Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / FilterableAttribute.cs / 1 / FilterableAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Web.UI { using System; using System.Collections; using System.ComponentModel; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AttributeUsage(AttributeTargets.Property | AttributeTargets.Class)] public sealed class FilterableAttribute : Attribute { ////// /// /// public static readonly FilterableAttribute Yes = new FilterableAttribute(true); ////// /// /// public static readonly FilterableAttribute No = new FilterableAttribute(false); ////// /// /// public static readonly FilterableAttribute Default = Yes; private bool _filterable = false; private static Hashtable _filterableTypes; static FilterableAttribute() { // Create a synchronized wrapper _filterableTypes = Hashtable.Synchronized(new Hashtable()); } ////// /// public FilterableAttribute(bool filterable) { _filterable = filterable; } ////// public bool Filterable { get { return _filterable; } } ///Indicates if the property is Filterable. ///public override bool Equals(object obj) { if (obj == this) { return true; } FilterableAttribute other = obj as FilterableAttribute; return (other != null) && (other.Filterable == _filterable); } /// public override int GetHashCode() { return _filterable.GetHashCode(); } /// public override bool IsDefaultAttribute() { return this.Equals(Default); } public static bool IsObjectFilterable(Object instance) { if (instance == null) throw new ArgumentNullException("instance"); return IsTypeFilterable(instance.GetType()); } public static bool IsPropertyFilterable(PropertyDescriptor propertyDescriptor) { FilterableAttribute filterableAttr = (FilterableAttribute)propertyDescriptor.Attributes[typeof(FilterableAttribute)]; if (filterableAttr != null) { return filterableAttr.Filterable; } return true; } public static bool IsTypeFilterable(Type type) { if (type == null) throw new ArgumentNullException("type"); object result = _filterableTypes[type]; if (result != null) { return (bool)result; } System.ComponentModel.AttributeCollection attrs = TypeDescriptor.GetAttributes(type); FilterableAttribute attr = (FilterableAttribute)attrs[typeof(FilterableAttribute)]; result = (attr != null) && attr.Filterable; _filterableTypes[type] = result; return (bool)result; } } }
Link Menu
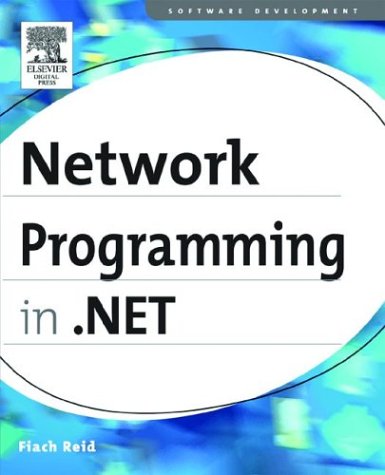
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _ConnectStream.cs
- PersistNameAttribute.cs
- ConnectionStringSettingsCollection.cs
- CodeMemberMethod.cs
- AccessDataSource.cs
- RunClient.cs
- CultureInfoConverter.cs
- SelectionEditingBehavior.cs
- SoundPlayer.cs
- DataViewListener.cs
- AppDomainShutdownMonitor.cs
- GroupBoxAutomationPeer.cs
- TrustLevel.cs
- EventsTab.cs
- FixedHyperLink.cs
- Encoding.cs
- RadioButtonFlatAdapter.cs
- DependencyPropertyKind.cs
- PathFigureCollection.cs
- TableLayoutSettings.cs
- CustomAttributeFormatException.cs
- METAHEADER.cs
- EventData.cs
- NetworkInformationException.cs
- TreeChangeInfo.cs
- ColumnBinding.cs
- XmlSchema.cs
- MulticastNotSupportedException.cs
- PolyLineSegment.cs
- PlatformCulture.cs
- DPAPIProtectedConfigurationProvider.cs
- TransactionException.cs
- ManualResetEvent.cs
- BinaryMethodMessage.cs
- FixedElement.cs
- TextTreeObjectNode.cs
- WindowClosedEventArgs.cs
- unsafeIndexingFilterStream.cs
- QilNode.cs
- AttributeAction.cs
- ModifiableIteratorCollection.cs
- BamlBinaryReader.cs
- CodeExporter.cs
- UtilityExtension.cs
- SelectionChangedEventArgs.cs
- CollectionViewSource.cs
- LocalizationParserHooks.cs
- InternalResources.cs
- ForEachAction.cs
- ElementHostPropertyMap.cs
- XmlIlVisitor.cs
- XmlReflectionMember.cs
- WebProxyScriptElement.cs
- WebPartEditorCancelVerb.cs
- NumericUpDownAcceleration.cs
- CommandBinding.cs
- Compiler.cs
- ElementUtil.cs
- SymLanguageVendor.cs
- AddInStore.cs
- __Error.cs
- MessageBox.cs
- InfoCardClaimCollection.cs
- DiscoveryVersionConverter.cs
- TableRow.cs
- TypeNameConverter.cs
- ToolStripDropDownDesigner.cs
- DiscoveryRequestHandler.cs
- RuleConditionDialog.Designer.cs
- DrawingContext.cs
- _SslStream.cs
- DataControlFieldTypeEditor.cs
- ActivityWithResult.cs
- ByteAnimation.cs
- XpsSerializationManagerAsync.cs
- MsmqInputChannelListenerBase.cs
- XmlQualifiedNameTest.cs
- ResourceSetExpression.cs
- COM2IPerPropertyBrowsingHandler.cs
- LayoutTableCell.cs
- TextElementEnumerator.cs
- EventLogEntryCollection.cs
- DesignerSerializationVisibilityAttribute.cs
- XmlSchemaImport.cs
- SerializableAttribute.cs
- EntityStoreSchemaGenerator.cs
- WebPartZoneCollection.cs
- DelimitedListTraceListener.cs
- TaskForm.cs
- unsafenativemethodsother.cs
- AssemblyBuilder.cs
- ConstantProjectedSlot.cs
- LocalFileSettingsProvider.cs
- CategoryNameCollection.cs
- MessageQueueKey.cs
- MaskInputRejectedEventArgs.cs
- CustomPopupPlacement.cs
- COM2Properties.cs
- EncodingTable.cs
- MultilineStringEditor.cs