Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / SchemaElementLookUpTable.cs / 1305376 / SchemaElementLookUpTable.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Data; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// Summary description for SchemaElementLookUpTable. /// internal sealed class SchemaElementLookUpTable: IEnumerable , ISchemaElementLookUpTable where T : SchemaElement { #region Instance Fields private Dictionary _keyToType = null; private List _keysInDefOrder = new List (); #endregion #region Public Methods /// /// /// public SchemaElementLookUpTable() { } ////// /// public int Count { get { return KeyToType.Count; } } ////// /// /// ///public bool ContainsKey(string key) { return KeyToType.ContainsKey(KeyFromName(key)); } /// /// /// /// ///public T LookUpEquivalentKey(string key) { key = KeyFromName(key); T element; if (KeyToType.TryGetValue(key, out element)) { return element; } return null; } /// /// /// public T this[string key] { get { return KeyToType[KeyFromName(key)]; } } ////// /// public T GetElementAt(int index) { return KeyToType[_keysInDefOrder[index]]; } ////// /// ///public IEnumerator GetEnumerator() { return new SchemaElementLookUpTableEnumerator (KeyToType,_keysInDefOrder); } IEnumerator System.Collections.IEnumerable.GetEnumerator() { return new SchemaElementLookUpTableEnumerator (KeyToType,_keysInDefOrder); } /// /// /// ///public IEnumerator GetFilteredEnumerator() where S : T { return new SchemaElementLookUpTableEnumerator(KeyToType,_keysInDefOrder); } ////// Add the given type to the schema look up table. If there is an error, it /// adds the error and returns false. otherwise, it adds the type to the lookuptable /// and returns true /// public AddErrorKind TryAdd(T type) { Debug.Assert(type != null, "type parameter is null"); if (String.IsNullOrEmpty(type.Identity)) { return AddErrorKind.MissingNameError; } string key = KeyFromElement(type); T element; if (KeyToType.TryGetValue(key, out element)) { return AddErrorKind.DuplicateNameError; } KeyToType.Add(key,type); _keysInDefOrder.Add(key); return AddErrorKind.Succeeded; } public void Add(T type, bool doNotAddErrorForEmptyName, Func
Link Menu
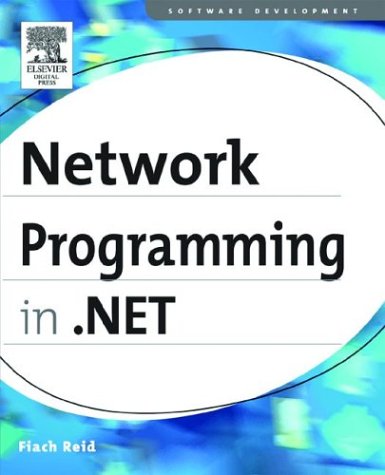
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OdbcConnectionFactory.cs
- LogStream.cs
- WebServiceTypeData.cs
- formatter.cs
- AxParameterData.cs
- PermissionSetTriple.cs
- Icon.cs
- TextDecoration.cs
- ParallelTimeline.cs
- StatusBarAutomationPeer.cs
- DataListItemEventArgs.cs
- PartialTrustVisibleAssembly.cs
- TypeUsage.cs
- AppDomainFactory.cs
- GPRECT.cs
- ModuleBuilder.cs
- CqlBlock.cs
- AutomationEvent.cs
- ListViewUpdatedEventArgs.cs
- AppDomainUnloadedException.cs
- XsdCachingReader.cs
- CustomError.cs
- CompiledQuery.cs
- SuppressedPackageProperties.cs
- XmlBaseWriter.cs
- DataGridViewToolTip.cs
- InvalidateEvent.cs
- Message.cs
- BinaryHeap.cs
- DesignerHelpers.cs
- ObjectTypeMapping.cs
- wgx_sdk_version.cs
- Model3DGroup.cs
- ObjectSet.cs
- InternalReceiveMessage.cs
- ComNativeDescriptor.cs
- ConfigXmlReader.cs
- SimpleBitVector32.cs
- XPathConvert.cs
- RootAction.cs
- WebUtil.cs
- ToolStripMenuItem.cs
- MatrixCamera.cs
- InternalTypeHelper.cs
- TableLayoutSettingsTypeConverter.cs
- EntityClientCacheKey.cs
- HtmlTable.cs
- SqlProvider.cs
- CultureInfoConverter.cs
- filewebresponse.cs
- CommonXSendMessage.cs
- BaseUriHelper.cs
- GroupBoxAutomationPeer.cs
- Listbox.cs
- Roles.cs
- WindowsFormsDesignerOptionService.cs
- ByteStack.cs
- ErrorHandler.cs
- ContentPosition.cs
- TextDecorationCollection.cs
- Executor.cs
- DynamicControl.cs
- DrawItemEvent.cs
- ManagedFilter.cs
- InstanceNormalEvent.cs
- ConfigurationManagerInternalFactory.cs
- LiteralDesigner.cs
- clipboard.cs
- XmlElementList.cs
- FixUpCollection.cs
- AuthenticationSection.cs
- PbrsForward.cs
- DataTemplateSelector.cs
- ImageMap.cs
- MailWriter.cs
- TextServicesDisplayAttribute.cs
- DiscriminatorMap.cs
- AlphaSortedEnumConverter.cs
- PrintEvent.cs
- Update.cs
- MediaElement.cs
- MgmtResManager.cs
- ColorConvertedBitmap.cs
- MenuItemCollection.cs
- Binding.cs
- ApplicationContext.cs
- XmlKeywords.cs
- Debug.cs
- FontDriver.cs
- WindowsBrush.cs
- MenuItemStyleCollection.cs
- NameTable.cs
- ElementNotAvailableException.cs
- Int64AnimationBase.cs
- LassoHelper.cs
- SpeechRecognitionEngine.cs
- EditorZone.cs
- RepeaterCommandEventArgs.cs
- InputLangChangeEvent.cs
- SetUserPreferenceRequest.cs