Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / Facet.cs / 1305376 / Facet.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Text; using System.Diagnostics; using System.Data.Entity; using System.Globalization; namespace System.Data.Metadata.Edm { ////// Class for representing a Facet object /// This object is Immutable (not just set to readonly) and /// some parts of the system are depending on that behavior /// [DebuggerDisplay("{Name,nq}={Value}")] public sealed class Facet : MetadataItem { #region Constructors ////// The constructor for constructing a Facet object with the facet description and a value /// /// The object describing this facet /// The value of the facet ///Thrown if facetDescription argument is null private Facet(FacetDescription facetDescription, object value) :base(MetadataFlags.Readonly) { EntityUtil.GenericCheckArgumentNull(facetDescription, "facetDescription"); _facetDescription = facetDescription; _value = value; } ////// Creates a Facet instance with the specified value for the given /// facet description. /// /// The object describing this facet /// The value of the facet ///Thrown if facetDescription argument is null internal static Facet Create(FacetDescription facetDescription, object value) { return Create(facetDescription, value, false); } ////// Creates a Facet instance with the specified value for the given /// facet description. /// /// The object describing this facet /// The value of the facet /// true to bypass caching and known values; false otherwise. ///Thrown if facetDescription argument is null internal static Facet Create(FacetDescription facetDescription, object value, bool bypassKnownValues) { EntityUtil.CheckArgumentNull(facetDescription, "facetDescription"); if (!bypassKnownValues) { // Reuse facets with a null value. if (object.ReferenceEquals(value, null)) { return facetDescription.NullValueFacet; } // Reuse facets with a default value. if (object.Equals(facetDescription.DefaultValue, value)) { return facetDescription.DefaultValueFacet; } // Special case boolean facets. if (facetDescription.FacetType.Identity == "Edm.Boolean") { bool boolValue = (bool)value; return facetDescription.GetBooleanFacet(boolValue); } } Facet result = new Facet(facetDescription, value); // Check the type of the value only if we know what the correct CLR type is if (value != null && !Helper.IsUnboundedFacetValue(result) && result.FacetType.ClrType != null) { Type valueType = value.GetType(); Debug.Assert( valueType == result.FacetType.ClrType || result.FacetType.ClrType.IsAssignableFrom(valueType), string.Format(CultureInfo.CurrentCulture, "The facet {0} has type {1}, but a value of type {2} was supplied.", result.Name, result.FacetType.ClrType, valueType) ); } return result; } #endregion #region Fields ///The object describing this facet. private readonly FacetDescription _facetDescription; ///The value assigned to this facet. private readonly object _value; #endregion #region Properties ////// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.Facet; } } ////// Gets the description object for describing the facet /// public FacetDescription Description { get { return _facetDescription; } } ////// Gets/Sets the name of the facet /// [MetadataProperty(PrimitiveTypeKind.String, false)] public String Name { get { return _facetDescription.FacetName; } } ////// Gets/Sets the type of the facet /// [MetadataProperty(BuiltInTypeKind.EdmType, false)] public EdmType FacetType { get { return _facetDescription.FacetType; } } ////// Gets/Sets the value of the facet /// ///Thrown if the Facet instance is in ReadOnly state [MetadataProperty(typeof(Object), false)] public Object Value { get { return _value; } } ////// Gets the identity for this item as a string /// internal override string Identity { get { return _facetDescription.FacetName; } } ////// Indicates whether the value of the facet is unbounded /// public bool IsUnbounded { get { return object.ReferenceEquals(Value, EdmConstants.UnboundedValue); } } #endregion #region Methods ////// Overriding System.Object.ToString to provide better String representation /// for this type. /// public override string ToString() { return this.Name; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Text; using System.Diagnostics; using System.Data.Entity; using System.Globalization; namespace System.Data.Metadata.Edm { ////// Class for representing a Facet object /// This object is Immutable (not just set to readonly) and /// some parts of the system are depending on that behavior /// [DebuggerDisplay("{Name,nq}={Value}")] public sealed class Facet : MetadataItem { #region Constructors ////// The constructor for constructing a Facet object with the facet description and a value /// /// The object describing this facet /// The value of the facet ///Thrown if facetDescription argument is null private Facet(FacetDescription facetDescription, object value) :base(MetadataFlags.Readonly) { EntityUtil.GenericCheckArgumentNull(facetDescription, "facetDescription"); _facetDescription = facetDescription; _value = value; } ////// Creates a Facet instance with the specified value for the given /// facet description. /// /// The object describing this facet /// The value of the facet ///Thrown if facetDescription argument is null internal static Facet Create(FacetDescription facetDescription, object value) { return Create(facetDescription, value, false); } ////// Creates a Facet instance with the specified value for the given /// facet description. /// /// The object describing this facet /// The value of the facet /// true to bypass caching and known values; false otherwise. ///Thrown if facetDescription argument is null internal static Facet Create(FacetDescription facetDescription, object value, bool bypassKnownValues) { EntityUtil.CheckArgumentNull(facetDescription, "facetDescription"); if (!bypassKnownValues) { // Reuse facets with a null value. if (object.ReferenceEquals(value, null)) { return facetDescription.NullValueFacet; } // Reuse facets with a default value. if (object.Equals(facetDescription.DefaultValue, value)) { return facetDescription.DefaultValueFacet; } // Special case boolean facets. if (facetDescription.FacetType.Identity == "Edm.Boolean") { bool boolValue = (bool)value; return facetDescription.GetBooleanFacet(boolValue); } } Facet result = new Facet(facetDescription, value); // Check the type of the value only if we know what the correct CLR type is if (value != null && !Helper.IsUnboundedFacetValue(result) && result.FacetType.ClrType != null) { Type valueType = value.GetType(); Debug.Assert( valueType == result.FacetType.ClrType || result.FacetType.ClrType.IsAssignableFrom(valueType), string.Format(CultureInfo.CurrentCulture, "The facet {0} has type {1}, but a value of type {2} was supplied.", result.Name, result.FacetType.ClrType, valueType) ); } return result; } #endregion #region Fields ///The object describing this facet. private readonly FacetDescription _facetDescription; ///The value assigned to this facet. private readonly object _value; #endregion #region Properties ////// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.Facet; } } ////// Gets the description object for describing the facet /// public FacetDescription Description { get { return _facetDescription; } } ////// Gets/Sets the name of the facet /// [MetadataProperty(PrimitiveTypeKind.String, false)] public String Name { get { return _facetDescription.FacetName; } } ////// Gets/Sets the type of the facet /// [MetadataProperty(BuiltInTypeKind.EdmType, false)] public EdmType FacetType { get { return _facetDescription.FacetType; } } ////// Gets/Sets the value of the facet /// ///Thrown if the Facet instance is in ReadOnly state [MetadataProperty(typeof(Object), false)] public Object Value { get { return _value; } } ////// Gets the identity for this item as a string /// internal override string Identity { get { return _facetDescription.FacetName; } } ////// Indicates whether the value of the facet is unbounded /// public bool IsUnbounded { get { return object.ReferenceEquals(Value, EdmConstants.UnboundedValue); } } #endregion #region Methods ////// Overriding System.Object.ToString to provide better String representation /// for this type. /// public override string ToString() { return this.Name; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
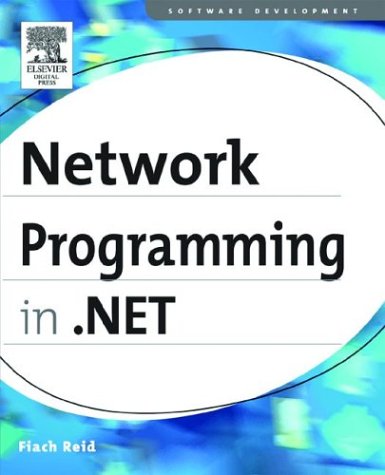
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ByteStreamGeometryContext.cs
- MembershipSection.cs
- NumberSubstitution.cs
- HandlerBase.cs
- QuadraticBezierSegment.cs
- ResizeGrip.cs
- cryptoapiTransform.cs
- ParserExtension.cs
- XhtmlTextWriter.cs
- AppDomainManager.cs
- FixedSOMFixedBlock.cs
- SqlFunctionAttribute.cs
- EventProviderBase.cs
- BitmapSource.cs
- ArcSegment.cs
- RelationshipEnd.cs
- InfocardClientCredentials.cs
- PropertyContainer.cs
- _HTTPDateParse.cs
- DesignerCategoryAttribute.cs
- ToolStripDropDownDesigner.cs
- Queue.cs
- LocatorPart.cs
- SettingsPropertyWrongTypeException.cs
- ColorDialog.cs
- SQLDateTime.cs
- ListViewItem.cs
- GeometryHitTestParameters.cs
- ReferenceEqualityComparer.cs
- Transform.cs
- NodeFunctions.cs
- NetworkInformationPermission.cs
- CompositeControl.cs
- _Semaphore.cs
- XDeferredAxisSource.cs
- HtmlTextBoxAdapter.cs
- BaseCAMarshaler.cs
- xmlglyphRunInfo.cs
- SqlConnectionPoolGroupProviderInfo.cs
- GZipObjectSerializer.cs
- EmptyControlCollection.cs
- KeyGesture.cs
- QualifiedId.cs
- DesignerDataSourceView.cs
- CompatibleComparer.cs
- SoapServerMethod.cs
- Vector.cs
- AlternateViewCollection.cs
- KeyMatchBuilder.cs
- BindingExpression.cs
- RuntimeHandles.cs
- KnownAssembliesSet.cs
- EntryPointNotFoundException.cs
- SqlConnectionFactory.cs
- IPEndPoint.cs
- IncrementalHitTester.cs
- PropertyManager.cs
- ImageMap.cs
- StrongNamePublicKeyBlob.cs
- NotImplementedException.cs
- ControlParameter.cs
- EpmAttributeNameBuilder.cs
- ErrorFormatterPage.cs
- IList.cs
- RichTextBoxAutomationPeer.cs
- PipeSecurity.cs
- ProviderUtil.cs
- CacheDependency.cs
- DeflateStreamAsyncResult.cs
- Reference.cs
- PixelFormatConverter.cs
- DataBindingList.cs
- ServiceReference.cs
- CatchDesigner.xaml.cs
- ImageButton.cs
- DynamicValidator.cs
- BamlLocalizabilityResolver.cs
- ListBindableAttribute.cs
- AnnotationResourceCollection.cs
- StyleXamlParser.cs
- TrackBar.cs
- ServiceContractDetailViewControl.cs
- FrameworkElement.cs
- SmiMetaData.cs
- AccessibilityHelperForXpWin2k3.cs
- SafeCryptHandles.cs
- HtmlShimManager.cs
- RowToParametersTransformer.cs
- EdmItemError.cs
- SqlLiftWhereClauses.cs
- IndentedWriter.cs
- SequentialUshortCollection.cs
- SystemMulticastIPAddressInformation.cs
- PropertySourceInfo.cs
- MethodBuilder.cs
- PolicyStatement.cs
- Context.cs
- AutoGeneratedFieldProperties.cs
- FormsAuthenticationModule.cs
- SystemMulticastIPAddressInformation.cs