Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CommonUI / System / Drawing / Color.cs / 1 / Color.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Globalization; using System.Text; using System.Runtime.Serialization.Formatters; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using Microsoft.Win32; using System.ComponentModel; #if !FEATURE_PAL using System.Drawing.Design; #endif using System.Runtime.InteropServices; ////// /// Represents an ARGB color. /// [ Serializable(), TypeConverter(typeof(ColorConverter)), DebuggerDisplay("{NameAndARGBValue}"), #if !FEATURE_PAL Editor("System.Drawing.Design.ColorEditor, " + AssemblyRef.SystemDrawingDesign, typeof(UITypeEditor)), #endif ] public struct Color { ////// /// public static readonly Color Empty = new Color(); // ------------------------------------------------------------------- // static list of "web" colors... // ///[To be supplied.] ////// /// public static Color Transparent { get { return new Color(KnownColor.Transparent); } } ///[To be supplied.] ////// /// public static Color AliceBlue { get { return new Color(KnownColor.AliceBlue); } } ///[To be supplied.] ////// /// public static Color AntiqueWhite { get { return new Color(KnownColor.AntiqueWhite); } } ///[To be supplied.] ////// /// public static Color Aqua { get { return new Color(KnownColor.Aqua); } } ///[To be supplied.] ////// /// public static Color Aquamarine { get { return new Color(KnownColor.Aquamarine); } } ///[To be supplied.] ////// /// public static Color Azure { get { return new Color(KnownColor.Azure); } } ///[To be supplied.] ////// /// public static Color Beige { get { return new Color(KnownColor.Beige); } } ///[To be supplied.] ////// /// public static Color Bisque { get { return new Color(KnownColor.Bisque); } } ///[To be supplied.] ////// /// public static Color Black { get { return new Color(KnownColor.Black); } } ///[To be supplied.] ////// /// public static Color BlanchedAlmond { get { return new Color(KnownColor.BlanchedAlmond); } } ///[To be supplied.] ////// /// public static Color Blue { get { return new Color(KnownColor.Blue); } } ///[To be supplied.] ////// /// public static Color BlueViolet { get { return new Color(KnownColor.BlueViolet); } } ///[To be supplied.] ////// /// public static Color Brown { get { return new Color(KnownColor.Brown); } } ///[To be supplied.] ////// /// public static Color BurlyWood { get { return new Color(KnownColor.BurlyWood); } } ///[To be supplied.] ////// /// public static Color CadetBlue { get { return new Color(KnownColor.CadetBlue); } } ///[To be supplied.] ////// /// public static Color Chartreuse { get { return new Color(KnownColor.Chartreuse); } } ///[To be supplied.] ////// /// public static Color Chocolate { get { return new Color(KnownColor.Chocolate); } } ///[To be supplied.] ////// /// public static Color Coral { get { return new Color(KnownColor.Coral); } } ///[To be supplied.] ////// /// public static Color CornflowerBlue { get { return new Color(KnownColor.CornflowerBlue); } } ///[To be supplied.] ////// /// public static Color Cornsilk { get { return new Color(KnownColor.Cornsilk); } } ///[To be supplied.] ////// /// public static Color Crimson { get { return new Color(KnownColor.Crimson); } } ///[To be supplied.] ////// /// public static Color Cyan { get { return new Color(KnownColor.Cyan); } } ///[To be supplied.] ////// /// public static Color DarkBlue { get { return new Color(KnownColor.DarkBlue); } } ///[To be supplied.] ////// /// public static Color DarkCyan { get { return new Color(KnownColor.DarkCyan); } } ///[To be supplied.] ////// /// public static Color DarkGoldenrod { get { return new Color(KnownColor.DarkGoldenrod); } } ///[To be supplied.] ////// /// public static Color DarkGray { get { return new Color(KnownColor.DarkGray); } } ///[To be supplied.] ////// /// public static Color DarkGreen { get { return new Color(KnownColor.DarkGreen); } } ///[To be supplied.] ////// /// public static Color DarkKhaki { get { return new Color(KnownColor.DarkKhaki); } } ///[To be supplied.] ////// /// public static Color DarkMagenta { get { return new Color(KnownColor.DarkMagenta); } } ///[To be supplied.] ////// /// public static Color DarkOliveGreen { get { return new Color(KnownColor.DarkOliveGreen); } } ///[To be supplied.] ////// /// public static Color DarkOrange { get { return new Color(KnownColor.DarkOrange); } } ///[To be supplied.] ////// /// public static Color DarkOrchid { get { return new Color(KnownColor.DarkOrchid); } } ///[To be supplied.] ////// /// public static Color DarkRed { get { return new Color(KnownColor.DarkRed); } } ///[To be supplied.] ////// /// public static Color DarkSalmon { get { return new Color(KnownColor.DarkSalmon); } } ///[To be supplied.] ////// /// public static Color DarkSeaGreen { get { return new Color(KnownColor.DarkSeaGreen); } } ///[To be supplied.] ////// /// public static Color DarkSlateBlue { get { return new Color(KnownColor.DarkSlateBlue); } } ///[To be supplied.] ////// /// public static Color DarkSlateGray { get { return new Color(KnownColor.DarkSlateGray); } } ///[To be supplied.] ////// /// public static Color DarkTurquoise { get { return new Color(KnownColor.DarkTurquoise); } } ///[To be supplied.] ////// /// public static Color DarkViolet { get { return new Color(KnownColor.DarkViolet); } } ///[To be supplied.] ////// /// public static Color DeepPink { get { return new Color(KnownColor.DeepPink); } } ///[To be supplied.] ////// /// public static Color DeepSkyBlue { get { return new Color(KnownColor.DeepSkyBlue); } } ///[To be supplied.] ////// /// public static Color DimGray { get { return new Color(KnownColor.DimGray); } } ///[To be supplied.] ////// /// public static Color DodgerBlue { get { return new Color(KnownColor.DodgerBlue); } } ///[To be supplied.] ////// /// public static Color Firebrick { get { return new Color(KnownColor.Firebrick); } } ///[To be supplied.] ////// /// public static Color FloralWhite { get { return new Color(KnownColor.FloralWhite); } } ///[To be supplied.] ////// /// public static Color ForestGreen { get { return new Color(KnownColor.ForestGreen); } } ///[To be supplied.] ////// /// public static Color Fuchsia { get { return new Color(KnownColor.Fuchsia); } } ///[To be supplied.] ////// /// public static Color Gainsboro { get { return new Color(KnownColor.Gainsboro); } } ///[To be supplied.] ////// /// public static Color GhostWhite { get { return new Color(KnownColor.GhostWhite); } } ///[To be supplied.] ////// /// public static Color Gold { get { return new Color(KnownColor.Gold); } } ///[To be supplied.] ////// /// public static Color Goldenrod { get { return new Color(KnownColor.Goldenrod); } } ///[To be supplied.] ////// /// public static Color Gray { get { return new Color(KnownColor.Gray); } } ///[To be supplied.] ////// /// public static Color Green { get { return new Color(KnownColor.Green); } } ///[To be supplied.] ////// /// public static Color GreenYellow { get { return new Color(KnownColor.GreenYellow); } } ///[To be supplied.] ////// /// public static Color Honeydew { get { return new Color(KnownColor.Honeydew); } } ///[To be supplied.] ////// /// public static Color HotPink { get { return new Color(KnownColor.HotPink); } } ///[To be supplied.] ////// /// public static Color IndianRed { get { return new Color(KnownColor.IndianRed); } } ///[To be supplied.] ////// /// public static Color Indigo { get { return new Color(KnownColor.Indigo); } } ///[To be supplied.] ////// /// public static Color Ivory { get { return new Color(KnownColor.Ivory); } } ///[To be supplied.] ////// /// public static Color Khaki { get { return new Color(KnownColor.Khaki); } } ///[To be supplied.] ////// /// public static Color Lavender { get { return new Color(KnownColor.Lavender); } } ///[To be supplied.] ////// /// public static Color LavenderBlush { get { return new Color(KnownColor.LavenderBlush); } } ///[To be supplied.] ////// /// public static Color LawnGreen { get { return new Color(KnownColor.LawnGreen); } } ///[To be supplied.] ////// /// public static Color LemonChiffon { get { return new Color(KnownColor.LemonChiffon); } } ///[To be supplied.] ////// /// public static Color LightBlue { get { return new Color(KnownColor.LightBlue); } } ///[To be supplied.] ////// /// public static Color LightCoral { get { return new Color(KnownColor.LightCoral); } } ///[To be supplied.] ////// /// public static Color LightCyan { get { return new Color(KnownColor.LightCyan); } } ///[To be supplied.] ////// /// public static Color LightGoldenrodYellow { get { return new Color(KnownColor.LightGoldenrodYellow); } } ///[To be supplied.] ////// /// public static Color LightGreen { get { return new Color(KnownColor.LightGreen); } } ///[To be supplied.] ////// /// public static Color LightGray { get { return new Color(KnownColor.LightGray); } } ///[To be supplied.] ////// /// public static Color LightPink { get { return new Color(KnownColor.LightPink); } } ///[To be supplied.] ////// /// public static Color LightSalmon { get { return new Color(KnownColor.LightSalmon); } } ///[To be supplied.] ////// /// public static Color LightSeaGreen { get { return new Color(KnownColor.LightSeaGreen); } } ///[To be supplied.] ////// /// public static Color LightSkyBlue { get { return new Color(KnownColor.LightSkyBlue); } } ///[To be supplied.] ////// /// public static Color LightSlateGray { get { return new Color(KnownColor.LightSlateGray); } } ///[To be supplied.] ////// /// public static Color LightSteelBlue { get { return new Color(KnownColor.LightSteelBlue); } } ///[To be supplied.] ////// /// public static Color LightYellow { get { return new Color(KnownColor.LightYellow); } } ///[To be supplied.] ////// /// public static Color Lime { get { return new Color(KnownColor.Lime); } } ///[To be supplied.] ////// /// public static Color LimeGreen { get { return new Color(KnownColor.LimeGreen); } } ///[To be supplied.] ////// /// public static Color Linen { get { return new Color(KnownColor.Linen); } } ///[To be supplied.] ////// /// public static Color Magenta { get { return new Color(KnownColor.Magenta); } } ///[To be supplied.] ////// /// public static Color Maroon { get { return new Color(KnownColor.Maroon); } } ///[To be supplied.] ////// /// public static Color MediumAquamarine { get { return new Color(KnownColor.MediumAquamarine); } } ///[To be supplied.] ////// /// public static Color MediumBlue { get { return new Color(KnownColor.MediumBlue); } } ///[To be supplied.] ////// /// public static Color MediumOrchid { get { return new Color(KnownColor.MediumOrchid); } } ///[To be supplied.] ////// /// public static Color MediumPurple { get { return new Color(KnownColor.MediumPurple); } } ///[To be supplied.] ////// /// public static Color MediumSeaGreen { get { return new Color(KnownColor.MediumSeaGreen); } } ///[To be supplied.] ////// /// public static Color MediumSlateBlue { get { return new Color(KnownColor.MediumSlateBlue); } } ///[To be supplied.] ////// /// public static Color MediumSpringGreen { get { return new Color(KnownColor.MediumSpringGreen); } } ///[To be supplied.] ////// /// public static Color MediumTurquoise { get { return new Color(KnownColor.MediumTurquoise); } } ///[To be supplied.] ////// /// public static Color MediumVioletRed { get { return new Color(KnownColor.MediumVioletRed); } } ///[To be supplied.] ////// /// public static Color MidnightBlue { get { return new Color(KnownColor.MidnightBlue); } } ///[To be supplied.] ////// /// public static Color MintCream { get { return new Color(KnownColor.MintCream); } } ///[To be supplied.] ////// /// public static Color MistyRose { get { return new Color(KnownColor.MistyRose); } } ///[To be supplied.] ////// /// public static Color Moccasin { get { return new Color(KnownColor.Moccasin); } } ///[To be supplied.] ////// /// public static Color NavajoWhite { get { return new Color(KnownColor.NavajoWhite); } } ///[To be supplied.] ////// /// public static Color Navy { get { return new Color(KnownColor.Navy); } } ///[To be supplied.] ////// /// public static Color OldLace { get { return new Color(KnownColor.OldLace); } } ///[To be supplied.] ////// /// public static Color Olive { get { return new Color(KnownColor.Olive); } } ///[To be supplied.] ////// /// public static Color OliveDrab { get { return new Color(KnownColor.OliveDrab); } } ///[To be supplied.] ////// /// public static Color Orange { get { return new Color(KnownColor.Orange); } } ///[To be supplied.] ////// /// public static Color OrangeRed { get { return new Color(KnownColor.OrangeRed); } } ///[To be supplied.] ////// /// public static Color Orchid { get { return new Color(KnownColor.Orchid); } } ///[To be supplied.] ////// /// public static Color PaleGoldenrod { get { return new Color(KnownColor.PaleGoldenrod); } } ///[To be supplied.] ////// /// public static Color PaleGreen { get { return new Color(KnownColor.PaleGreen); } } ///[To be supplied.] ////// /// public static Color PaleTurquoise { get { return new Color(KnownColor.PaleTurquoise); } } ///[To be supplied.] ////// /// public static Color PaleVioletRed { get { return new Color(KnownColor.PaleVioletRed); } } ///[To be supplied.] ////// /// public static Color PapayaWhip { get { return new Color(KnownColor.PapayaWhip); } } ///[To be supplied.] ////// /// public static Color PeachPuff { get { return new Color(KnownColor.PeachPuff); } } ///[To be supplied.] ////// /// public static Color Peru { get { return new Color(KnownColor.Peru); } } ///[To be supplied.] ////// /// public static Color Pink { get { return new Color(KnownColor.Pink); } } ///[To be supplied.] ////// /// public static Color Plum { get { return new Color(KnownColor.Plum); } } ///[To be supplied.] ////// /// public static Color PowderBlue { get { return new Color(KnownColor.PowderBlue); } } ///[To be supplied.] ////// /// public static Color Purple { get { return new Color(KnownColor.Purple); } } ///[To be supplied.] ////// /// public static Color Red { get { return new Color(KnownColor.Red); } } ///[To be supplied.] ////// /// public static Color RosyBrown { get { return new Color(KnownColor.RosyBrown); } } ///[To be supplied.] ////// /// public static Color RoyalBlue { get { return new Color(KnownColor.RoyalBlue); } } ///[To be supplied.] ////// /// public static Color SaddleBrown { get { return new Color(KnownColor.SaddleBrown); } } ///[To be supplied.] ////// /// public static Color Salmon { get { return new Color(KnownColor.Salmon); } } ///[To be supplied.] ////// /// public static Color SandyBrown { get { return new Color(KnownColor.SandyBrown); } } ///[To be supplied.] ////// /// public static Color SeaGreen { get { return new Color(KnownColor.SeaGreen); } } ///[To be supplied.] ////// /// public static Color SeaShell { get { return new Color(KnownColor.SeaShell); } } ///[To be supplied.] ////// /// public static Color Sienna { get { return new Color(KnownColor.Sienna); } } ///[To be supplied.] ////// /// public static Color Silver { get { return new Color(KnownColor.Silver); } } ///[To be supplied.] ////// /// public static Color SkyBlue { get { return new Color(KnownColor.SkyBlue); } } ///[To be supplied.] ////// /// public static Color SlateBlue { get { return new Color(KnownColor.SlateBlue); } } ///[To be supplied.] ////// /// public static Color SlateGray { get { return new Color(KnownColor.SlateGray); } } ///[To be supplied.] ////// /// public static Color Snow { get { return new Color(KnownColor.Snow); } } ///[To be supplied.] ////// /// public static Color SpringGreen { get { return new Color(KnownColor.SpringGreen); } } ///[To be supplied.] ////// /// public static Color SteelBlue { get { return new Color(KnownColor.SteelBlue); } } ///[To be supplied.] ////// /// public static Color Tan { get { return new Color(KnownColor.Tan); } } ///[To be supplied.] ////// /// public static Color Teal { get { return new Color(KnownColor.Teal); } } ///[To be supplied.] ////// /// public static Color Thistle { get { return new Color(KnownColor.Thistle); } } ///[To be supplied.] ////// /// public static Color Tomato { get { return new Color(KnownColor.Tomato); } } ///[To be supplied.] ////// /// public static Color Turquoise { get { return new Color(KnownColor.Turquoise); } } ///[To be supplied.] ////// /// public static Color Violet { get { return new Color(KnownColor.Violet); } } ///[To be supplied.] ////// /// public static Color Wheat { get { return new Color(KnownColor.Wheat); } } ///[To be supplied.] ////// /// public static Color White { get { return new Color(KnownColor.White); } } ///[To be supplied.] ////// /// public static Color WhiteSmoke { get { return new Color(KnownColor.WhiteSmoke); } } ///[To be supplied.] ////// /// public static Color Yellow { get { return new Color(KnownColor.Yellow); } } ///[To be supplied.] ////// /// public static Color YellowGreen { get { return new Color(KnownColor.YellowGreen); } } // // end "web" colors // ------------------------------------------------------------------- // NOTE : The "zero" pattern (all members being 0) must represent // : "not set". This allows "Color c;" to be correct. private static short StateKnownColorValid = 0x0001; private static short StateARGBValueValid = 0x0002; private static short StateValueMask = (short)(StateARGBValueValid); private static short StateNameValid = 0x0008; private static long NotDefinedValue = 0; /** * Shift count and bit mask for A, R, G, B components in ARGB mode! */ private const int ARGBAlphaShift = 24; private const int ARGBRedShift = 16; private const int ARGBGreenShift = 8; private const int ARGBBlueShift = 0; /// WARNING!!! WARNING!!! WARNING!!! WARNING!!! /// WARNING!!! WARNING!!! WARNING!!! WARNING!!! /// We can never change the layout of this class (adding or removing or changing the /// order of member variables) if you want to be compatible v1.0 version of the runtime. /// This is so that we can push into the runtime a custom marshaller for OLE_COLOR to Color. // user supplied name of color. Will not be filled in if // we map to a "knowncolor" // private readonly string name; // will contain standard 32bit sRGB (ARGB) // private readonly long value; // ignored, unless "state" says it is valid // private readonly short knownColor; // implementation specific information // private readonly short state; internal Color(KnownColor knownColor) { value = 0; state = StateKnownColorValid; name = null; this.knownColor = (short)knownColor; } private Color(long value, short state, string name, KnownColor knownColor) { this.value = value; this.state = state; this.name = name; this.knownColor = (short)knownColor; } ///[To be supplied.] ////// /// Gets the red component value for this public byte R { get { return(byte)((Value >> ARGBRedShift) & 0xFF); } } ///. /// /// /// Gets the green component value for this public byte G { get { return(byte)((Value >> ARGBGreenShift) & 0xFF); } } ///. /// /// /// public byte B { get { return(byte)((Value >> ARGBBlueShift) & 0xFF); } } ////// Gets the blue component value for this ///. /// /// /// public byte A { get { return(byte)((Value >> ARGBAlphaShift) & 0xFF); } } ////// Gets the alpha component value for this ///. /// /// /// public bool IsKnownColor { get { return((state & StateKnownColorValid) != 0); } } ////// Specifies whether this ///is a known (predefined) color. /// Predefined colors are defined in the /// enum. /// /// /// Specifies whether this public bool IsEmpty { get { return state == 0; } } ///is uninitialized. /// /// /// Specifies whether this public bool IsNamedColor { get { return ((state & StateNameValid) != 0) || IsKnownColor; } } ///has a name or is a . /// /// /// Determines if this color is a system color. /// public bool IsSystemColor { get { return IsKnownColor && ((((KnownColor) knownColor) <= KnownColor.WindowText) || (((KnownColor) knownColor) > KnownColor.YellowGreen)); } } // Not localized because it's only used for the DebuggerDisplayAttribute, and the values are // programmatic items. // Also, don't inline into the attribute for performance reasons. This way means the debugger // does 1 func-eval instead of 5. [SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters")] private string NameAndARGBValue { get { return string.Format(CultureInfo.CurrentCulture, "{{Name={0}, ARGB=({1}, {2}, {3}, {4})}}", Name, A, R, G, B); } } ////// /// public string Name { get { if ((state & StateNameValid) != 0) { return name; } if (IsKnownColor) { // first try the table so we can avoid the (slow!) .ToString() string tablename = KnownColorTable.KnownColorToName((KnownColor) knownColor); if (tablename != null) return tablename; Debug.Assert(false, "Could not find known color '" + ((KnownColor) knownColor) + "' in the KnownColorTable"); return ((KnownColor)knownColor).ToString(); } // if we reached here, just encode the value // return Convert.ToString(value, 16); } } ////// Gets the name of this ///. This will either return the user /// defined name of the color, if the color was created from a name, or /// the name of the known color. For custom colors, the RGB value will /// be returned. /// /// /// Actual color to be rendered. /// private long Value { get { if ((state & StateValueMask) != 0) { return value; } if (IsKnownColor) { return(int)KnownColorTable.KnownColorToArgb((KnownColor)knownColor); } return NotDefinedValue; } } private static void CheckByte(int value, string name) { if (value < 0 || value > 255) throw new ArgumentException(SR.GetString(SR.InvalidEx2BoundArgument, name, value, 0, 255)); } ////// /// Encodes the four values into ARGB (32 bit) format. /// private static long MakeArgb(byte alpha, byte red, byte green, byte blue) { return(long)((uint)(red << ARGBRedShift | green << ARGBGreenShift | blue << ARGBBlueShift | alpha << ARGBAlphaShift)) & 0xffffffff; } ////// /// public static Color FromArgb(int argb) { return new Color((long)argb & 0xffffffff, StateARGBValueValid, null, (KnownColor)0); } ////// Creates a Color from its 32-bit component /// (alpha, red, green, and blue) values. /// ////// /// public static Color FromArgb(int alpha, int red, int green, int blue) { CheckByte(alpha, "alpha"); CheckByte(red, "red"); CheckByte(green, "green"); CheckByte(blue, "blue"); return new Color(MakeArgb((byte) alpha, (byte) red, (byte) green, (byte) blue), StateARGBValueValid, null, (KnownColor)0); } ////// Creates a Color from its 32-bit component (alpha, red, /// green, and blue) values. /// ////// /// public static Color FromArgb(int alpha, Color baseColor) { CheckByte(alpha, "alpha"); return new Color(MakeArgb((byte) alpha, baseColor.R, baseColor.G, baseColor.B), StateARGBValueValid, null, (KnownColor)0); } ////// Creates a new ///from the specified , but with /// the new specified alpha value. /// /// /// public static Color FromArgb(int red, int green, int blue) { return FromArgb(255, red, green, blue); } ////// Creates a ///from the specified red, green, and /// blue values. /// /// /// public static Color FromKnownColor(KnownColor color) { if( !ClientUtils.IsEnumValid( color, (int) color, (int) KnownColor.ActiveBorder, (int) KnownColor.MenuHighlight ) ) { return Color.FromName(color.ToString()); } return new Color(color); } ////// Creates a ///from the specified . /// /// /// public static Color FromName(string name) { // try to get a known color first object color = ColorConverter.GetNamedColor(name); if (color != null) { return (Color)color; } // otherwise treat it as a named color return new Color(NotDefinedValue, StateNameValid, name, (KnownColor)0); } ////// Creates a ///with the specified name. /// /// /// public float GetBrightness() { float r = (float)R / 255.0f; float g = (float)G / 255.0f; float b = (float)B / 255.0f; float max, min; max = r; min = r; if (g > max) max = g; if (b > max) max = b; if (g < min) min = g; if (b < min) min = b; return(max + min) / 2; } ////// Returns the Hue-Saturation-Brightness (HSB) brightness /// for this ///. /// /// /// public Single GetHue() { if (R == G && G == B) return 0; // 0 makes as good an UNDEFINED value as any float r = (float)R / 255.0f; float g = (float)G / 255.0f; float b = (float)B / 255.0f; float max, min; float delta; float hue = 0.0f; max = r; min = r; if (g > max) max = g; if (b > max) max = b; if (g < min) min = g; if (b < min) min = b; delta = max - min; if (r == max) { hue = (g - b) / delta; } else if (g == max) { hue = 2 + (b - r) / delta; } else if (b == max) { hue = 4 + (r - g) / delta; } hue *= 60; if (hue < 0.0f) { hue += 360.0f; } return hue; } ////// Returns the Hue-Saturation-Brightness (HSB) hue /// value, in degrees, for this ///. /// If R == G == B, the hue is meaningless, and the return value is 0. /// /// /// public float GetSaturation() { float r = (float)R / 255.0f; float g = (float)G / 255.0f; float b = (float)B / 255.0f; float max, min; float l, s = 0; max = r; min = r; if (g > max) max = g; if (b > max) max = b; if (g < min) min = g; if (b < min) min = b; // if max == min, then there is no color and // the saturation is zero. // if (max != min) { l = (max + min) / 2; if (l <= .5) { s = (max - min)/(max + min); } else { s = (max - min)/(2 - max - min); } } return s; } ////// The Hue-Saturation-Brightness (HSB) saturation for this /// ////// . /// /// /// public int ToArgb() { return(int)Value; } ////// Returns the ARGB value of this ///. /// /// /// public KnownColor ToKnownColor() { return(KnownColor)knownColor; } ////// Returns the ///value for this color, if it is /// based on a . /// /// /// Converts this public override string ToString() { StringBuilder sb = new StringBuilder(32); sb.Append(GetType().Name); sb.Append(" ["); if ((state & StateNameValid) != 0) { sb.Append(Name); } else if ((state & StateKnownColorValid) != 0) { sb.Append(Name); } else if ((state & StateValueMask) != 0) { sb.Append("A="); sb.Append(A); sb.Append(", R="); sb.Append(R); sb.Append(", G="); sb.Append(G); sb.Append(", B="); sb.Append(B); } else { sb.Append("Empty"); } sb.Append("]"); return sb.ToString(); } ///to a human-readable /// string. /// /// /// public static bool operator ==(Color left, Color right) { if (left.value == right.value && left.state == right.state && left.knownColor == right.knownColor) { if (left.name == right.name) { return true; } if (left.name == (object) null || right.name == (object) null) { return false; } return left.name.Equals(right.name); } return false; } ////// Tests whether two specified ///objects /// are equivalent. /// /// /// public static bool operator !=(Color left, Color right) { return !(left == right); } ////// Tests whether two specified ///objects /// are equivalent. /// /// /// Tests whether the specified object is a /// public override bool Equals(object obj) { if (obj is Color) { Color right = (Color)obj; if (value == right.value && state == right.state && knownColor == right.knownColor) { if (name == right.name) { return true; } if (name == (object) null || right.name == (object) null) { return false; } return name.Equals(name); } } return false; } ////// and is equivalent to this . /// /// /// public override int GetHashCode() { return value.GetHashCode() ^ state.GetHashCode() ^ knownColor.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Globalization; using System.Text; using System.Runtime.Serialization.Formatters; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using Microsoft.Win32; using System.ComponentModel; #if !FEATURE_PAL using System.Drawing.Design; #endif using System.Runtime.InteropServices; ////// /// Represents an ARGB color. /// [ Serializable(), TypeConverter(typeof(ColorConverter)), DebuggerDisplay("{NameAndARGBValue}"), #if !FEATURE_PAL Editor("System.Drawing.Design.ColorEditor, " + AssemblyRef.SystemDrawingDesign, typeof(UITypeEditor)), #endif ] public struct Color { ////// /// public static readonly Color Empty = new Color(); // ------------------------------------------------------------------- // static list of "web" colors... // ///[To be supplied.] ////// /// public static Color Transparent { get { return new Color(KnownColor.Transparent); } } ///[To be supplied.] ////// /// public static Color AliceBlue { get { return new Color(KnownColor.AliceBlue); } } ///[To be supplied.] ////// /// public static Color AntiqueWhite { get { return new Color(KnownColor.AntiqueWhite); } } ///[To be supplied.] ////// /// public static Color Aqua { get { return new Color(KnownColor.Aqua); } } ///[To be supplied.] ////// /// public static Color Aquamarine { get { return new Color(KnownColor.Aquamarine); } } ///[To be supplied.] ////// /// public static Color Azure { get { return new Color(KnownColor.Azure); } } ///[To be supplied.] ////// /// public static Color Beige { get { return new Color(KnownColor.Beige); } } ///[To be supplied.] ////// /// public static Color Bisque { get { return new Color(KnownColor.Bisque); } } ///[To be supplied.] ////// /// public static Color Black { get { return new Color(KnownColor.Black); } } ///[To be supplied.] ////// /// public static Color BlanchedAlmond { get { return new Color(KnownColor.BlanchedAlmond); } } ///[To be supplied.] ////// /// public static Color Blue { get { return new Color(KnownColor.Blue); } } ///[To be supplied.] ////// /// public static Color BlueViolet { get { return new Color(KnownColor.BlueViolet); } } ///[To be supplied.] ////// /// public static Color Brown { get { return new Color(KnownColor.Brown); } } ///[To be supplied.] ////// /// public static Color BurlyWood { get { return new Color(KnownColor.BurlyWood); } } ///[To be supplied.] ////// /// public static Color CadetBlue { get { return new Color(KnownColor.CadetBlue); } } ///[To be supplied.] ////// /// public static Color Chartreuse { get { return new Color(KnownColor.Chartreuse); } } ///[To be supplied.] ////// /// public static Color Chocolate { get { return new Color(KnownColor.Chocolate); } } ///[To be supplied.] ////// /// public static Color Coral { get { return new Color(KnownColor.Coral); } } ///[To be supplied.] ////// /// public static Color CornflowerBlue { get { return new Color(KnownColor.CornflowerBlue); } } ///[To be supplied.] ////// /// public static Color Cornsilk { get { return new Color(KnownColor.Cornsilk); } } ///[To be supplied.] ////// /// public static Color Crimson { get { return new Color(KnownColor.Crimson); } } ///[To be supplied.] ////// /// public static Color Cyan { get { return new Color(KnownColor.Cyan); } } ///[To be supplied.] ////// /// public static Color DarkBlue { get { return new Color(KnownColor.DarkBlue); } } ///[To be supplied.] ////// /// public static Color DarkCyan { get { return new Color(KnownColor.DarkCyan); } } ///[To be supplied.] ////// /// public static Color DarkGoldenrod { get { return new Color(KnownColor.DarkGoldenrod); } } ///[To be supplied.] ////// /// public static Color DarkGray { get { return new Color(KnownColor.DarkGray); } } ///[To be supplied.] ////// /// public static Color DarkGreen { get { return new Color(KnownColor.DarkGreen); } } ///[To be supplied.] ////// /// public static Color DarkKhaki { get { return new Color(KnownColor.DarkKhaki); } } ///[To be supplied.] ////// /// public static Color DarkMagenta { get { return new Color(KnownColor.DarkMagenta); } } ///[To be supplied.] ////// /// public static Color DarkOliveGreen { get { return new Color(KnownColor.DarkOliveGreen); } } ///[To be supplied.] ////// /// public static Color DarkOrange { get { return new Color(KnownColor.DarkOrange); } } ///[To be supplied.] ////// /// public static Color DarkOrchid { get { return new Color(KnownColor.DarkOrchid); } } ///[To be supplied.] ////// /// public static Color DarkRed { get { return new Color(KnownColor.DarkRed); } } ///[To be supplied.] ////// /// public static Color DarkSalmon { get { return new Color(KnownColor.DarkSalmon); } } ///[To be supplied.] ////// /// public static Color DarkSeaGreen { get { return new Color(KnownColor.DarkSeaGreen); } } ///[To be supplied.] ////// /// public static Color DarkSlateBlue { get { return new Color(KnownColor.DarkSlateBlue); } } ///[To be supplied.] ////// /// public static Color DarkSlateGray { get { return new Color(KnownColor.DarkSlateGray); } } ///[To be supplied.] ////// /// public static Color DarkTurquoise { get { return new Color(KnownColor.DarkTurquoise); } } ///[To be supplied.] ////// /// public static Color DarkViolet { get { return new Color(KnownColor.DarkViolet); } } ///[To be supplied.] ////// /// public static Color DeepPink { get { return new Color(KnownColor.DeepPink); } } ///[To be supplied.] ////// /// public static Color DeepSkyBlue { get { return new Color(KnownColor.DeepSkyBlue); } } ///[To be supplied.] ////// /// public static Color DimGray { get { return new Color(KnownColor.DimGray); } } ///[To be supplied.] ////// /// public static Color DodgerBlue { get { return new Color(KnownColor.DodgerBlue); } } ///[To be supplied.] ////// /// public static Color Firebrick { get { return new Color(KnownColor.Firebrick); } } ///[To be supplied.] ////// /// public static Color FloralWhite { get { return new Color(KnownColor.FloralWhite); } } ///[To be supplied.] ////// /// public static Color ForestGreen { get { return new Color(KnownColor.ForestGreen); } } ///[To be supplied.] ////// /// public static Color Fuchsia { get { return new Color(KnownColor.Fuchsia); } } ///[To be supplied.] ////// /// public static Color Gainsboro { get { return new Color(KnownColor.Gainsboro); } } ///[To be supplied.] ////// /// public static Color GhostWhite { get { return new Color(KnownColor.GhostWhite); } } ///[To be supplied.] ////// /// public static Color Gold { get { return new Color(KnownColor.Gold); } } ///[To be supplied.] ////// /// public static Color Goldenrod { get { return new Color(KnownColor.Goldenrod); } } ///[To be supplied.] ////// /// public static Color Gray { get { return new Color(KnownColor.Gray); } } ///[To be supplied.] ////// /// public static Color Green { get { return new Color(KnownColor.Green); } } ///[To be supplied.] ////// /// public static Color GreenYellow { get { return new Color(KnownColor.GreenYellow); } } ///[To be supplied.] ////// /// public static Color Honeydew { get { return new Color(KnownColor.Honeydew); } } ///[To be supplied.] ////// /// public static Color HotPink { get { return new Color(KnownColor.HotPink); } } ///[To be supplied.] ////// /// public static Color IndianRed { get { return new Color(KnownColor.IndianRed); } } ///[To be supplied.] ////// /// public static Color Indigo { get { return new Color(KnownColor.Indigo); } } ///[To be supplied.] ////// /// public static Color Ivory { get { return new Color(KnownColor.Ivory); } } ///[To be supplied.] ////// /// public static Color Khaki { get { return new Color(KnownColor.Khaki); } } ///[To be supplied.] ////// /// public static Color Lavender { get { return new Color(KnownColor.Lavender); } } ///[To be supplied.] ////// /// public static Color LavenderBlush { get { return new Color(KnownColor.LavenderBlush); } } ///[To be supplied.] ////// /// public static Color LawnGreen { get { return new Color(KnownColor.LawnGreen); } } ///[To be supplied.] ////// /// public static Color LemonChiffon { get { return new Color(KnownColor.LemonChiffon); } } ///[To be supplied.] ////// /// public static Color LightBlue { get { return new Color(KnownColor.LightBlue); } } ///[To be supplied.] ////// /// public static Color LightCoral { get { return new Color(KnownColor.LightCoral); } } ///[To be supplied.] ////// /// public static Color LightCyan { get { return new Color(KnownColor.LightCyan); } } ///[To be supplied.] ////// /// public static Color LightGoldenrodYellow { get { return new Color(KnownColor.LightGoldenrodYellow); } } ///[To be supplied.] ////// /// public static Color LightGreen { get { return new Color(KnownColor.LightGreen); } } ///[To be supplied.] ////// /// public static Color LightGray { get { return new Color(KnownColor.LightGray); } } ///[To be supplied.] ////// /// public static Color LightPink { get { return new Color(KnownColor.LightPink); } } ///[To be supplied.] ////// /// public static Color LightSalmon { get { return new Color(KnownColor.LightSalmon); } } ///[To be supplied.] ////// /// public static Color LightSeaGreen { get { return new Color(KnownColor.LightSeaGreen); } } ///[To be supplied.] ////// /// public static Color LightSkyBlue { get { return new Color(KnownColor.LightSkyBlue); } } ///[To be supplied.] ////// /// public static Color LightSlateGray { get { return new Color(KnownColor.LightSlateGray); } } ///[To be supplied.] ////// /// public static Color LightSteelBlue { get { return new Color(KnownColor.LightSteelBlue); } } ///[To be supplied.] ////// /// public static Color LightYellow { get { return new Color(KnownColor.LightYellow); } } ///[To be supplied.] ////// /// public static Color Lime { get { return new Color(KnownColor.Lime); } } ///[To be supplied.] ////// /// public static Color LimeGreen { get { return new Color(KnownColor.LimeGreen); } } ///[To be supplied.] ////// /// public static Color Linen { get { return new Color(KnownColor.Linen); } } ///[To be supplied.] ////// /// public static Color Magenta { get { return new Color(KnownColor.Magenta); } } ///[To be supplied.] ////// /// public static Color Maroon { get { return new Color(KnownColor.Maroon); } } ///[To be supplied.] ////// /// public static Color MediumAquamarine { get { return new Color(KnownColor.MediumAquamarine); } } ///[To be supplied.] ////// /// public static Color MediumBlue { get { return new Color(KnownColor.MediumBlue); } } ///[To be supplied.] ////// /// public static Color MediumOrchid { get { return new Color(KnownColor.MediumOrchid); } } ///[To be supplied.] ////// /// public static Color MediumPurple { get { return new Color(KnownColor.MediumPurple); } } ///[To be supplied.] ////// /// public static Color MediumSeaGreen { get { return new Color(KnownColor.MediumSeaGreen); } } ///[To be supplied.] ////// /// public static Color MediumSlateBlue { get { return new Color(KnownColor.MediumSlateBlue); } } ///[To be supplied.] ////// /// public static Color MediumSpringGreen { get { return new Color(KnownColor.MediumSpringGreen); } } ///[To be supplied.] ////// /// public static Color MediumTurquoise { get { return new Color(KnownColor.MediumTurquoise); } } ///[To be supplied.] ////// /// public static Color MediumVioletRed { get { return new Color(KnownColor.MediumVioletRed); } } ///[To be supplied.] ////// /// public static Color MidnightBlue { get { return new Color(KnownColor.MidnightBlue); } } ///[To be supplied.] ////// /// public static Color MintCream { get { return new Color(KnownColor.MintCream); } } ///[To be supplied.] ////// /// public static Color MistyRose { get { return new Color(KnownColor.MistyRose); } } ///[To be supplied.] ////// /// public static Color Moccasin { get { return new Color(KnownColor.Moccasin); } } ///[To be supplied.] ////// /// public static Color NavajoWhite { get { return new Color(KnownColor.NavajoWhite); } } ///[To be supplied.] ////// /// public static Color Navy { get { return new Color(KnownColor.Navy); } } ///[To be supplied.] ////// /// public static Color OldLace { get { return new Color(KnownColor.OldLace); } } ///[To be supplied.] ////// /// public static Color Olive { get { return new Color(KnownColor.Olive); } } ///[To be supplied.] ////// /// public static Color OliveDrab { get { return new Color(KnownColor.OliveDrab); } } ///[To be supplied.] ////// /// public static Color Orange { get { return new Color(KnownColor.Orange); } } ///[To be supplied.] ////// /// public static Color OrangeRed { get { return new Color(KnownColor.OrangeRed); } } ///[To be supplied.] ////// /// public static Color Orchid { get { return new Color(KnownColor.Orchid); } } ///[To be supplied.] ////// /// public static Color PaleGoldenrod { get { return new Color(KnownColor.PaleGoldenrod); } } ///[To be supplied.] ////// /// public static Color PaleGreen { get { return new Color(KnownColor.PaleGreen); } } ///[To be supplied.] ////// /// public static Color PaleTurquoise { get { return new Color(KnownColor.PaleTurquoise); } } ///[To be supplied.] ////// /// public static Color PaleVioletRed { get { return new Color(KnownColor.PaleVioletRed); } } ///[To be supplied.] ////// /// public static Color PapayaWhip { get { return new Color(KnownColor.PapayaWhip); } } ///[To be supplied.] ////// /// public static Color PeachPuff { get { return new Color(KnownColor.PeachPuff); } } ///[To be supplied.] ////// /// public static Color Peru { get { return new Color(KnownColor.Peru); } } ///[To be supplied.] ////// /// public static Color Pink { get { return new Color(KnownColor.Pink); } } ///[To be supplied.] ////// /// public static Color Plum { get { return new Color(KnownColor.Plum); } } ///[To be supplied.] ////// /// public static Color PowderBlue { get { return new Color(KnownColor.PowderBlue); } } ///[To be supplied.] ////// /// public static Color Purple { get { return new Color(KnownColor.Purple); } } ///[To be supplied.] ////// /// public static Color Red { get { return new Color(KnownColor.Red); } } ///[To be supplied.] ////// /// public static Color RosyBrown { get { return new Color(KnownColor.RosyBrown); } } ///[To be supplied.] ////// /// public static Color RoyalBlue { get { return new Color(KnownColor.RoyalBlue); } } ///[To be supplied.] ////// /// public static Color SaddleBrown { get { return new Color(KnownColor.SaddleBrown); } } ///[To be supplied.] ////// /// public static Color Salmon { get { return new Color(KnownColor.Salmon); } } ///[To be supplied.] ////// /// public static Color SandyBrown { get { return new Color(KnownColor.SandyBrown); } } ///[To be supplied.] ////// /// public static Color SeaGreen { get { return new Color(KnownColor.SeaGreen); } } ///[To be supplied.] ////// /// public static Color SeaShell { get { return new Color(KnownColor.SeaShell); } } ///[To be supplied.] ////// /// public static Color Sienna { get { return new Color(KnownColor.Sienna); } } ///[To be supplied.] ////// /// public static Color Silver { get { return new Color(KnownColor.Silver); } } ///[To be supplied.] ////// /// public static Color SkyBlue { get { return new Color(KnownColor.SkyBlue); } } ///[To be supplied.] ////// /// public static Color SlateBlue { get { return new Color(KnownColor.SlateBlue); } } ///[To be supplied.] ////// /// public static Color SlateGray { get { return new Color(KnownColor.SlateGray); } } ///[To be supplied.] ////// /// public static Color Snow { get { return new Color(KnownColor.Snow); } } ///[To be supplied.] ////// /// public static Color SpringGreen { get { return new Color(KnownColor.SpringGreen); } } ///[To be supplied.] ////// /// public static Color SteelBlue { get { return new Color(KnownColor.SteelBlue); } } ///[To be supplied.] ////// /// public static Color Tan { get { return new Color(KnownColor.Tan); } } ///[To be supplied.] ////// /// public static Color Teal { get { return new Color(KnownColor.Teal); } } ///[To be supplied.] ////// /// public static Color Thistle { get { return new Color(KnownColor.Thistle); } } ///[To be supplied.] ////// /// public static Color Tomato { get { return new Color(KnownColor.Tomato); } } ///[To be supplied.] ////// /// public static Color Turquoise { get { return new Color(KnownColor.Turquoise); } } ///[To be supplied.] ////// /// public static Color Violet { get { return new Color(KnownColor.Violet); } } ///[To be supplied.] ////// /// public static Color Wheat { get { return new Color(KnownColor.Wheat); } } ///[To be supplied.] ////// /// public static Color White { get { return new Color(KnownColor.White); } } ///[To be supplied.] ////// /// public static Color WhiteSmoke { get { return new Color(KnownColor.WhiteSmoke); } } ///[To be supplied.] ////// /// public static Color Yellow { get { return new Color(KnownColor.Yellow); } } ///[To be supplied.] ////// /// public static Color YellowGreen { get { return new Color(KnownColor.YellowGreen); } } // // end "web" colors // ------------------------------------------------------------------- // NOTE : The "zero" pattern (all members being 0) must represent // : "not set". This allows "Color c;" to be correct. private static short StateKnownColorValid = 0x0001; private static short StateARGBValueValid = 0x0002; private static short StateValueMask = (short)(StateARGBValueValid); private static short StateNameValid = 0x0008; private static long NotDefinedValue = 0; /** * Shift count and bit mask for A, R, G, B components in ARGB mode! */ private const int ARGBAlphaShift = 24; private const int ARGBRedShift = 16; private const int ARGBGreenShift = 8; private const int ARGBBlueShift = 0; /// WARNING!!! WARNING!!! WARNING!!! WARNING!!! /// WARNING!!! WARNING!!! WARNING!!! WARNING!!! /// We can never change the layout of this class (adding or removing or changing the /// order of member variables) if you want to be compatible v1.0 version of the runtime. /// This is so that we can push into the runtime a custom marshaller for OLE_COLOR to Color. // user supplied name of color. Will not be filled in if // we map to a "knowncolor" // private readonly string name; // will contain standard 32bit sRGB (ARGB) // private readonly long value; // ignored, unless "state" says it is valid // private readonly short knownColor; // implementation specific information // private readonly short state; internal Color(KnownColor knownColor) { value = 0; state = StateKnownColorValid; name = null; this.knownColor = (short)knownColor; } private Color(long value, short state, string name, KnownColor knownColor) { this.value = value; this.state = state; this.name = name; this.knownColor = (short)knownColor; } ///[To be supplied.] ////// /// Gets the red component value for this public byte R { get { return(byte)((Value >> ARGBRedShift) & 0xFF); } } ///. /// /// /// Gets the green component value for this public byte G { get { return(byte)((Value >> ARGBGreenShift) & 0xFF); } } ///. /// /// /// public byte B { get { return(byte)((Value >> ARGBBlueShift) & 0xFF); } } ////// Gets the blue component value for this ///. /// /// /// public byte A { get { return(byte)((Value >> ARGBAlphaShift) & 0xFF); } } ////// Gets the alpha component value for this ///. /// /// /// public bool IsKnownColor { get { return((state & StateKnownColorValid) != 0); } } ////// Specifies whether this ///is a known (predefined) color. /// Predefined colors are defined in the /// enum. /// /// /// Specifies whether this public bool IsEmpty { get { return state == 0; } } ///is uninitialized. /// /// /// Specifies whether this public bool IsNamedColor { get { return ((state & StateNameValid) != 0) || IsKnownColor; } } ///has a name or is a . /// /// /// Determines if this color is a system color. /// public bool IsSystemColor { get { return IsKnownColor && ((((KnownColor) knownColor) <= KnownColor.WindowText) || (((KnownColor) knownColor) > KnownColor.YellowGreen)); } } // Not localized because it's only used for the DebuggerDisplayAttribute, and the values are // programmatic items. // Also, don't inline into the attribute for performance reasons. This way means the debugger // does 1 func-eval instead of 5. [SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters")] private string NameAndARGBValue { get { return string.Format(CultureInfo.CurrentCulture, "{{Name={0}, ARGB=({1}, {2}, {3}, {4})}}", Name, A, R, G, B); } } ////// /// public string Name { get { if ((state & StateNameValid) != 0) { return name; } if (IsKnownColor) { // first try the table so we can avoid the (slow!) .ToString() string tablename = KnownColorTable.KnownColorToName((KnownColor) knownColor); if (tablename != null) return tablename; Debug.Assert(false, "Could not find known color '" + ((KnownColor) knownColor) + "' in the KnownColorTable"); return ((KnownColor)knownColor).ToString(); } // if we reached here, just encode the value // return Convert.ToString(value, 16); } } ////// Gets the name of this ///. This will either return the user /// defined name of the color, if the color was created from a name, or /// the name of the known color. For custom colors, the RGB value will /// be returned. /// /// /// Actual color to be rendered. /// private long Value { get { if ((state & StateValueMask) != 0) { return value; } if (IsKnownColor) { return(int)KnownColorTable.KnownColorToArgb((KnownColor)knownColor); } return NotDefinedValue; } } private static void CheckByte(int value, string name) { if (value < 0 || value > 255) throw new ArgumentException(SR.GetString(SR.InvalidEx2BoundArgument, name, value, 0, 255)); } ////// /// Encodes the four values into ARGB (32 bit) format. /// private static long MakeArgb(byte alpha, byte red, byte green, byte blue) { return(long)((uint)(red << ARGBRedShift | green << ARGBGreenShift | blue << ARGBBlueShift | alpha << ARGBAlphaShift)) & 0xffffffff; } ////// /// public static Color FromArgb(int argb) { return new Color((long)argb & 0xffffffff, StateARGBValueValid, null, (KnownColor)0); } ////// Creates a Color from its 32-bit component /// (alpha, red, green, and blue) values. /// ////// /// public static Color FromArgb(int alpha, int red, int green, int blue) { CheckByte(alpha, "alpha"); CheckByte(red, "red"); CheckByte(green, "green"); CheckByte(blue, "blue"); return new Color(MakeArgb((byte) alpha, (byte) red, (byte) green, (byte) blue), StateARGBValueValid, null, (KnownColor)0); } ////// Creates a Color from its 32-bit component (alpha, red, /// green, and blue) values. /// ////// /// public static Color FromArgb(int alpha, Color baseColor) { CheckByte(alpha, "alpha"); return new Color(MakeArgb((byte) alpha, baseColor.R, baseColor.G, baseColor.B), StateARGBValueValid, null, (KnownColor)0); } ////// Creates a new ///from the specified , but with /// the new specified alpha value. /// /// /// public static Color FromArgb(int red, int green, int blue) { return FromArgb(255, red, green, blue); } ////// Creates a ///from the specified red, green, and /// blue values. /// /// /// public static Color FromKnownColor(KnownColor color) { if( !ClientUtils.IsEnumValid( color, (int) color, (int) KnownColor.ActiveBorder, (int) KnownColor.MenuHighlight ) ) { return Color.FromName(color.ToString()); } return new Color(color); } ////// Creates a ///from the specified . /// /// /// public static Color FromName(string name) { // try to get a known color first object color = ColorConverter.GetNamedColor(name); if (color != null) { return (Color)color; } // otherwise treat it as a named color return new Color(NotDefinedValue, StateNameValid, name, (KnownColor)0); } ////// Creates a ///with the specified name. /// /// /// public float GetBrightness() { float r = (float)R / 255.0f; float g = (float)G / 255.0f; float b = (float)B / 255.0f; float max, min; max = r; min = r; if (g > max) max = g; if (b > max) max = b; if (g < min) min = g; if (b < min) min = b; return(max + min) / 2; } ////// Returns the Hue-Saturation-Brightness (HSB) brightness /// for this ///. /// /// /// public Single GetHue() { if (R == G && G == B) return 0; // 0 makes as good an UNDEFINED value as any float r = (float)R / 255.0f; float g = (float)G / 255.0f; float b = (float)B / 255.0f; float max, min; float delta; float hue = 0.0f; max = r; min = r; if (g > max) max = g; if (b > max) max = b; if (g < min) min = g; if (b < min) min = b; delta = max - min; if (r == max) { hue = (g - b) / delta; } else if (g == max) { hue = 2 + (b - r) / delta; } else if (b == max) { hue = 4 + (r - g) / delta; } hue *= 60; if (hue < 0.0f) { hue += 360.0f; } return hue; } ////// Returns the Hue-Saturation-Brightness (HSB) hue /// value, in degrees, for this ///. /// If R == G == B, the hue is meaningless, and the return value is 0. /// /// /// public float GetSaturation() { float r = (float)R / 255.0f; float g = (float)G / 255.0f; float b = (float)B / 255.0f; float max, min; float l, s = 0; max = r; min = r; if (g > max) max = g; if (b > max) max = b; if (g < min) min = g; if (b < min) min = b; // if max == min, then there is no color and // the saturation is zero. // if (max != min) { l = (max + min) / 2; if (l <= .5) { s = (max - min)/(max + min); } else { s = (max - min)/(2 - max - min); } } return s; } ////// The Hue-Saturation-Brightness (HSB) saturation for this /// ////// . /// /// /// public int ToArgb() { return(int)Value; } ////// Returns the ARGB value of this ///. /// /// /// public KnownColor ToKnownColor() { return(KnownColor)knownColor; } ////// Returns the ///value for this color, if it is /// based on a . /// /// /// Converts this public override string ToString() { StringBuilder sb = new StringBuilder(32); sb.Append(GetType().Name); sb.Append(" ["); if ((state & StateNameValid) != 0) { sb.Append(Name); } else if ((state & StateKnownColorValid) != 0) { sb.Append(Name); } else if ((state & StateValueMask) != 0) { sb.Append("A="); sb.Append(A); sb.Append(", R="); sb.Append(R); sb.Append(", G="); sb.Append(G); sb.Append(", B="); sb.Append(B); } else { sb.Append("Empty"); } sb.Append("]"); return sb.ToString(); } ///to a human-readable /// string. /// /// /// public static bool operator ==(Color left, Color right) { if (left.value == right.value && left.state == right.state && left.knownColor == right.knownColor) { if (left.name == right.name) { return true; } if (left.name == (object) null || right.name == (object) null) { return false; } return left.name.Equals(right.name); } return false; } ////// Tests whether two specified ///objects /// are equivalent. /// /// /// public static bool operator !=(Color left, Color right) { return !(left == right); } ////// Tests whether two specified ///objects /// are equivalent. /// /// /// Tests whether the specified object is a /// public override bool Equals(object obj) { if (obj is Color) { Color right = (Color)obj; if (value == right.value && state == right.state && knownColor == right.knownColor) { if (name == right.name) { return true; } if (name == (object) null || right.name == (object) null) { return false; } return name.Equals(name); } } return false; } ////// and is equivalent to this . /// /// /// public override int GetHashCode() { return value.GetHashCode() ^ state.GetHashCode() ^ knownColor.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
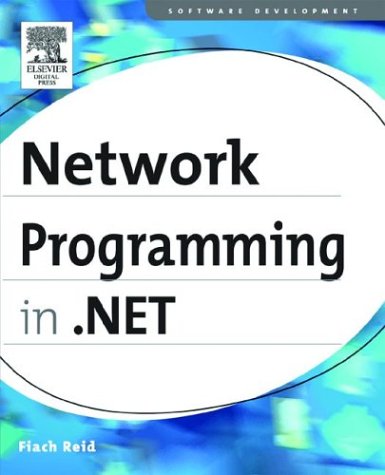
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PeerApplicationLaunchInfo.cs
- XComponentModel.cs
- OracleDataAdapter.cs
- GenericsInstances.cs
- PaginationProgressEventArgs.cs
- UpdateExpressionVisitor.cs
- _TimerThread.cs
- AdPostCacheSubstitution.cs
- DataGridViewRowPrePaintEventArgs.cs
- RSAPKCS1SignatureFormatter.cs
- DataServiceConfiguration.cs
- StateItem.cs
- DataGridViewCellEventArgs.cs
- Point3DValueSerializer.cs
- ActiveXSite.cs
- WebPartDescriptionCollection.cs
- SettingsPropertyCollection.cs
- DbConnectionInternal.cs
- TemplateContent.cs
- ClientTargetCollection.cs
- ApplicationServiceHelper.cs
- ArrayExtension.cs
- cookiecollection.cs
- CallbackHandler.cs
- ObjectConverter.cs
- StringUtil.cs
- OleDbDataAdapter.cs
- RefreshPropertiesAttribute.cs
- WmiPutTraceRecord.cs
- SweepDirectionValidation.cs
- DesignerExtenders.cs
- RemoteWebConfigurationHostServer.cs
- ProcessingInstructionAction.cs
- Matrix.cs
- MatrixAnimationUsingPath.cs
- CopyOnWriteList.cs
- CreateUserErrorEventArgs.cs
- SchemaElementLookUpTable.cs
- CodeDomSerializerBase.cs
- SqlDataSourceQueryEditorForm.cs
- PtsHelper.cs
- bindurihelper.cs
- ResourceCategoryAttribute.cs
- XmlDictionaryReader.cs
- XmlSerializerVersionAttribute.cs
- StackOverflowException.cs
- DateTimeFormatInfo.cs
- DependencyPropertyConverter.cs
- StrokeRenderer.cs
- CodeTypeMember.cs
- SchemaEntity.cs
- XmlnsDictionary.cs
- ObjectStateFormatter.cs
- CookielessHelper.cs
- MetadataItem_Static.cs
- ListenerHandler.cs
- InstanceLockedException.cs
- StringHelper.cs
- QuestionEventArgs.cs
- TextModifierScope.cs
- LogoValidationException.cs
- SessionEndingEventArgs.cs
- AlphaSortedEnumConverter.cs
- _LocalDataStoreMgr.cs
- DiscriminatorMap.cs
- StandardOleMarshalObject.cs
- Shape.cs
- WebPartDisplayModeCancelEventArgs.cs
- HttpProxyTransportBindingElement.cs
- ToolStripDropDownItem.cs
- ObjectDataProvider.cs
- CachedTypeface.cs
- RenderingEventArgs.cs
- DataSourceGeneratorException.cs
- Quaternion.cs
- CacheHelper.cs
- XmlEncodedRawTextWriter.cs
- StreamResourceInfo.cs
- UserControlDocumentDesigner.cs
- Zone.cs
- GeometryHitTestResult.cs
- ApplicationServiceManager.cs
- BufferBuilder.cs
- FileAuthorizationModule.cs
- ComponentManagerBroker.cs
- UrlAuthFailedErrorFormatter.cs
- GridView.cs
- HttpContext.cs
- OleDbParameter.cs
- HtmlTitle.cs
- ConstraintCollection.cs
- SqlRowUpdatedEvent.cs
- Identifier.cs
- Stopwatch.cs
- BaseTreeIterator.cs
- ISAPIApplicationHost.cs
- AddingNewEventArgs.cs
- BrowserInteropHelper.cs
- TraceListener.cs
- SQLBinaryStorage.cs