a lot and we want to have to assert AWP. Application.ThreadContext.FromCurrent().RemoveMessageFilter(ParentInternal.RestoreFocusFilter); ToolStripManager.ModalMenuFilter.ResumeMenuMode(); } RaiseEvent(EventDropDownClosed, e); } /// protected virtual void OnDropDownStyleChanged(EventArgs e) { RaiseEvent(EventDropDownStyleChanged, e); } /// protected virtual void OnSelectedIndexChanged(EventArgs e) { RaiseEvent(EventSelectedIndexChanged, e); } /// protected virtual void OnSelectionChangeCommitted(EventArgs e) { RaiseEvent(EventSelectionChangeCommitted, e); } /// protected virtual void OnTextUpdate(EventArgs e) { RaiseEvent(EventTextUpdate, e); } /// protected override void OnSubscribeControlEvents(Control control) { ComboBox comboBox = control as ComboBox; if (comboBox != null) { // Please keep this alphabetized and in [....] with Unsubscribe // comboBox.DropDown += new EventHandler(HandleDropDown); comboBox.DropDownClosed += new EventHandler(HandleDropDownClosed); comboBox.DropDownStyleChanged += new EventHandler(HandleDropDownStyleChanged); comboBox.SelectedIndexChanged += new EventHandler(HandleSelectedIndexChanged); comboBox.SelectionChangeCommitted += new EventHandler(HandleSelectionChangeCommitted); comboBox.TextUpdate += new EventHandler(HandleTextUpdate); } base.OnSubscribeControlEvents(control); } /// protected override void OnUnsubscribeControlEvents(Control control) { ComboBox comboBox = control as ComboBox; if (comboBox != null) { // Please keep this alphabetized and in [....] with Unsubscribe // comboBox.DropDown -= new EventHandler(HandleDropDown); comboBox.DropDownClosed -= new EventHandler(HandleDropDownClosed); comboBox.DropDownStyleChanged -= new EventHandler(HandleDropDownStyleChanged); comboBox.SelectedIndexChanged -= new EventHandler(HandleSelectedIndexChanged); comboBox.SelectionChangeCommitted -= new EventHandler(HandleSelectionChangeCommitted); comboBox.TextUpdate -= new EventHandler(HandleTextUpdate); } base.OnUnsubscribeControlEvents(control); } private bool ShouldSerializeDropDownWidth() { return ComboBox.ShouldSerializeDropDownWidth(); } internal override bool ShouldSerializeFont() { return !object.Equals(this.Font, ToolStripManager.DefaultFont); } public override string ToString() { return base.ToString() + ", Items.Count: " + Items.Count.ToString(CultureInfo.CurrentCulture); } internal class ToolStripComboBoxControl : ComboBox { private ToolStripComboBox owner = null; public ToolStripComboBoxControl() { this.FlatStyle = FlatStyle.Popup; SetStyle(ControlStyles.ResizeRedraw | ControlStyles.OptimizedDoubleBuffer, true); } public ToolStripComboBox Owner { get { return owner; } set { owner = value; } } private ProfessionalColorTable ColorTable { get { if (Owner != null) { ToolStripProfessionalRenderer renderer = Owner.Renderer as ToolStripProfessionalRenderer; if (renderer != null) { return renderer.ColorTable; } } return ProfessionalColors.ColorTable; } } internal override FlatComboAdapter CreateFlatComboAdapterInstance() { return new ToolStripComboBoxFlatComboAdapter(this); } internal class ToolStripComboBoxFlatComboAdapter : FlatComboAdapter { public ToolStripComboBoxFlatComboAdapter(ComboBox comboBox) : base(comboBox, /*smallButton=*/true) { } private static bool UseBaseAdapter(ComboBox comboBox) { ToolStripComboBoxControl toolStripComboBox = comboBox as ToolStripComboBoxControl; if (toolStripComboBox == null || !(toolStripComboBox.Owner.Renderer is ToolStripProfessionalRenderer)) { Debug.Assert(toolStripComboBox != null, "Why are we here and not a toolstrip combo?"); return true; } return false; } private static ProfessionalColorTable GetColorTable(ToolStripComboBoxControl toolStripComboBoxControl) { if (toolStripComboBoxControl != null) { return toolStripComboBoxControl.ColorTable; } return ProfessionalColors.ColorTable; } protected override Color GetOuterBorderColor(ComboBox comboBox) { if (UseBaseAdapter(comboBox)) { return base.GetOuterBorderColor(comboBox); } return (comboBox.Enabled) ? SystemColors.Window : GetColorTable(comboBox as ToolStripComboBoxControl).ComboBoxBorder; } protected override Color GetPopupOuterBorderColor(ComboBox comboBox, bool focused) { if (UseBaseAdapter(comboBox)) { return base.GetPopupOuterBorderColor(comboBox, focused); } if (!comboBox.Enabled) { return SystemColors.ControlDark; } return (focused) ? GetColorTable(comboBox as ToolStripComboBoxControl).ComboBoxBorder : SystemColors.Window; } protected override void DrawFlatComboDropDown(ComboBox comboBox, Graphics g, Rectangle dropDownRect) { if (UseBaseAdapter(comboBox)) { base.DrawFlatComboDropDown(comboBox, g, dropDownRect); return; } if (!comboBox.Enabled || !ToolStripManager.VisualStylesEnabled) { g.FillRectangle(SystemBrushes.Control, dropDownRect); } else { ToolStripComboBoxControl toolStripComboBox = comboBox as ToolStripComboBoxControl; ProfessionalColorTable colorTable = GetColorTable(toolStripComboBox); if (!comboBox.DroppedDown) { bool focused = comboBox.ContainsFocus || comboBox.MouseIsOver; if (focused) { using (Brush b = new LinearGradientBrush(dropDownRect, colorTable.ComboBoxButtonSelectedGradientBegin, colorTable.ComboBoxButtonSelectedGradientEnd, LinearGradientMode.Vertical)) { g.FillRectangle(b, dropDownRect); } } else if (toolStripComboBox.Owner.IsOnOverflow) { using (Brush b = new SolidBrush(colorTable.ComboBoxButtonOnOverflow)) { g.FillRectangle(b, dropDownRect); } } else { using (Brush b = new LinearGradientBrush(dropDownRect, colorTable.ComboBoxButtonGradientBegin, colorTable.ComboBoxButtonGradientEnd, LinearGradientMode.Vertical)) { g.FillRectangle(b, dropDownRect); } } } else { using (Brush b = new LinearGradientBrush(dropDownRect, colorTable.ComboBoxButtonPressedGradientBegin, colorTable.ComboBoxButtonPressedGradientEnd, LinearGradientMode.Vertical)) { g.FillRectangle(b, dropDownRect); } } } Brush brush = (comboBox.Enabled) ? SystemBrushes.ControlText : SystemBrushes.GrayText; Point middle = new Point(dropDownRect.Left + dropDownRect.Width / 2, dropDownRect.Top + dropDownRect.Height / 2); // if the width is odd - favor pushing it over one pixel right. middle.X += (dropDownRect.Width % 2); g.FillPolygon(brush, new Point[] { new Point(middle.X - 2, middle.Y - 1), new Point(middle.X + 3, middle.Y - 1), new Point(middle.X, middle.Y + 2) }); } } protected override bool IsInputKey(Keys keyData) { if ((keyData & Keys.Alt) == Keys.Alt) { if ((keyData & Keys.Down) == Keys.Down || (keyData & Keys.Up) == Keys.Up) { return true; } } return base.IsInputKey(keyData); } protected override void OnDropDownClosed(EventArgs e) { base.OnDropDownClosed(e); Invalidate(); Update(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Windows.Forms; using System.ComponentModel; using System.Diagnostics; using System.Drawing.Design; using System.Collections.Specialized; using System.Drawing.Drawing2D; using System.Windows.Forms.Design; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Windows.Forms.Internal; using System.Globalization; ////// [ToolStripItemDesignerAvailability(ToolStripItemDesignerAvailability.MenuStrip | ToolStripItemDesignerAvailability.ToolStrip | ToolStripItemDesignerAvailability.ContextMenuStrip)] [DefaultProperty("Items")] public class ToolStripComboBox : ToolStripControlHost { internal static readonly object EventDropDown = new object(); internal static readonly object EventDropDownClosed = new object(); internal static readonly object EventDropDownStyleChanged = new object(); internal static readonly object EventSelectedIndexChanged = new object(); internal static readonly object EventSelectionChangeCommitted = new object(); internal static readonly object EventTextUpdate = new object(); /// public ToolStripComboBox() : base(CreateControlInstance()) { ToolStripComboBoxControl combo = Control as ToolStripComboBoxControl; combo.Owner = this; } public ToolStripComboBox(string name) : this() { this.Name = name; } /// [EditorBrowsable(EditorBrowsableState.Never)] public ToolStripComboBox(Control c) : base(c) { throw new NotSupportedException(SR.GetString(SR.ToolStripMustSupplyItsOwnComboBox)); } private static Control CreateControlInstance() { ComboBox comboBox = new ToolStripComboBoxControl(); comboBox.FlatStyle = FlatStyle.Popup; comboBox.Font = ToolStripManager.DefaultFont; return comboBox; } /// [ DesignerSerializationVisibility(DesignerSerializationVisibility.Content), Localizable(true), SRDescription(SR.ComboBoxAutoCompleteCustomSourceDescr), Editor("System.Windows.Forms.Design.ListControlStringCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), Browsable(true), EditorBrowsable(EditorBrowsableState.Always) ] public System.Windows.Forms.AutoCompleteStringCollection AutoCompleteCustomSource { get { return ComboBox.AutoCompleteCustomSource; } set { ComboBox.AutoCompleteCustomSource = value;} } /// [ DefaultValue(AutoCompleteMode.None), SRDescription(SR.ComboBoxAutoCompleteModeDescr), Browsable(true), EditorBrowsable(EditorBrowsableState.Always) ] public AutoCompleteMode AutoCompleteMode { get { return ComboBox.AutoCompleteMode; } set { ComboBox.AutoCompleteMode = value;} } /// [ DefaultValue(AutoCompleteSource.None), SRDescription(SR.ComboBoxAutoCompleteSourceDescr), Browsable(true), EditorBrowsable(EditorBrowsableState.Always) ] public AutoCompleteSource AutoCompleteSource { get { return ComboBox.AutoCompleteSource; } set { ComboBox.AutoCompleteSource = value;} } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public override Image BackgroundImage { get { return base.BackgroundImage; } set { base.BackgroundImage = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override ImageLayout BackgroundImageLayout { get { return base.BackgroundImageLayout; } set { base.BackgroundImageLayout = value; } } /// [Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public ComboBox ComboBox { get{ return Control as ComboBox; } } /// protected override Size DefaultSize { get { return new Size(100,22); } } /// /// /// Deriving classes can override this to configure a default size for their control. /// This is more efficient than setting the size in the control's constructor. /// protected internal override Padding DefaultMargin { get { if (IsOnDropDown) { return new Padding(2); } else { return new Padding(1, 0, 1, 0); } } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never) ] public new event EventHandler DoubleClick { add { base.DoubleClick+=value; } remove { base.DoubleClick -= value; } } ///[SRCategory(SR.CatBehavior), SRDescription(SR.ComboBoxOnDropDownDescr)] public event EventHandler DropDown { add { Events.AddHandler(EventDropDown, value); } remove { Events.RemoveHandler(EventDropDown, value); } } /// [SRCategory(SR.CatBehavior), SRDescription(SR.ComboBoxOnDropDownClosedDescr)] public event EventHandler DropDownClosed { add { Events.AddHandler(EventDropDownClosed, value); } remove { Events.RemoveHandler(EventDropDownClosed, value); } } /// [SRCategory(SR.CatBehavior), SRDescription(SR.ComboBoxDropDownStyleChangedDescr)] public event EventHandler DropDownStyleChanged { add { Events.AddHandler(EventDropDownStyleChanged, value); } remove { Events.RemoveHandler(EventDropDownStyleChanged, value); } } /// [ SRCategory(SR.CatBehavior), SRDescription(SR.ComboBoxDropDownHeightDescr), Browsable(true), EditorBrowsable(EditorBrowsableState.Always), DefaultValue(106) ] public int DropDownHeight { get { return ComboBox.DropDownHeight; } set { ComboBox.DropDownHeight = value;} } /// [ SRCategory(SR.CatAppearance), DefaultValue(ComboBoxStyle.DropDown), SRDescription(SR.ComboBoxStyleDescr), RefreshPropertiesAttribute(RefreshProperties.Repaint) ] public ComboBoxStyle DropDownStyle { get { return ComboBox.DropDownStyle; } set { ComboBox.DropDownStyle = value;} } /// [ SRCategory(SR.CatBehavior), SRDescription(SR.ComboBoxDropDownWidthDescr) ] public int DropDownWidth { get { return ComboBox.DropDownWidth; } set { ComboBox.DropDownWidth = value;} } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ComboBoxDroppedDownDescr) ] public bool DroppedDown { get { return ComboBox.DroppedDown; } set { ComboBox.DroppedDown = value;} } /// [ SRCategory(SR.CatAppearance), DefaultValue(FlatStyle.Popup), Localizable(true), SRDescription(SR.ComboBoxFlatStyleDescr) ] public FlatStyle FlatStyle { get { return ComboBox.FlatStyle; } set { ComboBox.FlatStyle = value;} } /// [ SRCategory(SR.CatBehavior), DefaultValue(true), Localizable(true), SRDescription(SR.ComboBoxIntegralHeightDescr) ] public bool IntegralHeight { get { return ComboBox.IntegralHeight; } set { ComboBox.IntegralHeight = value;} } /// /// /// Collection of the items contained in this ComboBox. /// [ SRCategory(SR.CatData), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), Localizable(true), SRDescription(SR.ComboBoxItemsDescr), Editor("System.Windows.Forms.Design.ListControlStringCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] public ComboBox.ObjectCollection Items { get{ return ComboBox.Items; } } ///[ SRCategory(SR.CatBehavior), DefaultValue(8), Localizable(true), SRDescription(SR.ComboBoxMaxDropDownItemsDescr) ] public int MaxDropDownItems { get { return ComboBox.MaxDropDownItems; } set { ComboBox.MaxDropDownItems = value;} } /// [ SRCategory(SR.CatBehavior), DefaultValue(0), Localizable(true), SRDescription(SR.ComboBoxMaxLengthDescr) ] public int MaxLength { get { return ComboBox.MaxLength; } set { ComboBox.MaxLength = value; } } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ComboBoxSelectedIndexDescr) ] public int SelectedIndex { get { return ComboBox.SelectedIndex; } set { ComboBox.SelectedIndex = value;} } /// [SRCategory(SR.CatBehavior), SRDescription(SR.selectedIndexChangedEventDescr)] public event EventHandler SelectedIndexChanged { add { Events.AddHandler(EventSelectedIndexChanged, value); } remove { Events.RemoveHandler(EventSelectedIndexChanged, value); } } /// [ Browsable(false), Bindable(true), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ComboBoxSelectedItemDescr) ] public object SelectedItem { get { return ComboBox.SelectedItem; } set { ComboBox.SelectedItem = value;} } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ComboBoxSelectedTextDescr) ] public string SelectedText { get { return ComboBox.SelectedText; } set { ComboBox.SelectedText = value;} } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ComboBoxSelectionLengthDescr) ] public int SelectionLength { get { return ComboBox.SelectionLength; } set { ComboBox.SelectionLength = value;} } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ComboBoxSelectionStartDescr) ] public int SelectionStart { get { return ComboBox.SelectionStart; } set { ComboBox.SelectionStart = value;} } /// [ SRCategory(SR.CatBehavior), DefaultValue(false), SRDescription(SR.ComboBoxSortedDescr) ] public bool Sorted { get { return ComboBox.Sorted; } set {ComboBox.Sorted = value;} } /// [SRCategory(SR.CatBehavior), SRDescription(SR.ComboBoxOnTextUpdateDescr)] public event EventHandler TextUpdate { add { Events.AddHandler(EventTextUpdate, value); } remove { Events.RemoveHandler(EventTextUpdate, value); } } #region WrappedMethods /// public void BeginUpdate() { ComboBox.BeginUpdate(); } /// public void EndUpdate() { ComboBox.EndUpdate(); } /// public int FindString(string s) { return ComboBox.FindString(s); } /// public int FindString(string s, int startIndex) { return ComboBox.FindString(s, startIndex); } /// public int FindStringExact(string s) { return ComboBox.FindStringExact(s); } /// public int FindStringExact(string s, int startIndex) { return ComboBox.FindStringExact(s, startIndex); } /// public int GetItemHeight(int index) { return ComboBox.GetItemHeight(index); } /// public void Select(int start, int length) { ComboBox.Select(start, length); } /// public void SelectAll() { ComboBox.SelectAll(); } #endregion WrappedMethods /// public override Size GetPreferredSize(Size constrainingSize) { // Size preferredSize = base.GetPreferredSize(constrainingSize); preferredSize.Width = Math.Max(preferredSize.Width, 75); return preferredSize; } private void HandleDropDown(object sender, System.EventArgs e) { OnDropDown(e); } private void HandleDropDownClosed(object sender, System.EventArgs e) { OnDropDownClosed(e); } private void HandleDropDownStyleChanged(object sender, System.EventArgs e) { OnDropDownStyleChanged(e); } private void HandleSelectedIndexChanged(object sender, System.EventArgs e) { OnSelectedIndexChanged(e); } private void HandleSelectionChangeCommitted(object sender, System.EventArgs e) { OnSelectionChangeCommitted(e); } private void HandleTextUpdate(object sender, System.EventArgs e) { OnTextUpdate(e); } /// protected virtual void OnDropDown(EventArgs e) { if (ParentInternal != null) { // PERF, SECREVIEW: dont call Application.RemoveMessageFilter as this could // get called a lot and we want to have to assert AWP. Application.ThreadContext.FromCurrent().RemoveMessageFilter(ParentInternal.RestoreFocusFilter); ToolStripManager.ModalMenuFilter.SuspendMenuMode(); } RaiseEvent(EventDropDown, e); } /// protected virtual void OnDropDownClosed(EventArgs e) { if (ParentInternal != null) { // PERF, SECREVIEW: dont call Application.RemoveMessageFilter as this could // get called a lot and we want to have to assert AWP. Application.ThreadContext.FromCurrent().RemoveMessageFilter(ParentInternal.RestoreFocusFilter); ToolStripManager.ModalMenuFilter.ResumeMenuMode(); } RaiseEvent(EventDropDownClosed, e); } /// protected virtual void OnDropDownStyleChanged(EventArgs e) { RaiseEvent(EventDropDownStyleChanged, e); } /// protected virtual void OnSelectedIndexChanged(EventArgs e) { RaiseEvent(EventSelectedIndexChanged, e); } /// protected virtual void OnSelectionChangeCommitted(EventArgs e) { RaiseEvent(EventSelectionChangeCommitted, e); } /// protected virtual void OnTextUpdate(EventArgs e) { RaiseEvent(EventTextUpdate, e); } /// protected override void OnSubscribeControlEvents(Control control) { ComboBox comboBox = control as ComboBox; if (comboBox != null) { // Please keep this alphabetized and in [....] with Unsubscribe // comboBox.DropDown += new EventHandler(HandleDropDown); comboBox.DropDownClosed += new EventHandler(HandleDropDownClosed); comboBox.DropDownStyleChanged += new EventHandler(HandleDropDownStyleChanged); comboBox.SelectedIndexChanged += new EventHandler(HandleSelectedIndexChanged); comboBox.SelectionChangeCommitted += new EventHandler(HandleSelectionChangeCommitted); comboBox.TextUpdate += new EventHandler(HandleTextUpdate); } base.OnSubscribeControlEvents(control); } /// protected override void OnUnsubscribeControlEvents(Control control) { ComboBox comboBox = control as ComboBox; if (comboBox != null) { // Please keep this alphabetized and in [....] with Unsubscribe // comboBox.DropDown -= new EventHandler(HandleDropDown); comboBox.DropDownClosed -= new EventHandler(HandleDropDownClosed); comboBox.DropDownStyleChanged -= new EventHandler(HandleDropDownStyleChanged); comboBox.SelectedIndexChanged -= new EventHandler(HandleSelectedIndexChanged); comboBox.SelectionChangeCommitted -= new EventHandler(HandleSelectionChangeCommitted); comboBox.TextUpdate -= new EventHandler(HandleTextUpdate); } base.OnUnsubscribeControlEvents(control); } private bool ShouldSerializeDropDownWidth() { return ComboBox.ShouldSerializeDropDownWidth(); } internal override bool ShouldSerializeFont() { return !object.Equals(this.Font, ToolStripManager.DefaultFont); } public override string ToString() { return base.ToString() + ", Items.Count: " + Items.Count.ToString(CultureInfo.CurrentCulture); } internal class ToolStripComboBoxControl : ComboBox { private ToolStripComboBox owner = null; public ToolStripComboBoxControl() { this.FlatStyle = FlatStyle.Popup; SetStyle(ControlStyles.ResizeRedraw | ControlStyles.OptimizedDoubleBuffer, true); } public ToolStripComboBox Owner { get { return owner; } set { owner = value; } } private ProfessionalColorTable ColorTable { get { if (Owner != null) { ToolStripProfessionalRenderer renderer = Owner.Renderer as ToolStripProfessionalRenderer; if (renderer != null) { return renderer.ColorTable; } } return ProfessionalColors.ColorTable; } } internal override FlatComboAdapter CreateFlatComboAdapterInstance() { return new ToolStripComboBoxFlatComboAdapter(this); } internal class ToolStripComboBoxFlatComboAdapter : FlatComboAdapter { public ToolStripComboBoxFlatComboAdapter(ComboBox comboBox) : base(comboBox, /*smallButton=*/true) { } private static bool UseBaseAdapter(ComboBox comboBox) { ToolStripComboBoxControl toolStripComboBox = comboBox as ToolStripComboBoxControl; if (toolStripComboBox == null || !(toolStripComboBox.Owner.Renderer is ToolStripProfessionalRenderer)) { Debug.Assert(toolStripComboBox != null, "Why are we here and not a toolstrip combo?"); return true; } return false; } private static ProfessionalColorTable GetColorTable(ToolStripComboBoxControl toolStripComboBoxControl) { if (toolStripComboBoxControl != null) { return toolStripComboBoxControl.ColorTable; } return ProfessionalColors.ColorTable; } protected override Color GetOuterBorderColor(ComboBox comboBox) { if (UseBaseAdapter(comboBox)) { return base.GetOuterBorderColor(comboBox); } return (comboBox.Enabled) ? SystemColors.Window : GetColorTable(comboBox as ToolStripComboBoxControl).ComboBoxBorder; } protected override Color GetPopupOuterBorderColor(ComboBox comboBox, bool focused) { if (UseBaseAdapter(comboBox)) { return base.GetPopupOuterBorderColor(comboBox, focused); } if (!comboBox.Enabled) { return SystemColors.ControlDark; } return (focused) ? GetColorTable(comboBox as ToolStripComboBoxControl).ComboBoxBorder : SystemColors.Window; } protected override void DrawFlatComboDropDown(ComboBox comboBox, Graphics g, Rectangle dropDownRect) { if (UseBaseAdapter(comboBox)) { base.DrawFlatComboDropDown(comboBox, g, dropDownRect); return; } if (!comboBox.Enabled || !ToolStripManager.VisualStylesEnabled) { g.FillRectangle(SystemBrushes.Control, dropDownRect); } else { ToolStripComboBoxControl toolStripComboBox = comboBox as ToolStripComboBoxControl; ProfessionalColorTable colorTable = GetColorTable(toolStripComboBox); if (!comboBox.DroppedDown) { bool focused = comboBox.ContainsFocus || comboBox.MouseIsOver; if (focused) { using (Brush b = new LinearGradientBrush(dropDownRect, colorTable.ComboBoxButtonSelectedGradientBegin, colorTable.ComboBoxButtonSelectedGradientEnd, LinearGradientMode.Vertical)) { g.FillRectangle(b, dropDownRect); } } else if (toolStripComboBox.Owner.IsOnOverflow) { using (Brush b = new SolidBrush(colorTable.ComboBoxButtonOnOverflow)) { g.FillRectangle(b, dropDownRect); } } else { using (Brush b = new LinearGradientBrush(dropDownRect, colorTable.ComboBoxButtonGradientBegin, colorTable.ComboBoxButtonGradientEnd, LinearGradientMode.Vertical)) { g.FillRectangle(b, dropDownRect); } } } else { using (Brush b = new LinearGradientBrush(dropDownRect, colorTable.ComboBoxButtonPressedGradientBegin, colorTable.ComboBoxButtonPressedGradientEnd, LinearGradientMode.Vertical)) { g.FillRectangle(b, dropDownRect); } } } Brush brush = (comboBox.Enabled) ? SystemBrushes.ControlText : SystemBrushes.GrayText; Point middle = new Point(dropDownRect.Left + dropDownRect.Width / 2, dropDownRect.Top + dropDownRect.Height / 2); // if the width is odd - favor pushing it over one pixel right. middle.X += (dropDownRect.Width % 2); g.FillPolygon(brush, new Point[] { new Point(middle.X - 2, middle.Y - 1), new Point(middle.X + 3, middle.Y - 1), new Point(middle.X, middle.Y + 2) }); } } protected override bool IsInputKey(Keys keyData) { if ((keyData & Keys.Alt) == Keys.Alt) { if ((keyData & Keys.Down) == Keys.Down || (keyData & Keys.Up) == Keys.Up) { return true; } } return base.IsInputKey(keyData); } protected override void OnDropDownClosed(EventArgs e) { base.OnDropDownClosed(e); Invalidate(); Update(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
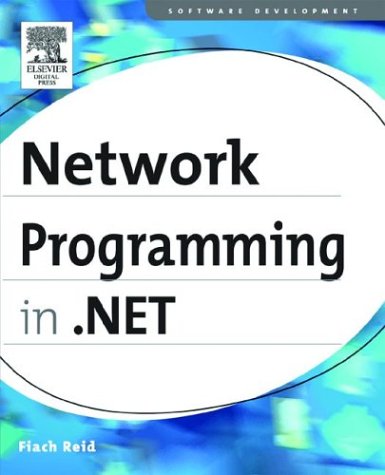
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EpmSyndicationContentSerializer.cs
- ServiceReference.cs
- FixedSOMElement.cs
- KeyGestureConverter.cs
- CodeSnippetStatement.cs
- SpecularMaterial.cs
- COSERVERINFO.cs
- SapiRecoContext.cs
- CacheAxisQuery.cs
- RepeatInfo.cs
- Button.cs
- ContractTypeNameCollection.cs
- NotFiniteNumberException.cs
- COM2Properties.cs
- LayoutUtils.cs
- ListViewSelectEventArgs.cs
- Floater.cs
- SiteMapNodeItemEventArgs.cs
- MenuStrip.cs
- NotificationContext.cs
- DPAPIProtectedConfigurationProvider.cs
- DataControlImageButton.cs
- BadImageFormatException.cs
- StringTraceRecord.cs
- ListView.cs
- DecoratedNameAttribute.cs
- Token.cs
- VirtualPathProvider.cs
- IRCollection.cs
- ComplusTypeValidator.cs
- ArraySubsetEnumerator.cs
- EventLogEntry.cs
- ImageFormat.cs
- XsdCachingReader.cs
- Delay.cs
- TransformGroup.cs
- Quaternion.cs
- SevenBitStream.cs
- AxisAngleRotation3D.cs
- WindowsSspiNegotiation.cs
- EntitySetDataBindingList.cs
- LinearGradientBrush.cs
- TouchFrameEventArgs.cs
- WorkflowWebService.cs
- UniqueIdentifierService.cs
- TreeNodeCollectionEditorDialog.cs
- LinkLabelLinkClickedEvent.cs
- Win32KeyboardDevice.cs
- XsltCompileContext.cs
- NativeCppClassAttribute.cs
- MergeEnumerator.cs
- DrawingDrawingContext.cs
- BuildDependencySet.cs
- CategoryValueConverter.cs
- SspiHelper.cs
- ListComponentEditor.cs
- BaseParaClient.cs
- DataControlCommands.cs
- TypeConverterAttribute.cs
- FormsAuthenticationTicket.cs
- ButtonBaseAdapter.cs
- DataGridViewAdvancedBorderStyle.cs
- InvalidFilterCriteriaException.cs
- SqlDataAdapter.cs
- OperatingSystem.cs
- Identity.cs
- MapPathBasedVirtualPathProvider.cs
- JpegBitmapDecoder.cs
- WindowsTitleBar.cs
- CompiledXpathExpr.cs
- WebControlToolBoxItem.cs
- OracleParameter.cs
- GenerateScriptTypeAttribute.cs
- ConsoleTraceListener.cs
- ToolBarTray.cs
- AttachmentCollection.cs
- PeerCollaborationPermission.cs
- PerformanceCounterManager.cs
- SQLCharsStorage.cs
- ListenDesigner.cs
- EntityParameterCollection.cs
- StateItem.cs
- MDIWindowDialog.cs
- QualifierSet.cs
- HybridDictionary.cs
- DataSourceCache.cs
- DataServiceProcessingPipelineEventArgs.cs
- XmlText.cs
- WebConfigurationFileMap.cs
- DeflateInput.cs
- ClientScriptManager.cs
- RestClientProxyHandler.cs
- ValueUtilsSmi.cs
- ClientSession.cs
- TableLayoutColumnStyleCollection.cs
- ScriptModule.cs
- BufferBuilder.cs
- Tokenizer.cs
- HttpListener.cs
- ColumnCollection.cs