Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CompMod / System / ComponentModel / CultureInfoConverter.cs / 1 / CultureInfoConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System.Collections; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.InteropServices; using System.Threading; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] public class CultureInfoConverter : TypeConverter { private StandardValuesCollection values; ///Provides a type converter to convert ////// objects to and from various other representations. /// Retrieves the "default" name for our culture. /// private string DefaultCultureString { get { return SR.GetString(SR.CultureInfoConverterDefaultCultureString); } } ////// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Gets a value indicating whether this converter can /// convert an object in the given source type to a System.Globalization.CultureInfo /// object using /// the specified context. /// ////// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) { string text = (string)value; CultureInfo retVal = null; CultureInfo currentUICulture = Thread.CurrentThread.CurrentUICulture; if (culture != null && culture.Equals(CultureInfo.InvariantCulture)) { Thread.CurrentThread.CurrentUICulture = culture; } try { // Look for the default culture info. // if (text == null || text.Length == 0 || string.Compare(text, DefaultCultureString, StringComparison.Ordinal) == 0) { retVal = CultureInfo.InvariantCulture; } // Now look in our set of installed cultures. // if (retVal == null) { ICollection values = GetStandardValues(context); IEnumerator e = values.GetEnumerator(); while (e.MoveNext()) { CultureInfo info = (CultureInfo)e.Current; if (info != null && string.Compare(info.DisplayName, text, StringComparison.Ordinal) == 0) { retVal = info; break; } } } // Now try to create a new culture info from this value // if (retVal == null) { try { retVal = new CultureInfo(text); } catch {} } // Finally, try to find a partial match // if (retVal == null) { text = text.ToLower(CultureInfo.CurrentCulture); IEnumerator e = values.GetEnumerator(); while (e.MoveNext()) { CultureInfo info = (CultureInfo)e.Current; if (info != null && info.DisplayName.ToLower(CultureInfo.CurrentCulture).StartsWith(text)) { retVal = info; break; } } } } finally { Thread.CurrentThread.CurrentUICulture = currentUICulture; } // No good. We can't support it. // if (retVal == null) { throw new ArgumentException(SR.GetString(SR.CultureInfoConverterInvalidCulture, (string)value)); } return retVal; } return base.ConvertFrom(context, culture, value); } ////// Converts the specified value object to a ////// object. /// /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(string)) { string retVal; CultureInfo currentUICulture = Thread.CurrentThread.CurrentUICulture; if (culture != null && culture.Equals(CultureInfo.InvariantCulture)) { Thread.CurrentThread.CurrentUICulture = culture; } try { if (value == null || value == CultureInfo.InvariantCulture) { retVal = DefaultCultureString; } else { retVal = ((CultureInfo)value).DisplayName; } } finally { Thread.CurrentThread.CurrentUICulture = currentUICulture; } return retVal; } if (destinationType == typeof(InstanceDescriptor) && value is CultureInfo) { CultureInfo c = (CultureInfo) value; ConstructorInfo ctor = typeof(CultureInfo).GetConstructor(new Type[] {typeof(string)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] {c.Name}); } } return base.ConvertTo(context, culture, value, destinationType); } ////// Converts the given /// value object to the /// specified destination type. /// ////// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (values == null) { CultureInfo[] installedCultures = CultureInfo.GetCultures(CultureTypes.SpecificCultures | CultureTypes.NeutralCultures); // Add Culture.InvariantCulture for a default culture, then sort the array. CultureInfo[] array = new CultureInfo[installedCultures.Length + 1]; Array.Copy(installedCultures, array, installedCultures.Length); Array.Sort(array, new CultureComparer()); Debug.Assert(array[0] == null); if (array[0] == null) { //we replace null with the real default culture because there are code paths // where the propgrid will send values from this returned array directly -- instead // of converting it to a string and then back to a value (which this relied on). array[0] = CultureInfo.InvariantCulture; //null isn't the value here -- invariantculture is. } values = new StandardValuesCollection(array); } return values; } ////// Gets a collection of standard values collection for a System.Globalization.CultureInfo /// object using the specified context. /// ////// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } ////// Gets a value indicating whether the list of standard values returned from /// System.ComponentModel.CultureInfoConverter.GetStandardValues is an exclusive list. /// ////// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } ////// Gets a value indicating whether this object supports a /// standard set of values that can be picked from a list using the specified /// context. /// ////// IComparer object used for sorting CultureInfos /// WARNING: If you change where null is positioned, then you must fix CultureConverter.GetStandardValues! /// private class CultureComparer : IComparer { public int Compare(object item1, object item2) { if (item1 == null) { // If both are null, then they are equal // if (item2 == null) { return 0; } // Otherwise, item1 is null, but item2 is valid (greater) // return -1; } if (item2 == null) { // item2 is null, so item 1 is greater // return 1; } String itemName1 = ((CultureInfo)item1).DisplayName; String itemName2 = ((CultureInfo)item2).DisplayName; CompareInfo compInfo = (CultureInfo.CurrentCulture).CompareInfo; return compInfo.Compare(itemName1, itemName2, CompareOptions.StringSort); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System.Collections; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.InteropServices; using System.Threading; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] public class CultureInfoConverter : TypeConverter { private StandardValuesCollection values; ///Provides a type converter to convert ////// objects to and from various other representations. /// Retrieves the "default" name for our culture. /// private string DefaultCultureString { get { return SR.GetString(SR.CultureInfoConverterDefaultCultureString); } } ////// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Gets a value indicating whether this converter can /// convert an object in the given source type to a System.Globalization.CultureInfo /// object using /// the specified context. /// ////// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) { string text = (string)value; CultureInfo retVal = null; CultureInfo currentUICulture = Thread.CurrentThread.CurrentUICulture; if (culture != null && culture.Equals(CultureInfo.InvariantCulture)) { Thread.CurrentThread.CurrentUICulture = culture; } try { // Look for the default culture info. // if (text == null || text.Length == 0 || string.Compare(text, DefaultCultureString, StringComparison.Ordinal) == 0) { retVal = CultureInfo.InvariantCulture; } // Now look in our set of installed cultures. // if (retVal == null) { ICollection values = GetStandardValues(context); IEnumerator e = values.GetEnumerator(); while (e.MoveNext()) { CultureInfo info = (CultureInfo)e.Current; if (info != null && string.Compare(info.DisplayName, text, StringComparison.Ordinal) == 0) { retVal = info; break; } } } // Now try to create a new culture info from this value // if (retVal == null) { try { retVal = new CultureInfo(text); } catch {} } // Finally, try to find a partial match // if (retVal == null) { text = text.ToLower(CultureInfo.CurrentCulture); IEnumerator e = values.GetEnumerator(); while (e.MoveNext()) { CultureInfo info = (CultureInfo)e.Current; if (info != null && info.DisplayName.ToLower(CultureInfo.CurrentCulture).StartsWith(text)) { retVal = info; break; } } } } finally { Thread.CurrentThread.CurrentUICulture = currentUICulture; } // No good. We can't support it. // if (retVal == null) { throw new ArgumentException(SR.GetString(SR.CultureInfoConverterInvalidCulture, (string)value)); } return retVal; } return base.ConvertFrom(context, culture, value); } ////// Converts the specified value object to a ////// object. /// /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(string)) { string retVal; CultureInfo currentUICulture = Thread.CurrentThread.CurrentUICulture; if (culture != null && culture.Equals(CultureInfo.InvariantCulture)) { Thread.CurrentThread.CurrentUICulture = culture; } try { if (value == null || value == CultureInfo.InvariantCulture) { retVal = DefaultCultureString; } else { retVal = ((CultureInfo)value).DisplayName; } } finally { Thread.CurrentThread.CurrentUICulture = currentUICulture; } return retVal; } if (destinationType == typeof(InstanceDescriptor) && value is CultureInfo) { CultureInfo c = (CultureInfo) value; ConstructorInfo ctor = typeof(CultureInfo).GetConstructor(new Type[] {typeof(string)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] {c.Name}); } } return base.ConvertTo(context, culture, value, destinationType); } ////// Converts the given /// value object to the /// specified destination type. /// ////// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (values == null) { CultureInfo[] installedCultures = CultureInfo.GetCultures(CultureTypes.SpecificCultures | CultureTypes.NeutralCultures); // Add Culture.InvariantCulture for a default culture, then sort the array. CultureInfo[] array = new CultureInfo[installedCultures.Length + 1]; Array.Copy(installedCultures, array, installedCultures.Length); Array.Sort(array, new CultureComparer()); Debug.Assert(array[0] == null); if (array[0] == null) { //we replace null with the real default culture because there are code paths // where the propgrid will send values from this returned array directly -- instead // of converting it to a string and then back to a value (which this relied on). array[0] = CultureInfo.InvariantCulture; //null isn't the value here -- invariantculture is. } values = new StandardValuesCollection(array); } return values; } ////// Gets a collection of standard values collection for a System.Globalization.CultureInfo /// object using the specified context. /// ////// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } ////// Gets a value indicating whether the list of standard values returned from /// System.ComponentModel.CultureInfoConverter.GetStandardValues is an exclusive list. /// ////// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } ////// Gets a value indicating whether this object supports a /// standard set of values that can be picked from a list using the specified /// context. /// ////// IComparer object used for sorting CultureInfos /// WARNING: If you change where null is positioned, then you must fix CultureConverter.GetStandardValues! /// private class CultureComparer : IComparer { public int Compare(object item1, object item2) { if (item1 == null) { // If both are null, then they are equal // if (item2 == null) { return 0; } // Otherwise, item1 is null, but item2 is valid (greater) // return -1; } if (item2 == null) { // item2 is null, so item 1 is greater // return 1; } String itemName1 = ((CultureInfo)item1).DisplayName; String itemName2 = ((CultureInfo)item2).DisplayName; CompareInfo compInfo = (CultureInfo.CurrentCulture).CompareInfo; return compInfo.Compare(itemName1, itemName2, CompareOptions.StringSort); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
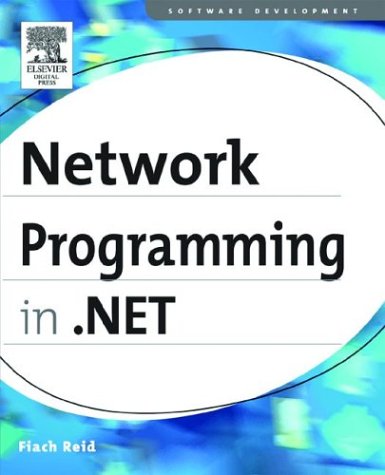
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectView.cs
- EmptyReadOnlyDictionaryInternal.cs
- SystemIPv4InterfaceProperties.cs
- LinkLabelLinkClickedEvent.cs
- FunctionDetailsReader.cs
- UserNameSecurityTokenProvider.cs
- CustomAttributeBuilder.cs
- XmlIlTypeHelper.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- MatrixCamera.cs
- ExpressionNode.cs
- Assert.cs
- AttributeEmitter.cs
- OdbcReferenceCollection.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- DoubleLink.cs
- StateInitializationDesigner.cs
- XmlReader.cs
- ToolbarAUtomationPeer.cs
- UnauthorizedWebPart.cs
- MsmqUri.cs
- MetadataItemEmitter.cs
- DebugView.cs
- SecurityManager.cs
- PinnedBufferMemoryStream.cs
- IfJoinedCondition.cs
- SqlCacheDependencySection.cs
- RadioButton.cs
- ZipFileInfoCollection.cs
- GeneralTransform3DGroup.cs
- GridProviderWrapper.cs
- CheckableControlBaseAdapter.cs
- ObjectKeyFrameCollection.cs
- DescriptionAttribute.cs
- ThousandthOfEmRealPoints.cs
- WMICapabilities.cs
- TypedElement.cs
- CheckPair.cs
- ZipIOLocalFileBlock.cs
- ServiceThrottlingBehavior.cs
- DrawingCollection.cs
- CompilerLocalReference.cs
- HtmlInputFile.cs
- Executor.cs
- Evidence.cs
- ZipPackage.cs
- ErrorTableItemStyle.cs
- Icon.cs
- SpeechSeg.cs
- PackageFilter.cs
- Message.cs
- TraceUtils.cs
- DbDataAdapter.cs
- SchemaType.cs
- RuleInfoComparer.cs
- WebPartAuthorizationEventArgs.cs
- XmlSchemaIdentityConstraint.cs
- DSASignatureDeformatter.cs
- DefaultMemberAttribute.cs
- ObjectPropertyMapping.cs
- OdbcConnectionFactory.cs
- ImmutableObjectAttribute.cs
- ModulesEntry.cs
- UInt32Storage.cs
- RequestContextBase.cs
- CompiledRegexRunnerFactory.cs
- StoreItemCollection.Loader.cs
- BinarySecretSecurityToken.cs
- TreeNodeCollectionEditor.cs
- ClusterSafeNativeMethods.cs
- _SslSessionsCache.cs
- SettingsPropertyValueCollection.cs
- PropertyFilterAttribute.cs
- MarkupCompiler.cs
- ComplexPropertyEntry.cs
- Win32.cs
- CorrelationToken.cs
- documentsequencetextpointer.cs
- TemplateField.cs
- ping.cs
- ClipboardData.cs
- CallContext.cs
- DistributedTransactionPermission.cs
- HyperLink.cs
- TextBreakpoint.cs
- XMLSyntaxException.cs
- DataServices.cs
- PeerReferralPolicy.cs
- RecognizerInfo.cs
- PanelDesigner.cs
- NonParentingControl.cs
- AsyncResult.cs
- SecurityPermission.cs
- OutputCacheSection.cs
- IIS7WorkerRequest.cs
- InstancePersistenceContext.cs
- FixedHighlight.cs
- CqlBlock.cs
- DictionaryBase.cs
- SimpleHandlerBuildProvider.cs