Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / RequestContextBase.cs / 2 / RequestContextBase.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System; using System.ServiceModel; using System.IO; using System.Net; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.Threading; using System.Web; using System.Diagnostics; using System.ServiceModel.Diagnostics; abstract class RequestContextBase : RequestContext { TimeSpan defaultSendTimeout; TimeSpan defaultCloseTimeout; CommunicationState state = CommunicationState.Opened; Message requestMessage; Exception requestMessageException; bool replySent; bool replyInitiated; bool aborted; object thisLock = new object(); protected RequestContextBase(Message requestMessage, TimeSpan defaultCloseTimeout, TimeSpan defaultSendTimeout) { this.defaultSendTimeout = defaultSendTimeout; this.defaultCloseTimeout = defaultCloseTimeout; this.requestMessage = requestMessage; } public override Message RequestMessage { get { if (this.requestMessageException != null) { #pragma warning suppress 56503 // [....], see outcome of DCR 50092 throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(this.requestMessageException); } return requestMessage; } } protected void SetRequestMessage(Message requestMessage) { DiagnosticUtility.DebugAssert(this.requestMessageException == null, "Cannot have both a requestMessage and a requestException."); this.requestMessage = requestMessage; } protected void SetRequestMessage(Exception requestMessageException) { DiagnosticUtility.DebugAssert(this.requestMessage == null, "Cannot have both a requestMessage and a requestException."); this.requestMessageException = requestMessageException; } protected bool ReplyInitiated { get { return this.replyInitiated; } } protected object ThisLock { get { return thisLock; } } public bool Aborted { get { return this.aborted; } } public TimeSpan DefaultCloseTimeout { get { return this.defaultCloseTimeout; } } public TimeSpan DefaultSendTimeout { get { return this.defaultSendTimeout; } } public override void Abort() { lock (ThisLock) { if (state == CommunicationState.Closed) return; state = CommunicationState.Closing; this.aborted = true; } if (DiagnosticUtility.ShouldTraceWarning) { TraceUtility.TraceEvent(TraceEventType.Warning, TraceCode.RequestContextAbort, this); } try { this.OnAbort(); } finally { state = CommunicationState.Closed; } } public override void Close() { this.Close(this.defaultCloseTimeout); } public override void Close(TimeSpan timeout) { if (timeout < TimeSpan.Zero) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("timeout", timeout, SR.GetString(SR.ValueMustBeNonNegative))); } bool sendAck = false; lock (ThisLock) { if (state != CommunicationState.Opened) return; state = CommunicationState.Closing; if (!this.replyInitiated) { this.replyInitiated = true; sendAck = true; } } TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); bool throwing = true; try { if (sendAck) { OnReply(null, timeoutHelper.RemainingTime()); } OnClose(timeoutHelper.RemainingTime()); state = CommunicationState.Closed; throwing = false; } finally { if (throwing) this.Abort(); } } protected override void Dispose(bool disposing) { base.Dispose(disposing); if (!disposing) return; if (this.replySent) { this.Close(); } else { this.Abort(); } } protected abstract void OnAbort(); protected abstract void OnClose(TimeSpan timeout); protected abstract void OnReply(Message message, TimeSpan timeout); protected abstract IAsyncResult OnBeginReply(Message message, TimeSpan timeout, AsyncCallback callback, object state); protected abstract void OnEndReply(IAsyncResult result); protected void ThrowIfInvalidReply() { if (state == CommunicationState.Closed || state == CommunicationState.Closing) { if (aborted) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new CommunicationObjectAbortedException(SR.GetString(SR.RequestContextAborted))); else throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ObjectDisposedException(this.GetType().FullName)); } if (this.replyInitiated) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ReplyAlreadySent))); } public override IAsyncResult BeginReply(Message message, AsyncCallback callback, object state) { return this.BeginReply(message, this.defaultSendTimeout, callback, state); } public override IAsyncResult BeginReply(Message message, TimeSpan timeout, AsyncCallback callback, object state) { // "null" is a valid reply (signals a 202-style "ack"), so we don't have a null-check here lock (this.thisLock) { this.ThrowIfInvalidReply(); this.replyInitiated = true; } return OnBeginReply(message, timeout, callback, state); } public override void EndReply(IAsyncResult result) { OnEndReply(result); this.replySent = true; } public override void Reply(Message message) { this.Reply(message, this.defaultSendTimeout); } public override void Reply(Message message, TimeSpan timeout) { // "null" is a valid reply (signals a 202-style "ack"), so we don't have a null-check here lock (this.thisLock) { this.ThrowIfInvalidReply(); this.replyInitiated = true; } this.OnReply(message, timeout); this.replySent = true; } internal Message InternalRequestMessage { get { return requestMessage; } } } class RequestContextMessageProperty : IDisposable { RequestContext context; object thisLock = new object(); public RequestContextMessageProperty(RequestContext context) { this.context = context; } public static string Name { get { return "requestContext"; } } void IDisposable.Dispose() { bool success = false; RequestContext thisContext; lock (this.thisLock) { if (this.context == null) return; thisContext = this.context; this.context = null; } try { thisContext.Close(); success = true; } catch (CommunicationException e) { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Information); } } catch (TimeoutException e) { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Information); } } finally { if (!success) { thisContext.Abort(); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
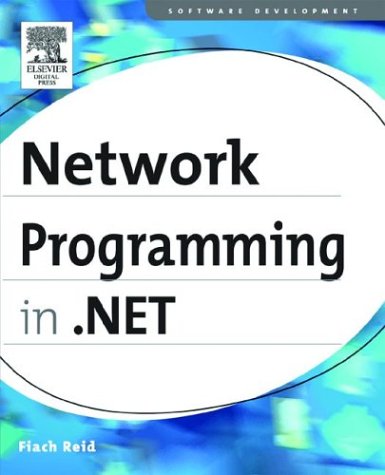
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SortExpressionBuilder.cs
- EventMetadata.cs
- DictionaryBase.cs
- OleServicesContext.cs
- IntPtr.cs
- ReadOnlyCollectionBase.cs
- DataGridViewColumnCollection.cs
- SettingsAttributes.cs
- TextChange.cs
- KeyInfo.cs
- ClientSideProviderDescription.cs
- BufferedGraphicsManager.cs
- EntityContainer.cs
- CallSite.cs
- BufferAllocator.cs
- DataGridAutoGeneratingColumnEventArgs.cs
- OletxTransactionManager.cs
- Variable.cs
- Odbc32.cs
- RegistryConfigurationProvider.cs
- SqlTypeSystemProvider.cs
- GradientBrush.cs
- StreamedFramingRequestChannel.cs
- DictationGrammar.cs
- PersonalizableAttribute.cs
- CodeThrowExceptionStatement.cs
- TextParagraphCache.cs
- Empty.cs
- AbstractSvcMapFileLoader.cs
- ImageCodecInfoPrivate.cs
- WriteableBitmap.cs
- HtmlControl.cs
- RootCodeDomSerializer.cs
- FormsIdentity.cs
- SafeLibraryHandle.cs
- TableAdapterManagerGenerator.cs
- StatusCommandUI.cs
- IntegrationExceptionEventArgs.cs
- FacetChecker.cs
- ExpressionConverter.cs
- XmlDataSourceView.cs
- LayoutEvent.cs
- RuntimeConfig.cs
- BatchWriter.cs
- HtmlInputButton.cs
- TextRunProperties.cs
- _DigestClient.cs
- XslException.cs
- RuntimeCompatibilityAttribute.cs
- XsltQilFactory.cs
- ParsedAttributeCollection.cs
- Command.cs
- DataGridSortCommandEventArgs.cs
- RemoteWebConfigurationHostServer.cs
- DeferredRunTextReference.cs
- DetailsViewPagerRow.cs
- Encoding.cs
- QilStrConcat.cs
- BasePattern.cs
- ParameterCollection.cs
- VisualBasicSettingsHandler.cs
- MILUtilities.cs
- SqlDataSourceCustomCommandEditor.cs
- RowVisual.cs
- TimeSpanOrInfiniteValidator.cs
- EventData.cs
- TextBoxAutomationPeer.cs
- WindowsHyperlink.cs
- StoreConnection.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- KnownColorTable.cs
- XmlElementList.cs
- ToolStripButton.cs
- StrongNamePublicKeyBlob.cs
- DSASignatureDeformatter.cs
- Throw.cs
- MemoryFailPoint.cs
- TextDecorationCollectionConverter.cs
- BinaryUtilClasses.cs
- oledbmetadatacolumnnames.cs
- BooleanExpr.cs
- SizeChangedEventArgs.cs
- WebPartActionVerb.cs
- Brush.cs
- IntegerValidatorAttribute.cs
- KeyValuePair.cs
- CatalogZone.cs
- AuthenticatedStream.cs
- RecognizerInfo.cs
- InternalDispatchObject.cs
- LateBoundBitmapDecoder.cs
- HttpHandlersSection.cs
- RemotingException.cs
- RepeatButton.cs
- MaskedTextBox.cs
- DataFormats.cs
- PackageRelationshipSelector.cs
- MenuBindingsEditor.cs
- ColumnMapTranslator.cs
- SchemaType.cs