Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media3D / Generated / ScaleTransform3D.cs / 2 / ScaleTransform3D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see [....]/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { sealed partial class ScaleTransform3D : AffineTransform3D { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new ScaleTransform3D Clone() { return (ScaleTransform3D)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new ScaleTransform3D CloneCurrentValue() { return (ScaleTransform3D)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ private static void ScaleXPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedScaleXValue = (double)e.NewValue; target.PropertyChanged(ScaleXProperty); } private static void ScaleYPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedScaleYValue = (double)e.NewValue; target.PropertyChanged(ScaleYProperty); } private static void ScaleZPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedScaleZValue = (double)e.NewValue; target.PropertyChanged(ScaleZProperty); } private static void CenterXPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedCenterXValue = (double)e.NewValue; target.PropertyChanged(CenterXProperty); } private static void CenterYPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedCenterYValue = (double)e.NewValue; target.PropertyChanged(CenterYProperty); } private static void CenterZPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ScaleTransform3D target = ((ScaleTransform3D) d); target._cachedCenterZValue = (double)e.NewValue; target.PropertyChanged(CenterZProperty); } #region Public Properties ////// ScaleX - double. Default value is 1.0. /// public double ScaleX { get { ReadPreamble(); return _cachedScaleXValue; } set { SetValueInternal(ScaleXProperty, value); } } ////// ScaleY - double. Default value is 1.0. /// public double ScaleY { get { ReadPreamble(); return _cachedScaleYValue; } set { SetValueInternal(ScaleYProperty, value); } } ////// ScaleZ - double. Default value is 1.0. /// public double ScaleZ { get { ReadPreamble(); return _cachedScaleZValue; } set { SetValueInternal(ScaleZProperty, value); } } ////// CenterX - double. Default value is 0.0. /// public double CenterX { get { ReadPreamble(); return _cachedCenterXValue; } set { SetValueInternal(CenterXProperty, value); } } ////// CenterY - double. Default value is 0.0. /// public double CenterY { get { ReadPreamble(); return _cachedCenterYValue; } set { SetValueInternal(CenterYProperty, value); } } ////// CenterZ - double. Default value is 0.0. /// public double CenterZ { get { ReadPreamble(); return _cachedCenterZValue; } set { SetValueInternal(CenterZProperty, value); } } #endregion Public Properties //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new ScaleTransform3D(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Obtain handles for animated properties DUCE.ResourceHandle hScaleXAnimations = GetAnimationResourceHandle(ScaleXProperty, channel); DUCE.ResourceHandle hScaleYAnimations = GetAnimationResourceHandle(ScaleYProperty, channel); DUCE.ResourceHandle hScaleZAnimations = GetAnimationResourceHandle(ScaleZProperty, channel); DUCE.ResourceHandle hCenterXAnimations = GetAnimationResourceHandle(CenterXProperty, channel); DUCE.ResourceHandle hCenterYAnimations = GetAnimationResourceHandle(CenterYProperty, channel); DUCE.ResourceHandle hCenterZAnimations = GetAnimationResourceHandle(CenterZProperty, channel); // Pack & send command packet DUCE.MILCMD_SCALETRANSFORM3D data; unsafe { data.Type = MILCMD.MilCmdScaleTransform3D; data.Handle = _duceResource.GetHandle(channel); if (hScaleXAnimations.IsNull) { data.scaleX = ScaleX; } data.hScaleXAnimations = hScaleXAnimations; if (hScaleYAnimations.IsNull) { data.scaleY = ScaleY; } data.hScaleYAnimations = hScaleYAnimations; if (hScaleZAnimations.IsNull) { data.scaleZ = ScaleZ; } data.hScaleZAnimations = hScaleZAnimations; if (hCenterXAnimations.IsNull) { data.centerX = CenterX; } data.hCenterXAnimations = hCenterXAnimations; if (hCenterYAnimations.IsNull) { data.centerY = CenterY; } data.hCenterYAnimations = hCenterYAnimations; if (hCenterZAnimations.IsNull) { data.centerZ = CenterZ; } data.hCenterZAnimations = hCenterZAnimations; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_SCALETRANSFORM3D)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_SCALETRANSFORM3D)) { AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the ScaleTransform3D.ScaleX property. /// public static readonly DependencyProperty ScaleXProperty = RegisterProperty("ScaleX", typeof(double), typeof(ScaleTransform3D), 1.0, new PropertyChangedCallback(ScaleXPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ////// The DependencyProperty for the ScaleTransform3D.ScaleY property. /// public static readonly DependencyProperty ScaleYProperty = RegisterProperty("ScaleY", typeof(double), typeof(ScaleTransform3D), 1.0, new PropertyChangedCallback(ScaleYPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ////// The DependencyProperty for the ScaleTransform3D.ScaleZ property. /// public static readonly DependencyProperty ScaleZProperty = RegisterProperty("ScaleZ", typeof(double), typeof(ScaleTransform3D), 1.0, new PropertyChangedCallback(ScaleZPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ////// The DependencyProperty for the ScaleTransform3D.CenterX property. /// public static readonly DependencyProperty CenterXProperty = RegisterProperty("CenterX", typeof(double), typeof(ScaleTransform3D), 0.0, new PropertyChangedCallback(CenterXPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ////// The DependencyProperty for the ScaleTransform3D.CenterY property. /// public static readonly DependencyProperty CenterYProperty = RegisterProperty("CenterY", typeof(double), typeof(ScaleTransform3D), 0.0, new PropertyChangedCallback(CenterYPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ////// The DependencyProperty for the ScaleTransform3D.CenterZ property. /// public static readonly DependencyProperty CenterZProperty = RegisterProperty("CenterZ", typeof(double), typeof(ScaleTransform3D), 0.0, new PropertyChangedCallback(CenterZPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); #endregion Dependency Properties //------------------------------------------------------ // // Internal Fields // //----------------------------------------------------- #region Internal Fields private double _cachedScaleXValue = 1.0; private double _cachedScaleYValue = 1.0; private double _cachedScaleZValue = 1.0; private double _cachedCenterXValue = 0.0; private double _cachedCenterYValue = 0.0; private double _cachedCenterZValue = 0.0; internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal const double c_ScaleX = 1.0; internal const double c_ScaleY = 1.0; internal const double c_ScaleZ = 1.0; internal const double c_CenterX = 0.0; internal const double c_CenterY = 0.0; internal const double c_CenterZ = 0.0; #endregion Internal Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
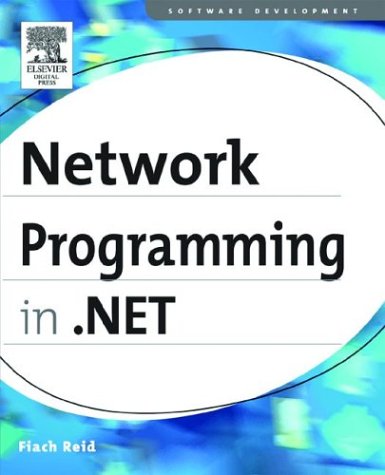
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebAdminConfigurationHelper.cs
- Expressions.cs
- AssociationSetEnd.cs
- ContentFilePart.cs
- ProfileService.cs
- StringSorter.cs
- RecipientServiceModelSecurityTokenRequirement.cs
- SmiConnection.cs
- AuthenticationModuleElement.cs
- securitycriticaldataformultiplegetandset.cs
- UriTemplateTrieNode.cs
- AudioSignalProblemOccurredEventArgs.cs
- ParameterBuilder.cs
- XsdDataContractExporter.cs
- OleDbParameter.cs
- AppDomainManager.cs
- OptimalBreakSession.cs
- SystemFonts.cs
- ComponentRenameEvent.cs
- PageRequestManager.cs
- KnownBoxes.cs
- FileController.cs
- RC2.cs
- ManifestResourceInfo.cs
- WebServiceEnumData.cs
- ComponentRenameEvent.cs
- RootDesignerSerializerAttribute.cs
- RowsCopiedEventArgs.cs
- MatrixIndependentAnimationStorage.cs
- ListViewItemSelectionChangedEvent.cs
- SafeCryptContextHandle.cs
- VisualTreeUtils.cs
- Control.cs
- TypeRestriction.cs
- Subtract.cs
- MSG.cs
- DrawToolTipEventArgs.cs
- EncoderReplacementFallback.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- InvokeSchedule.cs
- SafeNativeMethodsMilCoreApi.cs
- VersionUtil.cs
- BindingCollection.cs
- SqlUserDefinedTypeAttribute.cs
- RegexCapture.cs
- ScalarOps.cs
- EntityDataSourceView.cs
- CategoryNameCollection.cs
- ConnectionStringsSection.cs
- Color.cs
- DropDownButton.cs
- OutputWindow.cs
- MetaChildrenColumn.cs
- SystemIPInterfaceProperties.cs
- HttpCapabilitiesBase.cs
- ICspAsymmetricAlgorithm.cs
- InternalTypeHelper.cs
- TdsValueSetter.cs
- InputDevice.cs
- CreateUserErrorEventArgs.cs
- GeneralTransform2DTo3D.cs
- ZoneIdentityPermission.cs
- TableLayoutCellPaintEventArgs.cs
- CodeAccessPermission.cs
- _HeaderInfoTable.cs
- BitmapSource.cs
- SiteMapDataSourceDesigner.cs
- _KerberosClient.cs
- HostTimeoutsElement.cs
- DataServiceException.cs
- XmlSerializerSection.cs
- MemberPathMap.cs
- ListParaClient.cs
- LocationInfo.cs
- SimpleHandlerBuildProvider.cs
- InfocardExtendedInformationEntry.cs
- NativeMethods.cs
- CheckBox.cs
- OrderedDictionary.cs
- MobileDeviceCapabilitiesSectionHandler.cs
- HtmlTernaryTree.cs
- CryptoHandle.cs
- FileChangesMonitor.cs
- EventRouteFactory.cs
- Misc.cs
- Renderer.cs
- SafeRegistryHandle.cs
- GetCertificateRequest.cs
- SortExpressionBuilder.cs
- OdbcConnectionOpen.cs
- RenderCapability.cs
- Transform3D.cs
- ContextDataSource.cs
- HtmlControlPersistable.cs
- ObjectHelper.cs
- DateTimeFormat.cs
- MatrixTransform3D.cs
- BinaryFormatter.cs
- CmsUtils.cs
- DataServiceClientException.cs