Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / ToolStripEditorManager.cs / 1 / ToolStripEditorManager.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.Runtime.InteropServices; using System.ComponentModel; using System; using System.ComponentModel.Design; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Drawing; using System.Design; using System.Drawing.Design; using System.Windows.Forms; using System.Windows.Forms.ComponentModel; using System.Windows.Forms.Design; using System.Windows.Forms.Design.Behavior; using System.Collections; ////// /// This internal Class is used by all TOPLEVEL ToolStripItems to show the InSitu Editor. /// /// When the ToolStripItem receives the MouseDown on its Glyph it calls the "ActivateEditor" /// Function on this EditorManager. /// /// The ActivateEditor Function checks for any existing "EDITOR" active, closes that down /// and now opens the new editor on the "AdornerWindow". /// /// This class is also responsible for "hookingup" to the F2 Command on VS. /// /// internal class ToolStripEditorManager { // // Local copy of BehaviorService so that we can add the Insitu Editor to // the AdornerWindow. // private BehaviorService behaviorService; // // Component for this InSitu Editor... (this is a ToolStripItem) // that wants to go into InSitu // private IDesignerHost designerHost; // // This is always ToolStrip // private IComponent comp; // // The current Bounds of the Insitu Editor on Adorner Window.. // These are required for invalidation. // private Rectangle lastKnownEditorBounds = Rectangle.Empty; // // The encapsulated Editor. // private ToolStripEditorControl editor; // // Actual ToolStripEditor for the current ToolStripItem. // private ToolStripTemplateNode editorUI; // // The Current ToolStripItem that needs to go into the InSitu Mode. // We keep a local copy so that when a new item comes in, we can "ROLLBACK" // the existing edit. // private ToolStripItem currentItem; // // The designer for current ToolStripItem. // private ToolStripItemDesigner itemDesigner; // // Constructor // ///public ToolStripEditorManager(IComponent comp) { // get the parent this.comp = comp; this.behaviorService = (BehaviorService)comp.Site.GetService(typeof(BehaviorService)); this.designerHost = (IDesignerHost)comp.Site.GetService(typeof(IDesignerHost)); } //////////////////////////////////////////////////////////////////////////////////// //// //// //// Methods //// //// //// //////////////////////////////////////////////////////////////////////////////////// /// /// /// Activates the editor for the given item.If there's still an editor around /// for the previous-edited item, it is deactivated. /// Pass in 'null' to deactivate and remove the current editor, if any. /// ///internal void ActivateEditor(ToolStripItem item, bool clicked) { if (item != currentItem) { // Remove old editor // if (editor != null ) { behaviorService.AdornerWindowControl.Controls.Remove(editor); behaviorService.Invalidate(editor.Bounds); editorUI = null; editor = null; currentItem = null; itemDesigner.IsEditorActive = false; // Show the previously edited glyph if (currentItem != null) { currentItem = null; } } if (item != null) { // Add new editor from the item... // currentItem = item; if (designerHost != null) { itemDesigner = (ToolStripItemDesigner)designerHost.GetDesigner(currentItem); } editorUI = (ToolStripTemplateNode)itemDesigner.Editor; // If we got an editor, position and focus it. // if (editorUI != null) { // Hide this glyph while it's being edited // itemDesigner.IsEditorActive = true; editor = new ToolStripEditorControl(editorUI.EditorToolStrip, editorUI.Bounds); behaviorService.AdornerWindowControl.Controls.Add(editor); lastKnownEditorBounds = editor.Bounds; editor.BringToFront(); // this is important since the ToolStripEditorControl listens // to textchanged messages from TextBox. editorUI.ignoreFirstKeyUp = true; // Select the Editor... // Put Text and Select it ... editorUI.FocusEditor(currentItem); } } } } /// /// /// This will remove the Command for F2. /// ///internal void CloseManager() { } /// /// /// This LISTENs to the Editor Resize for resizing the Insitu edit on /// the Adorner Window ... CURRENTLY DISABLED. /// private void OnEditorResize(object sender, EventArgs e) { // THIS IS CURRENTLY DISABLE !!!!! // TO DO !! SHOULD WE SUPPORT AUTOSIZED INSITU ????? behaviorService.Invalidate(lastKnownEditorBounds); if (editor != null) { lastKnownEditorBounds = editor.Bounds; } } // --------------------------------------------------------------------------------- // Private Class Implemented for InSitu Editor. // This class just Wraps the ToolStripEditor from the TemplateNode and puts it in // a Panel. // //----------------------------------------------------------------------------------- private class ToolStripEditorControl : Panel { private Control wrappedEditor; private Rectangle bounds; [SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters")] public ToolStripEditorControl(Control editorToolStrip, Rectangle bounds) { this.wrappedEditor = editorToolStrip; this.bounds = bounds; this.wrappedEditor.Resize += new EventHandler(OnWrappedEditorResize); this.Controls.Add(editorToolStrip); this.Location = new Point(bounds.X, bounds.Y); this.Text = "InSituEditorWrapper"; UpdateSize(); } private void OnWrappedEditorResize(object sender, EventArgs e) { //UpdateSize(); } private void UpdateSize() { this.Size = new Size(wrappedEditor.Size.Width, wrappedEditor.Size.Height); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
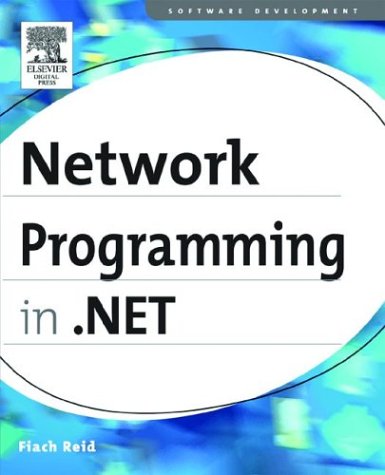
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewCancelEventArgs.cs
- VideoDrawing.cs
- Baml2006KnownTypes.cs
- FormsAuthenticationCredentials.cs
- xmlglyphRunInfo.cs
- GridErrorDlg.cs
- BitmapPalette.cs
- WorkItem.cs
- sqlinternaltransaction.cs
- Control.cs
- RegexWorker.cs
- LineServicesRun.cs
- counter.cs
- DataGridHeadersVisibilityToVisibilityConverter.cs
- ListBox.cs
- sortedlist.cs
- JsonWriter.cs
- QilInvoke.cs
- TemplatedWizardStep.cs
- XmlSchemaComplexContent.cs
- _DisconnectOverlappedAsyncResult.cs
- BooleanKeyFrameCollection.cs
- ProfileProvider.cs
- ContentType.cs
- SizeAnimationClockResource.cs
- EventLogException.cs
- SamlAttributeStatement.cs
- ListManagerBindingsCollection.cs
- DataTrigger.cs
- BrowsableAttribute.cs
- SmtpMail.cs
- TextTrailingCharacterEllipsis.cs
- PeerContact.cs
- DataSourceSerializationException.cs
- Token.cs
- CodeDelegateCreateExpression.cs
- JournalEntryListConverter.cs
- TextTreeObjectNode.cs
- TemplatedWizardStep.cs
- XPathPatternParser.cs
- BindingSource.cs
- XmlSchemaGroupRef.cs
- RbTree.cs
- SqlBuffer.cs
- RSAPKCS1SignatureFormatter.cs
- Directory.cs
- SimpleApplicationHost.cs
- StringReader.cs
- Function.cs
- EntityDataSourceDataSelection.cs
- ResourceIDHelper.cs
- Pen.cs
- DragDeltaEventArgs.cs
- CodeMemberField.cs
- AlignmentXValidation.cs
- TreeViewAutomationPeer.cs
- FontFamilyIdentifier.cs
- TcpAppDomainProtocolHandler.cs
- RouteParametersHelper.cs
- DetailsViewUpdatedEventArgs.cs
- NativeObjectSecurity.cs
- SlotInfo.cs
- Content.cs
- TemplateInstanceAttribute.cs
- ConfigurationValue.cs
- TimeoutValidationAttribute.cs
- Debug.cs
- SafeEventLogWriteHandle.cs
- SQLChars.cs
- ServiceManagerHandle.cs
- GeneralTransformCollection.cs
- UnknownBitmapDecoder.cs
- TypeUtils.cs
- DateTime.cs
- Pair.cs
- WorkflowDebuggerSteppingAttribute.cs
- CompilerError.cs
- PreProcessInputEventArgs.cs
- ConfigXmlWhitespace.cs
- Transform.cs
- EntityConnection.cs
- ForEachAction.cs
- QueryResult.cs
- GridViewItemAutomationPeer.cs
- TypeBuilder.cs
- TabPage.cs
- WindowsAuthenticationModule.cs
- Image.cs
- XmlNullResolver.cs
- RoleManagerModule.cs
- Attribute.cs
- OlePropertyStructs.cs
- Stack.cs
- StateItem.cs
- IfElseDesigner.xaml.cs
- NetworkStream.cs
- ComponentDispatcherThread.cs
- _ShellExpression.cs
- TextRange.cs
- LinkConverter.cs