Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Description / WebServiceEndpoint.cs / 1305376 / WebServiceEndpoint.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Description { using System; using System.ServiceModel; using System.ServiceModel.Channels; using System.Text; using System.Xml; using System.ServiceModel.Web; public abstract class WebServiceEndpoint : ServiceEndpoint { internal WebServiceEndpoint(ContractDescription contract, EndpointAddress address) : base(contract, new WebHttpBinding(), address) { } public HostNameComparisonMode HostNameComparisonMode { get { return this.webHttpBinding.HostNameComparisonMode; } set { this.webHttpBinding.HostNameComparisonMode = value; } } public long MaxBufferPoolSize { get { return this.webHttpBinding.MaxBufferPoolSize; } set { this.webHttpBinding.MaxBufferPoolSize = value; } } public int MaxBufferSize { get { return this.webHttpBinding.MaxBufferSize; } set { this.webHttpBinding.MaxBufferSize = value; } } public long MaxReceivedMessageSize { get { return this.webHttpBinding.MaxReceivedMessageSize; } set { this.webHttpBinding.MaxReceivedMessageSize = value; } } public TransferMode TransferMode { get { return this.webHttpBinding.TransferMode; } set { this.webHttpBinding.TransferMode = value; } } public XmlDictionaryReaderQuotas ReaderQuotas { get { return this.webHttpBinding.ReaderQuotas; } set { this.webHttpBinding.ReaderQuotas = value; } } public WebHttpSecurity Security { get { return this.webHttpBinding.Security; } } public Encoding WriteEncoding { get { return this.webHttpBinding.WriteEncoding; } set { this.webHttpBinding.WriteEncoding = value; } } public WebContentTypeMapper ContentTypeMapper { get { return this.webHttpBinding.ContentTypeMapper; } set { this.webHttpBinding.ContentTypeMapper = value; } } public bool CrossDomainScriptAccessEnabled { get { return this.webHttpBinding.CrossDomainScriptAccessEnabled; } set { this.webHttpBinding.CrossDomainScriptAccessEnabled = value; } } protected abstract Type WebEndpointType { get; } WebHttpBinding webHttpBinding { get { WebHttpBinding webHttpBinding = this.Binding as WebHttpBinding; if (webHttpBinding == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.GetString(SR2.WebHttpBindingNotFoundWithEndpoint, WebEndpointType.Name, typeof(WebHttpBinding).Name))); } return webHttpBinding; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
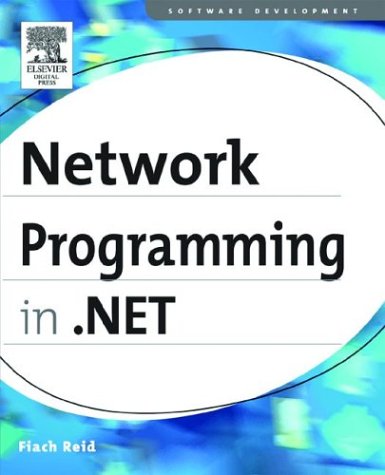
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigurationException.cs
- RecommendedAsConfigurableAttribute.cs
- xml.cs
- ContextProperty.cs
- StdRegProviderWrapper.cs
- ModuleConfigurationInfo.cs
- PeerApplication.cs
- DoubleLinkListEnumerator.cs
- MouseDevice.cs
- InstancePersistenceException.cs
- XmlEventCache.cs
- DataTableReaderListener.cs
- CapiSafeHandles.cs
- CheckBoxList.cs
- SafeProcessHandle.cs
- RequestCachePolicyConverter.cs
- FormViewUpdatedEventArgs.cs
- StringUtil.cs
- TransformGroup.cs
- LineServices.cs
- OracleException.cs
- XmlIlGenerator.cs
- PhysicalFontFamily.cs
- SqlFunctionAttribute.cs
- XmlDataSource.cs
- ValidationErrorCollection.cs
- StylusLogic.cs
- Gdiplus.cs
- ObjectListDesigner.cs
- SmiEventSink.cs
- GC.cs
- RelatedView.cs
- DefaultWorkflowTransactionService.cs
- Image.cs
- XmlDataLoader.cs
- DataGridViewSelectedRowCollection.cs
- SmtpClient.cs
- Permission.cs
- ContractMapping.cs
- SqlAggregateChecker.cs
- DrawingBrush.cs
- LongMinMaxAggregationOperator.cs
- PackagingUtilities.cs
- ToolBarButton.cs
- PartitionResolver.cs
- DecimalAnimation.cs
- GridViewCancelEditEventArgs.cs
- SerializationObjectManager.cs
- TimeSpanConverter.cs
- CommandID.cs
- ProfileService.cs
- PropertyEmitter.cs
- UserNameServiceElement.cs
- EnumValidator.cs
- OutputWindow.cs
- AspNetSynchronizationContext.cs
- _HeaderInfo.cs
- TaskHelper.cs
- CaretElement.cs
- LineInfo.cs
- Tuple.cs
- MatrixAnimationUsingKeyFrames.cs
- OleDbDataReader.cs
- ApplicationInfo.cs
- HierarchicalDataTemplate.cs
- DBCSCodePageEncoding.cs
- SizeAnimation.cs
- IdentifierCollection.cs
- AnnotationHighlightLayer.cs
- ExpressionWriter.cs
- DesignerLoader.cs
- EditCommandColumn.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- Rotation3DAnimationBase.cs
- PingReply.cs
- MetafileHeaderWmf.cs
- ConstraintStruct.cs
- HitTestParameters3D.cs
- Clipboard.cs
- FlowDocumentReaderAutomationPeer.cs
- UserUseLicenseDictionaryLoader.cs
- SkewTransform.cs
- WebPageTraceListener.cs
- WebPartEditorOkVerb.cs
- XmlMembersMapping.cs
- ExclusiveHandle.cs
- TaiwanLunisolarCalendar.cs
- DataControlPagerLinkButton.cs
- HtmlSelect.cs
- InheritanceRules.cs
- ListBoxAutomationPeer.cs
- UnsafeNativeMethods.cs
- ConfigXmlAttribute.cs
- BitVec.cs
- SByte.cs
- DataControlFieldHeaderCell.cs
- BamlCollectionHolder.cs
- EnumerableCollectionView.cs
- BStrWrapper.cs
- DrawingState.cs