Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ArraySet.cs / 2 / ArraySet.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// a set, collection of unordered, distinct objects, implemented as an array // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Linq; ///a set, collection of unordered, distinct objects, implemented as an array ///element type [DebuggerDisplay("Count = {count}")] internal struct ArraySet: IEnumerable where T : class { /// item array of T private T[] items; ///count of elements in the items array private int count; ///number of Add and RemoveAt operations private int version; ////// array set with an intial capacity /// /// initial capacity public ArraySet(int capacity) { this.items = new T[capacity]; this.count = 0; this.version = 0; } ///count of elements in the set public int Count { get { return this.count; } } ///get an item from an index in the set /// index to access public T this[int index] { get { Debug.Assert(index < this.count); return this.items[index]; } } ///add new element to the set /// element to add /// equality comparison function to avoid duplicates ///true if actually added, false if a duplicate was discovered public bool Add(T item, FuncequalityComparer) { if ((null != equalityComparer) && this.Contains(item, equalityComparer)) { return false; } int index = this.count++; if ((null == this.items) || (index == this.items.Length)) { // grow array in size, with minimum size being 32 Array.Resize (ref this.items, Math.Min(Math.Max(index, 16), Int32.MaxValue / 2) * 2); } this.items[index] = item; unchecked { this.version++; } return true; } /// is the element contained within the set /// item to find /// comparer ///true if the element is contained public bool Contains(T item, FuncequalityComparer) { return (0 <= this.IndexOf(item, equalityComparer)); } /// /// enumerator /// ///enumerator public IEnumeratorGetEnumerator() { for (int i = 0; i < this.count; ++i) { yield return this.items[i]; } } /// /// enumerator /// ///enumerator System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return this.GetEnumerator(); } ///Find the current index of element within the set /// item to find /// comparision function ///index of the item else (-1) public int IndexOf(T item, Funccomparer) { return this.IndexOf(item, IdentitySelect, comparer); } /// Find the current index of element within the set ///selected type /// item to find /// selector for item to compare /// item to compare ///index of the item else (-1) public int IndexOf(K item, Func select, Func comparer) { T[] array = this.items; if (null != array) { int length = this.count; for (int i = 0; i < length; ++i) { if (comparer(item, select(array[i]))) { return i; } } } return -1; } /// Remove the matched item from within the set /// item to find within the set /// comparer to find item to remove ///the item that was actually contained else its default public T Remove(T item, FuncequalityComparer) { int index = this.IndexOf(item, equalityComparer); if (0 <= index) { item = this.items[index]; this.RemoveAt(index); return item; } return default(T); } /// Remove an item at a specific index from within the set /// index of item to remove within the set public void RemoveAt(int index) { Debug.Assert(unchecked((uint)index < (uint)this.count), "index out of range"); T[] array = this.items; int lastIndex = --this.count; array[index] = array[lastIndex]; array[lastIndex] = default(T); if ((0 == lastIndex) && (256 <= array.Length)) { this.items = null; } else if ((256 < array.Length) && (lastIndex < array.Length / 4)) { // shrink to half size when count is a quarter Array.Resize(ref this.items, array.Length / 2); } unchecked { this.version++; } } ///Sort array based on selected value out of item being stored ///selected type /// selector /// comparer public void Sort(Func selector, Func comparer) { if (null != this.items) { SelectorComparer scomp; scomp.Selector = selector; scomp.Comparer = comparer; Array.Sort (this.items, 0, this.count, scomp); } } /// identity selector, returns self /// input ///output private static T IdentitySelect(T arg) { return arg; } ///Compare selected value out of t ///comparison type private struct SelectorComparer: IComparer { /// Select something out of T internal FuncSelector; /// Comparer of selected value internal FuncComparer; /// Compare /// x /// y ///int int IComparer.Compare(T x, T y) { return this.Comparer(this.Selector(x), this.Selector(y)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // //// a set, collection of unordered, distinct objects, implemented as an array // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Linq; ///a set, collection of unordered, distinct objects, implemented as an array ///element type [DebuggerDisplay("Count = {count}")] internal struct ArraySet: IEnumerable where T : class { /// item array of T private T[] items; ///count of elements in the items array private int count; ///number of Add and RemoveAt operations private int version; ////// array set with an intial capacity /// /// initial capacity public ArraySet(int capacity) { this.items = new T[capacity]; this.count = 0; this.version = 0; } ///count of elements in the set public int Count { get { return this.count; } } ///get an item from an index in the set /// index to access public T this[int index] { get { Debug.Assert(index < this.count); return this.items[index]; } } ///add new element to the set /// element to add /// equality comparison function to avoid duplicates ///true if actually added, false if a duplicate was discovered public bool Add(T item, FuncequalityComparer) { if ((null != equalityComparer) && this.Contains(item, equalityComparer)) { return false; } int index = this.count++; if ((null == this.items) || (index == this.items.Length)) { // grow array in size, with minimum size being 32 Array.Resize (ref this.items, Math.Min(Math.Max(index, 16), Int32.MaxValue / 2) * 2); } this.items[index] = item; unchecked { this.version++; } return true; } /// is the element contained within the set /// item to find /// comparer ///true if the element is contained public bool Contains(T item, FuncequalityComparer) { return (0 <= this.IndexOf(item, equalityComparer)); } /// /// enumerator /// ///enumerator public IEnumeratorGetEnumerator() { for (int i = 0; i < this.count; ++i) { yield return this.items[i]; } } /// /// enumerator /// ///enumerator System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return this.GetEnumerator(); } ///Find the current index of element within the set /// item to find /// comparision function ///index of the item else (-1) public int IndexOf(T item, Funccomparer) { return this.IndexOf(item, IdentitySelect, comparer); } /// Find the current index of element within the set ///selected type /// item to find /// selector for item to compare /// item to compare ///index of the item else (-1) public int IndexOf(K item, Func select, Func comparer) { T[] array = this.items; if (null != array) { int length = this.count; for (int i = 0; i < length; ++i) { if (comparer(item, select(array[i]))) { return i; } } } return -1; } /// Remove the matched item from within the set /// item to find within the set /// comparer to find item to remove ///the item that was actually contained else its default public T Remove(T item, FuncequalityComparer) { int index = this.IndexOf(item, equalityComparer); if (0 <= index) { item = this.items[index]; this.RemoveAt(index); return item; } return default(T); } /// Remove an item at a specific index from within the set /// index of item to remove within the set public void RemoveAt(int index) { Debug.Assert(unchecked((uint)index < (uint)this.count), "index out of range"); T[] array = this.items; int lastIndex = --this.count; array[index] = array[lastIndex]; array[lastIndex] = default(T); if ((0 == lastIndex) && (256 <= array.Length)) { this.items = null; } else if ((256 < array.Length) && (lastIndex < array.Length / 4)) { // shrink to half size when count is a quarter Array.Resize(ref this.items, array.Length / 2); } unchecked { this.version++; } } ///Sort array based on selected value out of item being stored ///selected type /// selector /// comparer public void Sort(Func selector, Func comparer) { if (null != this.items) { SelectorComparer scomp; scomp.Selector = selector; scomp.Comparer = comparer; Array.Sort (this.items, 0, this.count, scomp); } } /// identity selector, returns self /// input ///output private static T IdentitySelect(T arg) { return arg; } ///Compare selected value out of t ///comparison type private struct SelectorComparer: IComparer { /// Select something out of T internal FuncSelector; /// Comparer of selected value internal FuncComparer; /// Compare /// x /// y ///int int IComparer.Compare(T x, T y) { return this.Comparer(this.Selector(x), this.Selector(y)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
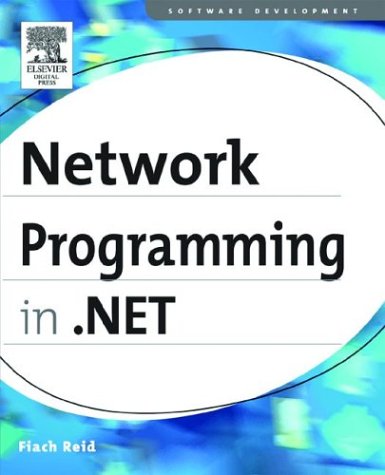
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigurationManagerInternalFactory.cs
- TextServicesHost.cs
- CollectionTypeElement.cs
- CodePageEncoding.cs
- ListenDesigner.cs
- UpdateTracker.cs
- validation.cs
- DataRowChangeEvent.cs
- LinqDataSourceDisposeEventArgs.cs
- DeclaredTypeValidator.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- IssuanceLicense.cs
- TextEditorCharacters.cs
- filewebrequest.cs
- ImageInfo.cs
- LinkArea.cs
- TriState.cs
- ListManagerBindingsCollection.cs
- ExpressionBindingCollection.cs
- SimpleTypesSurrogate.cs
- RegistrationContext.cs
- StringCollection.cs
- ValidationPropertyAttribute.cs
- DataBinder.cs
- DataGridToolTip.cs
- StateMachine.cs
- RuleSettings.cs
- KoreanLunisolarCalendar.cs
- BamlTreeMap.cs
- AutoCompleteStringCollection.cs
- DocumentGrid.cs
- GuidelineSet.cs
- HtmlForm.cs
- ChangeNode.cs
- ListCollectionView.cs
- DateTimeConstantAttribute.cs
- Directory.cs
- Point4DConverter.cs
- EntityUtil.cs
- MimePart.cs
- TCPClient.cs
- X509Extension.cs
- MaskedTextBoxDesignerActionList.cs
- TypeNameConverter.cs
- Message.cs
- BamlResourceDeserializer.cs
- DataBindingExpressionBuilder.cs
- TdsParserStaticMethods.cs
- DispatcherHookEventArgs.cs
- DataRowCollection.cs
- ExpressionBuilder.cs
- StrongTypingException.cs
- BlockUIContainer.cs
- TypeLoadException.cs
- DelimitedListTraceListener.cs
- ParenthesizePropertyNameAttribute.cs
- NativeMethodsOther.cs
- AlphaSortedEnumConverter.cs
- TaskHelper.cs
- ToolStripOverflowButton.cs
- ReturnValue.cs
- OpacityConverter.cs
- RegexEditorDialog.cs
- Viewport2DVisual3D.cs
- TableLayoutColumnStyleCollection.cs
- DataSourceBooleanViewSchemaConverter.cs
- RegexBoyerMoore.cs
- HtmlControlPersistable.cs
- MultiAsyncResult.cs
- WebPartHelpVerb.cs
- ToolStripContainerDesigner.cs
- SoapElementAttribute.cs
- EntitySet.cs
- CategoryNameCollection.cs
- XsdBuilder.cs
- SerializationFieldInfo.cs
- InputGestureCollection.cs
- OdbcParameter.cs
- TextSpan.cs
- ThicknessConverter.cs
- BamlLocalizableResource.cs
- Codec.cs
- ControlCachePolicy.cs
- OdbcConnectionPoolProviderInfo.cs
- MetabaseServerConfig.cs
- OracleParameter.cs
- MimeTypeAttribute.cs
- UpdateProgress.cs
- SetterBaseCollection.cs
- TrustManagerMoreInformation.cs
- PropertyMappingExceptionEventArgs.cs
- TransformerInfoCollection.cs
- AggregationMinMaxHelpers.cs
- TextFindEngine.cs
- SynchronousReceiveElement.cs
- TreeNodeEventArgs.cs
- AdornerLayer.cs
- Win32SafeHandles.cs
- BitmapSizeOptions.cs
- SelectionChangedEventArgs.cs