Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Internal / IO / Packaging / EncryptedPackageFilter.cs / 1 / EncryptedPackageFilter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implements indexing filter for EncryptedPackageEnvelope. // Invoked by XpsFilter if the file/stream being filtered // is an EncryptedPackageEnvelope. // // History: // 07/18/2005: ArindamB: Initial implementation //--------------------------------------------------------------------------- using System; using System.Windows; using System.Runtime.InteropServices; using System.IO.Packaging; using MS.Internal.Interop; namespace MS.Internal.IO.Packaging { #region EncryptedPackageFilter ////// Implements IFilter methods to support indexing on EncryptedPackageEnvelope. /// internal class EncryptedPackageFilter : IFilter { #region Constructor ////// Constructor. /// /// EncryptedPackageEnvelope to filter on internal EncryptedPackageFilter(EncryptedPackageEnvelope encryptedPackage) { if (encryptedPackage == null) { throw new ArgumentNullException("encryptedPackage"); } // // Since CorePropertiesFilter is implemented as // a managed filter (supports IManagedFilter interface), // IndexingFilterMarshaler is used to get IFilter interface out of it. // _filter = new IndexingFilterMarshaler( new CorePropertiesFilter( encryptedPackage.PackageProperties )); } #endregion Constructor #region IFilter methods ////// Initialzes the session for this filter. /// /// usage flags /// number of elements in aAttributes array /// array of FULLPROPSPEC structs to restrict responses ///IFILTER_FLAGS_NONE. Return value is effectively ignored by the caller. public IFILTER_FLAGS Init( [In] IFILTER_INIT grfFlags, [In] uint cAttributes, [In, MarshalAs(UnmanagedType.LPArray, SizeParamIndex = 1)] FULLPROPSPEC[] aAttributes) { return _filter.Init(grfFlags, cAttributes, aAttributes); } ////// Returns description of the next chunk. /// ///Chunk descriptor public STAT_CHUNK GetChunk() { return _filter.GetChunk(); } ////// Gets text content corresponding to current chunk. /// /// /// ///Not supported in indexing of core properties. public void GetText(ref uint bufCharacterCount, IntPtr pBuffer) { throw new COMException(SR.Get(SRID.FilterGetTextNotSupported), (int)FilterErrorCode.FILTER_E_NO_TEXT); } ////// Gets the property value corresponding to current chunk. /// ///property value public IntPtr GetValue() { return _filter.GetValue(); } ////// Retrieves an interface representing the specified portion of the object. /// /// /// ///Not implemented. Reserved for future use. public IntPtr BindRegion([In] FILTERREGION origPos, [In] ref Guid riid) { throw new NotImplementedException(SR.Get(SRID.FilterBindRegionNotImplemented)); } #endregion IFilter methods #region Fields ////// Only filtering that is supported on EncryptedPackageEnvelope /// is of core properties. This points to EncryptedPackageCorePropertiesFilter /// wrapped by FilterMarshaler. /// private IFilter _filter = null; #endregion Fields } #endregion EncryptedPackageFilter } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implements indexing filter for EncryptedPackageEnvelope. // Invoked by XpsFilter if the file/stream being filtered // is an EncryptedPackageEnvelope. // // History: // 07/18/2005: ArindamB: Initial implementation //--------------------------------------------------------------------------- using System; using System.Windows; using System.Runtime.InteropServices; using System.IO.Packaging; using MS.Internal.Interop; namespace MS.Internal.IO.Packaging { #region EncryptedPackageFilter ////// Implements IFilter methods to support indexing on EncryptedPackageEnvelope. /// internal class EncryptedPackageFilter : IFilter { #region Constructor ////// Constructor. /// /// EncryptedPackageEnvelope to filter on internal EncryptedPackageFilter(EncryptedPackageEnvelope encryptedPackage) { if (encryptedPackage == null) { throw new ArgumentNullException("encryptedPackage"); } // // Since CorePropertiesFilter is implemented as // a managed filter (supports IManagedFilter interface), // IndexingFilterMarshaler is used to get IFilter interface out of it. // _filter = new IndexingFilterMarshaler( new CorePropertiesFilter( encryptedPackage.PackageProperties )); } #endregion Constructor #region IFilter methods ////// Initialzes the session for this filter. /// /// usage flags /// number of elements in aAttributes array /// array of FULLPROPSPEC structs to restrict responses ///IFILTER_FLAGS_NONE. Return value is effectively ignored by the caller. public IFILTER_FLAGS Init( [In] IFILTER_INIT grfFlags, [In] uint cAttributes, [In, MarshalAs(UnmanagedType.LPArray, SizeParamIndex = 1)] FULLPROPSPEC[] aAttributes) { return _filter.Init(grfFlags, cAttributes, aAttributes); } ////// Returns description of the next chunk. /// ///Chunk descriptor public STAT_CHUNK GetChunk() { return _filter.GetChunk(); } ////// Gets text content corresponding to current chunk. /// /// /// ///Not supported in indexing of core properties. public void GetText(ref uint bufCharacterCount, IntPtr pBuffer) { throw new COMException(SR.Get(SRID.FilterGetTextNotSupported), (int)FilterErrorCode.FILTER_E_NO_TEXT); } ////// Gets the property value corresponding to current chunk. /// ///property value public IntPtr GetValue() { return _filter.GetValue(); } ////// Retrieves an interface representing the specified portion of the object. /// /// /// ///Not implemented. Reserved for future use. public IntPtr BindRegion([In] FILTERREGION origPos, [In] ref Guid riid) { throw new NotImplementedException(SR.Get(SRID.FilterBindRegionNotImplemented)); } #endregion IFilter methods #region Fields ////// Only filtering that is supported on EncryptedPackageEnvelope /// is of core properties. This points to EncryptedPackageCorePropertiesFilter /// wrapped by FilterMarshaler. /// private IFilter _filter = null; #endregion Fields } #endregion EncryptedPackageFilter } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
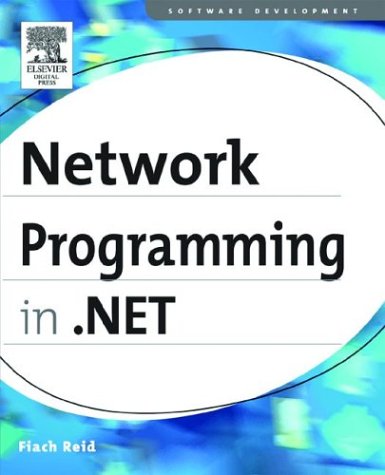
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AttachedAnnotation.cs
- UpdateProgress.cs
- ClientConfigPaths.cs
- DataGridTable.cs
- CombinedGeometry.cs
- WebPartMovingEventArgs.cs
- Pair.cs
- SqlDataAdapter.cs
- XmlDsigSep2000.cs
- RuntimeWrappedException.cs
- PriorityBindingExpression.cs
- ViewValidator.cs
- RegexRunnerFactory.cs
- SimpleParser.cs
- ImageInfo.cs
- TaskFileService.cs
- TextBox.cs
- XmlSchemaSimpleContentExtension.cs
- ChtmlSelectionListAdapter.cs
- SafePEFileHandle.cs
- OracleConnectionFactory.cs
- DataBindingHandlerAttribute.cs
- DataGridTextBox.cs
- ProvidePropertyAttribute.cs
- HMACSHA1.cs
- AstNode.cs
- ImageAnimator.cs
- AccessViolationException.cs
- DesignerProperties.cs
- ArraySubsetEnumerator.cs
- XmlCDATASection.cs
- Soap.cs
- RadioButtonAutomationPeer.cs
- InfoCardBaseException.cs
- GlobalizationSection.cs
- ButtonColumn.cs
- EventLog.cs
- errorpatternmatcher.cs
- Peer.cs
- DataRelationCollection.cs
- GeometryGroup.cs
- CompilerState.cs
- SemanticResultValue.cs
- OdbcCommand.cs
- BindValidationContext.cs
- SafeLibraryHandle.cs
- UrlMappingCollection.cs
- ResourcesBuildProvider.cs
- Run.cs
- ComponentResourceKeyConverter.cs
- Highlights.cs
- OutputCacheSettingsSection.cs
- SelectionUIHandler.cs
- UInt32.cs
- SID.cs
- PTUtility.cs
- SQLSingle.cs
- WebHttpDispatchOperationSelector.cs
- SafeThemeHandle.cs
- WSSecurityXXX2005.cs
- QueryStringParameter.cs
- PersonalizationProviderCollection.cs
- RegexWriter.cs
- RowBinding.cs
- JoinSymbol.cs
- CommonProperties.cs
- TransformCollection.cs
- DurableMessageDispatchInspector.cs
- EntityTypeEmitter.cs
- TrustSection.cs
- ClickablePoint.cs
- SR.Designer.cs
- Line.cs
- ConfigurationConverterBase.cs
- CurrencyWrapper.cs
- MissingSatelliteAssemblyException.cs
- Path.cs
- EffectiveValueEntry.cs
- RawMouseInputReport.cs
- FileUtil.cs
- MultiPageTextView.cs
- UniqueIdentifierService.cs
- ProvidersHelper.cs
- PreviewPrintController.cs
- StoragePropertyMapping.cs
- PerformanceCounterPermissionEntryCollection.cs
- GridViewRow.cs
- InheritanceRules.cs
- EventManager.cs
- ParsedRoute.cs
- NamespaceQuery.cs
- _SSPISessionCache.cs
- BaseHashHelper.cs
- DbParameterHelper.cs
- OracleFactory.cs
- CommunicationException.cs
- EventToken.cs
- TypographyProperties.cs
- KeyValuePairs.cs
- ADMembershipUser.cs