Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Model / PropertyChange.cs / 1305376 / PropertyChange.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System; using System.Collections.Generic; using System.Text; using System.Globalization; using System.Runtime; class PropertyChange : ModelChange { public ModelItem Owner { get; set; } public string PropertyName { get; set; } public ModelItem OldValue { get; set; } public ModelItem NewValue { get; set; } public ModelTreeManager ModelTreeManager { get; set; } public override string Description { get { return string.Format(CultureInfo.InvariantCulture, "{0} - {1}", SR.PropertyChangeEditingScopeDescription, this.PropertyName); } } public override bool Apply() { Fx.Assert(this.ModelTreeManager != null, "Modeltreemanager cannot be null"); Fx.Assert(this.Owner != null, "Owner modelitem cannot be null"); Fx.Assert(!String.IsNullOrEmpty(this.PropertyName), " property name cannot be null or emptry"); ModelPropertyImpl dataModelProperty = (ModelPropertyImpl)this.Owner.Properties[this.PropertyName]; ModelItem oldValue = dataModelProperty.Value; if ((oldValue == null && this.NewValue == null) || (oldValue != null && this.NewValue != null && oldValue.GetCurrentValue().Equals(this.NewValue.GetCurrentValue()))) { return false; } dataModelProperty.SetValueCore(this.NewValue); this.ModelTreeManager.NotifyPropertyChange(dataModelProperty); return true; } public override Change GetInverse() { return new PropertyChange() { ModelTreeManager = this.ModelTreeManager, Owner = this.Owner, OldValue = this.NewValue, NewValue = this.OldValue, PropertyName = this.PropertyName }; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System; using System.Collections.Generic; using System.Text; using System.Globalization; using System.Runtime; class PropertyChange : ModelChange { public ModelItem Owner { get; set; } public string PropertyName { get; set; } public ModelItem OldValue { get; set; } public ModelItem NewValue { get; set; } public ModelTreeManager ModelTreeManager { get; set; } public override string Description { get { return string.Format(CultureInfo.InvariantCulture, "{0} - {1}", SR.PropertyChangeEditingScopeDescription, this.PropertyName); } } public override bool Apply() { Fx.Assert(this.ModelTreeManager != null, "Modeltreemanager cannot be null"); Fx.Assert(this.Owner != null, "Owner modelitem cannot be null"); Fx.Assert(!String.IsNullOrEmpty(this.PropertyName), " property name cannot be null or emptry"); ModelPropertyImpl dataModelProperty = (ModelPropertyImpl)this.Owner.Properties[this.PropertyName]; ModelItem oldValue = dataModelProperty.Value; if ((oldValue == null && this.NewValue == null) || (oldValue != null && this.NewValue != null && oldValue.GetCurrentValue().Equals(this.NewValue.GetCurrentValue()))) { return false; } dataModelProperty.SetValueCore(this.NewValue); this.ModelTreeManager.NotifyPropertyChange(dataModelProperty); return true; } public override Change GetInverse() { return new PropertyChange() { ModelTreeManager = this.ModelTreeManager, Owner = this.Owner, OldValue = this.NewValue, NewValue = this.OldValue, PropertyName = this.PropertyName }; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
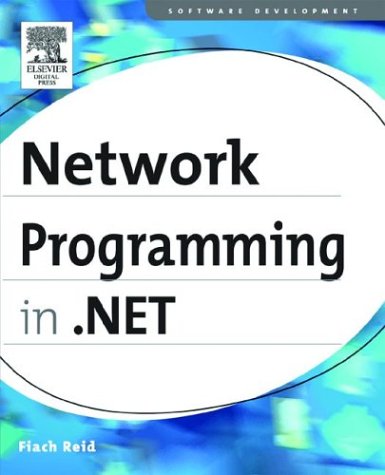
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DodSequenceMerge.cs
- AutomationPatternInfo.cs
- XmlNodeList.cs
- WebPartEditVerb.cs
- TypeSemantics.cs
- IntSecurity.cs
- MediaContext.cs
- ResourceDescriptionAttribute.cs
- IODescriptionAttribute.cs
- shaperfactory.cs
- NestedContainer.cs
- figurelength.cs
- EntityDesignerUtils.cs
- ProfessionalColorTable.cs
- SystemIcmpV4Statistics.cs
- ConsoleCancelEventArgs.cs
- TableRow.cs
- PropertyConverter.cs
- _NestedSingleAsyncResult.cs
- Journaling.cs
- Transform3DCollection.cs
- ThemeInfoAttribute.cs
- DesignerTransactionCloseEvent.cs
- ConsoleKeyInfo.cs
- SystemWebCachingSectionGroup.cs
- ListViewTableRow.cs
- EventKeyword.cs
- ChtmlPageAdapter.cs
- TrimSurroundingWhitespaceAttribute.cs
- PropertySourceInfo.cs
- DeclarativeCatalogPartDesigner.cs
- TrackingLocationCollection.cs
- BufferedGraphicsContext.cs
- DependencyPropertyValueSerializer.cs
- WebBrowserNavigatingEventHandler.cs
- ScalarConstant.cs
- PolicyChain.cs
- KeyBinding.cs
- XmlStreamStore.cs
- IQueryable.cs
- QueryContinueDragEvent.cs
- SmiTypedGetterSetter.cs
- iisPickupDirectory.cs
- Latin1Encoding.cs
- Atom10FormatterFactory.cs
- ContextQuery.cs
- BrowserInteropHelper.cs
- AdPostCacheSubstitution.cs
- SelectionChangedEventArgs.cs
- ColorConverter.cs
- TabControl.cs
- SuppressIldasmAttribute.cs
- XmlSchemaInfo.cs
- ITextView.cs
- AttributeData.cs
- MessageSmuggler.cs
- CodeDOMProvider.cs
- smtpconnection.cs
- StringDictionaryCodeDomSerializer.cs
- XmlMemberMapping.cs
- DetailsViewActionList.cs
- CompilerError.cs
- BindingListCollectionView.cs
- DynamicQueryableWrapper.cs
- QueryStatement.cs
- ApplicationSettingsBase.cs
- PriorityBindingExpression.cs
- CodeBlockBuilder.cs
- DbXmlEnabledProviderManifest.cs
- HwndProxyElementProvider.cs
- HwndSubclass.cs
- XmlDeclaration.cs
- AliasedSlot.cs
- LocalizableAttribute.cs
- RSACryptoServiceProvider.cs
- StreamInfo.cs
- SQLInt32Storage.cs
- LocalIdCollection.cs
- MultiView.cs
- PolicyLevel.cs
- InvokeProviderWrapper.cs
- SessionPageStatePersister.cs
- DataGridViewCellCollection.cs
- Bitmap.cs
- BookmarkTable.cs
- SecurityTokenSerializer.cs
- DelayedRegex.cs
- HandlerFactoryWrapper.cs
- XmlNamespaceManager.cs
- HtmlTextArea.cs
- ProfileServiceManager.cs
- MSHTMLHost.cs
- WindowsPen.cs
- XMLSchema.cs
- PixelFormatConverter.cs
- InputMethodStateChangeEventArgs.cs
- PropertyEmitter.cs
- GenerateTemporaryAssemblyTask.cs
- _LocalDataStoreMgr.cs
- ToolBarOverflowPanel.cs