Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / xsp / System / Web / Extensions / ApplicationServices / ProfileService.cs / 1 / ProfileService.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.ApplicationServices { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Configuration; using System.Security.Permissions; using System.Security.Principal; using System.ServiceModel; using System.ServiceModel.Activation; using System.Web; using System.Web.Management; using System.Web.Profile; using System.Web.Resources; [ServiceContract(Namespace="http://asp.net/ApplicationServices/v200")] [ServiceKnownType("GetKnownTypes", typeof(KnownTypesProvider))] [AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Required)] [AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal)] [ServiceBehavior(Namespace="http://asp.net/ApplicationServices/v200", InstanceContextMode = InstanceContextMode.Single, ConcurrencyMode = ConcurrencyMode.Multiple)] public class ProfileService { ////////////////////////////////////////////////////////////////////// ////// Raised to allow developers to validate property values being set by the client /// private static object _validatingPropertiesEventHandlerLock = new object(); private static EventHandler_validatingProperties; public static event EventHandler ValidatingProperties { add { lock (_validatingPropertiesEventHandlerLock) { _validatingProperties += value; } } remove { lock (_validatingPropertiesEventHandlerLock) { _validatingProperties -= value; } } } /// /// Raises the ValidatingPropertiesEvent if atleast one handler is assigned. /// private void OnValidatingProperties(ValidatingPropertiesEventArgs e) { EventHandlerhandler = _validatingProperties; if (null != handler) { handler(this, e); } } //hiding public constructor internal ProfileService() { } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// [OperationContract] public Dictionary GetPropertiesForCurrentUser(IEnumerable properties, bool authenticatedUserOnly) { if (properties == null) { throw new ArgumentNullException("properties"); } ApplicationServiceHelper.EnsureProfileServiceEnabled(); if (authenticatedUserOnly) { ApplicationServiceHelper.EnsureAuthenticated(HttpContext.Current); } Dictionary retDict = new Dictionary (); ProfileBase pb = null; try { pb = GetProfileForCurrentUser(authenticatedUserOnly); } catch (Exception e) { LogException(e); throw; } if (pb == null) { return null; } Dictionary allowedGet = ApplicationServiceHelper.ProfileAllowedGet; if (allowedGet == null || allowedGet.Count == 0) { // there are no readable properties return retDict; } foreach (string property in properties) { if (property == null) { throw new ArgumentNullException("properties"); } if (allowedGet.ContainsKey(property)) { try { SettingsPropertyValue value = GetPropertyValue(pb, property); if (value != null) { retDict.Add(property, value.PropertyValue); value.IsDirty = false; } } catch (Exception e) { LogException(e); throw; } } } return retDict; } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// [OperationContract] public Dictionary GetAllPropertiesForCurrentUser(bool authenticatedUserOnly) { ApplicationServiceHelper.EnsureProfileServiceEnabled(); if (authenticatedUserOnly) { ApplicationServiceHelper.EnsureAuthenticated(HttpContext.Current); } Dictionary retDict = new Dictionary (); try { ProfileBase pb = GetProfileForCurrentUser(authenticatedUserOnly); if (pb == null) { return null; } Dictionary allowedGet = ApplicationServiceHelper.ProfileAllowedGet; if (allowedGet == null || allowedGet.Count == 0) { // there are no readable properties return retDict; } foreach (KeyValuePair entry in allowedGet) { string propertyName = entry.Key; SettingsPropertyValue value = GetPropertyValue(pb, propertyName); if (value != null) { retDict.Add(propertyName, value.PropertyValue); value.IsDirty = false; } } } catch (Exception e) { LogException(e); throw; } return retDict; } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// [OperationContract] public Collection SetPropertiesForCurrentUser(IDictionary values, bool authenticatedUserOnly) { if (values == null) { throw new ArgumentNullException("values"); } ApplicationServiceHelper.EnsureProfileServiceEnabled(); if (authenticatedUserOnly) { ApplicationServiceHelper.EnsureAuthenticated(HttpContext.Current); } Collection sc = new Collection (); try { ValidatingPropertiesEventArgs vp = new ValidatingPropertiesEventArgs(values); OnValidatingProperties(vp); Dictionary allowedSet = ApplicationServiceHelper.ProfileAllowedSet; ProfileBase pb = GetProfileForCurrentUser(authenticatedUserOnly); foreach (KeyValuePair kvp in values) { string propertyName = kvp.Key; if (pb == null) { sc.Add(propertyName); continue; } if (vp.FailedProperties.Contains(propertyName)) { sc.Add(propertyName); continue; } if (allowedSet == null) { sc.Add(propertyName); continue; } if (!allowedSet.ContainsKey(propertyName)) { sc.Add(propertyName); continue; } SettingsProperty settingProperty = ProfileBase.Properties[propertyName]; if (settingProperty == null) { // property not found sc.Add(propertyName); continue; } if (settingProperty.IsReadOnly || (pb.IsAnonymous && !(bool)settingProperty.Attributes["AllowAnonymous"])) { // property is readonly, or the profile is anonymous and the property isn't enabled for anonymous access sc.Add(propertyName); continue; } SettingsPropertyValue value = GetPropertyValue(pb, kvp.Key); if (value == null) { // property not found sc.Add(propertyName); continue; } else { try { pb[propertyName] = kvp.Value; } catch (System.Configuration.Provider.ProviderException) { // provider specific error sc.Add(propertyName); } catch (System.Configuration.SettingsPropertyNotFoundException) { sc.Add(propertyName); } catch (System.Configuration.SettingsPropertyWrongTypeException) { sc.Add(propertyName); } } } pb.Save(); } catch (Exception e) { LogException(e); throw; } return sc; } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// [OperationContract] public ProfilePropertyMetadata[] GetPropertiesMetadata() { ApplicationServiceHelper.EnsureProfileServiceEnabled(); try { // todo: convert to array is temporary -- this method should just return Collection<> like the other profileservice does. Collection metadatas = ApplicationServiceHelper.GetProfilePropertiesMetadata(); ProfilePropertyMetadata[] metadatasArray = new ProfilePropertyMetadata[metadatas.Count]; metadatas.CopyTo(metadatasArray, 0); return metadatasArray; } catch (Exception e) { LogException(e); throw; } } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// private static SettingsPropertyValue GetPropertyValue(ProfileBase pb, string name) { SettingsProperty prop = ProfileBase.Properties[name]; if (prop == null) { return null; } SettingsPropertyValue p = pb.PropertyValues[name]; if (p == null) { // not fetched from provider pb.GetPropertyValue(name); // to force a fetch from the provider p = pb.PropertyValues[name]; if (p == null) { return null; } } return p; } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// private static ProfileBase GetProfileForCurrentUser(bool authenticatedUserOnly) { HttpContext context = HttpContext.Current; IPrincipal user = ApplicationServiceHelper.GetCurrentUser(context); string name = null; bool isAuthenticated = false; if (user == null || user.Identity == null || string.IsNullOrEmpty(user.Identity.Name)) { // anonymous user? isAuthenticated = false; if (!authenticatedUserOnly && context != null && !string.IsNullOrEmpty(context.Request.AnonymousID)) { // Use Anonymous ID? name = context.Request.AnonymousID; } } else { name = user.Identity.Name; isAuthenticated = user.Identity.IsAuthenticated; } if (!isAuthenticated && (authenticatedUserOnly || string.IsNullOrEmpty(name))) { if (context != null) throw new HttpException(AtlasWeb.UserIsNotAuthenticated); else throw new Exception(AtlasWeb.UserIsNotAuthenticated); } return ProfileBase.Create(name, isAuthenticated); } private void LogException(Exception e) { WebServiceErrorEvent errorevent = new WebServiceErrorEvent(AtlasWeb.UnhandledExceptionEventLogMessage, this, e); errorevent.Raise(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.ApplicationServices { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Configuration; using System.Security.Permissions; using System.Security.Principal; using System.ServiceModel; using System.ServiceModel.Activation; using System.Web; using System.Web.Management; using System.Web.Profile; using System.Web.Resources; [ServiceContract(Namespace="http://asp.net/ApplicationServices/v200")] [ServiceKnownType("GetKnownTypes", typeof(KnownTypesProvider))] [AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Required)] [AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal)] [ServiceBehavior(Namespace="http://asp.net/ApplicationServices/v200", InstanceContextMode = InstanceContextMode.Single, ConcurrencyMode = ConcurrencyMode.Multiple)] public class ProfileService { ////////////////////////////////////////////////////////////////////// ////// Raised to allow developers to validate property values being set by the client /// private static object _validatingPropertiesEventHandlerLock = new object(); private static EventHandler_validatingProperties; public static event EventHandler ValidatingProperties { add { lock (_validatingPropertiesEventHandlerLock) { _validatingProperties += value; } } remove { lock (_validatingPropertiesEventHandlerLock) { _validatingProperties -= value; } } } /// /// Raises the ValidatingPropertiesEvent if atleast one handler is assigned. /// private void OnValidatingProperties(ValidatingPropertiesEventArgs e) { EventHandlerhandler = _validatingProperties; if (null != handler) { handler(this, e); } } //hiding public constructor internal ProfileService() { } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// [OperationContract] public Dictionary GetPropertiesForCurrentUser(IEnumerable properties, bool authenticatedUserOnly) { if (properties == null) { throw new ArgumentNullException("properties"); } ApplicationServiceHelper.EnsureProfileServiceEnabled(); if (authenticatedUserOnly) { ApplicationServiceHelper.EnsureAuthenticated(HttpContext.Current); } Dictionary retDict = new Dictionary (); ProfileBase pb = null; try { pb = GetProfileForCurrentUser(authenticatedUserOnly); } catch (Exception e) { LogException(e); throw; } if (pb == null) { return null; } Dictionary allowedGet = ApplicationServiceHelper.ProfileAllowedGet; if (allowedGet == null || allowedGet.Count == 0) { // there are no readable properties return retDict; } foreach (string property in properties) { if (property == null) { throw new ArgumentNullException("properties"); } if (allowedGet.ContainsKey(property)) { try { SettingsPropertyValue value = GetPropertyValue(pb, property); if (value != null) { retDict.Add(property, value.PropertyValue); value.IsDirty = false; } } catch (Exception e) { LogException(e); throw; } } } return retDict; } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// [OperationContract] public Dictionary GetAllPropertiesForCurrentUser(bool authenticatedUserOnly) { ApplicationServiceHelper.EnsureProfileServiceEnabled(); if (authenticatedUserOnly) { ApplicationServiceHelper.EnsureAuthenticated(HttpContext.Current); } Dictionary retDict = new Dictionary (); try { ProfileBase pb = GetProfileForCurrentUser(authenticatedUserOnly); if (pb == null) { return null; } Dictionary allowedGet = ApplicationServiceHelper.ProfileAllowedGet; if (allowedGet == null || allowedGet.Count == 0) { // there are no readable properties return retDict; } foreach (KeyValuePair entry in allowedGet) { string propertyName = entry.Key; SettingsPropertyValue value = GetPropertyValue(pb, propertyName); if (value != null) { retDict.Add(propertyName, value.PropertyValue); value.IsDirty = false; } } } catch (Exception e) { LogException(e); throw; } return retDict; } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// [OperationContract] public Collection SetPropertiesForCurrentUser(IDictionary values, bool authenticatedUserOnly) { if (values == null) { throw new ArgumentNullException("values"); } ApplicationServiceHelper.EnsureProfileServiceEnabled(); if (authenticatedUserOnly) { ApplicationServiceHelper.EnsureAuthenticated(HttpContext.Current); } Collection sc = new Collection (); try { ValidatingPropertiesEventArgs vp = new ValidatingPropertiesEventArgs(values); OnValidatingProperties(vp); Dictionary allowedSet = ApplicationServiceHelper.ProfileAllowedSet; ProfileBase pb = GetProfileForCurrentUser(authenticatedUserOnly); foreach (KeyValuePair kvp in values) { string propertyName = kvp.Key; if (pb == null) { sc.Add(propertyName); continue; } if (vp.FailedProperties.Contains(propertyName)) { sc.Add(propertyName); continue; } if (allowedSet == null) { sc.Add(propertyName); continue; } if (!allowedSet.ContainsKey(propertyName)) { sc.Add(propertyName); continue; } SettingsProperty settingProperty = ProfileBase.Properties[propertyName]; if (settingProperty == null) { // property not found sc.Add(propertyName); continue; } if (settingProperty.IsReadOnly || (pb.IsAnonymous && !(bool)settingProperty.Attributes["AllowAnonymous"])) { // property is readonly, or the profile is anonymous and the property isn't enabled for anonymous access sc.Add(propertyName); continue; } SettingsPropertyValue value = GetPropertyValue(pb, kvp.Key); if (value == null) { // property not found sc.Add(propertyName); continue; } else { try { pb[propertyName] = kvp.Value; } catch (System.Configuration.Provider.ProviderException) { // provider specific error sc.Add(propertyName); } catch (System.Configuration.SettingsPropertyNotFoundException) { sc.Add(propertyName); } catch (System.Configuration.SettingsPropertyWrongTypeException) { sc.Add(propertyName); } } } pb.Save(); } catch (Exception e) { LogException(e); throw; } return sc; } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// [OperationContract] public ProfilePropertyMetadata[] GetPropertiesMetadata() { ApplicationServiceHelper.EnsureProfileServiceEnabled(); try { // todo: convert to array is temporary -- this method should just return Collection<> like the other profileservice does. Collection metadatas = ApplicationServiceHelper.GetProfilePropertiesMetadata(); ProfilePropertyMetadata[] metadatasArray = new ProfilePropertyMetadata[metadatas.Count]; metadatas.CopyTo(metadatasArray, 0); return metadatasArray; } catch (Exception e) { LogException(e); throw; } } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// private static SettingsPropertyValue GetPropertyValue(ProfileBase pb, string name) { SettingsProperty prop = ProfileBase.Properties[name]; if (prop == null) { return null; } SettingsPropertyValue p = pb.PropertyValues[name]; if (p == null) { // not fetched from provider pb.GetPropertyValue(name); // to force a fetch from the provider p = pb.PropertyValues[name]; if (p == null) { return null; } } return p; } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// private static ProfileBase GetProfileForCurrentUser(bool authenticatedUserOnly) { HttpContext context = HttpContext.Current; IPrincipal user = ApplicationServiceHelper.GetCurrentUser(context); string name = null; bool isAuthenticated = false; if (user == null || user.Identity == null || string.IsNullOrEmpty(user.Identity.Name)) { // anonymous user? isAuthenticated = false; if (!authenticatedUserOnly && context != null && !string.IsNullOrEmpty(context.Request.AnonymousID)) { // Use Anonymous ID? name = context.Request.AnonymousID; } } else { name = user.Identity.Name; isAuthenticated = user.Identity.IsAuthenticated; } if (!isAuthenticated && (authenticatedUserOnly || string.IsNullOrEmpty(name))) { if (context != null) throw new HttpException(AtlasWeb.UserIsNotAuthenticated); else throw new Exception(AtlasWeb.UserIsNotAuthenticated); } return ProfileBase.Create(name, isAuthenticated); } private void LogException(Exception e) { WebServiceErrorEvent errorevent = new WebServiceErrorEvent(AtlasWeb.UnhandledExceptionEventLogMessage, this, e); errorevent.Raise(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
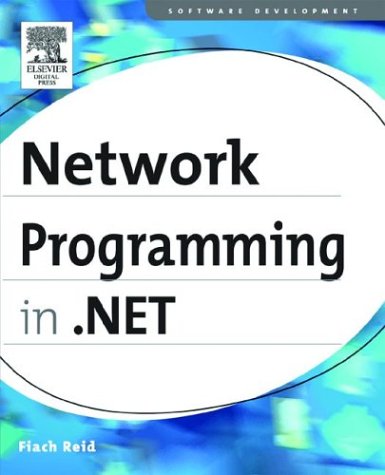
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UncommonField.cs
- EncodingTable.cs
- XmlValidatingReader.cs
- ExtendedProtectionPolicy.cs
- SqlReferenceCollection.cs
- ConfigurationValue.cs
- CompilationUtil.cs
- DataGridColumnHeadersPresenterAutomationPeer.cs
- NaturalLanguageHyphenator.cs
- TreeWalker.cs
- ProjectionPruner.cs
- SqlNode.cs
- safex509handles.cs
- UIElementIsland.cs
- SortedDictionary.cs
- TemplateField.cs
- HotSpotCollection.cs
- WindowsRebar.cs
- ListViewItemMouseHoverEvent.cs
- coordinatorfactory.cs
- AvtEvent.cs
- PathStreamGeometryContext.cs
- SiteMembershipCondition.cs
- DeviceFilterDictionary.cs
- XmlSchemaCollection.cs
- ReadOnlyTernaryTree.cs
- ToolStripGrip.cs
- TemplatingOptionsDialog.cs
- BitmapCodecInfo.cs
- IWorkflowDebuggerService.cs
- PartDesigner.cs
- SEHException.cs
- DbParameterCollection.cs
- RuntimeArgumentHandle.cs
- RadioButton.cs
- ElementProxy.cs
- WindowsStatusBar.cs
- WindowsSolidBrush.cs
- Brush.cs
- SqlFileStream.cs
- SearchForVirtualItemEventArgs.cs
- HandleDictionary.cs
- IdentityModelDictionary.cs
- Drawing.cs
- ControlCachePolicy.cs
- QuotedPrintableStream.cs
- PerformanceCounterPermissionAttribute.cs
- HttpListenerContext.cs
- HttpPostLocalhostServerProtocol.cs
- DisableDpiAwarenessAttribute.cs
- SqlDeflator.cs
- ComponentGuaranteesAttribute.cs
- FixedSOMImage.cs
- mediaeventargs.cs
- QueryHandler.cs
- ProfilePropertySettingsCollection.cs
- Effect.cs
- SiteMapNode.cs
- VirtualDirectoryMapping.cs
- SqlDataRecord.cs
- DecimalAnimation.cs
- DesignOnlyAttribute.cs
- HttpHandlersSection.cs
- TableRowCollection.cs
- WizardPanel.cs
- Literal.cs
- DictionaryEntry.cs
- DropShadowBitmapEffect.cs
- Scheduling.cs
- EntityKeyElement.cs
- Simplifier.cs
- DataMemberConverter.cs
- HebrewNumber.cs
- CursorConverter.cs
- DynamicScriptObject.cs
- DtdParser.cs
- precedingquery.cs
- Baml6Assembly.cs
- BaseTemplatedMobileComponentEditor.cs
- DefaultIfEmptyQueryOperator.cs
- ReflectPropertyDescriptor.cs
- ExtensionQuery.cs
- XmlSerializerVersionAttribute.cs
- TextElementCollection.cs
- XMLDiffLoader.cs
- PtsContext.cs
- DefaultValueTypeConverter.cs
- SqlFunctionAttribute.cs
- DescendantBaseQuery.cs
- RuntimeCompatibilityAttribute.cs
- ControlValuePropertyAttribute.cs
- ToolStripDropDownMenu.cs
- RadioButton.cs
- SerialErrors.cs
- PersianCalendar.cs
- DescendantBaseQuery.cs
- LineSegment.cs
- StrokeCollectionConverter.cs
- NameSpaceExtractor.cs
- EnvironmentPermission.cs