Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Objects / FieldDescriptor.cs / 2 / FieldDescriptor.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Data.Common; using System.Data.Objects.DataClasses; using System.Data.Metadata.Edm; namespace System.Data.Objects { internal sealed class FieldDescriptor : PropertyDescriptor { private readonly EdmProperty _property; private readonly Type _fieldType; private readonly Type _componentType; private readonly bool _isReadOnly; ////// Construct a new instance of the FieldDescriptor class that describes a property /// on components of the supplied type. /// /// Type of object whose property is described by this FieldDescriptor. /// /// True if property value on component can be modified; otherwise false. /// /// /// EdmProperty that describes the property on the component. /// internal FieldDescriptor(Type componentType, bool isReadOnly, EdmProperty property) : base(property.Name, null) { _componentType = componentType; _property = property; _isReadOnly = isReadOnly; _fieldType = DetermineClrType(_property.TypeUsage); System.Diagnostics.Debug.Assert(_fieldType != null, "FieldDescriptor's CLR type has unexpected value of null."); } ////// Determine a CLR Type to use a property descriptro form an EDM TypeUsage /// /// The EDM TypeUsage containing metadata about the type ///A CLR type that represents that EDM type private Type DetermineClrType(TypeUsage typeUsage) { Type result = null; EdmType edmType = typeUsage.EdmType; switch (edmType.BuiltInTypeKind) { case BuiltInTypeKind.EntityType: case BuiltInTypeKind.ComplexType: result = edmType.ClrType; break; case BuiltInTypeKind.RefType: result = typeof(EntityKey); break; case BuiltInTypeKind.CollectionType: TypeUsage elementTypeUse = ((CollectionType)edmType).TypeUsage; result = DetermineClrType(elementTypeUse); result = typeof(IEnumerable<>).MakeGenericType(result); break; case BuiltInTypeKind.PrimitiveType: result = edmType.ClrType; Facet nullable; if (result.IsValueType && typeUsage.Facets.TryGetValue(DbProviderManifest.NullableFacetName, false, out nullable) && ((bool)nullable.Value)) { result = typeof(Nullable<>).MakeGenericType(result); } break; case BuiltInTypeKind.RowType: result = typeof(IDataRecord); break; default: throw EntityUtil.UnexpectedMetadataType(edmType); } return result; } ////// Get ///instance associated with this field descriptor. /// /// The internal EdmProperty EdmProperty { get { return _property; } } public override Type ComponentType { get { return _componentType; } } public override bool IsReadOnly { get { return _isReadOnly; } } public override Type PropertyType { get { return _fieldType; } } public override bool CanResetValue(object component) { return false; } public override object GetValue(object component) { EntityUtil.CheckArgumentNull(component, "component"); if (!_componentType.IsAssignableFrom(component.GetType())) { throw EntityUtil.IncompatibleArgument(); } object propertyValue; if (component is IEntityWithChangeTracker) //POCO will relax this requirement. { propertyValue = LightweightCodeGenerator.GetValue(_property, component); } else { DbDataRecord dbDataRecord = component as DbDataRecord; if (dbDataRecord != null) { propertyValue = (dbDataRecord.GetValue(dbDataRecord.GetOrdinal(_property.Name))); } else { throw EntityUtil.NotSupported(); } } return propertyValue; } public override void ResetValue(object component) { throw EntityUtil.NotSupported(); } public override void SetValue(object component, object value) { EntityUtil.CheckArgumentNull(component, "component"); if (!_componentType.IsAssignableFrom(component.GetType())) { throw EntityUtil.IncompatibleArgument(); } if (!_isReadOnly && component is IEntityWithChangeTracker) //POCO will relax this requirement { LightweightCodeGenerator.SetValue(_property, component, value); } // if not entity it must be readonly else { throw EntityUtil.WriteOperationNotAllowedOnReadOnlyBindingList(); } } public override bool ShouldSerializeValue(object component) { return false; } public override bool IsBrowsable { get { return true; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //instance associated with this field descriptor, /// or null if there is no EDM property association. /// // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Data.Common; using System.Data.Objects.DataClasses; using System.Data.Metadata.Edm; namespace System.Data.Objects { internal sealed class FieldDescriptor : PropertyDescriptor { private readonly EdmProperty _property; private readonly Type _fieldType; private readonly Type _componentType; private readonly bool _isReadOnly; ////// Construct a new instance of the FieldDescriptor class that describes a property /// on components of the supplied type. /// /// Type of object whose property is described by this FieldDescriptor. /// /// True if property value on component can be modified; otherwise false. /// /// /// EdmProperty that describes the property on the component. /// internal FieldDescriptor(Type componentType, bool isReadOnly, EdmProperty property) : base(property.Name, null) { _componentType = componentType; _property = property; _isReadOnly = isReadOnly; _fieldType = DetermineClrType(_property.TypeUsage); System.Diagnostics.Debug.Assert(_fieldType != null, "FieldDescriptor's CLR type has unexpected value of null."); } ////// Determine a CLR Type to use a property descriptro form an EDM TypeUsage /// /// The EDM TypeUsage containing metadata about the type ///A CLR type that represents that EDM type private Type DetermineClrType(TypeUsage typeUsage) { Type result = null; EdmType edmType = typeUsage.EdmType; switch (edmType.BuiltInTypeKind) { case BuiltInTypeKind.EntityType: case BuiltInTypeKind.ComplexType: result = edmType.ClrType; break; case BuiltInTypeKind.RefType: result = typeof(EntityKey); break; case BuiltInTypeKind.CollectionType: TypeUsage elementTypeUse = ((CollectionType)edmType).TypeUsage; result = DetermineClrType(elementTypeUse); result = typeof(IEnumerable<>).MakeGenericType(result); break; case BuiltInTypeKind.PrimitiveType: result = edmType.ClrType; Facet nullable; if (result.IsValueType && typeUsage.Facets.TryGetValue(DbProviderManifest.NullableFacetName, false, out nullable) && ((bool)nullable.Value)) { result = typeof(Nullable<>).MakeGenericType(result); } break; case BuiltInTypeKind.RowType: result = typeof(IDataRecord); break; default: throw EntityUtil.UnexpectedMetadataType(edmType); } return result; } ////// Get ///instance associated with this field descriptor. /// /// The internal EdmProperty EdmProperty { get { return _property; } } public override Type ComponentType { get { return _componentType; } } public override bool IsReadOnly { get { return _isReadOnly; } } public override Type PropertyType { get { return _fieldType; } } public override bool CanResetValue(object component) { return false; } public override object GetValue(object component) { EntityUtil.CheckArgumentNull(component, "component"); if (!_componentType.IsAssignableFrom(component.GetType())) { throw EntityUtil.IncompatibleArgument(); } object propertyValue; if (component is IEntityWithChangeTracker) //POCO will relax this requirement. { propertyValue = LightweightCodeGenerator.GetValue(_property, component); } else { DbDataRecord dbDataRecord = component as DbDataRecord; if (dbDataRecord != null) { propertyValue = (dbDataRecord.GetValue(dbDataRecord.GetOrdinal(_property.Name))); } else { throw EntityUtil.NotSupported(); } } return propertyValue; } public override void ResetValue(object component) { throw EntityUtil.NotSupported(); } public override void SetValue(object component, object value) { EntityUtil.CheckArgumentNull(component, "component"); if (!_componentType.IsAssignableFrom(component.GetType())) { throw EntityUtil.IncompatibleArgument(); } if (!_isReadOnly && component is IEntityWithChangeTracker) //POCO will relax this requirement { LightweightCodeGenerator.SetValue(_property, component, value); } // if not entity it must be readonly else { throw EntityUtil.WriteOperationNotAllowedOnReadOnlyBindingList(); } } public override bool ShouldSerializeValue(object component) { return false; } public override bool IsBrowsable { get { return true; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.instance associated with this field descriptor, /// or null if there is no EDM property association. ///
Link Menu
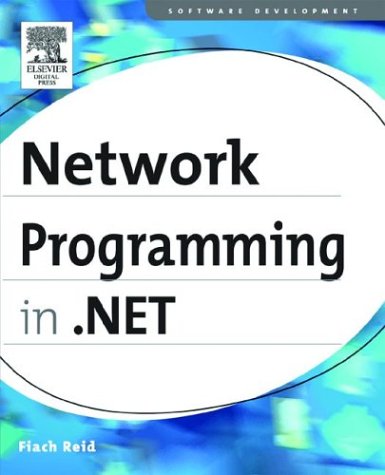
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlCharCheckingWriter.cs
- ArrayExtension.cs
- ContentValidator.cs
- DirtyTextRange.cs
- EventPrivateKey.cs
- Ipv6Element.cs
- LayeredChannelFactory.cs
- DataColumn.cs
- InvalidWMPVersionException.cs
- Compensate.cs
- Lease.cs
- StringCollectionMarkupSerializer.cs
- ColorContext.cs
- listviewsubitemcollectioneditor.cs
- CodeArgumentReferenceExpression.cs
- HttpResponse.cs
- CursorConverter.cs
- EventLogHandle.cs
- RayMeshGeometry3DHitTestResult.cs
- ImmutableObjectAttribute.cs
- PropertyBuilder.cs
- TranslateTransform.cs
- OleDbErrorCollection.cs
- ObjectQueryState.cs
- DesignerAdRotatorAdapter.cs
- AudioDeviceOut.cs
- ControlType.cs
- ServiceDescriptionData.cs
- NotifyParentPropertyAttribute.cs
- JoinSymbol.cs
- HelloMessageCD1.cs
- ZipIOModeEnforcingStream.cs
- panel.cs
- TypeElementCollection.cs
- CapiSafeHandles.cs
- SecuritySessionServerSettings.cs
- Converter.cs
- PageContentAsyncResult.cs
- TaiwanLunisolarCalendar.cs
- ToolStripManager.cs
- DataPagerCommandEventArgs.cs
- MenuCommands.cs
- _NtlmClient.cs
- HttpPostedFile.cs
- FormViewPageEventArgs.cs
- DropSource.cs
- LicenseContext.cs
- GeometryHitTestParameters.cs
- PictureBox.cs
- ReferencedCollectionType.cs
- RichTextBox.cs
- OneWayChannelListener.cs
- ValidatorCollection.cs
- GenericUriParser.cs
- DayRenderEvent.cs
- ApplicationFileParser.cs
- XmlBoundElement.cs
- DataGridTextBox.cs
- WhiteSpaceTrimStringConverter.cs
- DbProviderFactoriesConfigurationHandler.cs
- UrlAuthorizationModule.cs
- Panel.cs
- StaticExtensionConverter.cs
- XPathNodePointer.cs
- DataListCommandEventArgs.cs
- InvalidContentTypeException.cs
- XmlEntity.cs
- ColorMap.cs
- OdbcConnectionOpen.cs
- DeclaredTypeValidatorAttribute.cs
- TableChangeProcessor.cs
- SoapSchemaMember.cs
- RuntimeHelpers.cs
- QilStrConcatenator.cs
- RtType.cs
- ZoneLinkButton.cs
- PermissionToken.cs
- ReadOnlyAttribute.cs
- RadioButtonFlatAdapter.cs
- PrintDialog.cs
- Fault.cs
- WinEventQueueItem.cs
- SQLInt32Storage.cs
- LongSumAggregationOperator.cs
- BuilderPropertyEntry.cs
- InvokeHandlers.cs
- ExecutedRoutedEventArgs.cs
- sqlstateclientmanager.cs
- ListSortDescriptionCollection.cs
- PackageProperties.cs
- MemoryStream.cs
- CollectionContainer.cs
- Message.cs
- InkCanvasFeedbackAdorner.cs
- SerializationFieldInfo.cs
- DBBindings.cs
- BamlLocalizableResourceKey.cs
- BindUriHelper.cs
- FormViewPageEventArgs.cs
- BaseDataList.cs