Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / XmlUtils / System / Xml / Xsl / Runtime / XmlAggregates.cs / 1 / XmlAggregates.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Xml; using System.Diagnostics; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// Computes aggregates over a sequence of Int32 values. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct Int32Aggregator { private int result; private int cnt; public void Create() { this.cnt = 0; } public void Sum(int value) { if (this.cnt == 0) { this.result = value; this.cnt = 1; } else { this.result += value; } } public void Average(int value) { if (this.cnt == 0) this.result = value; else this.result += value; this.cnt++; } public void Minimum(int value) { if (this.cnt == 0 || value < this.result) this.result = value; this.cnt = 1; } public void Maximum(int value) { if (this.cnt == 0 || value > this.result) this.result = value; this.cnt = 1; } public int SumResult { get { return this.result; } } public int AverageResult { get { return this.result / this.cnt; } } public int MinimumResult { get { return this.result; } } public int MaximumResult { get { return this.result; } } public bool IsEmpty { get { return this.cnt == 0; } } } ////// Computes aggregates over a sequence of Int64 values. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct Int64Aggregator { private long result; private int cnt; public void Create() { this.cnt = 0; } public void Sum(long value) { if (this.cnt == 0) { this.result = value; this.cnt = 1; } else { this.result += value; } } public void Average(long value) { if (this.cnt == 0) this.result = value; else this.result += value; this.cnt++; } public void Minimum(long value) { if (this.cnt == 0 || value < this.result) this.result = value; this.cnt = 1; } public void Maximum(long value) { if (this.cnt == 0 || value > this.result) this.result = value; this.cnt = 1; } public long SumResult { get { return this.result; } } public long AverageResult { get { return this.result / (long) this.cnt; } } public long MinimumResult { get { return this.result; } } public long MaximumResult { get { return this.result; } } public bool IsEmpty { get { return this.cnt == 0; } } } ////// Computes aggregates over a sequence of Decimal values. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct DecimalAggregator { private decimal result; private int cnt; public void Create() { this.cnt = 0; } public void Sum(decimal value) { if (this.cnt == 0) { this.result = value; this.cnt = 1; } else { this.result += value; } } public void Average(decimal value) { if (this.cnt == 0) this.result = value; else this.result += value; this.cnt++; } public void Minimum(decimal value) { if (this.cnt == 0 || value < this.result) this.result = value; this.cnt = 1; } public void Maximum(decimal value) { if (this.cnt == 0 || value > this.result) this.result = value; this.cnt = 1; } public decimal SumResult { get { return this.result; } } public decimal AverageResult { get { return this.result / (decimal) this.cnt; } } public decimal MinimumResult { get { return this.result; } } public decimal MaximumResult { get { return this.result; } } public bool IsEmpty { get { return this.cnt == 0; } } } ////// Computes aggregates over a sequence of Double values. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct DoubleAggregator { private double result; private int cnt; public void Create() { this.cnt = 0; } public void Sum(double value) { if (this.cnt == 0) { this.result = value; this.cnt = 1; } else { this.result += value; } } public void Average(double value) { if (this.cnt == 0) this.result = value; else this.result += value; this.cnt++; } public void Minimum(double value) { if (this.cnt == 0 || value < this.result || double.IsNaN(value)) this.result = value; this.cnt = 1; } public void Maximum(double value) { if (this.cnt == 0 || value > this.result || double.IsNaN(value)) this.result = value; this.cnt = 1; } public double SumResult { get { return this.result; } } public double AverageResult { get { return this.result / (double) this.cnt; } } public double MinimumResult { get { return this.result; } } public double MaximumResult { get { return this.result; } } public bool IsEmpty { get { return this.cnt == 0; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Xml; using System.Diagnostics; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// Computes aggregates over a sequence of Int32 values. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct Int32Aggregator { private int result; private int cnt; public void Create() { this.cnt = 0; } public void Sum(int value) { if (this.cnt == 0) { this.result = value; this.cnt = 1; } else { this.result += value; } } public void Average(int value) { if (this.cnt == 0) this.result = value; else this.result += value; this.cnt++; } public void Minimum(int value) { if (this.cnt == 0 || value < this.result) this.result = value; this.cnt = 1; } public void Maximum(int value) { if (this.cnt == 0 || value > this.result) this.result = value; this.cnt = 1; } public int SumResult { get { return this.result; } } public int AverageResult { get { return this.result / this.cnt; } } public int MinimumResult { get { return this.result; } } public int MaximumResult { get { return this.result; } } public bool IsEmpty { get { return this.cnt == 0; } } } ////// Computes aggregates over a sequence of Int64 values. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct Int64Aggregator { private long result; private int cnt; public void Create() { this.cnt = 0; } public void Sum(long value) { if (this.cnt == 0) { this.result = value; this.cnt = 1; } else { this.result += value; } } public void Average(long value) { if (this.cnt == 0) this.result = value; else this.result += value; this.cnt++; } public void Minimum(long value) { if (this.cnt == 0 || value < this.result) this.result = value; this.cnt = 1; } public void Maximum(long value) { if (this.cnt == 0 || value > this.result) this.result = value; this.cnt = 1; } public long SumResult { get { return this.result; } } public long AverageResult { get { return this.result / (long) this.cnt; } } public long MinimumResult { get { return this.result; } } public long MaximumResult { get { return this.result; } } public bool IsEmpty { get { return this.cnt == 0; } } } ////// Computes aggregates over a sequence of Decimal values. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct DecimalAggregator { private decimal result; private int cnt; public void Create() { this.cnt = 0; } public void Sum(decimal value) { if (this.cnt == 0) { this.result = value; this.cnt = 1; } else { this.result += value; } } public void Average(decimal value) { if (this.cnt == 0) this.result = value; else this.result += value; this.cnt++; } public void Minimum(decimal value) { if (this.cnt == 0 || value < this.result) this.result = value; this.cnt = 1; } public void Maximum(decimal value) { if (this.cnt == 0 || value > this.result) this.result = value; this.cnt = 1; } public decimal SumResult { get { return this.result; } } public decimal AverageResult { get { return this.result / (decimal) this.cnt; } } public decimal MinimumResult { get { return this.result; } } public decimal MaximumResult { get { return this.result; } } public bool IsEmpty { get { return this.cnt == 0; } } } ////// Computes aggregates over a sequence of Double values. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct DoubleAggregator { private double result; private int cnt; public void Create() { this.cnt = 0; } public void Sum(double value) { if (this.cnt == 0) { this.result = value; this.cnt = 1; } else { this.result += value; } } public void Average(double value) { if (this.cnt == 0) this.result = value; else this.result += value; this.cnt++; } public void Minimum(double value) { if (this.cnt == 0 || value < this.result || double.IsNaN(value)) this.result = value; this.cnt = 1; } public void Maximum(double value) { if (this.cnt == 0 || value > this.result || double.IsNaN(value)) this.result = value; this.cnt = 1; } public double SumResult { get { return this.result; } } public double AverageResult { get { return this.result / (double) this.cnt; } } public double MinimumResult { get { return this.result; } } public double MaximumResult { get { return this.result; } } public bool IsEmpty { get { return this.cnt == 0; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
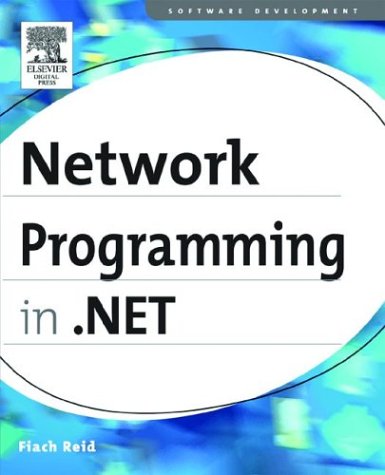
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EncryptedKey.cs
- XslAst.cs
- CultureInfo.cs
- X509Extension.cs
- SQlBooleanStorage.cs
- PersistChildrenAttribute.cs
- AspProxy.cs
- ArglessEventHandlerProxy.cs
- WebServiceData.cs
- util.cs
- ItemContainerPattern.cs
- ComponentEvent.cs
- DetailsViewDeleteEventArgs.cs
- DataTableNewRowEvent.cs
- XmlConvert.cs
- TagPrefixInfo.cs
- ContainerControlDesigner.cs
- KeyEventArgs.cs
- HttpCapabilitiesEvaluator.cs
- PageSettings.cs
- TemplatedAdorner.cs
- SettingsSection.cs
- MachinePropertyVariants.cs
- Floater.cs
- XmlIlGenerator.cs
- SemanticAnalyzer.cs
- ResXBuildProvider.cs
- SqlProvider.cs
- ObjectDataSourceStatusEventArgs.cs
- Vector3DConverter.cs
- EndSelectCardRequest.cs
- StreamUpgradeProvider.cs
- CompoundFileStreamReference.cs
- ActivationArguments.cs
- TextEditorParagraphs.cs
- IsolationInterop.cs
- DataServiceException.cs
- TableCell.cs
- RootBrowserWindow.cs
- Evidence.cs
- WindowsStatic.cs
- SecurityRuntime.cs
- FilteredDataSetHelper.cs
- ellipse.cs
- ImageAutomationPeer.cs
- MarkupExtensionParser.cs
- WriteFileContext.cs
- PolygonHotSpot.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- Window.cs
- DivideByZeroException.cs
- ClientRoleProvider.cs
- RMPublishingDialog.cs
- SubpageParagraph.cs
- Message.cs
- IItemProperties.cs
- BulletedListEventArgs.cs
- ValidationEventArgs.cs
- CommentAction.cs
- BinHexDecoder.cs
- DynamicValueConverter.cs
- InputGestureCollection.cs
- RewritingValidator.cs
- IndentedWriter.cs
- DotExpr.cs
- CalculatedColumn.cs
- ModelUIElement3D.cs
- TemplatedAdorner.cs
- DrawingAttributesDefaultValueFactory.cs
- EnumCodeDomSerializer.cs
- ToolStripControlHost.cs
- ExpressionPrefixAttribute.cs
- bindurihelper.cs
- InternalSafeNativeMethods.cs
- ListBindingHelper.cs
- PartitionResolver.cs
- OLEDB_Enum.cs
- DataGridComboBoxColumn.cs
- IdentityValidationException.cs
- Group.cs
- IdentityHolder.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- RemoteWebConfigurationHost.cs
- CodeParameterDeclarationExpression.cs
- Parser.cs
- ControlPropertyNameConverter.cs
- Util.cs
- GlyphingCache.cs
- GridView.cs
- BrowsableAttribute.cs
- SqlConnectionPoolGroupProviderInfo.cs
- BrowserCapabilitiesCompiler.cs
- HashAlgorithm.cs
- AttributedMetaModel.cs
- XsdValidatingReader.cs
- WindowsTitleBar.cs
- ToolTipAutomationPeer.cs
- StreamGeometry.cs
- SharedConnectionWorkflowTransactionService.cs
- FeedUtils.cs