Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / RunTime / CorrelationToken.cs / 1305376 / CorrelationToken.cs
using System; using System.Xml; using System.ComponentModel; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Collections.Specialized; using System.Diagnostics; using System.Runtime.Serialization; using System.Workflow.ComponentModel; using System.Workflow.ComponentModel.Design; using System.Workflow.ComponentModel.Serialization; using System.Workflow.Runtime; using System.Globalization; namespace System.Workflow.Runtime { [DesignerSerializer(typeof(DependencyObjectCodeDomSerializer), typeof(CodeDomSerializer))] public sealed class CorrelationToken : DependencyObject, IPropertyValueProvider { internal static readonly DependencyProperty NameProperty = DependencyProperty.Register("Name", typeof(string), typeof(CorrelationToken), new PropertyMetadata(DependencyPropertyOptions.Metadata, new Attribute[] { new BrowsableAttribute(false)})); internal static readonly DependencyProperty OwnerActivityNameProperty = DependencyProperty.Register("OwnerActivityName", typeof(string), typeof(CorrelationToken), new PropertyMetadata(DependencyPropertyOptions.Metadata, new Attribute[] { new TypeConverterAttribute(typeof(PropertyValueProviderTypeConverter)) })); // instance properties internal static readonly DependencyProperty PropertiesProperty = DependencyProperty.Register("Properties", typeof(ICollection), typeof(CorrelationToken),new PropertyMetadata(new Attribute[] { new BrowsableAttribute(false), new DesignerSerializationVisibilityAttribute(DesignerSerializationVisibility.Hidden)})); internal static readonly DependencyProperty SubscriptionsProperty = DependencyProperty.Register("Subscriptions", typeof(IList >), typeof(CorrelationToken)); internal static readonly DependencyProperty InitializedProperty = DependencyProperty.Register("Initialized", typeof(bool), typeof(CorrelationToken), new PropertyMetadata(false, new Attribute[] { new BrowsableAttribute(false), new DesignerSerializationVisibilityAttribute(DesignerSerializationVisibility.Hidden) })); public CorrelationToken() { } public CorrelationToken(string name) { this.Name = name; } [Browsable(false)] public string Name { get { return (string)GetValue(NameProperty); } set { SetValue(NameProperty, value); } } [TypeConverter(typeof(PropertyValueProviderTypeConverter))] public string OwnerActivityName { get { return (string)GetValue(OwnerActivityNameProperty); } set { SetValue(OwnerActivityNameProperty, value); } } [Browsable(false)] [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public ICollection Properties { get { return GetValue(PropertiesProperty) as ICollection ; } } [Browsable(false)] [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public bool Initialized { get { return (bool)GetValue(InitializedProperty); } } ICollection IPropertyValueProvider.GetPropertyValues(ITypeDescriptorContext context) { StringCollection names = new StringCollection(); if (string.Equals(context.PropertyDescriptor.Name, "OwnerActivityName", StringComparison.Ordinal)) { ISelectionService selectionService = context.GetService(typeof(ISelectionService)) as ISelectionService; if (selectionService != null && selectionService.SelectionCount == 1 && selectionService.PrimarySelection is Activity) { Activity currentActivity = selectionService.PrimarySelection as Activity; foreach (Activity activity in GetEnclosingCompositeActivities(currentActivity)) { string activityId = activity.QualifiedName; if (!names.Contains(activityId)) names.Add(activityId); } } } return names; } public void Initialize(Activity activity, ICollection propertyValues) { if (this.Initialized) throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture,ExecutionStringManager.CorrelationAlreadyInitialized, this.Name)); SetValue(PropertiesProperty,propertyValues); // fire correlation initialized events CorrelationTokenEventArgs eventArgs = new CorrelationTokenEventArgs(this, true); IList > subscribers = GetValue(SubscriptionsProperty) as IList >; if (subscribers != null) { foreach (ActivityExecutorDelegateInfo subscriber in subscribers) { subscriber.InvokeDelegate(ContextActivityUtils.ContextActivity(activity), eventArgs, true, false); } } SetValue(InitializedProperty,true); WorkflowTrace.Runtime.TraceEvent(TraceEventType.Information, 0, "CorrelationToken initialized for {0} owner activity {1} ", this.Name, this.OwnerActivityName); } internal void Uninitialize(Activity activity) { SetValue(PropertiesProperty, null); // fire correlation uninitialized events CorrelationTokenEventArgs eventArgs = new CorrelationTokenEventArgs(this,false); IList > subscribers = GetValue(SubscriptionsProperty) as IList >; if (subscribers != null) { ActivityExecutorDelegateInfo [] clonedSubscribers = new ActivityExecutorDelegateInfo [subscribers.Count]; subscribers.CopyTo(clonedSubscribers, 0); foreach (ActivityExecutorDelegateInfo subscriber in clonedSubscribers) { subscriber.InvokeDelegate(ContextActivityUtils.ContextActivity(activity), eventArgs, true, false); } } //SetValue(InitializedProperty, false); WorkflowTrace.Runtime.TraceEvent(TraceEventType.Information, 0, "CorrelationToken Uninitialized for {0} owner activity {1}", this.Name, this.OwnerActivityName); } public void SubscribeForCorrelationTokenInitializedEvent(Activity activity, IActivityEventListener dataChangeListener) { if (dataChangeListener == null) throw new ArgumentNullException("dataChangeListener"); if (activity == null) throw new ArgumentNullException("activity"); ActivityExecutorDelegateInfo subscriber = new ActivityExecutorDelegateInfo (dataChangeListener, ContextActivityUtils.ContextActivity(activity), true); IList > subscriptions = GetValue(SubscriptionsProperty) as IList >; if (subscriptions == null) { subscriptions = new List >(); SetValue(SubscriptionsProperty,subscriptions); } subscriptions.Add(subscriber); } public void UnsubscribeFromCorrelationTokenInitializedEvent(Activity activity, IActivityEventListener dataChangeListener) { if (dataChangeListener == null) throw new ArgumentNullException("dataChangeListener"); if (activity == null) throw new ArgumentNullException("activity"); ActivityExecutorDelegateInfo subscriber = new ActivityExecutorDelegateInfo (dataChangeListener, ContextActivityUtils.ContextActivity(activity), true); IList > subscriptions = GetValue(SubscriptionsProperty) as IList >; if (subscriptions != null) { subscriptions.Remove(subscriber); } } private static IEnumerable GetEnclosingCompositeActivities(Activity startActivity) { Activity currentActivity = null; Stack activityStack = new Stack (); activityStack.Push(startActivity); while ((currentActivity = activityStack.Pop()) != null) { if ((typeof(CompositeActivity).IsAssignableFrom(currentActivity.GetType())) && currentActivity.Enabled) { yield return currentActivity; } activityStack.Push(currentActivity.Parent); } yield break; } } [Serializable] public sealed class CorrelationTokenCollection : KeyedCollection { public static readonly DependencyProperty CorrelationTokenCollectionProperty = DependencyProperty.RegisterAttached("CorrelationTokenCollection", typeof(CorrelationTokenCollection), typeof(CorrelationTokenCollection)); public CorrelationTokenCollection() { } public CorrelationToken GetItem(string key) { return this[key]; } protected override string GetKeyForItem(CorrelationToken item) { return item.Name; } protected override void ClearItems() { base.ClearItems(); } protected override void InsertItem(int index, CorrelationToken item) { if (item == null) throw new ArgumentNullException("item"); base.InsertItem(index, item); } protected override void RemoveItem(int index) { base.RemoveItem(index); } protected override void SetItem(int index, CorrelationToken item) { if (item == null) throw new ArgumentNullException("item"); base.SetItem(index, item); } internal static void UninitializeCorrelationTokens(Activity activity) { CorrelationTokenCollection collection = activity.GetValue(CorrelationTokenCollectionProperty) as CorrelationTokenCollection; if (collection != null) { foreach (CorrelationToken correlator in collection) { correlator.Uninitialize(activity); } } } public static CorrelationToken GetCorrelationToken(Activity activity, string correlationTokenName, string ownerActivityName) { if (null == correlationTokenName) throw new ArgumentNullException("correlationTokenName"); if (null == ownerActivityName) throw new ArgumentNullException("ownerActivityName"); if (null == activity) throw new ArgumentNullException("activity"); Activity contextActivity = ContextActivityUtils.ContextActivity(activity); Activity owner = null; if (!String.IsNullOrEmpty(ownerActivityName)) { while (contextActivity != null) { owner = contextActivity.GetActivityByName(ownerActivityName, true); if (owner != null) break; contextActivity = ContextActivityUtils.ParentContextActivity(contextActivity); } if (owner == null) owner = Helpers.ParseActivityForBind(activity, ownerActivityName); } if (owner == null) throw new InvalidOperationException(ExecutionStringManager.OwnerActivityMissing); CorrelationTokenCollection collection = owner.GetValue(CorrelationTokenCollectionProperty) as CorrelationTokenCollection; if (collection == null) { collection = new CorrelationTokenCollection(); owner.SetValue(CorrelationTokenCollection.CorrelationTokenCollectionProperty, collection); } if (!collection.Contains(correlationTokenName)) { collection.Add(new CorrelationToken(correlationTokenName)); } return collection[correlationTokenName]; } } [Serializable] public class CorrelationProperty { private object value = null; private string name; public CorrelationProperty(string name, object value) { if (null == name) { throw new ArgumentNullException("name"); } this.name = name; this.value = value; } public object Value { get { return this.value; } } public string Name { get { return this.name; } } } public sealed class CorrelationTokenEventArgs : EventArgs { CorrelationToken correlator; bool initialized; internal CorrelationTokenEventArgs(CorrelationToken correlator, bool initialized) { this.correlator = correlator; this.initialized = initialized; } public bool IsInitializing { get { return this.initialized; } } public CorrelationToken CorrelationToken { get { return this.correlator; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
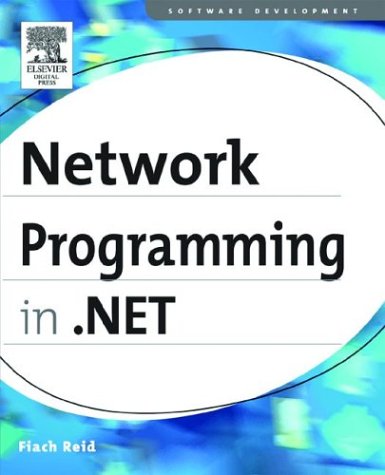
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HashUtility.cs
- Bezier.cs
- FileLoadException.cs
- BaseTemplateBuildProvider.cs
- DateTimeOffset.cs
- DataGridViewTopLeftHeaderCell.cs
- SHA384Managed.cs
- ColorBuilder.cs
- ErrorHandler.cs
- TaskHelper.cs
- MaskPropertyEditor.cs
- BindUriHelper.cs
- AssociatedControlConverter.cs
- ApplicationBuildProvider.cs
- PointCollection.cs
- ObjectAnimationBase.cs
- XamlUtilities.cs
- CfgParser.cs
- ToolStripItemEventArgs.cs
- OletxTransactionManager.cs
- FixedFindEngine.cs
- XmlQueryTypeFactory.cs
- DeferredSelectedIndexReference.cs
- XmlNamedNodeMap.cs
- InteropBitmapSource.cs
- DetailsViewRow.cs
- FilterQueryOptionExpression.cs
- HttpProfileBase.cs
- FolderNameEditor.cs
- CurrentChangingEventArgs.cs
- SafeRightsManagementHandle.cs
- SqlCommand.cs
- DataGridViewControlCollection.cs
- HandoffBehavior.cs
- TabControl.cs
- ImageConverter.cs
- DataSourceCacheDurationConverter.cs
- WebConfigurationHostFileChange.cs
- SqlReorderer.cs
- _AuthenticationState.cs
- ListSourceHelper.cs
- DataGridTextBoxColumn.cs
- BamlTreeUpdater.cs
- httpserverutility.cs
- SQLRoleProvider.cs
- BuildManager.cs
- ImpersonateTokenRef.cs
- _ProxyRegBlob.cs
- VisualTreeUtils.cs
- AdapterUtil.cs
- DetailsViewRow.cs
- OdbcFactory.cs
- PerformanceCounterCategory.cs
- Tokenizer.cs
- SmiGettersStream.cs
- DbCommandDefinition.cs
- SQLDateTime.cs
- DesignTimeTemplateParser.cs
- GeneralTransform.cs
- TextReader.cs
- TextAutomationPeer.cs
- TraceInternal.cs
- SourceChangedEventArgs.cs
- DataGridViewUtilities.cs
- TextRange.cs
- ThrowHelper.cs
- TripleDES.cs
- UdpAnnouncementEndpoint.cs
- ellipse.cs
- TableAdapterManagerGenerator.cs
- RoutedEventArgs.cs
- ConstraintStruct.cs
- StylusDownEventArgs.cs
- PageHandlerFactory.cs
- UTF8Encoding.cs
- EventLogLink.cs
- Typography.cs
- AlignmentYValidation.cs
- Pkcs9Attribute.cs
- LoadRetryHandler.cs
- OleDbParameter.cs
- InvokeWebServiceDesigner.cs
- Logging.cs
- GridViewRowCollection.cs
- TextViewBase.cs
- HideDisabledControlAdapter.cs
- SliderAutomationPeer.cs
- AttributeTable.cs
- DataSourceCache.cs
- XmlTypeAttribute.cs
- BitmapMetadataBlob.cs
- IpcChannelHelper.cs
- DateTimeOffsetStorage.cs
- BrowserCapabilitiesCodeGenerator.cs
- SerializationSectionGroup.cs
- Wizard.cs
- WorkflowTimerService.cs
- mil_commands.cs
- WebConfigurationHostFileChange.cs
- TextParagraphView.cs