Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / PtsHost / ListParaClient.cs / 1 / ListParaClient.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ListParaClient.cs // // Description: ListParaClient is responsible for handling display // related data of list paragraphs. // // History: // 02/07/2004 : ghermann - created. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Security; using System.Windows; using System.Windows.Media; using System.Windows.Documents; using MS.Internal.Documents; using MS.Internal.Text; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { ////// ListParaClient is responsible for handling display related data /// of break paragraphs. /// internal sealed class ListParaClient : ContainerParaClient { ////// Constructor. /// /// /// ListParagraph associated with this para client. /// internal ListParaClient(ListParagraph paragraph) : base(paragraph) { } ////// Validate visual node associated with paragraph. /// /// /// Inherited update info /// ////// Critical - as this calls Critical function PTS.FsQuerySubtrackDetails /// and some PtsHelper functions. /// Safe - operation is safe and method doesn't disclose any information got through critical calls /// [SecurityCritical, SecurityTreatAsSafe] internal override void ValidateVisual(PTS.FSKUPDATE fskupdInherited) { // Query paragraph details PTS.FSSUBTRACKDETAILS subtrackDetails; PTS.Validate(PTS.FsQuerySubtrackDetails(PtsContext.Context, _paraHandle.Value, out subtrackDetails)); // Draw border and background info. MbpInfo mbp = MbpInfo.FromElement(Paragraph.Element); if (ThisFlowDirection != PageFlowDirection) { mbp.MirrorBP(); } uint fswdir = PTS.FlowDirectionToFswdir((FlowDirection)Paragraph.Element.GetValue(FrameworkElement.FlowDirectionProperty)); Brush backgroundBrush = (Brush)Paragraph.Element.GetValue(TextElement.BackgroundProperty); TextProperties textProperties = new TextProperties(Paragraph.Element, StaticTextPointer.Null, false /* inline objects */, false /* get background */); // There might be possibility to get empty sub-track, skip the sub-track in such case. if (subtrackDetails.cParas != 0) { PTS.FSPARADESCRIPTION [] arrayParaDesc; PtsHelper.ParaListFromSubtrack(PtsContext, _paraHandle.Value, ref subtrackDetails, out arrayParaDesc); using(DrawingContext ctx = _visual.RenderOpen()) { _visual.DrawBackgroundAndBorderIntoContext(ctx, backgroundBrush, mbp.BorderBrush, mbp.Border, _rect.FromTextDpi(), IsFirstChunk, IsLastChunk); // Get list of paragraphs ListMarkerLine listMarkerLine = new ListMarkerLine(Paragraph.StructuralCache.TextFormatterHost, this); int indexFirstParaInSubtrack = 0; for(int index = 0; index < subtrackDetails.cParas; index++) { List list = Paragraph.Element as List; BaseParaClient listItemParaClient = PtsContext.HandleToObject(arrayParaDesc[index].pfsparaclient) as BaseParaClient; PTS.ValidateHandle(listItemParaClient); if(index == 0) { indexFirstParaInSubtrack = list.GetListItemIndex(listItemParaClient.Paragraph.Element as ListItem); } if(listItemParaClient.IsFirstChunk) { int dvBaseline = listItemParaClient.GetFirstTextLineBaseline(); if(PageFlowDirection != ThisFlowDirection) { ctx.PushTransform(new MatrixTransform(-1.0, 0.0, 0.0, 1.0, TextDpi.FromTextDpi(2 * listItemParaClient.Rect.u + listItemParaClient.Rect.du), 0.0)); } int adjustedIndex; if (int.MaxValue - index < indexFirstParaInSubtrack) { adjustedIndex = int.MaxValue; } else { adjustedIndex = indexFirstParaInSubtrack + index; } LineProperties lineProps = new LineProperties(Paragraph.Element, Paragraph.StructuralCache.FormattingOwner, textProperties, new MarkerProperties(list, adjustedIndex)); listMarkerLine.FormatAndDrawVisual(ctx, lineProps, listItemParaClient.Rect.u, dvBaseline); if(PageFlowDirection != ThisFlowDirection) { ctx.Pop(); } } } listMarkerLine.Dispose(); } // Render list of paragraphs PtsHelper.UpdateParaListVisuals(PtsContext, _visual.Children, fskupdInherited, arrayParaDesc); } else { _visual.Children.Clear(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ListParaClient.cs // // Description: ListParaClient is responsible for handling display // related data of list paragraphs. // // History: // 02/07/2004 : ghermann - created. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Security; using System.Windows; using System.Windows.Media; using System.Windows.Documents; using MS.Internal.Documents; using MS.Internal.Text; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { ////// ListParaClient is responsible for handling display related data /// of break paragraphs. /// internal sealed class ListParaClient : ContainerParaClient { ////// Constructor. /// /// /// ListParagraph associated with this para client. /// internal ListParaClient(ListParagraph paragraph) : base(paragraph) { } ////// Validate visual node associated with paragraph. /// /// /// Inherited update info /// ////// Critical - as this calls Critical function PTS.FsQuerySubtrackDetails /// and some PtsHelper functions. /// Safe - operation is safe and method doesn't disclose any information got through critical calls /// [SecurityCritical, SecurityTreatAsSafe] internal override void ValidateVisual(PTS.FSKUPDATE fskupdInherited) { // Query paragraph details PTS.FSSUBTRACKDETAILS subtrackDetails; PTS.Validate(PTS.FsQuerySubtrackDetails(PtsContext.Context, _paraHandle.Value, out subtrackDetails)); // Draw border and background info. MbpInfo mbp = MbpInfo.FromElement(Paragraph.Element); if (ThisFlowDirection != PageFlowDirection) { mbp.MirrorBP(); } uint fswdir = PTS.FlowDirectionToFswdir((FlowDirection)Paragraph.Element.GetValue(FrameworkElement.FlowDirectionProperty)); Brush backgroundBrush = (Brush)Paragraph.Element.GetValue(TextElement.BackgroundProperty); TextProperties textProperties = new TextProperties(Paragraph.Element, StaticTextPointer.Null, false /* inline objects */, false /* get background */); // There might be possibility to get empty sub-track, skip the sub-track in such case. if (subtrackDetails.cParas != 0) { PTS.FSPARADESCRIPTION [] arrayParaDesc; PtsHelper.ParaListFromSubtrack(PtsContext, _paraHandle.Value, ref subtrackDetails, out arrayParaDesc); using(DrawingContext ctx = _visual.RenderOpen()) { _visual.DrawBackgroundAndBorderIntoContext(ctx, backgroundBrush, mbp.BorderBrush, mbp.Border, _rect.FromTextDpi(), IsFirstChunk, IsLastChunk); // Get list of paragraphs ListMarkerLine listMarkerLine = new ListMarkerLine(Paragraph.StructuralCache.TextFormatterHost, this); int indexFirstParaInSubtrack = 0; for(int index = 0; index < subtrackDetails.cParas; index++) { List list = Paragraph.Element as List; BaseParaClient listItemParaClient = PtsContext.HandleToObject(arrayParaDesc[index].pfsparaclient) as BaseParaClient; PTS.ValidateHandle(listItemParaClient); if(index == 0) { indexFirstParaInSubtrack = list.GetListItemIndex(listItemParaClient.Paragraph.Element as ListItem); } if(listItemParaClient.IsFirstChunk) { int dvBaseline = listItemParaClient.GetFirstTextLineBaseline(); if(PageFlowDirection != ThisFlowDirection) { ctx.PushTransform(new MatrixTransform(-1.0, 0.0, 0.0, 1.0, TextDpi.FromTextDpi(2 * listItemParaClient.Rect.u + listItemParaClient.Rect.du), 0.0)); } int adjustedIndex; if (int.MaxValue - index < indexFirstParaInSubtrack) { adjustedIndex = int.MaxValue; } else { adjustedIndex = indexFirstParaInSubtrack + index; } LineProperties lineProps = new LineProperties(Paragraph.Element, Paragraph.StructuralCache.FormattingOwner, textProperties, new MarkerProperties(list, adjustedIndex)); listMarkerLine.FormatAndDrawVisual(ctx, lineProps, listItemParaClient.Rect.u, dvBaseline); if(PageFlowDirection != ThisFlowDirection) { ctx.Pop(); } } } listMarkerLine.Dispose(); } // Render list of paragraphs PtsHelper.UpdateParaListVisuals(PtsContext, _visual.Children, fskupdInherited, arrayParaDesc); } else { _visual.Children.Clear(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
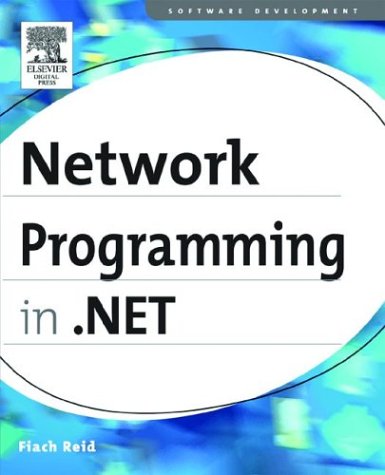
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolTip.cs
- UpdateTranslator.cs
- ParagraphResult.cs
- GridViewAutoFormat.cs
- DrawingContextDrawingContextWalker.cs
- ProxyAttribute.cs
- TableCellCollection.cs
- SelectingProviderEventArgs.cs
- ScrollProviderWrapper.cs
- XmlNodeWriter.cs
- VerificationAttribute.cs
- HtmlElement.cs
- MembershipValidatePasswordEventArgs.cs
- DataConnectionHelper.cs
- SwitchElementsCollection.cs
- WebPartMenuStyle.cs
- CompoundFileStorageReference.cs
- DiagnosticsConfigurationHandler.cs
- PresentationSource.cs
- NTAccount.cs
- UidManager.cs
- ResourceContainerWrapper.cs
- RawStylusInputCustomData.cs
- ClientEventManager.cs
- InvalidOleVariantTypeException.cs
- BrowserCapabilitiesFactoryBase.cs
- DispatcherEventArgs.cs
- UIElement3D.cs
- WeakEventTable.cs
- SamlAttributeStatement.cs
- Flowchart.cs
- VarRefManager.cs
- DoubleAnimation.cs
- X509InitiatorCertificateClientElement.cs
- SpecialNameAttribute.cs
- TextOnlyOutput.cs
- EntityWithChangeTrackerStrategy.cs
- BordersPage.cs
- StylusCaptureWithinProperty.cs
- TreeViewItemAutomationPeer.cs
- FixedSOMContainer.cs
- TextDecorations.cs
- IImplicitResourceProvider.cs
- Scanner.cs
- ResolveCompletedEventArgs.cs
- PromptBuilder.cs
- ExpressionsCollectionEditor.cs
- KeyNotFoundException.cs
- ToolStripDropDownMenu.cs
- PropertyItemInternal.cs
- ImageMap.cs
- OperationContractAttribute.cs
- WrappedOptions.cs
- DataGridRow.cs
- ItemCollection.cs
- EncryptedKey.cs
- SqlDataSourceStatusEventArgs.cs
- WebPartTransformer.cs
- GradientStop.cs
- ButtonRenderer.cs
- WebResourceUtil.cs
- NamespaceQuery.cs
- Literal.cs
- DropSource.cs
- MdiWindowListItemConverter.cs
- GeometryConverter.cs
- DisplayInformation.cs
- DaylightTime.cs
- Odbc32.cs
- PictureBoxDesigner.cs
- RelationshipManager.cs
- ProfilePropertySettings.cs
- SmtpReplyReaderFactory.cs
- ClockController.cs
- Merger.cs
- ValidationVisibilityAttribute.cs
- WebPartZoneCollection.cs
- IdnElement.cs
- PermissionRequestEvidence.cs
- ListViewAutomationPeer.cs
- WaitHandleCannotBeOpenedException.cs
- SystemIPGlobalProperties.cs
- ArrayItemValue.cs
- ReflectEventDescriptor.cs
- WebUtil.cs
- ColumnResult.cs
- SetStoryboardSpeedRatio.cs
- DecimalConverter.cs
- ShaperBuffers.cs
- DirectionalLight.cs
- Rect3D.cs
- HiddenField.cs
- XsltQilFactory.cs
- HasCopySemanticsAttribute.cs
- IIS7WorkerRequest.cs
- TrackingExtract.cs
- LocatorBase.cs
- XmlAttributeCache.cs
- ManualResetEvent.cs
- XmlSchemas.cs