Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / XamlBuildTask / Microsoft / Build / Tasks / Xaml / AttributeData.cs / 1305376 / AttributeData.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Xaml; using System.ComponentModel; using System.Runtime; namespace Microsoft.Build.Tasks.Xaml { sealed class AttributeData : Attribute { IListparameters; IList properties; public XamlType Type { get; set; } public IList Parameters { get { if (parameters == null) { parameters = new List (); } return parameters; } set { parameters = value; } } public IList Properties { get { if (properties == null) { properties = new List (); } return properties; } set { properties = value; } } // We get here when we are inside x:ClassAttributes or x:Property.Attributes. We expect the first element to be the Attribute SO. public static AttributeData LoadAttributeData(XamlReader reader, NamespaceTable namespaceTable, string rootNamespace) { AttributeData attributeData = null; reader.Read(); if (reader.NodeType == XamlNodeType.StartObject) { attributeData = new AttributeData { Type = reader.Type }; bool readNext = false; while (readNext || reader.Read()) { namespaceTable.ManageNamespace(reader); readNext = false; if (reader.NodeType == XamlNodeType.StartMember) { if (reader.Member == XamlLanguage.Arguments) { attributeData.Parameters = ReadParameters(reader.ReadSubtree(), namespaceTable, rootNamespace); readNext = true; } else if (!reader.Member.IsDirective) { AttributeParameterInfo propertyInfo = ReadAttributeProperty(reader.ReadSubtree(), namespaceTable, rootNamespace); attributeData.Properties.Add(propertyInfo); readNext = true; } } } } return attributeData; } // Read the Property on the attribute. private static AttributeParameterInfo ReadAttributeProperty(XamlReader reader, NamespaceTable namespaceTable, string rootNamespace) { reader.Read(); Fx.Assert(reader.Member != null, "Member element should not be null"); XamlMember member = reader.Member; AttributeParameterInfo propertyInfo = new AttributeParameterInfo(); propertyInfo.Name = member.Name; if (member.Type != null && !member.Type.IsUnknown) { propertyInfo.Type = member.Type.UnderlyingType; } ReadParamInfo(reader, namespaceTable, rootNamespace, propertyInfo); return propertyInfo; } // Read the parameters on the Attribute. We expect the parameters to be in the order in which they are supposed to appear in the output code. // Here we are inside x:Arguments and we expect a list of parameters. private static IList ReadParameters(XamlReader reader, NamespaceTable namespaceTable, string rootNamespace) { IList parameters = new List (); bool readNext = false; while (readNext || reader.Read()) { readNext = false; if (reader.NodeType == XamlNodeType.StartObject) { AttributeParameterInfo paramInfo = new AttributeParameterInfo(); ReadParamInfo(reader.ReadSubtree(), namespaceTable, rootNamespace, paramInfo); parameters.Add(paramInfo); readNext = true; } } return parameters; } // Read the actual parameter info, i.e. the type of the paramter and its value. // The first element could be a V or an SO. private static void ReadParamInfo(XamlReader reader, NamespaceTable namespaceTable, string rootNamespace, AttributeParameterInfo paramInfo) { reader.Read(); bool readNext = false; do { readNext = false; if (reader.NodeType == XamlNodeType.StartObject && reader.Type == XamlLanguage.Array) { paramInfo.IsArray = true; XamlReader xamlArrayReader = reader.ReadSubtree(); xamlArrayReader.Read(); while (readNext || xamlArrayReader.Read()) { readNext = false; if (xamlArrayReader.NodeType == XamlNodeType.StartMember && xamlArrayReader.Member.Name == "Type") { xamlArrayReader.Read(); if (xamlArrayReader.NodeType == XamlNodeType.Value) { XamlType arrayType = XamlBuildTaskServices.GetXamlTypeFromString(xamlArrayReader.Value as string, namespaceTable, xamlArrayReader.SchemaContext); paramInfo.Type = arrayType.UnderlyingType; } } else if (xamlArrayReader.NodeType == XamlNodeType.StartObject) { AttributeParameterInfo arrayEntry = new AttributeParameterInfo(); ReadParamInfo(xamlArrayReader.ReadSubtree(), namespaceTable, rootNamespace, arrayEntry); paramInfo.ArrayContents.Add(arrayEntry); readNext = true; } } } else if(reader.NodeType == XamlNodeType.StartObject || reader.NodeType == XamlNodeType.Value) { paramInfo.IsArray = false; string paramVal; Type paramType; GetParamValueType(reader.ReadSubtree(), paramInfo.Type, namespaceTable, rootNamespace, out paramVal, out paramType); paramInfo.Value = paramVal; paramInfo.Type = paramType; } } while (readNext || reader.Read()); } // Get the paramter value. If the value is enclosed inside nodes of the type, then get the parameter type as well. // Else infer the type from the type of the property. private static void GetParamValueType(XamlReader reader, Type type, NamespaceTable namespaceTable, string rootNamespace, out string paramValue, out Type paramType) { paramValue = String.Empty; paramType = type; while (reader.Read()) { if (reader.NodeType == XamlNodeType.Value) { if (paramType != null) { paramValue = GetParamValue(reader.Value as string, paramType, reader, namespaceTable, rootNamespace); } else { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.AttributeParameterTypeUnknown(reader.Value as string))); } } else if (reader.NodeType == XamlNodeType.StartObject) { if (reader.Type == XamlLanguage.Null) { paramValue = null; paramType = null; } else if (reader.Type == XamlLanguage.Type) { paramType = typeof(Type); } else { paramType = reader.Type.UnderlyingType; } } } } // Get the parameter value that is to be put in the generated code as is. private static string GetParamValue(string value, Type type, XamlReader reader, NamespaceTable namespaceTable, string rootNamespace) { string paramValue; if (type.IsEnum) { paramValue = type.FullName + "." + value; } else if (type.IsAssignableFrom(typeof(System.Type))) { XamlType typeName = XamlBuildTaskServices.GetXamlTypeFromString(value, namespaceTable, reader.SchemaContext); string clrTypeName; if (!XamlBuildTaskServices.TryGetClrTypeName(typeName, rootNamespace, out clrTypeName)) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.TypeNameUnknown(XamlBuildTaskServices.GetFullTypeName(typeName)))); } paramValue = clrTypeName; } else if (type == typeof(String)) { paramValue = value; } else if (type.IsPrimitive) { value = value.TrimStart('"'); value = value.TrimEnd('"'); if (type == typeof(bool)) { if (string.Compare(value, "true", StringComparison.OrdinalIgnoreCase) == 0) { paramValue = "true"; } else if (string.Compare(value, "false", StringComparison.OrdinalIgnoreCase) == 0) { paramValue = "false"; } else { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.UnknownBooleanValue(value))); } } else { paramValue = value; } } else { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.AttributeParamTypeNotSupported(type.FullName))); } return paramValue; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Xaml; using System.ComponentModel; using System.Runtime; namespace Microsoft.Build.Tasks.Xaml { sealed class AttributeData : Attribute { IList parameters; IList properties; public XamlType Type { get; set; } public IList Parameters { get { if (parameters == null) { parameters = new List (); } return parameters; } set { parameters = value; } } public IList Properties { get { if (properties == null) { properties = new List (); } return properties; } set { properties = value; } } // We get here when we are inside x:ClassAttributes or x:Property.Attributes. We expect the first element to be the Attribute SO. public static AttributeData LoadAttributeData(XamlReader reader, NamespaceTable namespaceTable, string rootNamespace) { AttributeData attributeData = null; reader.Read(); if (reader.NodeType == XamlNodeType.StartObject) { attributeData = new AttributeData { Type = reader.Type }; bool readNext = false; while (readNext || reader.Read()) { namespaceTable.ManageNamespace(reader); readNext = false; if (reader.NodeType == XamlNodeType.StartMember) { if (reader.Member == XamlLanguage.Arguments) { attributeData.Parameters = ReadParameters(reader.ReadSubtree(), namespaceTable, rootNamespace); readNext = true; } else if (!reader.Member.IsDirective) { AttributeParameterInfo propertyInfo = ReadAttributeProperty(reader.ReadSubtree(), namespaceTable, rootNamespace); attributeData.Properties.Add(propertyInfo); readNext = true; } } } } return attributeData; } // Read the Property on the attribute. private static AttributeParameterInfo ReadAttributeProperty(XamlReader reader, NamespaceTable namespaceTable, string rootNamespace) { reader.Read(); Fx.Assert(reader.Member != null, "Member element should not be null"); XamlMember member = reader.Member; AttributeParameterInfo propertyInfo = new AttributeParameterInfo(); propertyInfo.Name = member.Name; if (member.Type != null && !member.Type.IsUnknown) { propertyInfo.Type = member.Type.UnderlyingType; } ReadParamInfo(reader, namespaceTable, rootNamespace, propertyInfo); return propertyInfo; } // Read the parameters on the Attribute. We expect the parameters to be in the order in which they are supposed to appear in the output code. // Here we are inside x:Arguments and we expect a list of parameters. private static IList ReadParameters(XamlReader reader, NamespaceTable namespaceTable, string rootNamespace) { IList parameters = new List (); bool readNext = false; while (readNext || reader.Read()) { readNext = false; if (reader.NodeType == XamlNodeType.StartObject) { AttributeParameterInfo paramInfo = new AttributeParameterInfo(); ReadParamInfo(reader.ReadSubtree(), namespaceTable, rootNamespace, paramInfo); parameters.Add(paramInfo); readNext = true; } } return parameters; } // Read the actual parameter info, i.e. the type of the paramter and its value. // The first element could be a V or an SO. private static void ReadParamInfo(XamlReader reader, NamespaceTable namespaceTable, string rootNamespace, AttributeParameterInfo paramInfo) { reader.Read(); bool readNext = false; do { readNext = false; if (reader.NodeType == XamlNodeType.StartObject && reader.Type == XamlLanguage.Array) { paramInfo.IsArray = true; XamlReader xamlArrayReader = reader.ReadSubtree(); xamlArrayReader.Read(); while (readNext || xamlArrayReader.Read()) { readNext = false; if (xamlArrayReader.NodeType == XamlNodeType.StartMember && xamlArrayReader.Member.Name == "Type") { xamlArrayReader.Read(); if (xamlArrayReader.NodeType == XamlNodeType.Value) { XamlType arrayType = XamlBuildTaskServices.GetXamlTypeFromString(xamlArrayReader.Value as string, namespaceTable, xamlArrayReader.SchemaContext); paramInfo.Type = arrayType.UnderlyingType; } } else if (xamlArrayReader.NodeType == XamlNodeType.StartObject) { AttributeParameterInfo arrayEntry = new AttributeParameterInfo(); ReadParamInfo(xamlArrayReader.ReadSubtree(), namespaceTable, rootNamespace, arrayEntry); paramInfo.ArrayContents.Add(arrayEntry); readNext = true; } } } else if(reader.NodeType == XamlNodeType.StartObject || reader.NodeType == XamlNodeType.Value) { paramInfo.IsArray = false; string paramVal; Type paramType; GetParamValueType(reader.ReadSubtree(), paramInfo.Type, namespaceTable, rootNamespace, out paramVal, out paramType); paramInfo.Value = paramVal; paramInfo.Type = paramType; } } while (readNext || reader.Read()); } // Get the paramter value. If the value is enclosed inside nodes of the type, then get the parameter type as well. // Else infer the type from the type of the property. private static void GetParamValueType(XamlReader reader, Type type, NamespaceTable namespaceTable, string rootNamespace, out string paramValue, out Type paramType) { paramValue = String.Empty; paramType = type; while (reader.Read()) { if (reader.NodeType == XamlNodeType.Value) { if (paramType != null) { paramValue = GetParamValue(reader.Value as string, paramType, reader, namespaceTable, rootNamespace); } else { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.AttributeParameterTypeUnknown(reader.Value as string))); } } else if (reader.NodeType == XamlNodeType.StartObject) { if (reader.Type == XamlLanguage.Null) { paramValue = null; paramType = null; } else if (reader.Type == XamlLanguage.Type) { paramType = typeof(Type); } else { paramType = reader.Type.UnderlyingType; } } } } // Get the parameter value that is to be put in the generated code as is. private static string GetParamValue(string value, Type type, XamlReader reader, NamespaceTable namespaceTable, string rootNamespace) { string paramValue; if (type.IsEnum) { paramValue = type.FullName + "." + value; } else if (type.IsAssignableFrom(typeof(System.Type))) { XamlType typeName = XamlBuildTaskServices.GetXamlTypeFromString(value, namespaceTable, reader.SchemaContext); string clrTypeName; if (!XamlBuildTaskServices.TryGetClrTypeName(typeName, rootNamespace, out clrTypeName)) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.TypeNameUnknown(XamlBuildTaskServices.GetFullTypeName(typeName)))); } paramValue = clrTypeName; } else if (type == typeof(String)) { paramValue = value; } else if (type.IsPrimitive) { value = value.TrimStart('"'); value = value.TrimEnd('"'); if (type == typeof(bool)) { if (string.Compare(value, "true", StringComparison.OrdinalIgnoreCase) == 0) { paramValue = "true"; } else if (string.Compare(value, "false", StringComparison.OrdinalIgnoreCase) == 0) { paramValue = "false"; } else { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.UnknownBooleanValue(value))); } } else { paramValue = value; } } else { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.AttributeParamTypeNotSupported(type.FullName))); } return paramValue; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
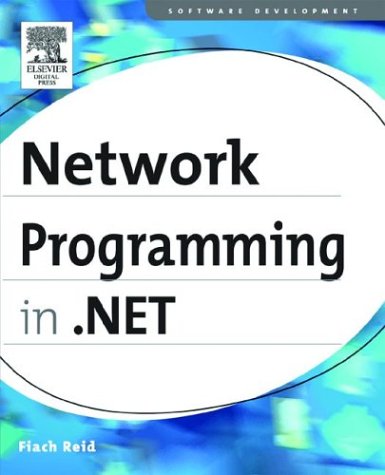
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Merger.cs
- DirectoryObjectSecurity.cs
- NotCondition.cs
- ZoomPercentageConverter.cs
- BaseAutoFormat.cs
- XmlDataProvider.cs
- InputEventArgs.cs
- ProfileGroupSettings.cs
- HttpFileCollection.cs
- XsltOutput.cs
- PrivilegedConfigurationManager.cs
- OdbcUtils.cs
- TextServicesCompartmentEventSink.cs
- filewebresponse.cs
- Membership.cs
- XsltSettings.cs
- XmlSchemaAnnotation.cs
- SerializationInfoEnumerator.cs
- Operand.cs
- UnsafeNativeMethodsMilCoreApi.cs
- X500Name.cs
- HttpResponseBase.cs
- PerfService.cs
- GraphicsState.cs
- WebPartCloseVerb.cs
- RadialGradientBrush.cs
- HandlerBase.cs
- PiiTraceSource.cs
- CqlQuery.cs
- MediaTimeline.cs
- ContentFilePart.cs
- FieldDescriptor.cs
- Exceptions.cs
- HttpBrowserCapabilitiesBase.cs
- CapabilitiesUse.cs
- RuleSettings.cs
- Selector.cs
- CryptoHelper.cs
- AnnotationResourceCollection.cs
- ContextBase.cs
- PrintController.cs
- OutOfProcStateClientManager.cs
- CodeMethodInvokeExpression.cs
- ResourceIDHelper.cs
- ImageFormatConverter.cs
- Zone.cs
- ReadContentAsBinaryHelper.cs
- Soap11ServerProtocol.cs
- RtfToXamlLexer.cs
- Button.cs
- Buffer.cs
- RadioButton.cs
- HostingEnvironment.cs
- SocketException.cs
- MenuStrip.cs
- UnionCodeGroup.cs
- MemoryMappedFileSecurity.cs
- RelatedPropertyManager.cs
- ModelUtilities.cs
- TargetParameterCountException.cs
- ConfigXmlElement.cs
- Mutex.cs
- PageClientProxyGenerator.cs
- EmbossBitmapEffect.cs
- CodeAttributeArgumentCollection.cs
- RecordsAffectedEventArgs.cs
- Image.cs
- RunInstallerAttribute.cs
- XmlNamespaceMapping.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- DBCSCodePageEncoding.cs
- ResourcePermissionBase.cs
- DesignerActionListCollection.cs
- listitem.cs
- AsyncOperation.cs
- NCryptSafeHandles.cs
- ComponentChangedEvent.cs
- ParagraphResult.cs
- WebSysDisplayNameAttribute.cs
- TrimSurroundingWhitespaceAttribute.cs
- WinEventHandler.cs
- XmlCharacterData.cs
- EarlyBoundInfo.cs
- NamespaceQuery.cs
- ChangePassword.cs
- ActivityMarkupSerializer.cs
- SafeRightsManagementSessionHandle.cs
- MetadataStore.cs
- InternalTypeHelper.cs
- DecodeHelper.cs
- AddInAttribute.cs
- DictionaryBase.cs
- CodeBlockBuilder.cs
- autovalidator.cs
- ProjectionNode.cs
- WebDisplayNameAttribute.cs
- UserMapPath.cs
- GridProviderWrapper.cs
- ToolStripPanelCell.cs
- NotificationContext.cs