Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Configuration / System / Configuration / LongValidator.cs / 1 / LongValidator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.IO; using System.Reflection; using System.Security.Permissions; using System.Xml; using System.Collections.Specialized; using System.Globalization; using System.ComponentModel; using System.Security; using System.Text; using System.Text.RegularExpressions; namespace System.Configuration { public class LongValidator : ConfigurationValidatorBase { private enum ValidationFlags { None = 0x0000, ExclusiveRange = 0x0001, // If set the value must be outside of the range instead of inside } private ValidationFlags _flags = ValidationFlags.None; private long _minValue = long.MinValue; private long _maxValue = long.MaxValue; private long _resolution = 1; public LongValidator(long minValue, long maxValue) : this(minValue, maxValue, false, 1) { } public LongValidator(long minValue, long maxValue, bool rangeIsExclusive) : this(minValue, maxValue, rangeIsExclusive, 1) { } public LongValidator(long minValue, long maxValue, bool rangeIsExclusive, long resolution) { if (resolution <= 0) { throw new ArgumentOutOfRangeException("resolution"); } if (minValue > maxValue) { throw new ArgumentOutOfRangeException("minValue", SR.GetString(SR.Validator_min_greater_than_max)); } _minValue = minValue; _maxValue = maxValue; _resolution = resolution; _flags = rangeIsExclusive ? ValidationFlags.ExclusiveRange : ValidationFlags.None; } public override bool CanValidate(Type type) { return (type == typeof(long)); } public override void Validate(object value) { ValidatorUtils.HelperParamValidation(value, typeof(long)); ValidatorUtils.ValidateScalar((long)value, _minValue, _maxValue, _resolution, _flags == ValidationFlags.ExclusiveRange); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.IO; using System.Reflection; using System.Security.Permissions; using System.Xml; using System.Collections.Specialized; using System.Globalization; using System.ComponentModel; using System.Security; using System.Text; using System.Text.RegularExpressions; namespace System.Configuration { public class LongValidator : ConfigurationValidatorBase { private enum ValidationFlags { None = 0x0000, ExclusiveRange = 0x0001, // If set the value must be outside of the range instead of inside } private ValidationFlags _flags = ValidationFlags.None; private long _minValue = long.MinValue; private long _maxValue = long.MaxValue; private long _resolution = 1; public LongValidator(long minValue, long maxValue) : this(minValue, maxValue, false, 1) { } public LongValidator(long minValue, long maxValue, bool rangeIsExclusive) : this(minValue, maxValue, rangeIsExclusive, 1) { } public LongValidator(long minValue, long maxValue, bool rangeIsExclusive, long resolution) { if (resolution <= 0) { throw new ArgumentOutOfRangeException("resolution"); } if (minValue > maxValue) { throw new ArgumentOutOfRangeException("minValue", SR.GetString(SR.Validator_min_greater_than_max)); } _minValue = minValue; _maxValue = maxValue; _resolution = resolution; _flags = rangeIsExclusive ? ValidationFlags.ExclusiveRange : ValidationFlags.None; } public override bool CanValidate(Type type) { return (type == typeof(long)); } public override void Validate(object value) { ValidatorUtils.HelperParamValidation(value, typeof(long)); ValidatorUtils.ValidateScalar((long)value, _minValue, _maxValue, _resolution, _flags == ValidationFlags.ExclusiveRange); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
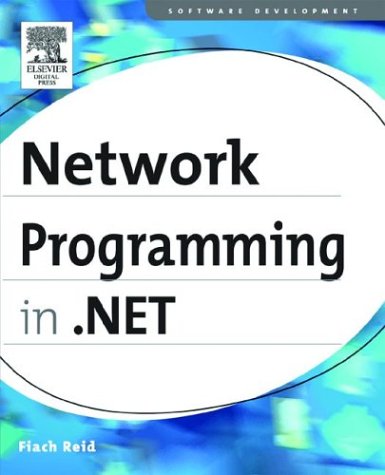
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeSystemMetrics.cs
- ModelChangedEventArgsImpl.cs
- XmlArrayItemAttributes.cs
- MenuItem.cs
- COM2Enum.cs
- ObjectTypeMapping.cs
- LayoutSettings.cs
- ScrollData.cs
- HtmlElementEventArgs.cs
- RepeaterItemCollection.cs
- TextRenderer.cs
- Transform.cs
- XpsManager.cs
- PageTheme.cs
- WebScriptMetadataMessageEncodingBindingElement.cs
- VisualProxy.cs
- IsolatedStorageFilePermission.cs
- AsyncPostBackErrorEventArgs.cs
- XmlMapping.cs
- EventPropertyMap.cs
- DrawTreeNodeEventArgs.cs
- EntityTransaction.cs
- XmlSchemaParticle.cs
- InputDevice.cs
- CLSCompliantAttribute.cs
- SrgsRule.cs
- Attributes.cs
- LinqDataSourceSelectEventArgs.cs
- MimeMapping.cs
- SemaphoreSlim.cs
- DebugInfoGenerator.cs
- DocumentGridPage.cs
- FrameworkContentElementAutomationPeer.cs
- WebPartDeleteVerb.cs
- BuilderInfo.cs
- AssemblyBuilder.cs
- WindowsToolbarAsMenu.cs
- QilInvoke.cs
- MailAddress.cs
- MaskPropertyEditor.cs
- LassoHelper.cs
- ContainerControl.cs
- MouseBinding.cs
- CodeIterationStatement.cs
- CodeObject.cs
- XmlSerializerSection.cs
- InstallerTypeAttribute.cs
- X509Certificate2Collection.cs
- SafeArrayRankMismatchException.cs
- TextDocumentView.cs
- SizeLimitedCache.cs
- EdgeProfileValidation.cs
- sqlmetadatafactory.cs
- Vector3DAnimationBase.cs
- EUCJPEncoding.cs
- sqlmetadatafactory.cs
- ByteAnimationUsingKeyFrames.cs
- UntypedNullExpression.cs
- DataGridViewAccessibleObject.cs
- ShapingEngine.cs
- x509utils.cs
- XmlUtilWriter.cs
- TextSelectionHighlightLayer.cs
- DrawingContextDrawingContextWalker.cs
- SizeChangedInfo.cs
- Misc.cs
- ParseChildrenAsPropertiesAttribute.cs
- ByteAnimationUsingKeyFrames.cs
- WindowsListViewGroupHelper.cs
- ECDiffieHellmanCng.cs
- TimeZoneInfo.cs
- VerticalAlignConverter.cs
- OleTxTransactionInfo.cs
- BinHexEncoder.cs
- Pair.cs
- UIElementIsland.cs
- HtmlTableCell.cs
- TagPrefixInfo.cs
- OdbcConnectionFactory.cs
- PagesSection.cs
- SynchronizationLockException.cs
- WmpBitmapEncoder.cs
- SiteMap.cs
- WebEventTraceProvider.cs
- QilInvoke.cs
- ConfigurationElement.cs
- DataViewSetting.cs
- BindableTemplateBuilder.cs
- OdbcException.cs
- ManagedWndProcTracker.cs
- PageHandlerFactory.cs
- ActivationArguments.cs
- ChannelRequirements.cs
- FileUtil.cs
- RectKeyFrameCollection.cs
- connectionpool.cs
- PointUtil.cs
- PolygonHotSpot.cs
- WorkflowServiceHost.cs
- DynamicQueryableWrapper.cs