Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Services / Monitoring / system / Diagnosticts / CounterSample.cs / 1 / CounterSample.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Diagnostics { using System.Diagnostics; using System; ////// A struct holding the raw data for a performance counter. /// public struct CounterSample { private long rawValue; private long baseValue; private long timeStamp; private long counterFrequency; private PerformanceCounterType counterType; private long timeStamp100nSec; private long systemFrequency; private long counterTimeStamp; // Dummy holder for an empty sample ////// public static CounterSample Empty = new CounterSample(0, 0, 0, 0, 0, 0, PerformanceCounterType.NumberOfItems32); ///[To be supplied.] ////// public CounterSample(long rawValue, long baseValue, long counterFrequency, long systemFrequency, long timeStamp, long timeStamp100nSec, PerformanceCounterType counterType) { this.rawValue = rawValue; this.baseValue = baseValue; this.timeStamp = timeStamp; this.counterFrequency = counterFrequency; this.counterType = counterType; this.timeStamp100nSec = timeStamp100nSec; this.systemFrequency = systemFrequency; this.counterTimeStamp = 0; } ///[To be supplied.] ////// public CounterSample(long rawValue, long baseValue, long counterFrequency, long systemFrequency, long timeStamp, long timeStamp100nSec, PerformanceCounterType counterType, long counterTimeStamp) { this.rawValue = rawValue; this.baseValue = baseValue; this.timeStamp = timeStamp; this.counterFrequency = counterFrequency; this.counterType = counterType; this.timeStamp100nSec = timeStamp100nSec; this.systemFrequency = systemFrequency; this.counterTimeStamp = counterTimeStamp; } ///[To be supplied.] ////// Raw value of the counter. /// public long RawValue { get { return this.rawValue; } } internal ulong UnsignedRawValue { get { return (ulong)this.rawValue; } } ////// Optional base raw value for the counter (only used if multiple counter based). /// public long BaseValue { get { return this.baseValue; } } ////// Raw system frequency /// public long SystemFrequency { get { return this.systemFrequency; } } ////// Raw counter frequency /// public long CounterFrequency { get { return this.counterFrequency; } } ////// Raw counter frequency /// public long CounterTimeStamp { get { return this.counterTimeStamp; } } ////// Raw timestamp /// public long TimeStamp { get { return this.timeStamp; } } ////// Raw high fidelity timestamp /// public long TimeStamp100nSec { get { return this.timeStamp100nSec; } } ////// Counter type /// public PerformanceCounterType CounterType { get { return this.counterType; } } ////// Static functions to calculate the performance value off the sample /// public static float Calculate(CounterSample counterSample) { return CounterSampleCalculator.ComputeCounterValue(counterSample); } ////// Static functions to calculate the performance value off the samples /// public static float Calculate(CounterSample counterSample, CounterSample nextCounterSample) { return CounterSampleCalculator.ComputeCounterValue(counterSample, nextCounterSample); } public override bool Equals(Object o) { return ( o is CounterSample) && Equals((CounterSample)o); } public bool Equals(CounterSample sample) { return (rawValue == sample.rawValue) && (baseValue == sample.baseValue) && (timeStamp == sample.timeStamp) && (counterFrequency == sample.counterFrequency) && (counterType == sample.counterType) && (timeStamp100nSec == sample.timeStamp100nSec) && (systemFrequency == sample.systemFrequency) && (counterTimeStamp == sample.counterTimeStamp); } public override int GetHashCode() { return rawValue.GetHashCode(); } public static bool operator ==(CounterSample a, CounterSample b) { return a.Equals(b); } public static bool operator !=(CounterSample a, CounterSample b) { return !(a.Equals(b)); } } }
Link Menu
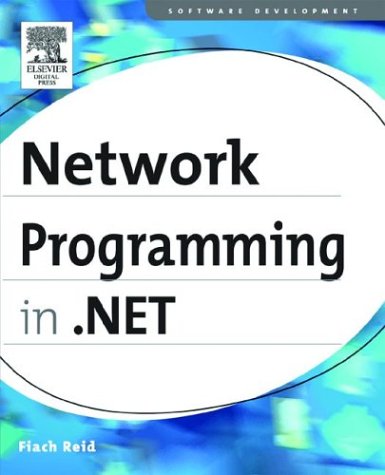
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridTableCollection.cs
- HttpRequest.cs
- ParameterCollection.cs
- BoundColumn.cs
- WhitespaceRule.cs
- FormattedText.cs
- SingleAnimationUsingKeyFrames.cs
- ParseNumbers.cs
- PlainXmlDeserializer.cs
- HtmlInputReset.cs
- DefaultDiscoveryServiceExtension.cs
- HtmlTable.cs
- HostedHttpRequestAsyncResult.cs
- SqlNodeTypeOperators.cs
- RotationValidation.cs
- LineMetrics.cs
- CssTextWriter.cs
- FixedStringLookup.cs
- RtfToXamlReader.cs
- ADConnectionHelper.cs
- TableColumnCollectionInternal.cs
- SoapReflectionImporter.cs
- BaseTemplateParser.cs
- XmlSchemaSimpleTypeRestriction.cs
- KeyInfo.cs
- PropertyTabChangedEvent.cs
- BaseProcessProtocolHandler.cs
- DrawingCollection.cs
- WindowsFormsSectionHandler.cs
- DataServiceHostFactory.cs
- MergeExecutor.cs
- Visual3D.cs
- IPipelineRuntime.cs
- PixelFormats.cs
- PEFileEvidenceFactory.cs
- __Filters.cs
- ErrorHandler.cs
- Line.cs
- ToolTipService.cs
- OleDbWrapper.cs
- NamespaceMapping.cs
- Native.cs
- Rect3D.cs
- HandlerWithFactory.cs
- TableRowGroup.cs
- MenuBase.cs
- AutomationAttributeInfo.cs
- TemplatedAdorner.cs
- TextFormatterContext.cs
- DataTableTypeConverter.cs
- SpecialFolderEnumConverter.cs
- AppLevelCompilationSectionCache.cs
- PartialList.cs
- BitmapEffectGroup.cs
- PartialTrustVisibleAssemblyCollection.cs
- ISessionStateStore.cs
- DesignerDataSourceView.cs
- ScrollChrome.cs
- SpeechDetectedEventArgs.cs
- TraceSection.cs
- ToolStripScrollButton.cs
- NativeObjectSecurity.cs
- DateTimeUtil.cs
- SemanticValue.cs
- QuaternionValueSerializer.cs
- XmlResolver.cs
- WeakKeyDictionary.cs
- WebServiceResponse.cs
- Single.cs
- DocumentReference.cs
- ConfigPathUtility.cs
- Char.cs
- SpnEndpointIdentity.cs
- MenuBindingsEditor.cs
- Crc32Helper.cs
- Condition.cs
- VoiceObjectToken.cs
- ModuleBuilderData.cs
- PeerName.cs
- RbTree.cs
- InputReportEventArgs.cs
- HttpHostedTransportConfiguration.cs
- DataMisalignedException.cs
- BitmapImage.cs
- ECDiffieHellman.cs
- IsolatedStorage.cs
- FrameworkElementFactory.cs
- MessageQueuePermissionEntry.cs
- KeyedHashAlgorithm.cs
- DocumentManager.cs
- XmlEntity.cs
- oledbconnectionstring.cs
- SafeRightsManagementPubHandle.cs
- MessageEnumerator.cs
- CryptoProvider.cs
- GridPattern.cs
- FileInfo.cs
- MessageDecoder.cs
- CompositeScriptReferenceEventArgs.cs
- ObjectStateFormatter.cs