Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Diagnostics / Eventing / Reader / EventMetadata.cs / 1305376 / EventMetadata.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: EventMetadata ** ** Purpose: ** This public class describes the metadata for a specific event ** raised by Provider. An instance of this class is obtained from ** ProviderMetadata class. ** ============================================================*/ using System.Globalization; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Text; using System.Diagnostics.CodeAnalysis; namespace System.Diagnostics.Eventing.Reader { ////// Event Metadata /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class EventMetadata { private long id; private byte version; private byte channelId; private byte level; private short opcode; private int task; private long keywords; private string template; private string description; ProviderMetadata pmReference; internal EventMetadata(uint id, byte version, byte channelId, byte level, byte opcode, short task, long keywords, string template, string description, ProviderMetadata pmReference) { this.id = id; this.version = version; this.channelId = channelId; this.level = level; this.opcode = opcode; this.task = task; this.keywords = keywords; this.template = template; this.description = description; this.pmReference = pmReference; } // // Max value will be UINT32.MaxValue - it is a long because this property // is really a UINT32. The legacy API allows event message ids to be declared // as UINT32 and these event/messages may be migrated into a Provider's // manifest as UINT32. Note that EventRecord ids are // still declared as int, because those ids max value is UINT16.MaxValue // and rest of the bits of the legacy event id would be stored in // Qualifiers property. // public long Id { get { return this.id; } } public byte Version { get { return this.version; } } public EventLogLink LogLink { get { return new EventLogLink((uint)this.channelId, this.pmReference); } } public EventLevel Level { get { return new EventLevel(this.level, this.pmReference); } } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", MessageId = "Opcode", Justification = "[....]: Shipped public in 3.5, breaking change to fix now.")] public EventOpcode Opcode { get { return new EventOpcode(this.opcode, this.pmReference); } } public EventTask Task { get { return new EventTask(this.task, this.pmReference); } } public IEnumerableKeywords { get { List list = new List (); ulong theKeywords = (ulong)this.keywords; ulong mask = 0x8000000000000000; //for every bit //for (int i = 0; i < 64 && theKeywords != 0; i++) for (int i = 0; i < 64; i++) { //if this bit is set if ((theKeywords & mask) > 0) { //the mask is the keyword we will be searching for. list.Add(new EventKeyword((long)mask, this.pmReference)); //theKeywords = theKeywords - mask; } //modify the mask to check next bit. mask = mask >> 1; } return list; } } public string Template { get { return this.template; } } public string Description { get { return this.description; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
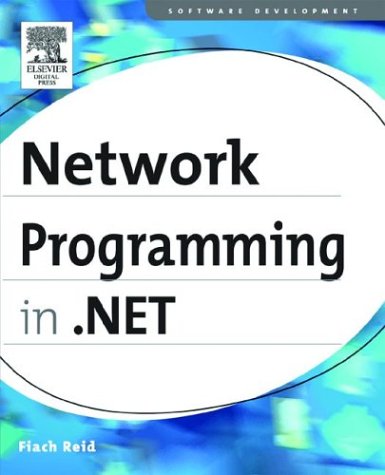
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ChangeTracker.cs
- CoTaskMemHandle.cs
- MethodCallConverter.cs
- MultipleViewProviderWrapper.cs
- GetMemberBinder.cs
- DesignerDataTableBase.cs
- TypefaceMetricsCache.cs
- BlockingCollection.cs
- XmlChoiceIdentifierAttribute.cs
- ISAPIWorkerRequest.cs
- Unit.cs
- ImageClickEventArgs.cs
- DataControlField.cs
- ProcessDesigner.cs
- DynamicRendererThreadManager.cs
- DataTableReaderListener.cs
- DesignBindingConverter.cs
- TakeQueryOptionExpression.cs
- X509Utils.cs
- Command.cs
- PolyBezierSegment.cs
- PathGeometry.cs
- RowCache.cs
- SqlNotificationEventArgs.cs
- PersonalizablePropertyEntry.cs
- ReachIDocumentPaginatorSerializerAsync.cs
- KeyedQueue.cs
- TrackingProfileSerializer.cs
- SortAction.cs
- TextBlock.cs
- BlurEffect.cs
- EntityCommandDefinition.cs
- Rect3D.cs
- DataGridViewCellParsingEventArgs.cs
- GeometryConverter.cs
- SignedPkcs7.cs
- ConfigXmlCDataSection.cs
- MailWriter.cs
- Ports.cs
- ViewStateException.cs
- Timer.cs
- XmlTextReaderImplHelpers.cs
- ShaperBuffers.cs
- SqlError.cs
- LazyTextWriterCreator.cs
- XhtmlTextWriter.cs
- SqlDataSourceCache.cs
- TextTreeInsertUndoUnit.cs
- WorkflowDurableInstance.cs
- StringCollection.cs
- InstanceKeyCompleteException.cs
- ParseNumbers.cs
- ServicesUtilities.cs
- ThicknessConverter.cs
- SectionRecord.cs
- XmlSerializerVersionAttribute.cs
- ValidationPropertyAttribute.cs
- CharacterString.cs
- SqlError.cs
- Formatter.cs
- ControlValuePropertyAttribute.cs
- TypeConverters.cs
- InvalidFilterCriteriaException.cs
- Bits.cs
- ECDsaCng.cs
- WebPartEditorApplyVerb.cs
- QuaternionAnimationUsingKeyFrames.cs
- OleDbRowUpdatingEvent.cs
- CaseStatementSlot.cs
- CursorEditor.cs
- TreeView.cs
- NavigatingCancelEventArgs.cs
- GenericPrincipal.cs
- parserscommon.cs
- MediaElementAutomationPeer.cs
- ImageListStreamer.cs
- WindowsComboBox.cs
- DesignerCategoryAttribute.cs
- PropertyValueChangedEvent.cs
- ValueType.cs
- DirectoryRootQuery.cs
- Executor.cs
- OletxTransactionFormatter.cs
- UndoManager.cs
- MasterPage.cs
- AttachedPropertiesService.cs
- CustomErrorsSection.cs
- DataServiceBuildProvider.cs
- Util.cs
- KernelTypeValidation.cs
- SafeBitVector32.cs
- FactoryMaker.cs
- ChangeConflicts.cs
- QueryCacheKey.cs
- Viewport3DAutomationPeer.cs
- ComplexTypeEmitter.cs
- InternalBufferManager.cs
- DotExpr.cs
- FileDetails.cs
- CorrelationService.cs