Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Data / System / Data / Common / DbProviderFactoriesConfigurationHandler.cs / 1 / DbProviderFactoriesConfigurationHandler.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.Common { using System; using System.Collections; using System.Configuration; using System.Data; using System.Diagnostics; using System.Globalization; using System.Xml; //// // //// // this class is delayed created, use ConfigurationSettings.GetSection("system.data") to obtain #if WINFSInternalOnly internal #else public #endif class DbProviderFactoriesConfigurationHandler : IConfigurationSectionHandler { // V1.2.3300 internal const string sectionName = "system.data"; internal const string providerGroup = "DbProviderFactories"; public DbProviderFactoriesConfigurationHandler() { // V1.2.3300 } virtual public object Create(object parent, object configContext, XmlNode section) { // V1.2.3300 #if DEBUG try { #endif return CreateStatic(parent, configContext, section); #if DEBUG } catch(Exception e) { ADP.TraceExceptionWithoutRethrow(e); // it will be rethrown throw; } #endif } static internal object CreateStatic(object parent, object configContext, XmlNode section) { object config = parent; if (null != section) { config = HandlerBase.CloneParent(parent as DataSet, false); bool foundFactories = false; HandlerBase.CheckForUnrecognizedAttributes(section); foreach (XmlNode child in section.ChildNodes) { if (HandlerBase.IsIgnorableAlsoCheckForNonElement(child)) { continue; } string sectionGroup = child.Name; switch(sectionGroup) { case DbProviderFactoriesConfigurationHandler.providerGroup: if (foundFactories) { throw ADP.ConfigSectionsUnique(DbProviderFactoriesConfigurationHandler.providerGroup); } foundFactories = true; HandleProviders(config as DataSet, configContext, child, sectionGroup); break; default: throw ADP.ConfigUnrecognizedElement(child); } } } return config; } // sectionName - i.e. "providerconfiguration" private static void HandleProviders(DataSet config, object configContext, XmlNode section, string sectionName) { DataTableCollection tables = config.Tables; DataTable dataTable = tables[sectionName]; bool tableExisted = (null != dataTable); dataTable = DbProviderDictionarySectionHandler.CreateStatic(dataTable, configContext, section); if (!tableExisted) { tables.Add(dataTable); } } // based off of DictionarySectionHandler private static class DbProviderDictionarySectionHandler/* : IConfigurationSectionHandler*/ { /* internal DbProviderDictionarySectionHandler() { } public object Create(Object parent, Object context, XmlNode section) { return CreateStatic(parent, context, section); } */ static internal DataTable CreateStatic(DataTable config, Object context, XmlNode section) { if (null != section) { HandlerBase.CheckForUnrecognizedAttributes(section); if (null == config) { config = DbProviderFactoriesConfigurationHandler.CreateProviderDataTable(); } // else already copied via DataSet.Copy foreach (XmlNode child in section.ChildNodes) { if (HandlerBase.IsIgnorableAlsoCheckForNonElement(child)) { continue; } switch(child.Name) { case "add": HandleAdd(child, config); break; case "remove": HandleRemove(child, config); break; case "clear": HandleClear(child, config); break; default: throw ADP.ConfigUnrecognizedElement(child); } } config.AcceptChanges(); } return config; } static private void HandleAdd(XmlNode child, DataTable config) { HandlerBase.CheckForChildNodes(child); DataRow values = config.NewRow(); values[0] = HandlerBase.RemoveAttribute(child, "name", true, false); values[1] = HandlerBase.RemoveAttribute(child, "description", true, false); values[2] = HandlerBase.RemoveAttribute(child, "invariant", true, false); values[3] = HandlerBase.RemoveAttribute(child, "type", true, false); // because beta shipped recognizing "support=hex#", need to give // more time for other providers to remove it from the .config files HandlerBase.RemoveAttribute(child, "support", false, false); HandlerBase.CheckForUnrecognizedAttributes(child); config.Rows.Add(values); } static private void HandleRemove(XmlNode child, DataTable config) { HandlerBase.CheckForChildNodes(child); String invr = HandlerBase.RemoveAttribute(child, "invariant", true, false); HandlerBase.CheckForUnrecognizedAttributes(child); DataRow row = config.Rows.Find(invr); if (null != row) { // ignore invariants that don't exist row.Delete(); } } static private void HandleClear(XmlNode child, DataTable config) { HandlerBase.CheckForChildNodes(child); HandlerBase.CheckForUnrecognizedAttributes(child); config.Clear(); } } internal static DataTable CreateProviderDataTable() { DataColumn frme = new DataColumn("Name", typeof(string)); frme.ReadOnly = true; DataColumn desc = new DataColumn("Description", typeof(string)); desc.ReadOnly = true; DataColumn invr = new DataColumn("InvariantName", typeof(string)); invr.ReadOnly = true; DataColumn qual = new DataColumn("AssemblyQualifiedName", typeof(string)); qual.ReadOnly = true; DataColumn[] primaryKey = new DataColumn[] { invr }; DataColumn[] columns = new DataColumn[] {frme, desc, invr, qual }; DataTable table = new DataTable(DbProviderFactoriesConfigurationHandler.providerGroup); table.Locale = CultureInfo.InvariantCulture; table.Columns.AddRange(columns); table.PrimaryKey = primaryKey; return table; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.// //// // // //
Link Menu
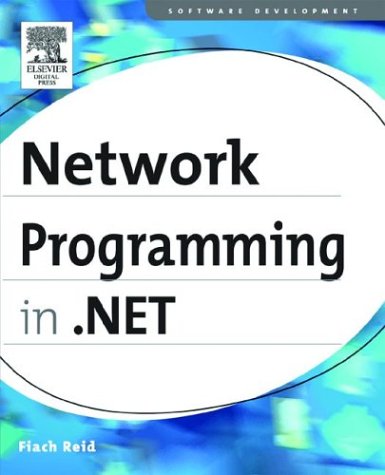
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ItemDragEvent.cs
- GcSettings.cs
- PairComparer.cs
- Binding.cs
- ToolStripItemClickedEventArgs.cs
- TextParagraphProperties.cs
- NumericUpDownAccelerationCollection.cs
- OracleInfoMessageEventArgs.cs
- AppSettingsExpressionBuilder.cs
- DeviceFiltersSection.cs
- BookmarkInfo.cs
- VisualBrush.cs
- QueryOperationResponseOfT.cs
- PermissionRequestEvidence.cs
- SqlServices.cs
- HostAdapter.cs
- PassportAuthenticationModule.cs
- BitmapEncoder.cs
- EditorZoneBase.cs
- FixUpCollection.cs
- RectAnimationBase.cs
- Code.cs
- AxImporter.cs
- PrefixQName.cs
- AspCompat.cs
- MetafileHeader.cs
- XmlStreamedByteStreamReader.cs
- ProfileProvider.cs
- TouchEventArgs.cs
- ValueOfAction.cs
- ElementNotAvailableException.cs
- ConnectionPointConverter.cs
- HttpModuleAction.cs
- CLRBindingWorker.cs
- InternalsVisibleToAttribute.cs
- EdmToObjectNamespaceMap.cs
- GradientSpreadMethodValidation.cs
- EventProxy.cs
- DataColumnPropertyDescriptor.cs
- SignedPkcs7.cs
- DataGridPagingPage.cs
- DoubleIndependentAnimationStorage.cs
- ConnectionManagementSection.cs
- SequentialOutput.cs
- InplaceBitmapMetadataWriter.cs
- ProjectionCamera.cs
- DropShadowBitmapEffect.cs
- UIElement.cs
- TrackingProfileCache.cs
- CaseInsensitiveHashCodeProvider.cs
- Dynamic.cs
- NumberFormatInfo.cs
- FuncCompletionCallbackWrapper.cs
- ScriptReferenceEventArgs.cs
- XmlEnumAttribute.cs
- EventLogWatcher.cs
- SerializationInfo.cs
- CryptoConfig.cs
- FormViewUpdatedEventArgs.cs
- Transform3D.cs
- PerformanceCounterPermissionAttribute.cs
- StreamingContext.cs
- XmlIterators.cs
- FileUtil.cs
- diagnosticsswitches.cs
- PageParserFilter.cs
- ReferenceEqualityComparer.cs
- HandledMouseEvent.cs
- XmlUtil.cs
- PropertyDescriptors.cs
- SchemaCreator.cs
- ParseNumbers.cs
- TriState.cs
- RemoveStoryboard.cs
- NativeMethods.cs
- ColumnMap.cs
- ProtocolImporter.cs
- AttachedPropertyBrowsableAttribute.cs
- DesignerActionUIService.cs
- HttpRequestCacheValidator.cs
- ProtocolsConfigurationEntry.cs
- XmlEncodedRawTextWriter.cs
- NotSupportedException.cs
- WsiProfilesElementCollection.cs
- MainMenu.cs
- RightsDocument.cs
- ResolvePPIDRequest.cs
- ManagedIStream.cs
- CertificateManager.cs
- QueryOutputWriter.cs
- InstanceCompleteException.cs
- LZCodec.cs
- ToolStripItemCollection.cs
- BindingCompleteEventArgs.cs
- XmlSchemaGroupRef.cs
- Help.cs
- HttpCookieCollection.cs
- ListSortDescriptionCollection.cs
- SqlClientPermission.cs
- ListParagraph.cs