Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Inlined / LongMinMaxAggregationOperator.cs / 1305376 / LongMinMaxAggregationOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // LongMinMaxAggregationOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined min/max aggregation and its enumerator, for longs. /// internal sealed class LongMinMaxAggregationOperator : InlinedAggregationOperator{ private readonly int m_sign; // The sign (-1 for min, 1 for max). //---------------------------------------------------------------------------------------- // Constructs a new instance of a min/max associative operator. // internal LongMinMaxAggregationOperator(IEnumerable child, int sign) : base(child) { Contract.Assert(sign == -1 || sign == 1, "invalid sign"); m_sign = sign; } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override long InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // Throw an error for empty results. if (!enumerator.MoveNext()) { singularExceptionToThrow = new InvalidOperationException(SR.GetString(SR.NoElements)); return default(long); } long best = enumerator.Current; // Based on the sign, do either a min or max reduction. if (m_sign == -1) { while (enumerator.MoveNext()) { long current = enumerator.Current; if (current < best) { best = current; } } } else { while (enumerator.MoveNext()) { long current = enumerator.Current; if (current > best) { best = current; } } } return best; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new LongMinMaxAggregationOperatorEnumerator (source, index, m_sign, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class LongMinMaxAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator { private QueryOperatorEnumerator m_source; // The source data. private int m_sign; // The sign for comparisons (-1 means min, 1 means max). //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal LongMinMaxAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, int sign, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; m_sign = sign; } //--------------------------------------------------------------------------------------- // Tallies up the min/max of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref long currentElement) { // Based on the sign, do either a min or max reduction. QueryOperatorEnumerator source = m_source; TKey keyUnused = default(TKey); if (source.MoveNext(ref currentElement, ref keyUnused)) { int i = 0; // We just scroll through the enumerator and find the min or max. if (m_sign == -1) { long elem = default(long); while (source.MoveNext(ref elem, ref keyUnused)) { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); if (elem < currentElement) { currentElement = elem; } } } else { long elem = default(long); while (source.MoveNext(ref elem, ref keyUnused)) { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); if (elem > currentElement) { currentElement = elem; } } } // The sum has been calculated. Now just return. return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
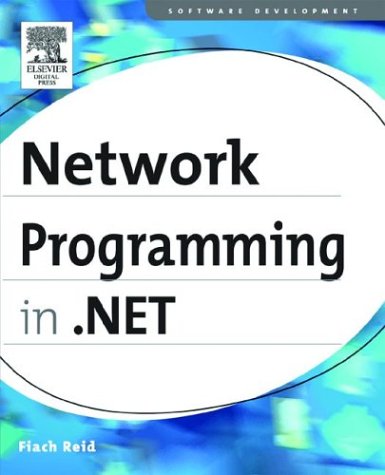
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- httpserverutility.cs
- DomNameTable.cs
- DispatcherFrame.cs
- QilXmlReader.cs
- Timer.cs
- TagMapCollection.cs
- IdentityHolder.cs
- EventDrivenDesigner.cs
- AppDomain.cs
- COM2ExtendedTypeConverter.cs
- compensatingcollection.cs
- ListViewGroupCollectionEditor.cs
- XPathNavigatorReader.cs
- DeploymentSection.cs
- AppDomainAttributes.cs
- validationstate.cs
- SystemIPInterfaceStatistics.cs
- Formatter.cs
- PagePropertiesChangingEventArgs.cs
- SizeValueSerializer.cs
- ListMarkerSourceInfo.cs
- BufferedReceiveManager.cs
- XsltCompileContext.cs
- DependencyPropertyValueSerializer.cs
- KoreanCalendar.cs
- SchemaCollectionPreprocessor.cs
- PropertyEmitterBase.cs
- RSACryptoServiceProvider.cs
- ReturnValue.cs
- XamlBuildTaskServices.cs
- XmlDataProvider.cs
- ILGen.cs
- XmlNodeChangedEventArgs.cs
- ValidationHelpers.cs
- NativeMethods.cs
- TextRangeProviderWrapper.cs
- CodeCatchClauseCollection.cs
- FocusTracker.cs
- TargetConverter.cs
- HttpCookie.cs
- DependencyPropertyKind.cs
- Point3DCollection.cs
- MsmqProcessProtocolHandler.cs
- SqlNodeAnnotations.cs
- BinaryParser.cs
- OperationContextScope.cs
- DiscoveryClientChannelBase.cs
- Message.cs
- AuthenticationService.cs
- PeerNearMe.cs
- SqlDelegatedTransaction.cs
- ApplicationFileParser.cs
- DataPagerFieldItem.cs
- QuaternionAnimation.cs
- SecurityUtils.cs
- Attributes.cs
- MimeBasePart.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- StyleBamlTreeBuilder.cs
- ButtonStandardAdapter.cs
- PublishLicense.cs
- GatewayIPAddressInformationCollection.cs
- ContentPlaceHolderDesigner.cs
- Size.cs
- _Connection.cs
- AmbientProperties.cs
- InputProviderSite.cs
- NTAccount.cs
- LocationSectionRecord.cs
- UserMapPath.cs
- MetadataArtifactLoaderCompositeFile.cs
- WebPartTracker.cs
- ControlCachePolicy.cs
- DataBindingValueUIHandler.cs
- HorizontalAlignConverter.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- Classification.cs
- SafeFileMapViewHandle.cs
- Operators.cs
- WsatConfiguration.cs
- GridViewColumnCollection.cs
- RichTextBoxConstants.cs
- NativeActivityAbortContext.cs
- HttpDictionary.cs
- Closure.cs
- TextTreePropertyUndoUnit.cs
- Crypto.cs
- dbdatarecord.cs
- RNGCryptoServiceProvider.cs
- XmlDomTextWriter.cs
- XmlNamespaceManager.cs
- CodeCompileUnit.cs
- RewritingSimplifier.cs
- LogicalCallContext.cs
- CodeGotoStatement.cs
- ObjectTokenCategory.cs
- DataGridViewComboBoxColumn.cs
- BoundPropertyEntry.cs
- CurrentChangingEventManager.cs
- _PooledStream.cs