Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Providers / ResourceContainerWrapper.cs / 1305376 / ResourceContainerWrapper.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Wrapper class for a resource set. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { #region Namespaces. using System; using System.Collections.Generic; using System.Diagnostics; using System.Reflection; #endregion Namespaces. ////// Wrapper class for a resource set. A resource set object can be shared across services, /// this wrapper class contains the resouce set information and also service specific /// information about that resource set. /// [DebuggerDisplay("{Name}: {ResourceType}")] internal class ResourceSetWrapper { #region Fields ///Reference to the wrapped resource set private readonly ResourceSet resourceSet; ///Reference to the wrapped resource type. private readonly ResourceType resourceType; ///Access rights to this resource set. private EntitySetRights rights; ///Page Size for this resource set. private int pageSize; ///Methods to be called when composing read queries to allow authorization. private MethodInfo[] readAuthorizationMethods; ///Methods to be called when validating write methods to allow authorization. private MethodInfo[] writeAuthorizationMethods; ///Whether the types contained in the set has mappings for friendly feeds are V1 compatible or not private bool? epmIsV1Compatible; #if DEBUG ///Is true, if the resource set is fully initialized and validated. No more changes can be made once its set to readonly. private bool isReadOnly; #endif #endregion Fields #region Constructors ////// Constructs a new ResourceSetWrapper instance using the ResourceSet instance to be enclosed. /// /// ResourceSet instance to be wrapped by the current instance /// Resource type (normalized to a single instance by the caller). public ResourceSetWrapper(ResourceSet resourceSet, ResourceType resourceType) { Debug.Assert(resourceSet != null, "resourceSet != null"); if (!resourceSet.IsReadOnly) { throw new DataServiceException(500, Strings.DataServiceProviderWrapper_ResourceContainerNotReadonly(resourceSet.Name)); } this.resourceSet = resourceSet; this.resourceType = resourceType; } #endregion Constructors #region Properties ///Name of the resource set. public string Name { get { return this.resourceSet.Name; } } ///Reference to resource type that this resource set is a collection of public ResourceType ResourceType { get { return this.resourceType; } } ///Whether the resource set is visible to service consumers. public bool IsVisible { get { #if DEBUG Debug.Assert(this.isReadOnly, "IsVisible - entity set settings not initialized."); #endif return this.rights != EntitySetRights.None; } } ///Access rights to this resource set. public EntitySetRights Rights { get { #if DEBUG Debug.Assert(this.isReadOnly, "Rights - entity set settings not initialized."); #endif return this.rights; } } ///Page Size for this resource set. public int PageSize { get { #if DEBUG Debug.Assert(this.isReadOnly, "Rights - entity set settings not initialized."); #endif return this.pageSize; } } ///Retursn the list of query interceptors for this set (possibly null). public MethodInfo[] QueryInterceptors { [DebuggerStepThrough] get { #if DEBUG Debug.Assert(this.isReadOnly, "QueryInterceptors - entity set settings not initialized."); #endif return this.readAuthorizationMethods; } } ///Returns the list of change interceptors for this set (possible null). public MethodInfo[] ChangeInterceptors { [DebuggerStepThrough] get { #if DEBUG Debug.Assert(this.isReadOnly, "ChangeInterceptors - entity set settings not initialized."); #endif return this.writeAuthorizationMethods; } } ///Returns the wrapped resource set instance. internal ResourceSet ResourceSet { [DebuggerStepThrough] get { #if DEBUG Debug.Assert(this.resourceSet != null, "this.resourceSet != null"); #endif return this.resourceSet; } } #endregion Properties #region Methods ////// Apply the given configuration to the resource set. /// /// data service configuration instance. public void ApplyConfiguration(DataServiceConfiguration configuration) { #if DEBUG Debug.Assert(!this.isReadOnly, "Can only apply the configuration once."); #endif // Set entity set rights this.rights = configuration.GetResourceSetRights(this.resourceSet); // Set page size this.pageSize = configuration.GetResourceSetPageSize(this.resourceSet); if (this.pageSize < 0) { throw new DataServiceException(500, Strings.DataService_SDP_PageSizeMustbeNonNegative(this.pageSize, this.Name)); } // Add QueryInterceptors this.readAuthorizationMethods = configuration.GetReadAuthorizationMethods(this.resourceSet); // Add ChangeInterceptors this.writeAuthorizationMethods = configuration.GetWriteAuthorizationMethods(this.resourceSet); #if DEBUG this.isReadOnly = true; #endif } ///Whether the types contained in the set has mappings for friendly feeds are V1 compatible or not /// Data service provider instance. ///False if there's any type in this set which has friendly feed mappings with KeepInContent=false. True otherwise. internal bool EpmIsV1Compatible(DataServiceProviderWrapper provider) { #if DEBUG Debug.Assert(provider != null, "provider != null"); Debug.Assert(this.resourceSet != null, "this.resourceSet != null"); Debug.Assert(this.isReadOnly, "EpmIsV1Compatible - entity set settings not initialized."); #endif if (!this.epmIsV1Compatible.HasValue) { // Go through all types contained in the set. If any one type is EpmIsV1Compatible == false, // the whole set is EpmIsV1Compatible=false. ResourceType baseType = this.resourceSet.ResourceType; bool isV1Compatible = baseType.EpmIsV1Compatible; // If the base type is not epm v1 compatible or it has no derived type, we need not look any further. if (isV1Compatible && provider.HasDerivedTypes(baseType)) { foreach (ResourceType derivedType in provider.GetDerivedTypes(baseType)) { if (!derivedType.EpmIsV1Compatible) { // We can stop as soon as we find the first type that is not epm v1 compatible. isV1Compatible = false; break; } } } this.epmIsV1Compatible = isV1Compatible; } return this.epmIsV1Compatible.Value; } #endregion Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
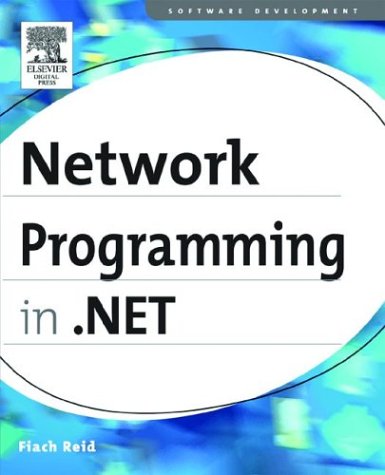
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RootProfilePropertySettingsCollection.cs
- Matrix3D.cs
- ProtectedConfigurationProviderCollection.cs
- relpropertyhelper.cs
- AddInEnvironment.cs
- Effect.cs
- PointIndependentAnimationStorage.cs
- SynchronizedDispatch.cs
- PointConverter.cs
- XmlnsCache.cs
- CorrelationToken.cs
- QuaternionIndependentAnimationStorage.cs
- TypedRowHandler.cs
- StateBag.cs
- InsufficientExecutionStackException.cs
- Base64Decoder.cs
- IsolatedStorageFile.cs
- DataSourceControl.cs
- DeviceContexts.cs
- FactoryRecord.cs
- ImmutableCollection.cs
- TreeView.cs
- SQLInt32.cs
- EventKeyword.cs
- WebPartZone.cs
- ObjectNotFoundException.cs
- ChooseAction.cs
- ExpressionPrinter.cs
- SystemDiagnosticsSection.cs
- LinkGrep.cs
- WebServiceTypeData.cs
- IDReferencePropertyAttribute.cs
- CharacterBuffer.cs
- ToolboxControl.cs
- NativeRightsManagementAPIsStructures.cs
- CodeTypeParameterCollection.cs
- LicFileLicenseProvider.cs
- M3DUtil.cs
- CancellationHandlerDesigner.cs
- AutoGeneratedField.cs
- DynamicDiscoSearcher.cs
- ToolStripCustomTypeDescriptor.cs
- _TransmitFileOverlappedAsyncResult.cs
- DotExpr.cs
- MimePart.cs
- TextDataBindingHandler.cs
- Math.cs
- TimeSpanValidatorAttribute.cs
- Solver.cs
- IPAddress.cs
- XmlSchemaAttribute.cs
- storepermissionattribute.cs
- RuleInfoComparer.cs
- WpfKnownType.cs
- PageBorderless.cs
- CounterCreationDataCollection.cs
- ComponentCollection.cs
- FactoryGenerator.cs
- PtsPage.cs
- ProvidersHelper.cs
- CommentGlyph.cs
- DataGridAddNewRow.cs
- DesignTimeTemplateParser.cs
- AtomMaterializerLog.cs
- EntryPointNotFoundException.cs
- TextSpanModifier.cs
- XmlSchemaAttributeGroup.cs
- XamlDesignerSerializationManager.cs
- Int64Animation.cs
- CultureInfoConverter.cs
- MsmqIntegrationMessagePool.cs
- PersonalizationStateInfoCollection.cs
- CodeEventReferenceExpression.cs
- IsolatedStorageException.cs
- SqlCommandSet.cs
- DataObject.cs
- DetailsViewUpdateEventArgs.cs
- PinnedBufferMemoryStream.cs
- ButtonBase.cs
- RegexFCD.cs
- VBCodeProvider.cs
- Timeline.cs
- EngineSiteSapi.cs
- GridViewAutomationPeer.cs
- PublisherMembershipCondition.cs
- RadialGradientBrush.cs
- WorkflowViewStateService.cs
- BindingCompleteEventArgs.cs
- Ray3DHitTestResult.cs
- ConstrainedGroup.cs
- ButtonBaseAutomationPeer.cs
- SessionStateSection.cs
- DeclarativeConditionsCollection.cs
- SelectionRange.cs
- HtmlMeta.cs
- HTTPNotFoundHandler.cs
- ToolStripPanelRow.cs
- controlskin.cs
- VisualTreeUtils.cs
- SessionPageStatePersister.cs