Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / RelationshipEndMember.cs / 1 / RelationshipEndMember.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Data.Common; namespace System.Data.Metadata.Edm { ////// Initializes a new instance of the RelationshipEndMember class /// public abstract class RelationshipEndMember : EdmMember { #region Constructors ////// Initializes a new instance of RelationshipEndMember /// /// name of the relationship end member /// Ref type that this end refers to /// The multiplicity of this relationship end ///Thrown if name or endRefType arguments is null ///Thrown if name argument is empty string internal RelationshipEndMember(string name, RefType endRefType, RelationshipMultiplicity multiplicity) : base(name, TypeUsage.Create(endRefType, new FacetValues{ Nullable = false })) { _relationshipMultiplicity = multiplicity; _deleteBehavior = OperationAction.None; } #endregion #region Fields private OperationAction _deleteBehavior; private RelationshipMultiplicity _relationshipMultiplicity; #endregion #region Properties ////// Returns the operational behaviour for this end /// [MetadataProperty(BuiltInTypeKind.OperationAction, true)] public OperationAction DeleteBehavior { get { return _deleteBehavior; } internal set { Util.ThrowIfReadOnly(this); _deleteBehavior = value; } } ////// Returns the multiplicity for this relationship end /// [MetadataProperty(BuiltInTypeKind.RelationshipMultiplicity, false)] public RelationshipMultiplicity RelationshipMultiplicity { get { return _relationshipMultiplicity; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Data.Common; namespace System.Data.Metadata.Edm { ////// Initializes a new instance of the RelationshipEndMember class /// public abstract class RelationshipEndMember : EdmMember { #region Constructors ////// Initializes a new instance of RelationshipEndMember /// /// name of the relationship end member /// Ref type that this end refers to /// The multiplicity of this relationship end ///Thrown if name or endRefType arguments is null ///Thrown if name argument is empty string internal RelationshipEndMember(string name, RefType endRefType, RelationshipMultiplicity multiplicity) : base(name, TypeUsage.Create(endRefType, new FacetValues{ Nullable = false })) { _relationshipMultiplicity = multiplicity; _deleteBehavior = OperationAction.None; } #endregion #region Fields private OperationAction _deleteBehavior; private RelationshipMultiplicity _relationshipMultiplicity; #endregion #region Properties ////// Returns the operational behaviour for this end /// [MetadataProperty(BuiltInTypeKind.OperationAction, true)] public OperationAction DeleteBehavior { get { return _deleteBehavior; } internal set { Util.ThrowIfReadOnly(this); _deleteBehavior = value; } } ////// Returns the multiplicity for this relationship end /// [MetadataProperty(BuiltInTypeKind.RelationshipMultiplicity, false)] public RelationshipMultiplicity RelationshipMultiplicity { get { return _relationshipMultiplicity; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
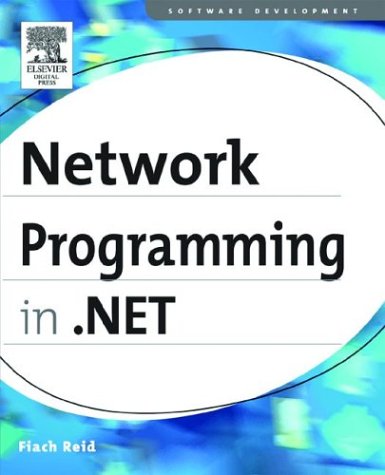
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XsdDateTime.cs
- AppearanceEditorPart.cs
- SingleBodyParameterMessageFormatter.cs
- WindowsGraphicsWrapper.cs
- WebPartZoneBase.cs
- LinqToSqlWrapper.cs
- ListViewGroupConverter.cs
- GB18030Encoding.cs
- ResourceDefaultValueAttribute.cs
- CustomSignedXml.cs
- StreamGeometry.cs
- SimpleApplicationHost.cs
- StylusPointCollection.cs
- CommentEmitter.cs
- XmlDocumentType.cs
- Misc.cs
- AnnotationObservableCollection.cs
- ControlUtil.cs
- ToolStripSplitStackLayout.cs
- WindowsToolbarAsMenu.cs
- QueryContinueDragEventArgs.cs
- TraceEventCache.cs
- Rule.cs
- BindingExpressionBase.cs
- HandlerMappingMemo.cs
- EqualityComparer.cs
- SequenceDesignerAccessibleObject.cs
- WebPartEventArgs.cs
- ListComponentEditorPage.cs
- Cell.cs
- RemoteHelper.cs
- BigInt.cs
- DialogResultConverter.cs
- DoubleAnimationUsingPath.cs
- LoginName.cs
- ImportCatalogPart.cs
- SqlPersistenceWorkflowInstanceDescription.cs
- StickyNoteHelper.cs
- DataGridColumnCollectionEditor.cs
- CategoryEditor.cs
- AssociativeAggregationOperator.cs
- UpdateCommand.cs
- Visual3D.cs
- FontSource.cs
- ScrollProperties.cs
- SqlNodeAnnotations.cs
- DataProviderNameConverter.cs
- WebPartCatalogAddVerb.cs
- WebServiceEndpoint.cs
- ApplicationActivator.cs
- ReferentialConstraint.cs
- WindowsAuthenticationEventArgs.cs
- ToolStripControlHost.cs
- WindowsListBox.cs
- FragmentQueryKB.cs
- TriggerCollection.cs
- SystemWebSectionGroup.cs
- Authorization.cs
- UrlMapping.cs
- BaseDataBoundControl.cs
- DataListItemEventArgs.cs
- RichTextBoxConstants.cs
- WebControlAdapter.cs
- XsltQilFactory.cs
- TrustManagerPromptUI.cs
- GPPOINT.cs
- ConfigurationStrings.cs
- AsymmetricSignatureFormatter.cs
- VideoDrawing.cs
- SiblingIterators.cs
- ImageCodecInfoPrivate.cs
- HtmlTableRow.cs
- DataGridViewDataErrorEventArgs.cs
- DSASignatureDeformatter.cs
- AmbientLight.cs
- MediaPlayer.cs
- ITreeGenerator.cs
- AuthorizationSection.cs
- SimpleExpression.cs
- CodeGeneratorOptions.cs
- XmlDataImplementation.cs
- DataReaderContainer.cs
- ConfigXmlComment.cs
- ComboBoxRenderer.cs
- AutoSizeComboBox.cs
- TypeSystem.cs
- OleDragDropHandler.cs
- MultiByteCodec.cs
- latinshape.cs
- ContentDefinition.cs
- FigureHelper.cs
- ListViewUpdatedEventArgs.cs
- FixedSOMSemanticBox.cs
- RichTextBoxConstants.cs
- PaperSource.cs
- TypeSystem.cs
- TraceUtility.cs
- XmlStringTable.cs
- WebPartCancelEventArgs.cs
- BitmapEffectGeneralTransform.cs