Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / Controls / ValidationErrorCollection.cs / 1 / ValidationErrorCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: // ValidationErrorCollection contains the list of ValidationErrors from // the various Bindings and MultiBindings on an Element. ValidationErrorCollection // be set through the Validation.ErrorsProperty. // // See specs at http://avalon/connecteddata/Specs/Validation.mht // // History: // 5/3/2004 mharper: created. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Windows; using System.Windows.Controls; using System.Windows.Data; namespace MS.Internal.Controls { ////// ValidationErrorCollection contains the list of ValidationErrors from /// the various Bindings and MultiBindings on an Element. ValidationErrorCollection /// be set through the Validation.ErrorsProperty. /// internal class ValidationErrorCollection : ObservableCollection{ /// /// Empty collection that serves as a default value for /// Validation.ErrorsProperty. /// public static readonly ReadOnlyObservableCollectionEmpty = new ReadOnlyObservableCollection (new ValidationErrorCollection()); /// /// called by base class Collection<T> when an item is added to list; /// protected override void InsertItem(int index, ValidationError item) { int existingIndex = FindErrorForBinding(item.BindingInError); // if there is already a ValidationError for this binding, // then throw if (existingIndex > -1) { throw new ArgumentException(SR.Get(SRID.DuplicatesNotAllowed), "validationError"); } base.InsertItem(index, item); } //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods static internal ReadOnlyObservableCollectionGetReadOnlyErrors(DependencyObject d) { ValidationErrorCollection errors = Validation.GetErrorsInternal(d); if (errors != null) { if (errors._readonlyWrapper == null) { errors._readonlyWrapper = new ReadOnlyObservableCollection (errors); } return errors._readonlyWrapper; } else { return Empty; } } #endregion Internal Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods private int FindErrorForBinding(object binding) { for (int i = 0; i < this.Count; i++) { if (this[i].BindingInError == binding) { return i; } } return -1; } #endregion Private Methods ReadOnlyObservableCollection _readonlyWrapper; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: // ValidationErrorCollection contains the list of ValidationErrors from // the various Bindings and MultiBindings on an Element. ValidationErrorCollection // be set through the Validation.ErrorsProperty. // // See specs at http://avalon/connecteddata/Specs/Validation.mht // // History: // 5/3/2004 mharper: created. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Windows; using System.Windows.Controls; using System.Windows.Data; namespace MS.Internal.Controls { ////// ValidationErrorCollection contains the list of ValidationErrors from /// the various Bindings and MultiBindings on an Element. ValidationErrorCollection /// be set through the Validation.ErrorsProperty. /// internal class ValidationErrorCollection : ObservableCollection{ /// /// Empty collection that serves as a default value for /// Validation.ErrorsProperty. /// public static readonly ReadOnlyObservableCollectionEmpty = new ReadOnlyObservableCollection (new ValidationErrorCollection()); /// /// called by base class Collection<T> when an item is added to list; /// protected override void InsertItem(int index, ValidationError item) { int existingIndex = FindErrorForBinding(item.BindingInError); // if there is already a ValidationError for this binding, // then throw if (existingIndex > -1) { throw new ArgumentException(SR.Get(SRID.DuplicatesNotAllowed), "validationError"); } base.InsertItem(index, item); } //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods static internal ReadOnlyObservableCollectionGetReadOnlyErrors(DependencyObject d) { ValidationErrorCollection errors = Validation.GetErrorsInternal(d); if (errors != null) { if (errors._readonlyWrapper == null) { errors._readonlyWrapper = new ReadOnlyObservableCollection (errors); } return errors._readonlyWrapper; } else { return Empty; } } #endregion Internal Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods private int FindErrorForBinding(object binding) { for (int i = 0; i < this.Count; i++) { if (this[i].BindingInError == binding) { return i; } } return -1; } #endregion Private Methods ReadOnlyObservableCollection _readonlyWrapper; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
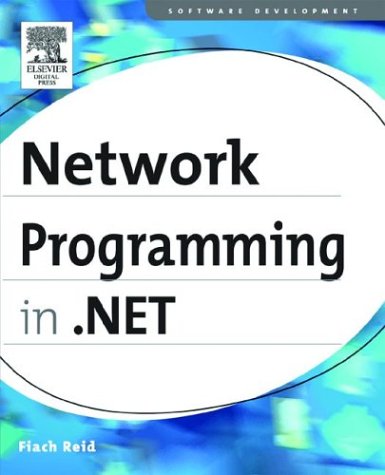
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XamlSerializerUtil.cs
- TableCellCollection.cs
- ResXDataNode.cs
- SmiGettersStream.cs
- HGlobalSafeHandle.cs
- Baml6ConstructorInfo.cs
- SqlExpressionNullability.cs
- TextElementCollection.cs
- XmlEncodedRawTextWriter.cs
- BitArray.cs
- FixedSOMTable.cs
- TransformValueSerializer.cs
- MetaModel.cs
- ListViewSelectEventArgs.cs
- StringComparer.cs
- DataGridViewBindingCompleteEventArgs.cs
- BindingWorker.cs
- LOSFormatter.cs
- Substitution.cs
- PointCollectionValueSerializer.cs
- ExtentKey.cs
- MatrixTransform.cs
- PaintValueEventArgs.cs
- RecordManager.cs
- TimerElapsedEvenArgs.cs
- RuntimeCompatibilityAttribute.cs
- DoWorkEventArgs.cs
- RepeatBehavior.cs
- TreeNodeCollection.cs
- XmlDataDocument.cs
- ToolStripEditorManager.cs
- SecurityKeyIdentifierClause.cs
- ToolTip.cs
- diagnosticsswitches.cs
- CodeGeneratorAttribute.cs
- MaskedTextProvider.cs
- SectionVisual.cs
- OrderByLifter.cs
- ToolBarDesigner.cs
- GeometryModel3D.cs
- Calendar.cs
- BaseTemplateCodeDomTreeGenerator.cs
- FileEnumerator.cs
- ValidationEventArgs.cs
- WebServiceParameterData.cs
- EffectiveValueEntry.cs
- SetIterators.cs
- InitializingNewItemEventArgs.cs
- ICspAsymmetricAlgorithm.cs
- BlockingCollection.cs
- RuleProcessor.cs
- SystemPens.cs
- DataControlFieldCollection.cs
- RelationHandler.cs
- SecurityElement.cs
- DataControlImageButton.cs
- ClientSettingsProvider.cs
- LZCodec.cs
- ConnectionProviderAttribute.cs
- SessionPageStateSection.cs
- IPAddressCollection.cs
- CachedPathData.cs
- UTF8Encoding.cs
- PartialClassGenerationTaskInternal.cs
- DynamicField.cs
- ColorConvertedBitmapExtension.cs
- CommandEventArgs.cs
- ProtocolsSection.cs
- ObjectDataSourceSelectingEventArgs.cs
- HttpListenerRequest.cs
- ToolStripRenderer.cs
- ProcessHostMapPath.cs
- SchemaImporterExtensionElement.cs
- Constant.cs
- DataBinding.cs
- BaseParser.cs
- XamlSerializationHelper.cs
- Clipboard.cs
- ConditionalExpression.cs
- SQLBinary.cs
- MsmqIntegrationSecurity.cs
- ProbeMatchesApril2005.cs
- InternalReceiveMessage.cs
- ProcessModelSection.cs
- TraceEventCache.cs
- DataGridToolTip.cs
- XPathAncestorQuery.cs
- XmlSchemaType.cs
- PartialToken.cs
- FrameworkEventSource.cs
- CqlGenerator.cs
- ClientBuildManagerCallback.cs
- SqlAggregateChecker.cs
- ColorInterpolationModeValidation.cs
- ConditionalAttribute.cs
- TextTreeTextBlock.cs
- EasingKeyFrames.cs
- NamespaceQuery.cs
- _NativeSSPI.cs
- NetPipeSectionData.cs