Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / InputOutput / Ports.cs / 2 / Ports.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file implements utility functions relating to messaging using System; using System.ServiceModel.Channels; using System.Diagnostics; using System.Globalization; using System.ServiceModel; using System.Security.Cryptography; using System.Text; using System.Xml; using Microsoft.Transactions.Bridge; using Microsoft.Transactions.Wsat.Messaging; using Microsoft.Transactions.Wsat.Protocol; using DiagnosticUtility = Microsoft.Transactions.Bridge.DiagnosticUtility; using ServiceModelDiagnosticUtility = System.ServiceModel.DiagnosticUtility; namespace Microsoft.Transactions.Wsat.InputOutput { static class Ports { public static Guid GetGuidFromTransactionId (string transactionId) { // E.g. "a01b8bc6781641c4b138c1acdcc940ef" const int minGuidLength = 32; int minTransactionIdLength = CoordinationContext.UuidScheme.Length + minGuidLength; if (transactionId.Length >= minTransactionIdLength && transactionId.StartsWith(CoordinationContext.UuidScheme, StringComparison.OrdinalIgnoreCase)) { Guid txIdGuid; if (ServiceModelDiagnosticUtility.Utility.TryCreateGuid( transactionId.Substring(CoordinationContext.UuidScheme.Length), out txIdGuid)) { return txIdGuid; } } // // Interop path // // Create a guid deterministically, using the transaction id as input // For obvious reasons, we need a 128-bit hash. // We can't use entropy because we want to be deterministic. Collisions are only fatal // if they occur often enough to coincide in a log. // // We do this to avoid deadlocks in RMs who might participant in the same // transaction through WS-AT and some other protocol that doesn't know about // the WS-AT specific id HashAlgorithm hash = SHA256.Create(); using (hash) { hash.Initialize(); byte[] buffer = Encoding.UTF8.GetBytes(transactionId); byte[] fullHash = hash.ComputeHash(buffer); // Grab the first 128 bits off the hash - see MB 40808 for more details byte[] hash128 = new byte[16]; Array.Copy(fullHash, hash128, hash128.Length); return new Guid(hash128); } } // // Addresses // public static string TryGetAddress(Proxy proxy) { if (proxy == null) { return "unknown"; } return proxy.To.Uri.AbsoluteUri; } public static string TryGetFromAddress(Message message) { string address; EndpointAddress replyTo = Library.GetReplyToHeader(message.Headers); if (replyTo != null) { address = replyTo.Uri.AbsoluteUri; if (address != null) { return address; } } return "unknown"; } public static bool TryGetEnlistment(Message message, out Guid enlistmentId, out ControlProtocol protocol) { enlistmentId = Guid.Empty; protocol = ControlProtocol.None; try { if (!EnlistmentHeader.ReadFrom(message, out enlistmentId, out protocol)) { return false; } } catch (InvalidEnlistmentHeaderException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); return false; } return true; } public static bool TryGetEnlistment(Message message, out Guid enlistmentId) { ControlProtocol dontCare; return TryGetEnlistment(message, out enlistmentId, out dontCare); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
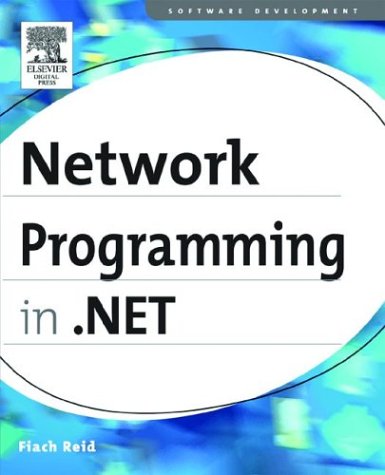
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlWriterTraceListener.cs
- ApplicationContext.cs
- CompilerScopeManager.cs
- WindowsListViewItemStartMenu.cs
- XmlNodeChangedEventManager.cs
- RegionIterator.cs
- HandlerMappingMemo.cs
- thaishape.cs
- DetailsViewAutoFormat.cs
- AccessorTable.cs
- ThreadAbortException.cs
- TreeNodeStyle.cs
- X509Utils.cs
- DelimitedListTraceListener.cs
- EncodingInfo.cs
- ImageInfo.cs
- DataControlField.cs
- XamlBrushSerializer.cs
- PolyBezierSegment.cs
- ReflectionServiceProvider.cs
- ETagAttribute.cs
- FrameworkContentElement.cs
- NavigationProperty.cs
- WindowsTokenRoleProvider.cs
- QilReplaceVisitor.cs
- CancellationState.cs
- DynamicVirtualDiscoSearcher.cs
- Italic.cs
- CaseInsensitiveHashCodeProvider.cs
- formatstringdialog.cs
- SystemParameters.cs
- EmissiveMaterial.cs
- ToolStripDropDownItemDesigner.cs
- RSAOAEPKeyExchangeFormatter.cs
- SessionStateUtil.cs
- ValidationError.cs
- XamlPathDataSerializer.cs
- RectKeyFrameCollection.cs
- IISMapPath.cs
- WeakRefEnumerator.cs
- RandomDelaySendsAsyncResult.cs
- DesignerVerbCollection.cs
- GridViewRowPresenterBase.cs
- EntitySetBase.cs
- SqlCacheDependency.cs
- TreeNodeBinding.cs
- RadialGradientBrush.cs
- Animatable.cs
- ServiceSettingsResponseInfo.cs
- DesignRelationCollection.cs
- SmiEventSink_Default.cs
- TextSpanModifier.cs
- SoapAttributeOverrides.cs
- GeometryHitTestResult.cs
- ILGenerator.cs
- GestureRecognizer.cs
- _ProxyChain.cs
- ConfigXmlText.cs
- DoWorkEventArgs.cs
- WindowsProgressbar.cs
- TypeConvertions.cs
- Canvas.cs
- SqlTransaction.cs
- DocumentViewerConstants.cs
- SrgsRule.cs
- ModelTypeConverter.cs
- WindowsListViewItemStartMenu.cs
- StorageMappingFragment.cs
- CatalogPart.cs
- KnownBoxes.cs
- DoubleLinkListEnumerator.cs
- EntityDataSourceWrapperCollection.cs
- ResponseStream.cs
- Asn1IntegerConverter.cs
- GridViewColumn.cs
- SmiXetterAccessMap.cs
- XamlStyleSerializer.cs
- BooleanToSelectiveScrollingOrientationConverter.cs
- CqlWriter.cs
- WebPartDisplayMode.cs
- Effect.cs
- GacUtil.cs
- Substitution.cs
- TabPage.cs
- RequestChannel.cs
- SortExpressionBuilder.cs
- HyperLinkField.cs
- HandleExceptionArgs.cs
- ResourceContainer.cs
- TileBrush.cs
- SiteMapNode.cs
- SchemaNamespaceManager.cs
- HuffModule.cs
- BulletedListEventArgs.cs
- ReliabilityContractAttribute.cs
- XmlNodeChangedEventManager.cs
- WindowsAuthenticationModule.cs
- ValueTable.cs
- ClrProviderManifest.cs
- Icon.cs